贪心算法思想
- leetcode455. 分发饼干
- leetcode1005. K 次取反后最大化的数组和
- leetcode376. 摆动序列
- leetcode738. 单调递增的数字
- leetcode122. 买卖股票的最佳时机 II
- leetcode714. 买卖股票的最佳时机含手续费
- leetcode406. 根据身高重建队列
- leetcode135. 分发糖果
- leetcode55. 跳跃游戏
- leetcode45. 跳跃游戏 II
- leetcode435. 无重叠区间
- leetcode452. 用最少数量的箭引爆气球
- leetcode968. 监控二叉树
- leetcode134. 加油站
leetcode455. 分发饼干
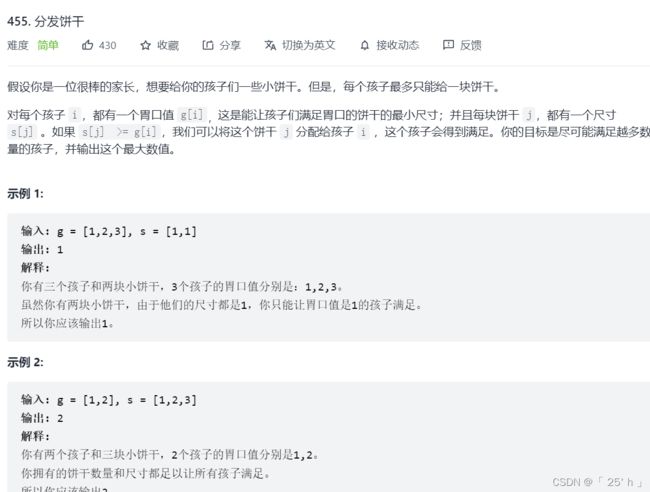
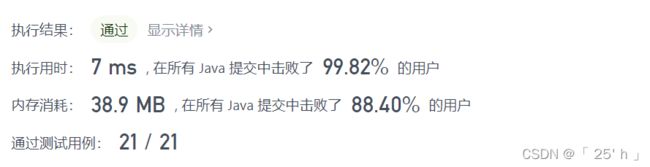
class Solution {
public int findContentChildren(int[] g, int[] s) {
Arrays.sort(g);
Arrays.sort(s);
int childIndex = 0, cakeIndex = 0;
while (childIndex < g.length) {
while (cakeIndex < s.length && g[childIndex] > s[cakeIndex]) {
cakeIndex++;
}
if (cakeIndex == s.length) return childIndex;
childIndex++;
cakeIndex++;
}
return g.length;
}
}
leetcode1005. K 次取反后最大化的数组和
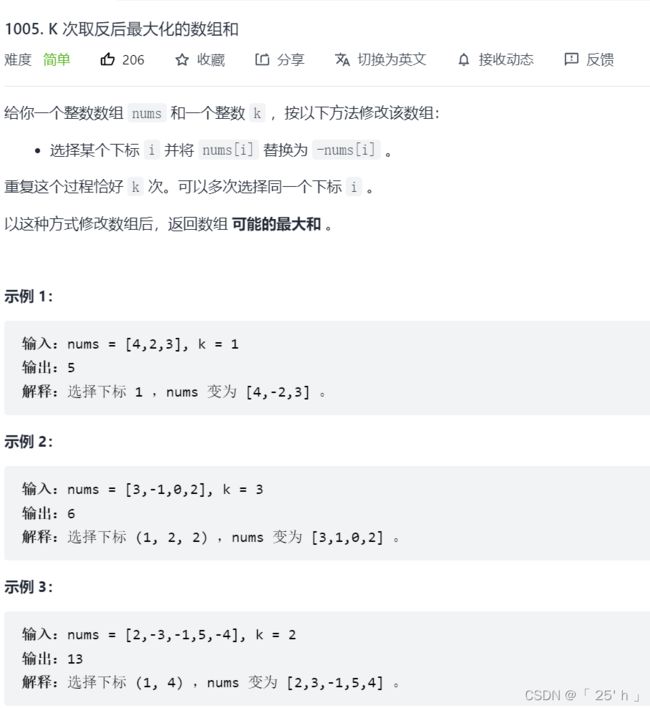
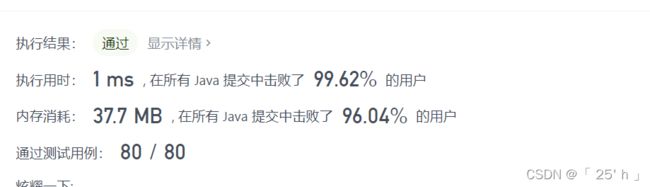
class Solution {
public int largestSumAfterKNegations(int[] nums, int k) {
Arrays.sort(nums);
int sum = 0, i;
for (i = 0; i < nums.length && nums[i] < 0 && i < k; i++) {
nums[i] = -nums[i];
}
Arrays.sort(nums);
for (int val : nums) sum += val;
if (((k - i) & 1) == 0) return sum;
return sum - 2 * nums[0];
}
}
leetcode376. 摆动序列
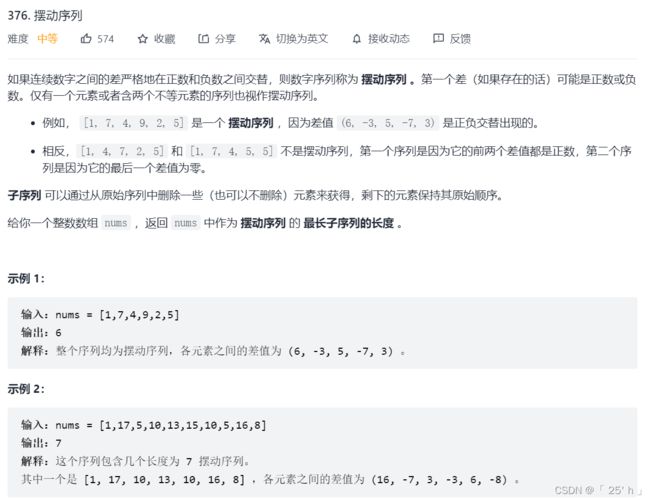
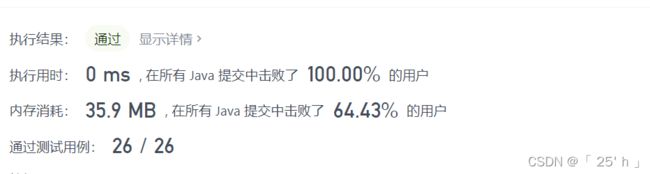
class Solution {
public int wiggleMaxLength(int[] nums) {
if (nums.length <= 1) return nums.length;
return Math.max(process(nums, true), process(nums, false));
}
private int process(int[] nums, boolean flag) {
int i = 1, res = 0;
while (i < nums.length) {
if (flag) while (i < nums.length && nums[i] >= nums[i - 1]) i++;
else while (i < nums.length && nums[i] <= nums[i - 1]) i++;
flag = !flag;
res++;
}
return res;
}
}
leetcode738. 单调递增的数字
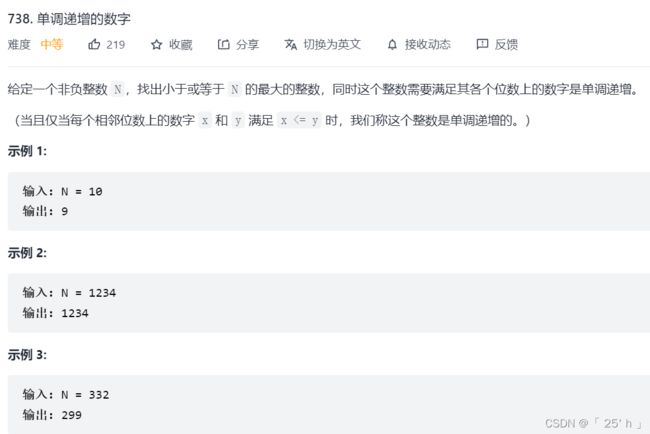
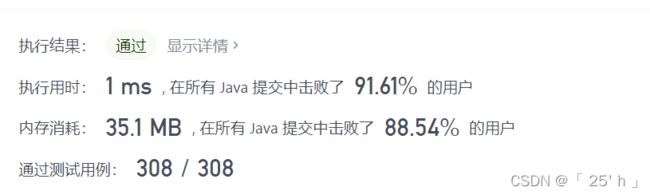
class Solution {
public int monotoneIncreasingDigits(int N) {
String num = String.valueOf(N);
char[] arr = num.toCharArray();
int len = arr.length;
if (len < 2) return N;
int pre = len;
for (int i = len - 2; i >= 0; i--) {
if (arr[i] > arr[i + 1]) {
arr[i]--;
for (int j = i + 1; j < pre; j++) {
arr[j] = '9';
}
pre = i + 1;
}
}
return Integer.parseInt(new String(arr));
}
}
leetcode122. 买卖股票的最佳时机 II
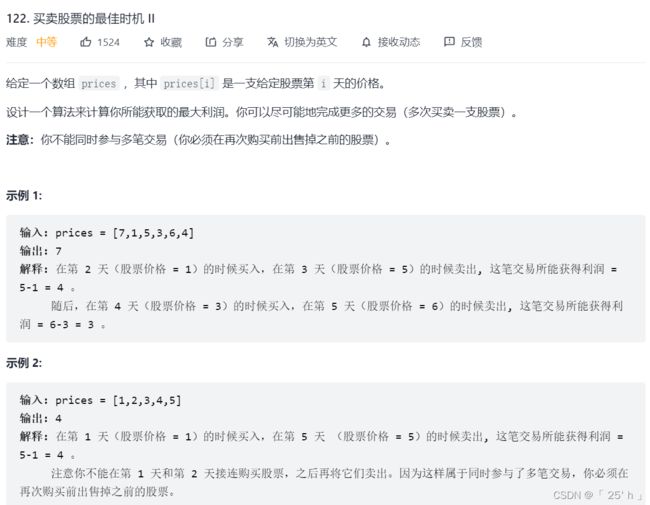
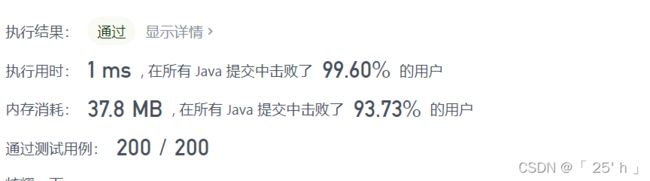
贪心题目思路讲解
class Solution {
public int maxProfit(int[] prices) {
int res = 0;
for (int i = 0; i < prices.length - 1; i++) {
if (prices[i + 1] > prices[i]) {
res += prices[i + 1] - prices[i];
}
}
return res;
}
}
leetcode714. 买卖股票的最佳时机含手续费
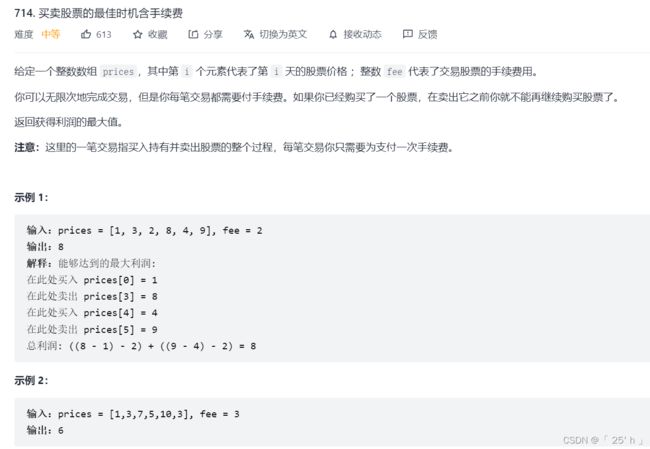
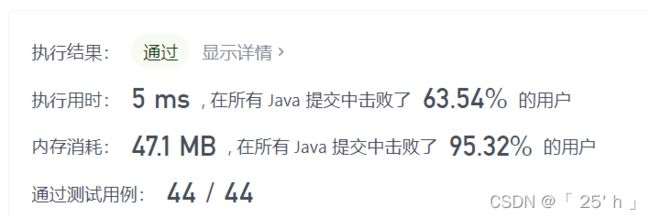
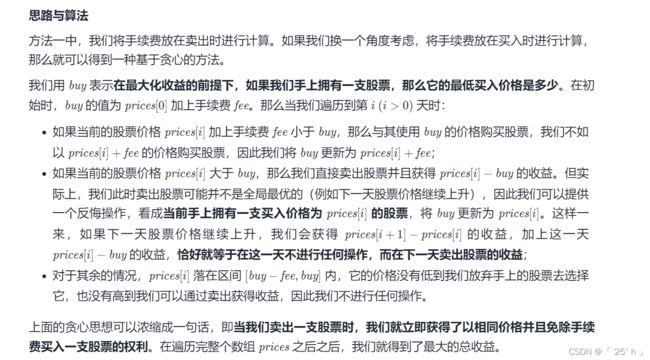
class Solution {
public int maxProfit(int[] prices, int fee) {
int profit = 0;
int buy = Integer.MAX_VALUE;
for (int i = 0; i < prices.length - 1; ++i) {
buy = Math.min(prices[i] + fee, buy);
if (prices[i + 1] > buy) {
profit += (prices[i + 1] - buy);
buy = prices[i + 1];
}
}
return profit;
}
}
leetcode406. 根据身高重建队列
、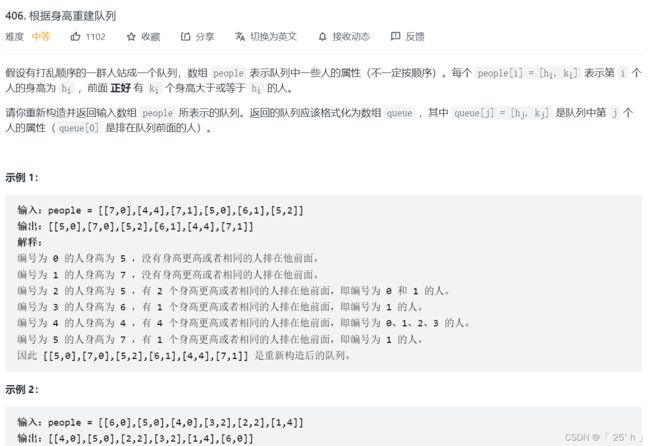
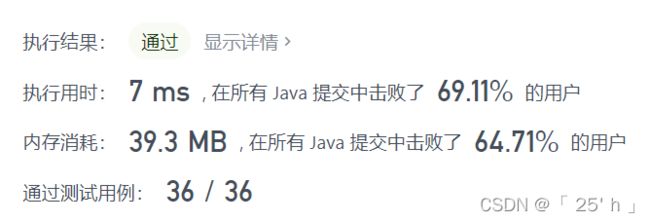
class Solution {
public int[][] reconstructQueue(int[][] people) {
int N = people.length;
Arrays.sort(people, (o1, o2) -> o1[0] == o2[0] ? o1[1] - o2[1] : o2[0] - o1[0]);
List<int[]> list = new ArrayList<>(N);
for (int[] val : people) list.add(val[1], val);
return list.toArray(new int[N][2]);
}
}
leetcode135. 分发糖果

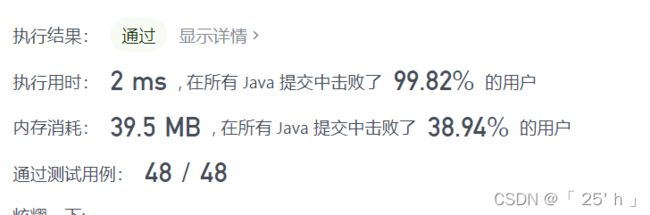
class Solution {
public int candy(int[] ratings) {
int[] candy = new int[ratings.length];
Arrays.fill(candy, 1);
for (int i = 1; i < ratings.length; i++) {
if (ratings[i] > ratings[i - 1]) {
candy[i] = candy[i - 1] + 1;
}
}
for (int i = ratings.length - 2; i >= 0; i--) {
if (ratings[i] > ratings[i + 1]) {
candy[i] = Math.max(candy[i], candy[i + 1] + 1);
}
}
int count = 0;
for (int value : candy) {
count += value;
}
return count;
}
}
leetcode55. 跳跃游戏
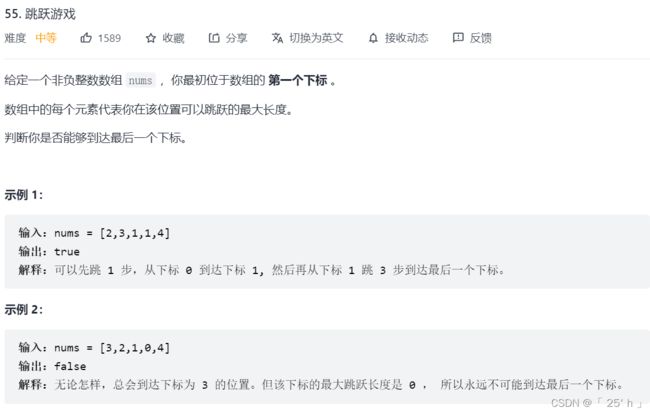
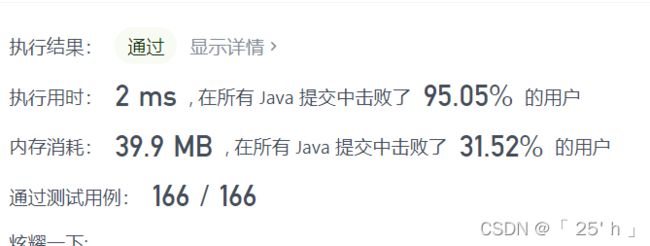
class Solution {
public boolean canJump(int[] nums) {
int pre = 0;
for (int i = 0; i < nums.length; i++) {
if (i > pre) return false;
pre = Math.max(pre, i + nums[i]);
}
return true;
}
}
leetcode45. 跳跃游戏 II
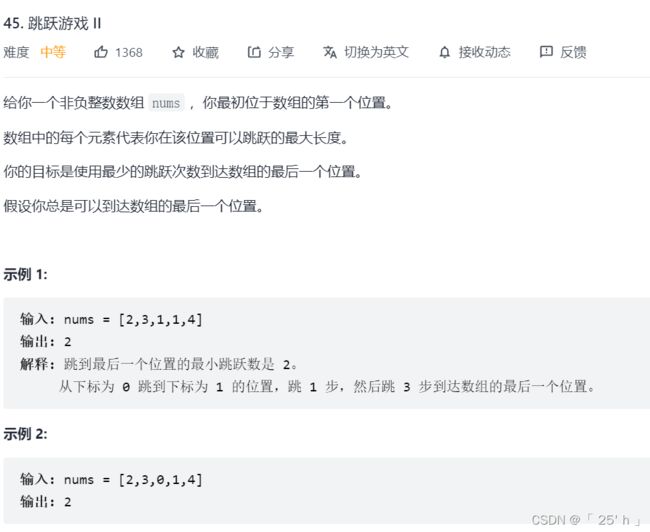
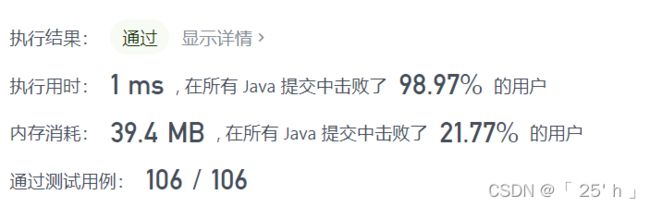
- 和加油站相似,加油站是路过加油站的油量最大值(堆),这是坐标向右伸展的最大值(可以用堆,不过我们只是需要最大值,所以没必要)
class Solution {
public int jump(int[] nums) {
if (nums.length <= 1) return 0;
int thisL = nums[0], res = 1, index = 0, maxL = thisL;
while (thisL < nums.length - 1) {
while (index <= thisL) {
maxL = Math.max(maxL, index + nums[index++]);
}
if (thisL >= maxL) return 0;
thisL = maxL;
res++;
}
return res;
}
}
leetcode435. 无重叠区间
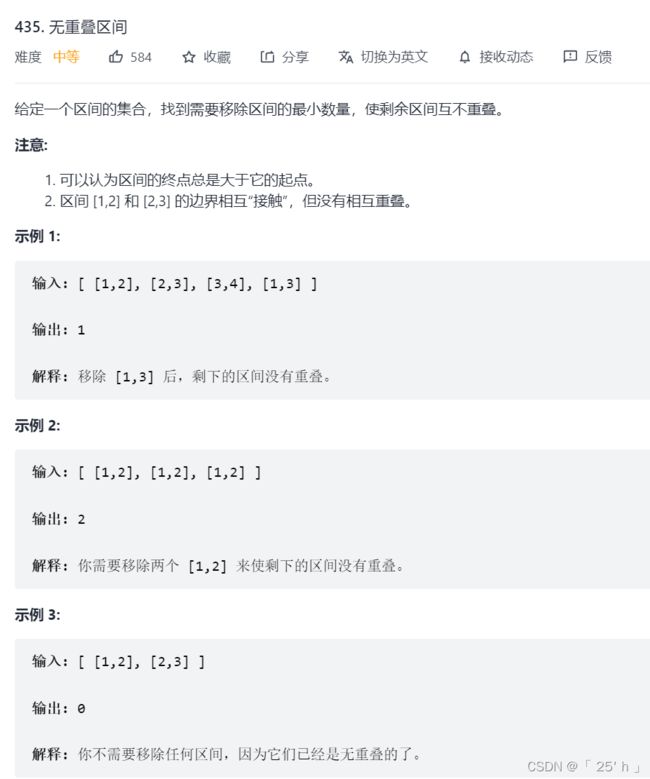

class Solution {
public int eraseOverlapIntervals(int[][] intervals) {
Arrays.sort(intervals, (o1, o2) -> o1[0] - o2[0]);
int res = 0, pre = intervals[0][1];
for (int i = 1; i < intervals.length; i++) {
if (pre > intervals[i][0]) {
pre = Math.min(pre, intervals[i][1]);
res++;
} else {
pre = intervals[i][1];
}
}
return res;
}
}
leetcode452. 用最少数量的箭引爆气球
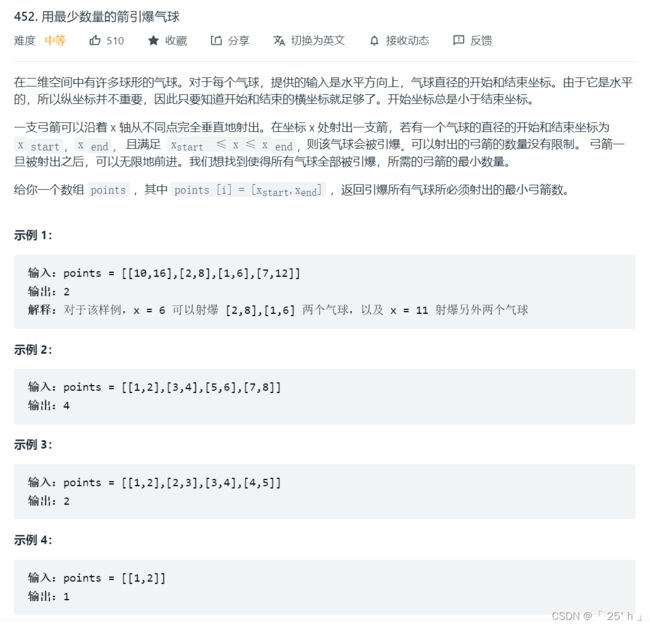
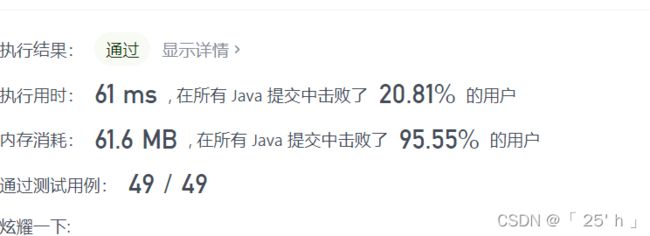
class Solution {
public int findMinArrowShots(int[][] points) {
int N = points.length;
if (N <= 1) return N;
Arrays.sort(points, Comparator.comparingInt(o -> o[0]));
int res = 1, pre = points[0][1];
for (int i = 1; i < N; i++) {
if (points[i][0] <= pre) pre = Math.min(pre, points[i][1]);
else {
res++;
pre = points[i][1];
}
}
return res;
}
}
leetcode968. 监控二叉树
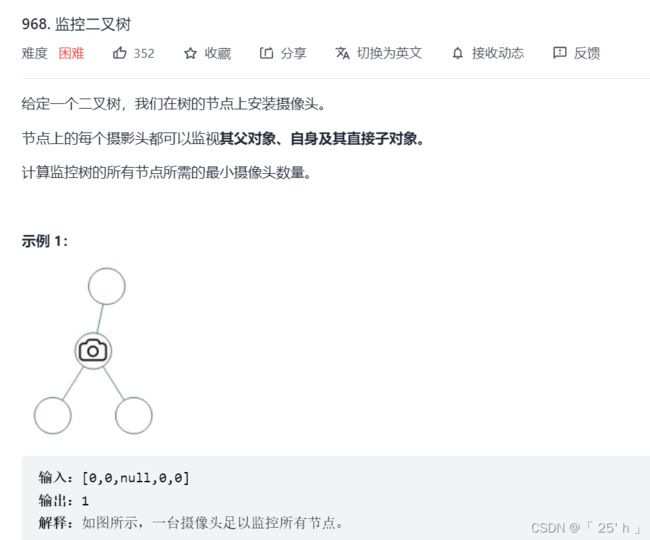
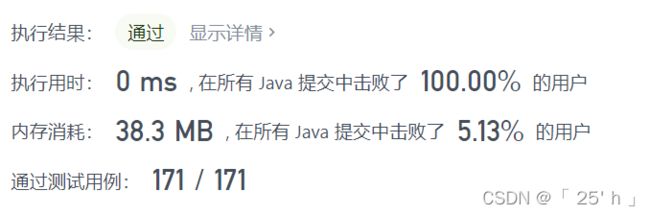
- 主要是节点状态的表示:
- 0:表示当前节点没有照亮
- 1:表示当前节点被照亮但是没有灯
- 2:当前节点有灯
class Solution {
int res = 0;
public int minCameraCover(TreeNode root) {
if (root == null) return res;
if (process(root) == 0) res++;
return res;
}
private int process(TreeNode root) {
if (root == null) return 1;
int left = process(root.left);
int right = process(root.right);
if (left == 0 || right == 0) {
res++;
return 2;
}
if (left == 1 && right == 1) {
return 0;
}
return 1;
}
}
leetcode134. 加油站
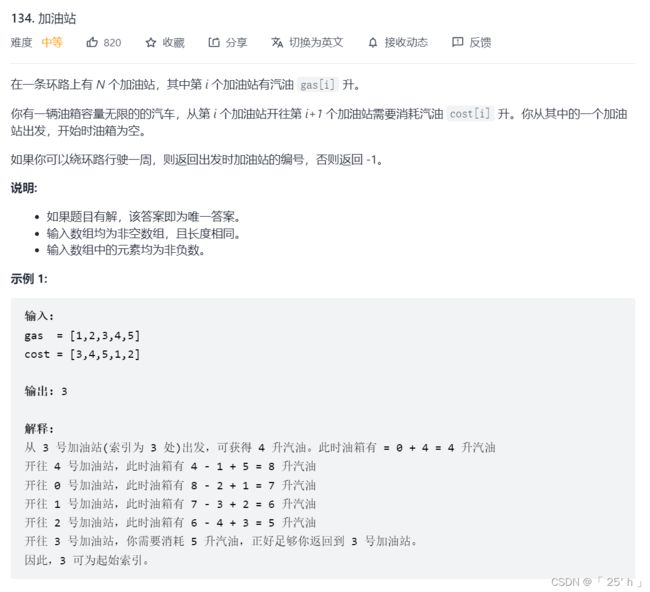
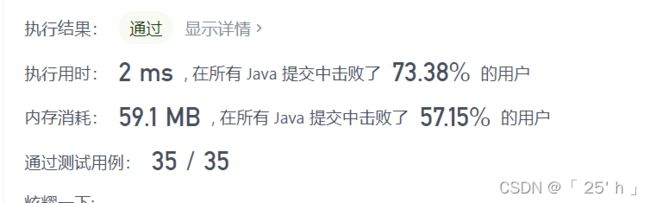
class Solution {
public int canCompleteCircuit(int[] gas, int[] cost) {
int curSum = 0;
int totalSum = 0;
int index = 0;
for (int i = 0; i < gas.length; i++) {
curSum += gas[i] - cost[i];
totalSum += gas[i] - cost[i];
if (curSum < 0) {
index = (i + 1) % gas.length;
curSum = 0;
}
}
return totalSum < 0 ? -1 : index;
}
}