一、类中函数的重定义
#include
#include
#include"Shape.h"
#include"rectangle.h"
#include"circle.h"
int main()
{
Shape s1{ Color::blue,false };
Circle c1{ 3.9,Color::green,true };
Rectangle r1{ 4.0,1.0,Color::white,true };
std::cout << s1.toString() << std::endl;
std::cout << c1.toString() << std::endl;
std::cout << r1.toString() << std::endl;
std::cout << "c1 area:" << c1.getArea() << std::endl;
std::cout << "r1 area:" << r1.getarea() << std::endl;
std::cin.get();
}
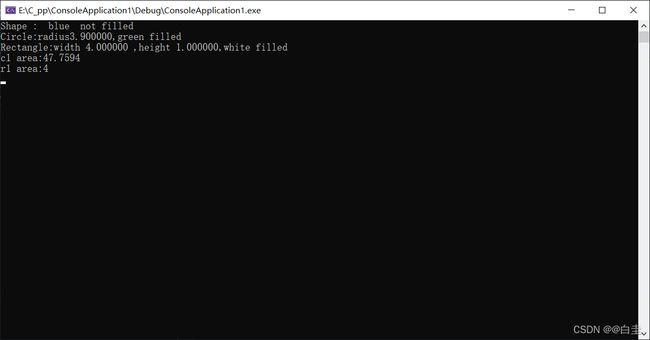
结果如上图所示。
二、相关文件
#include "Shape.h"
Shape::Shape(Color color_, bool filled_)
{
color = color_;
filled = filled_;
}
Color Shape::getColor() { return color; }
void Shape::setColor(Color color_) { color = color_; }
bool Shape::isFilled() { return filled; }
void Shape::setFilled(bool filled_) { filled = filled_; }
string Shape::toString()
{
std::array<string, 6> c{ "white"s,"black"s, "red"s, "green"s, "blue"s, "yellow"s, };
return "Shape : " + c[static_cast<int>(color)] + " " + (filled ? "filled"s : "not filled"s);
}
string Shape::colorTostring()
{
return colorNames[static_cast<int>(color)];
}
string Shape::filledToString()
{
return (filled ? "filled"s : "not filled"s);
}
#include"rectangle.h"
Rectangle::Rectangle(double w, double h, Color c, bool f) :width{ w }, height{ h }, Shape{c,f}{}
double Rectangle::getWidth() const
{
return width;
}
void Rectangle::setWidth(double width)
{
this->width = width;
}
double Rectangle::getHeight() const
{
return height;
}
void Rectangle::setHeight(double height)
{
this->height = height;
}
double Rectangle::getarea()
{
return width * height;
}
string Rectangle::toString()
{
return("Rectangle:width " + std::to_string(width) + " ," + "height " + std::to_string(height) + "," +
colorTostring() + " " + filledToString());
}
#include"circle.h"
Circle::Circle()
{
radius = 1.0;
}
Circle::Circle(double radius_, Color color_, bool filled_) :Shape{ color_, filled_ }
{
radius = radius_;
}
double Circle::getArea()
{
return (3.14 * radius * radius);
}
double Circle::getRadius() const
{
return radius;
}
Circle& Circle::setRadius(double radius)
{
this->radius = radius;
return (*this);
}
string Circle::toString()
{
return ("Circle:radius" + std::to_string(radius) + "," + colorTostring() + " " + filledToString());
}
#pragma once
#include"Shape.h"
class Circle:public Shape
{
private:
double radius;
public:
Circle();
Circle(double radius_, Color color_, bool filled_);
double getArea();
public:
double getRadius() const;
Circle& setRadius(double radius);
string toString();
};
#pragma once
#include
#include
#include
using std::string;
using namespace std::string_literals;
enum class Color
{
white,black,red,green,blue,yellow,
};
class Shape
{
private:
Color color{ Color::black };
bool filled{ false };
std::array<string, 6>colorNames{ "white"s,"blcak"s, "red"s, "green"s, "blue"s, "yellow"s, };
public:
Shape() = default;
Shape(Color color_, bool filled_);
Color getColor();
void setColor(Color color_);
bool isFilled();
void setFilled(bool filled_);
string filledToString();
string colorTostring();
string toString();
};
#pragma once
#include"Shape.h"
class Rectangle : public Shape
{
private:
double width{ 1.0 };
double height{ 1.0 };
public:
Rectangle() = default;
Rectangle(double w, double h,Color c,bool f);
double getWidth() const;
void setWidth(double width);
double getHeight() const;
void setHeight(double height);
double getarea();
string toString();
};