网络编程的目的:传播交流信息,数据交换
想要达到的效果:1. 如何准确得得得定位网络上的一台主机,ip+端口
2. 如何传输数据
javaweb:网页编程 B/S
网络编程:TCP/IP C/S
ip:地址:InetAddress
唯一定位一台网络上的计算机
127.0.0.1:本机地址
ip地址得到分类:
ipv4 ipv6
ipv4 127.0.0.1,四个字节组成
ipv6 128位, 8个无符号整数
2001:0bb2:aaaa:0015:0000:0000:1aaa:1312
公网(互联网) 私网(局域网)
package com.ljb.demo1;
import com.sun.jmx.snmp.InetAddressAcl;
import java.net.InetAddress;
import java.net.UnknownHostException;
//测试ip
public class TestInetAddress {
public static void main(String[] args) {
try {
//查询本地地址
InetAddress inetAddress = InetAddress.getByName("127.0.0.1");
System.out.println(inetAddress);
InetAddress inetAddress2 = InetAddress.getByName("localhost");
System.out.println(inetAddress2);
//查询网址的ip地址
InetAddress inetAddress3 = InetAddress.getByName("www.baidu.com");
System.out.println(inetAddress3);
//常用方法
System.out.println(inetAddress3.getHostAddress());
System.out.println(inetAddress3.getCanonicalHostName());//规范的名字ip
System.out.println(inetAddress3.getHostName());//
} catch (UnknownHostException e) {
e.printStackTrace();
}
}
}
端口表示计算机上的一个程序的进程
不同的进程有不同的端口
被规定0-65535
单个协议不能冲突
端口分类
公有端口 0-1023
程序注册端口
动态私有:49152-65535
netsat -ano # 查所有的端口
netsat -ano|findstr "5900" # 查指定的端口
tasklist|findstr "5900" # 查看指定端口的进程
ctrl+shift + esc
package com.ljb.demo1;
import java.net.InetSocketAddress;
public class TestInetSocketAddress {
public static void main(String[] args) {
InetSocketAddress socketAddress = new InetSocketAddress("127.0.0.1", 8080);
InetSocketAddress socket = new InetSocketAddress("localhost", 8080);
System.out.println(socketAddress);
System.out.println(socket);
System.out.println(socket.getPort());//端口
System.out.println(socket.getHostName());//地址
System.out.println(socket.getHostString());
}
}
协议:约定,就好比我们之间的谈话
网络通信协议:速率,传输码率, 代码结构,传输控制
问题:非常的复杂
大事化小:分层!
TCP/UDP:实际上是一组协议
重要:
出名的协议:
TCP/UDP 对比:
TCP:打电话
连接:稳定
三次握手,四次握手
最少需要三次,保证稳定连接
A:你愁啥
B:瞅你咋地
A:干一场
A:我要走了
B:你真的要走了码
A:我真的要走了
B:那你走吧
客户端, 服务端
传输完成,释放连接,效率低
UDP:发短信
客户端:
服务端:
package com.ljb.demo2;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.net.ServerSocket;
import java.net.Socket;
public class TestServer {
public static void main(String[] args) {
ServerSocket serverSocket = null;
Socket socket = null;
InputStream is = null;
ByteArrayOutputStream bos = null;
try {
//1.我得有一个地址
serverSocket = new ServerSocket(9696);
while (true){
//2.等待客户端连接
socket = serverSocket.accept();
//3.获取客户端的信息
is = socket.getInputStream();
//管道流
bos = new ByteArrayOutputStream();
byte[] b = new byte[1024];
int len;
while ((len=is.read(b))!=1){
bos.write(b, 0, len);
}
System.out.println(bos.toString());
}
} catch (IOException e) {
e.printStackTrace();
}finally {
//关闭资源
if (bos!=null){
try {
bos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (is!=null){
try {
is.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (socket!=null){
try {
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (serverSocket!=null){
try {
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
package com.ljb.demo2;
import java.io.IOException;
import java.io.OutputStream;
import java.net.InetAddress;
import java.net.Socket;
import java.net.UnknownHostException;
//客户端
public class TestClient {
public static void main(String[] args) {
Socket socket = null;
OutputStream os = null;
try {
//1.要知道服务器的地址
InetAddress address = InetAddress.getByName("127.0.0.1");
//2.创建一个socket连接
socket = new Socket(address,9696);
//3.发送消息
os = socket.getOutputStream();
os.write("你好, 李锦彪".getBytes());
} catch (Exception e) {
e.printStackTrace();
}finally {
if (os!=null){
try {
os.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (socket!=null){
try {
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
服务端
package com.ljb.demo3;
import java.io.*;
import java.net.ServerSocket;
import java.net.Socket;
import java.util.Scanner;
public class TcpServer {
public static void main(String[] args) throws Exception {
//1、创建服务
ServerSocket serverSocket = new ServerSocket(9696);
//2、监听客户端的连接
Socket socket = serverSocket.accept();//阻塞式
//3、获取输入流
InputStream is = socket.getInputStream();
//4、文件输出
FileOutputStream fos = new FileOutputStream("receive.png");
byte[] bytes = new byte[1024];
int len;
while ((len=is.read(bytes))!=-1){
fos.write(bytes, 0, len);
}
//通知客户端我接受完毕
OutputStream os = socket.getOutputStream();
os.write("我接受完毕,小老弟".getBytes());
// 5、关闭连接
fos.close();
is.close();
socket.close();
serverSocket.close();
}
}
客户端
package com.ljb.demo3;
import java.io.*;
import java.net.InetAddress;
import java.net.Socket;
public class TcpClient {
public static void main(String[] args) throws Exception {
//1、创建一个socket连接
Socket socket = new Socket(InetAddress.getByName("127.0.0.1"), 9696);
//2、创建一个输出流
OutputStream os = socket.getOutputStream();
//3、获取文件
FileInputStream fis = new FileInputStream(new File("D:\\IDEA Store\\网络编程学习\\src\\A-女白.png"));
//4、写出文件
byte[] bytes = new byte[1024];
int len;
while ((len=fis.read(bytes))!=-1){
os.write(bytes, 0, len);
}
//通知服务器已经接收完了
socket.shutdownOutput();//我已经传输完了
//确定服务端接收完毕、
InputStream is = socket.getInputStream();
//String byte[]
ByteArrayOutputStream baos = new ByteArrayOutputStream();
byte[] bytes1 = new byte[1024];
int len2;
while ((len2=is.read(bytes1))!=-1){
baos.write(bytes1, 0, len2);
}
System.out.println(baos.toString());
//5、关闭连接
baos.close();
fis.close();
os.close();
socket.close();
}
}
发短信,导弹
服务端
package com.ljb.demo4;
import java.net.DatagramPacket;
import java.net.DatagramSocket;
//还是要等待客户端的连接
public class Udpserver {
public static void main(String[] args) throws Exception {
//开放端口
DatagramSocket socket = new DatagramSocket(9696);
//接收数据包
byte[] bytes = new byte[1024];
DatagramPacket dp = new DatagramPacket(bytes, 0, bytes.length);
socket.receive(dp);
System.out.println(new String(dp.getData()));
}
}
客户端
package com.ljb.demo4;
import java.net.DatagramPacket;
import java.net.DatagramSocket;
import java.net.InetAddress;
//不需要连接服务器
public class Udpclient {
public static void main(String[] args) throws Exception {
//1、建立一个socket
DatagramSocket socket = new DatagramSocket();
//2、建个包
String msg = "你好啊, 李锦彪";
//发送给谁
InetAddress inetAddress = InetAddress.getByName("127.0.0.1");
int port = 9696;
//数据,数据的长度起始, 要发送给谁
DatagramPacket dp = new DatagramPacket(msg.getBytes(), 0, msg.getBytes().length, inetAddress, port);
//发送包
socket.send(dp);
//关闭连接
socket.close();
}
}
receive
package com.ljb.demo5;
import java.net.DatagramPacket;
import java.net.DatagramSocket;
public class UdpReceive {
public static void main(String[] args) throws Exception {
DatagramSocket socket = new DatagramSocket(6666);
while (true){
//准备接收
byte[] bytes = new byte[1024];
DatagramPacket dp = new DatagramPacket(bytes, 0, bytes.length);
socket.receive(dp);//阻塞式接收包裹
//断开连接
byte[] data = dp.getData();
String receiveData = new String(data, 0, data.length).trim();//trim()去掉多余的字节
System.out.println(receiveData);
if (receiveData.equals("0")){
break;
}
}
socket.close();
}
}
sender
package com.ljb.demo5;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.DatagramPacket;
import java.net.DatagramSocket;
import java.net.InetSocketAddress;
public class UdpSender {
public static void main(String[] args) throws Exception {
DatagramSocket socket = new DatagramSocket(9696);
//准备数据,控制台读取
System.out.println("请输入:");
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
while (true){
String data = br.readLine();
byte[] bytes = data.getBytes();
DatagramPacket dp = new DatagramPacket(bytes, 0, bytes.length, new InetSocketAddress("localhost", 6666));
socket.send(dp);
if (data.equals("0")){
break;
}
}
socket.close();
}
}
对话线程
package com.ljb.demo5;
public class TalkStudent {
public static void main(String[] args) {
//开启两个线程
new Thread(new TalkSend(7777, "localhost", 9999)).start();
new Thread(new TalkReceive(8888, "老师")).start();
}
}
----------------------------------------------------------
package com.ljb.demo5;
public class TalkTeacher {
public static void main(String[] args) {
new Thread(new TalkSend(5555, "localhost", 8888)).start();
new Thread(new TalkReceive(9999, "学生")).start();
}
}
receive
package com.ljb.demo5;
import java.net.DatagramPacket;
import java.net.DatagramSocket;
import java.net.SocketException;
public class TalkReceive implements Runnable{
DatagramSocket socket = null;
private int port;
private String msgName;
public TalkReceive(int port, String msgName) {
this.port = port;
this.msgName = msgName;
try {
socket = new DatagramSocket(port);
} catch (SocketException e) {
e.printStackTrace();
}
}
@Override
public void run() {
while (true){
try{
//准备接收
byte[] bytes = new byte[1024];
DatagramPacket dp = new DatagramPacket(bytes, 0, bytes.length);
socket.receive(dp);//阻塞式接收包裹
//断开连接
byte[] data = dp.getData();
String receiveData = new String(data, 0, data.length).trim();//trim()去掉多余的字节
System.out.println(msgName+":"+receiveData);
if (receiveData.equals("0")){
break;
}
}catch (Exception e){
e.printStackTrace();
}
}
socket.close();
}
}
sender
package com.ljb.demo5;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.DatagramPacket;
import java.net.DatagramSocket;
import java.net.InetSocketAddress;
import java.net.SocketException;
public class TalkSend implements Runnable{
DatagramSocket socket = null;
BufferedReader br = null;
private int fromport;
private String toip;
private int toport;
public TalkSend(int fromport, String toip, int toport) {
this.fromport = fromport;
this.toip = toip;
this.toport = toport;
try {
socket = new DatagramSocket(this.fromport);
br = new BufferedReader(new InputStreamReader(System.in));
} catch (SocketException e) {
e.printStackTrace();
}
}
@Override
public void run() {
//准备数据,控制台读取
while (true){
try {
String data = br.readLine();
byte[] bytes = data.getBytes();
DatagramPacket dp = new DatagramPacket(bytes, 0, bytes.length, new InetSocketAddress(this.toip, this.toport));
socket.send(dp);
if (data.equals("0")){
break;
}
}catch (Exception e){
e.printStackTrace();
}
}
socket.close();
}
}
https://www.baidu.com/
协议://ip地址:端口/项目名/资源
}
@Override
public void run() {
//准备数据,控制台读取
while (true){
try {
String data = br.readLine();
byte[] bytes = data.getBytes();
DatagramPacket dp = new DatagramPacket(bytes, 0, bytes.length, new InetSocketAddress(this.toip, this.toport));
socket.send(dp);
if (data.equals("0")){
break;
}
}catch (Exception e){
e.printStackTrace();
}
}
socket.close();
}
}
### 1.7 URL
https://www.baidu.com/
协议://ip地址:端口/项目名/资源
#### 1.7.1下载
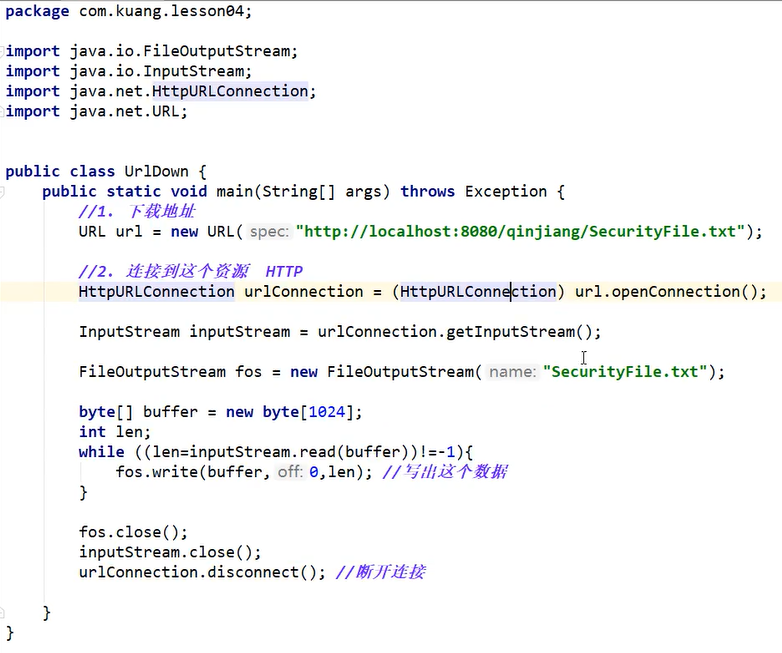