一、字典中添加和修改数据
my_dict = {'name': 're'}
print(my_dict)
my_dict['age'] = 20
print(my_dict)
my_dict['age'] = 25
print(my_dict)
my_dict[1] = 'int'
print(my_dict)
my_dict[1.0] = 'float'
print(my_dict)
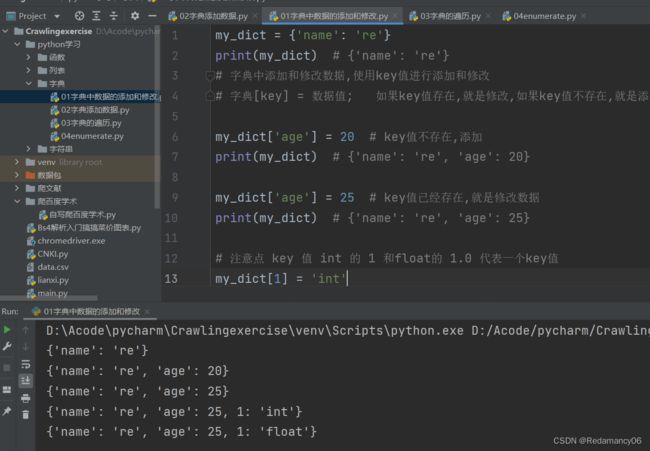
二、字典中删除数据
my_dict = {'name': 're', 'age': 20, 1: 'float', 2: 'bb'}
del my_dict[1]
print(my_dict)
result = my_dict.pop('age')
print(my_dict)
print(result)
my_dict.clear()
print(my_dict)
del my_dict
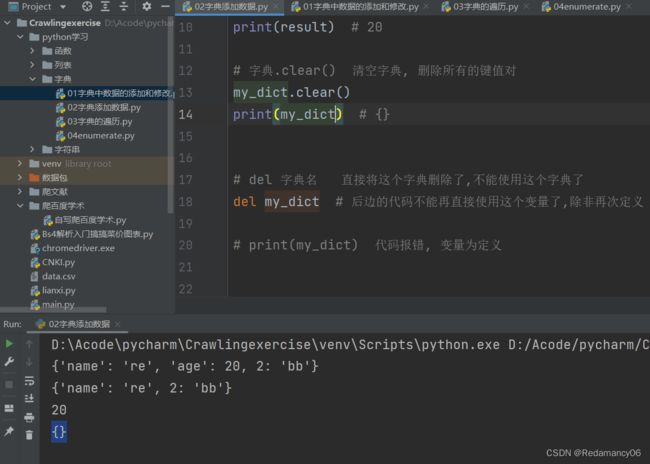
三、字典中遍历数据
1. for 循环直接遍历字典, 遍历的是字典的 key 值
my_dict = {'name': 're', 'age': 20, 'gender': '男'}
for key in my_dict:
print(key, my_dict[key])
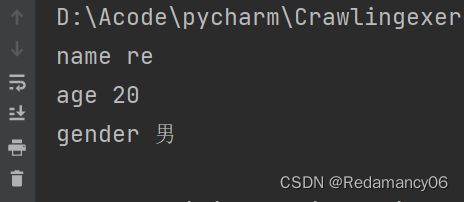
2. 字典.keys()
my_dict = {'name': 're', 'age': 20, 'gender': '男'}
result = my_dict.keys()
print(result, type(result))
for key in result:
print(key)
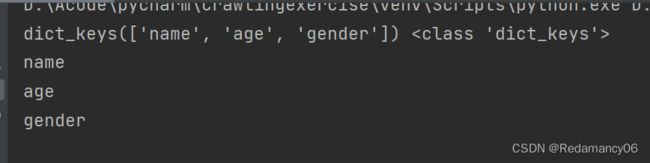
3. 字典.values()
my_dict = {'name': 're', 'age': 20, 'gender': '男'}
result = my_dict.values()
print(result, type(result))
for value in my_dict.values():
print(value)
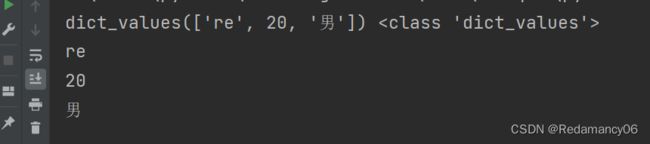
4. 字典.items()
my_dict = {'name': 're', 'age': 20, 'gender': '男'}
result = my_dict.items()
print(result, type(result))
for item in my_dict.items():
print(item[0], item[1])
print('=' * 30)
for k, v in my_dict.items():
print(k, v)
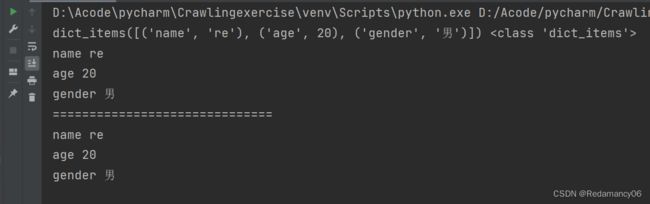
四、enumerate 函数
my_list = ['a', 'b', 'c', 'd', 'e']
for i in my_list:
print(i)
for i in my_list:
print(my_list.index(i), i)
for j in enumerate(my_list):
print(j)
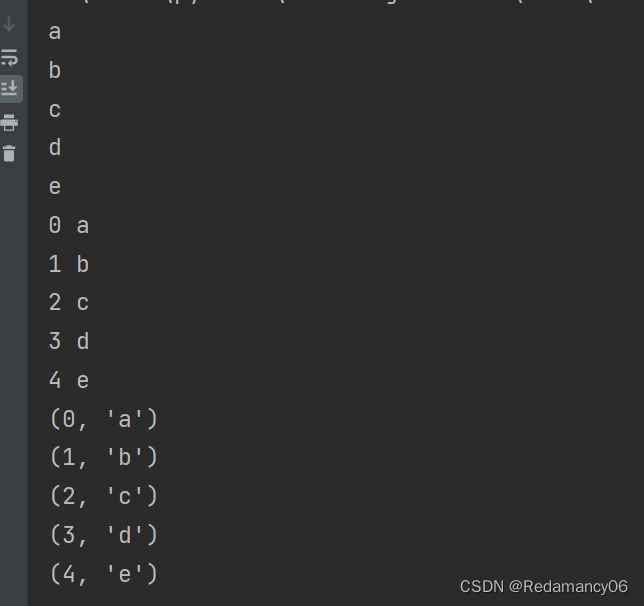
五、公共方法
运算符 |
Python 表达式 |
结果 |
描述 |
支持的数据类型 |
+ |
[1, 2] + [3, 4] |
[1, 2, 3, 4] |
合并 |
字符串、列表、元组 |
* |
[‘Hi!’] * 4 |
[‘Hi!’, ‘Hi!’, ‘Hi!’, ‘Hi!’] |
复制 |
字符串、列表、元组 |
in |
3 in (1, 2, 3) |
true |
元素是否存在 |
字符串、列表、元组、字典 |
not in |
4 not in (1, 2, 3) |
true |
元素是否不存在 |
字符串、列表、元组、字典 |
注意: 如果是字典的话,判断的是 key 值是否存在或不存在 |
|
|
|
|
max/min 对于字典来说,比较的字典的 key值的大小 |
|
|
|
|