这个是当时我做的java课设
import javax.imageio.ImageIO;
import java.awt.*;
import java.awt.event.MouseAdapter;
import java.awt.event.MouseEvent;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
public class five_in_a_rowGame {
Frame frame = new Frame("五子棋游戏");
BufferedImage write;
BufferedImage black;
BufferedImage table;
final int TABLE_WIDTH =660;
final int TABLE_HEIGHT =692;
final int BORDER_SIZE =22;
final int rate = TABLE_WIDTH/BORDER_SIZE;
int x_offset=30;
int y_offset=30;
int [][] board = new int [BORDER_SIZE+8][BORDER_SIZE+8];//0为没有棋子,1为白棋,2为黑棋
private class MyCanvas extends Canvas{
public void paint(Graphics g){
g.drawImage(table,0,0,null);
for(int i=4;i<=BORDER_SIZE;i++){
for(int j=4;j<=BORDER_SIZE;j++){
if(board[i][j] == 2){
g.drawImage(black,rate*(i-4)+x_offset,rate*(j-4)+y_offset,null);
}
if(board[i][j] == 1){
g.drawImage(write,rate*(i-4)+x_offset,rate*(j-4)+y_offset,null);
}
}
}
if(judgeByStraightLine()){
g.setFont(new Font("Courier",Font.BOLD,40));
g.drawString("游戏结束",300,345);
}
}
}
MyCanvas myCanvas =new MyCanvas();
Panel panel = new Panel();
int board_type =1;
Button writebutton = new Button("白棋");
Button blackbutton = new Button("黑棋");
Button backbutton = new Button("删除");
public void refreshColor(Color WriteButColor,Color BlackButColor,Color BcakButColor){
writebutton.setBackground(WriteButColor);
blackbutton.setBackground(BlackButColor);
backbutton.setBackground(BcakButColor);
}
public void init() throws Exception{
writebutton.addActionListener(e -> {
board_type =1;
refreshColor(Color.GREEN,Color.GRAY,Color.GRAY);
});
blackbutton.addActionListener(e -> {
board_type=2;
refreshColor(Color.GRAY,Color.GREEN,Color.GRAY);
});
backbutton.addActionListener(e->{
board_type=0;
refreshColor(Color.GRAY,Color.GRAY,Color.GREEN);
});
panel.add(writebutton);
panel.add(blackbutton);
panel.add(backbutton);
frame.add(panel,BorderLayout.SOUTH);
write = ImageIO.read(new File("E:\\awt\\images\\white.jpg"));
black = ImageIO.read(new File("E:\\awt\\images\\black.jpg"));
table = ImageIO.read(new File("E:\\awt\\images\\table.jpg"));
myCanvas.repaint();
myCanvas.addMouseListener(new MouseAdapter() {
@Override
public void mouseClicked(MouseEvent e) {
int pox =(e.getX()-x_offset)/rate;
int poy =(e.getY()-y_offset)/rate;
board[pox+4][poy+4] =board_type;
myCanvas.repaint();
if(board_type==1){
board_type=2;
}else {
board_type=1;
}
}
});
myCanvas.setPreferredSize(new Dimension(TABLE_WIDTH,TABLE_HEIGHT));
frame.add(myCanvas);
frame.pack();
frame.setVisible(true);
frame.addWindowListener(new WindowAdapter() {
@Override
public void windowClosing(WindowEvent e) {
System.exit(0);
}
});
}
public boolean judgeByStraightLine(){
for(int i=4;i<=BORDER_SIZE;i++){
for(int j=4;j<=BORDER_SIZE;j++) {
if (judgepart(i,j))
return true;
}
}
return false;
}
public boolean judgepart(int r,int c){
if(judgeSLRpart(r,c)||judgeSLCpart(r,c)||judgeTLPpart(r,c)||judgeTLNpart(r,c)){
return true;
}else {
return false;
}
}
public boolean judgeSLRpart(int r,int c){
if(judgeSLRpartW(r,c)||judgeSLRpartB(r,c)){
return true;
}else {
return false;
}
}
public boolean judgeSLRpartW(int r,int c) {
for (int i = r; i <= r + 4; i++) {
if (board[i][c] != 1)
return false;
}
return true;
}
public boolean judgeSLRpartB(int r,int c){
for(int i=r;i<=r+4;i++){
if(board[i][c]!=2)
return false;
}
return true;
}
public boolean judgeSLCpart(int r,int c){
if(judgeSLCpartW(c,r)||judgeSLCpartB(c,r)){
return true;
}else {
return false;
}
}
public boolean judgeSLCpartW(int c,int r){
for(int i=c;i<=c+4;i++){
if(board[r][i]!=1)
return false;
}
return true;
}
public boolean judgeSLCpartB(int c,int r){
for(int i =c;i<=c+4;i++){
if(board[r][i]!=2)
return false;
}
return true;
}
public boolean judgeTLPpart(int c,int r){
if(judgeTLPpartW(c,r)||judgeTLPpartB(c,r)){
return true;
}else {
return false;
}
}
public boolean judgeTLPpartW(int c,int r){
for(int i =c,j=r;i<=c+4;i++,j++){
if(board[i][j]!=1){
return false;
}
}
return true;
}
public boolean judgeTLPpartB(int c,int r){
for(int i =c,j=r;i<=c+4;i++,j++){
if(board[i][j]!=2){
return false;
}
}
return true;
}
public boolean judgeTLNpart(int r,int c){
if(judgeTLNpartB(r,c)||judgeTLNpartW(r,c)){
return true;
}else {
return false;
}
}
public boolean judgeTLNpartW(int r,int c){
for(int i=r,j=c;j>=c-4;i++,j--){
if(board[i][j]!=1){
return false;
}
}
return true;
}
public boolean judgeTLNpartB(int r,int c){
for(int i=r,j=c;j>=c-4;i++,j--){
if(board[i][j]!=2){
return false;
}
}
return true;
}
}
class main{
public static void main(String[] args) {
try {
new five_in_a_rowGame().init();
} catch (Exception e) {
e.printStackTrace();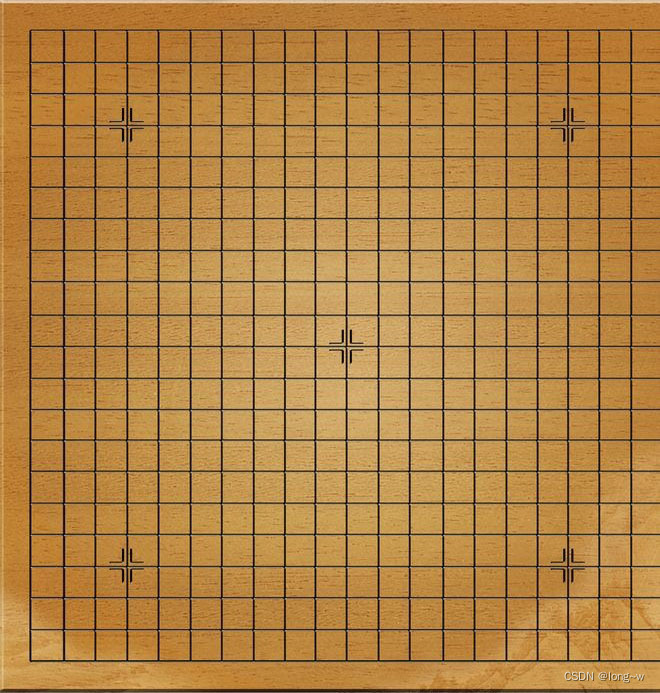
}
}
}