图片文件与字节数组字符串互转(Base64编解码)
1.图片文件转化为字节数组字符串,并对其进行Base64编码处理
参数imgFile:图片文件
// 将图片文件转化为字节数组字符串,并对其进行Base64编码处理
public static String imageToBase64ByLocal(String imgFile) {
try {
InputStream in = null;
byte[] data = null;
// 读取图片字节数组
in = new FileInputStream(imgFile);
data = new byte[in.available()];
in.read(data);
in.close();
// 对字节数组Base64编码
BASE64Encoder encoder = new BASE64Encoder();
// 返回Base64编码过的字节数组字符串
return encoder.encode(data);
} catch (IOException e) {
System.out.println(e);
}
return null;
}
运行结果:

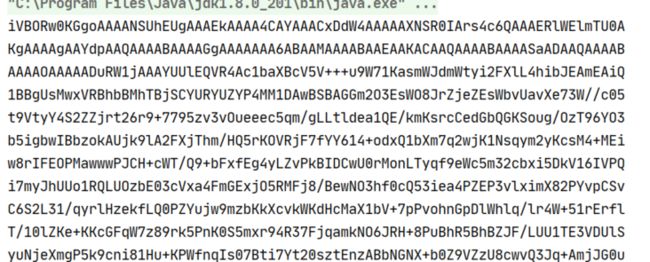
2.字节数组字符串转换为图片文件,并进行Base64解码
参数imgStr:待转换的字节数组字符串
参数imgFilePath:转换后的图片存放地址
public static boolean base64ToImage(String imgStr, String imgFilePath) { // 对字节数组字符串进行Base64解码并生成图片
// 图像数据为空
if (isEmpty(imgStr)) {
return false;
}
try {
BASE64Decoder decoder = new BASE64Decoder();
// Base64解码
byte[] b = decoder.decodeBuffer(imgStr);
for (int i = 0; i < b.length; ++i) {
if (b[i] < 0) {
// 调整异常数据
b[i] += 256;
}
}
OutputStream out = new FileOutputStream(imgFilePath);
out.write(b);
out.flush();
out.close();
return true;
} catch (Exception e) {
System.out.println(e);
}
return false;
}
private static boolean isEmpty(String input) {
return input == null || "".equals(input);
}
运行结果:
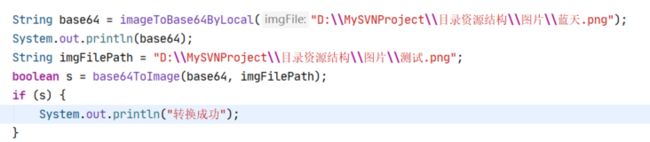

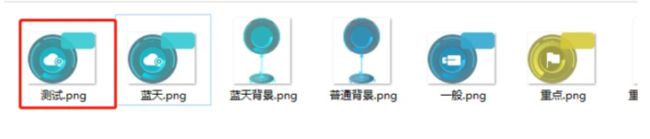
图片上传云存储,返回url
将图片上传至云存储,返回图片的url
/**
* 上传图片到云存储,返回url和图片名称
* @param multipartFile
* @return
*/
@Override
public BaseResponse
测试:
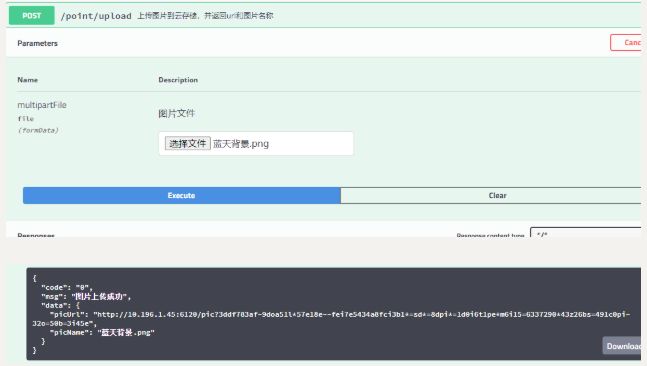