Verilog数字系统设计——8位数字比较器
一、8位比较器
1.1、8位比较器概念
如果输入A[7:0]小于等于输入B[7:0]则输出1,否则输出0;
1.2、1位比较器实现
先比较最高位(第八位)
- 相等 ——> 比较第七位…(以此类推)
使用异或门(xnor)
a |
b |
out |
0 |
0 |
1 |
1 |
0 |
0 |
0 |
1 |
0 |
1 |
1 |
1 |
- 不相等 ——> 得出结果
out=~ab(一个非门,一个与门)
1.2、8位比较器实现
写出8个与门
再用一个或门连接
二、门级原语实现
2.1、方法一
Compare8_1_gate module:
module compare8_3(out,a,b);
input [7:0] a,b;
output out;
not u0(na0,a[0]);
and u1(rs0,na0,b[0]);
xnor u2(rs1,a[0],b[0]);
not u3(na1,a[1]);
and u4(rs2,na1,b[1]);
xnor u5(rs3,a[1],b[1]);
not u6(na2,a[2]);
and u7(rs4,na2,b[2]);
xnor u8(rs5,a[2],b[2]);
not u9(na3,a[3]);
and u10(rs6,na3,b[3]);
xnor u11(rs7,a[3],b[3]);
not u12(na4,a[4]);
and u13(rs8,na4,b[4]);
xnor u14(rs9,a[4],b[4]);
not u15(na5,a[5]);
and u16(rs10,na5,b[5]);
xnor u17(rs11,a[5],b[5]);
not u18(na6,a[6]);
and u19(rs12,na6,b[6]);
xnor u20(rs13,a[6],b[6]);
not u21(na7,a[7]);
and u22(rs14,na7,b[7]);
xnor u23(rs15,a[7],b[7]);
and y0(rs16,rs15,rs12);
and y1(rs17,rs15,rs13,rs10);
and y2(rs18,rs15,rs13,rs11,rs8);
and y3(rs19,rs15,rs13,rs11,rs9,rs6);
and y4(rs20,rs15,rs13,rs11,rs9,rs7,rs4);
and y5(rs21,rs15,rs13,rs11,rs9,rs7,rs5,rs2);
and y6(rs22,rs15,rs13,rs11,rs9,rs7,rs5,rs3,rs0);
and y7(rs23,rs15,rs13,rs11,rs9,rs7,rs5,rs3,rs1);
or y_out(out,rs14,rs16,rs17,rs18,rs19,rs20,rs21,rs22,rs23);
endmodule
2.2、方法二(循环生成)
module compare8_gate(out,a,b);
output wire out;
input wire [7:0] a,b;
generate
genvar j;
for(j=0;j<=7;j=j+1)
begin:loop_adder
not u0(na,a[j]);
and u1(rs,na,b[j]);
2.3、test module
module test_compare8_1_gate;
reg [7:0] a_t;
reg [7:0] b_t;
wire out_t;
compare8_gate myCompare8_1(
.out(out_t),
.a(a_t),
.b(b_t));
initial
begin
a_t=8'b0000_0000;
b_t=8'b0000_0000;
#20
a_t=8'b0010_0000;
b_t=8'b0001_0000;
2.4、仿真截图
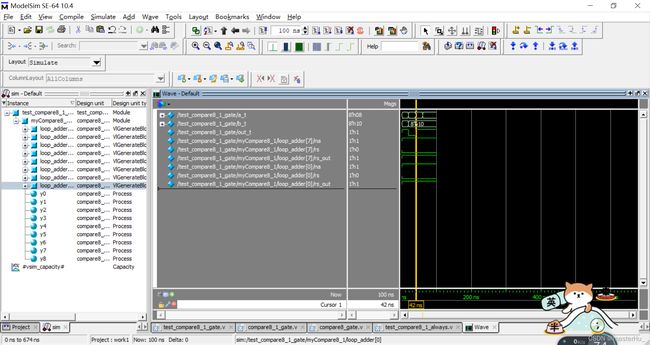
三、assign 和always 语句实现
3.1、assign module
module compare8_1_always(out,a,b);
parameter size=7;
output reg out;
input wire [size:0] a,b;
always @(a or b)
if(a<=b) out = 1;
else out = 0;
endmodule
3.2、always module
module test_compare8_1;
reg [7:0] a_t,b_t;
wire out_t;
compare8_1_always test_compare_al(.out(out_t),.a(a_t),.b(b_t));
compare8_1_assign test_compare_as(.out(out_t),.a(a_t),.b(b_t));
initial
begin
a_t = 8'b0000_0000;
b_t = 8'b0000_0000;
end
always #20 {a_t,b_t}={a_t,b_t}+8'b0000_0001;
endmodule
3.3、仿真截图
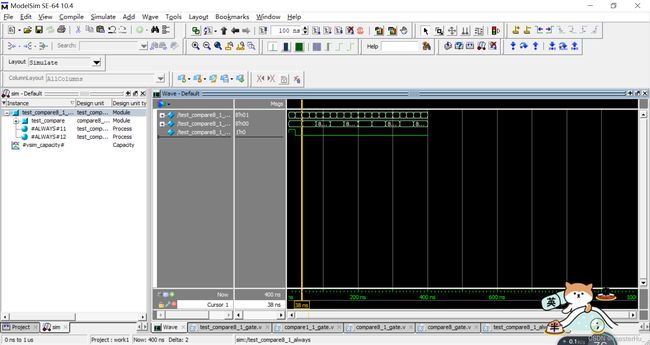