文章目录
- 数据列表 - Collection
- 代码实现 - Code
-
- 代码
- 成员
- 函数
-
- 内置函数
-
- __init__(self, model=None, datas=None)函数
- __len__(self)函数
- __and__(self, other)函数
- __or__(self, other)函数
- __contains__(self, item)函数
- __delitem__(self, key)函数
- __isub__(self, other)函数
- __iadd__(self, other)函数
- __getitem__(self, item)函数
- __setitem__(self, key, value)函数
- __bool__(self)函数
- __copy__(self)函数
- 成员函数
-
- isnumber(self)函数
- count(self)函数
- add(self, item)函数
- insert(self, index, item)函数
- addrange(self, items)函数
- remove(self, item)函数
- removeat(self, index)函数
- copy(self)函数
- indexof(self, item)函数
- reverse(self)函数
- sort(self, action=None)函数
- random(self, count, dicts=None)函数
- single(self, item)函数
- counter(self, item)函数
- max(self, action=None)函数
- min(self, action=None)函数
- `where(self, expression)`函数
- delete(self, expression)函数
- all(self, expression)函数
- firstordefault(self, default=None)函数
- first(self)函数
- foreach(self, action)函数
- Collection存在的意义
数据列表 - Collection
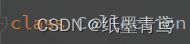
Collection 层次结构 中的根接口。Collection 表示一组对象,这些对象也称为 collection 的元素。一些 collection 允许有重复的元素,而另一些则不允许。一些 collection 是有序的,而另一些则是无序的。
而本框架下的Collection是带有Type标签的,即指定数据类型,在非相同类型插入的情况下会出现插入错误
代码实现 - Code
代码
class Collection:
def __init__(self, model=None, datas=None):
self._model = model
self._type = type(model)
self._datas = []
if datas is not None:
self.addrange(datas)
def __len__(self):
return len(self._datas)
def __and__(self, other):
return self.union(other)
def __or__(self, other):
return self.intersect(other)
def __contains__(self, item):
return item in self._datas
def __iter__(self):
self._index = 0
return self
def __del__(self):
self.clear()
def __delitem__(self, key):
self.removeat(key)
def __next__(self):
if self._index < len(self._datas):
result = self._datas[self._index]
self._index += 1
return result
else:
raise StopIteration
def __str__(self):
return str(self._datas)
def __isub__(self, other):
if other is Collection:
for i in other:
if i in self:
self.remove(i)
return self
self.remove(other)
return self
def __iadd__(self, other):
if other is Collection:
self.addrange(other)
return self
self.add(other)
return self
def __getitem__(self, item):
if type(item) == int:
return self._datas[item]
else:
raise Exception("Index Must Be Number")
def __setitem__(self, key, value):
if type(key) == int and key < len(self._datas) - 1 and type(value) == self._type:
self._datas[key] = value
def __bool__(self):
return len(self._datas) != 0
def __copy__(self):
return self.copy()
def isnumber(self):
return self._type == int or self._type == float
def count(self):
return len(self._datas)
def add(self, item):
if self._model is None:
self._model = item
self._type = type(item)
self._datas.append(item)
return
if self._type != type(item):
raise Exception("Type Error")
else:
self._datas.append(item)
def insert(self, index, item):
if type(index) != int:
raise Exception("Index must be number")
if self._type != type(item):
raise Exception("Type Error")
self._datas.insert(index, item)
def addrange(self, items):
try:
for i in items:
if self._type != type(i) and self._model is not None:
raise Exception("Type Error")
else:
self.add(i)
except:
raise Exception("Could Not Add Items")
def remove(self, item):
if item in self._datas:
self._datas.remove(item)
else:
raise Exception("Could Not Find Object")
def removeat(self, index):
if len(self._datas) - 1 < index:
raise Exception("Over float")
else:
del self._datas[index]
def copy(self):
result = Collection(self._model, self._datas)
return result
def clear(self):
self._datas.clear()
def indexof(self, item):
for index, data in enumerate(self._datas):
if item == data:
return index
else:
continue
def type(self):
return self._type
def model(self):
return self._model
def reverse(self):
self._datas.reverse()
def sort(self, action=None):
self._datas.sort(key=action)
def random(self, count, dicts=None):
if self._type == int or self._type == float or self._model is None:
if dicts is None:
for i in range(count):
self.add(random.randint(-65535, 65535))
else:
for i in range(count):
self.add(dicts[random.randint(0, len(dicts))])
else:
raise Exception("Type Error")
def single(self, item):
if item not in self._datas:
raise Exception('Data is not Exist')
count = 0
for i in self._datas:
if i == item:
count += 1
return count == 1
def counter(self, item):
if item not in self._datas:
raise Exception('Data is not Exist')
count = 0
for i in self._datas:
if i == item:
count += 1
return count
def max(self, action=None):
if self._type == int or self._type == float:
if action is not None:
result = 0
for i in self._datas:
result = i if action(i) > action(result) else result
return result
result = 0
for i in self._datas:
result = i if i > result else result
return result
else:
if action is not None:
raise Exception('Type Error')
else:
result = 0
for i in self._datas:
result = i if action(i) > action(result) else result
return result
def min(self, action=None):
if self._type == int or self._type == float:
if action is not Nohne:
result = 0
for i in self._datas:
result = i if action(i) < action(result) else result
return result
result = 0
for i in self._datas:
result = i if i < result else result
return result
else:
if action is not None:
raise Exception('Type Error')
else:
result = 0
for i in self._datas:
result = i if action(i) < action(result) else result
return result
def where(self, expression):
datas = []
for i in self._datas:
if expression(i):
datas.append(i)
else:
continue
result = Collection(self._model, datas)
return result
def delete(self, expression):
data = self.copy()
for i in data:
if expression(i):
self.remove(i)
def union(self, collection):
if self._type != collection.type():
raise Exception('Type Error')
else:
result = Collection()
cp_self = self.copy()
cp_col = collection.copy()
for i in range(len(cp_self)):
if cp_self[i] in cp_col:
result.add(cp_self[i])
cp_col.remove(cp_self[i])
return result
def intersect(self, collection):
if self._type != collection.type():
raise Exception('Type Error')
else:
result = Collection()
for i in self:
result.add(i)
for i in collection:
result.add(i)
return result
def all(self, expression):
for i in self._datas:
if not expression(i):
return False
else:
continue
return True
def firstordefault(self, default=None):
if len(self._datas) == 0:
return default
else:
return self._datas[0]
def first(self):
if len(self._datas) == 0:
raise Exception("No Data In Collection")
else:
return self._datas[0]
def avg(self, action=None):
if action is None:
sums = 0
for i in self._datas:
sums += i
return sums / len(self._datas)
else:
sums = 0
for i in self._datas:
sums += action(i)
return sums / len(self._datas)
def sum(self, action=None):
if action is None:
sums = 0
for i in self._datas:
sums += i
return sums
else:
sums = 0
for i in self._datas:
sums += action(i)
return sums
def foreach(self, action):
result = Collection()
for i in range(len(self._datas)):
result.add(action(self._datas[i]))
return result
def tolist(self, function=None):
if function is None:
return self._datas
else:
result = []
for i in self._datas:
result.append(function(i))
return result
def totuple(self, function=None):
if function is None:
return tuple(self._datas)
else:
result = []
for i in self._datas:
result.append(function(i))
return tuple(result)
def tostring(self):
return type(self._model)
def todictionary(self, function):
data = {}
for i in self._datas:
keyvalue = function(i)
data[keyvalue[0]] = keyvalue[1]
return data
成员
字段名称 |
类型 |
作用 |
_model |
object |
储存原始数据 |
_type |
type |
储存原始类型 |
_datas |
list |
数据集合 |
函数
内置函数
init(self, model=None, datas=None)函数
参数名 |
类型 |
介绍 |
默认值 |
model |
object |
原始数据 |
None |
datas |
__iter__ |
初始化赋值数据 |
None |
source = [1, 2, 3, 4, 5]
collection = Collection(0, source)
print(collection)
>>>[1, 2, 3, 4, 5]
len(self)函数
类型 |
介绍 |
int |
Collection的元素数量 |
source = [1, 2, 3, 4, 5]
collection = Collection(0, source)
print(len(collection))
>>>>5
and(self, other)函数
参数名 |
类型 |
介绍 |
默认值 |
other |
Collection |
取并集的Collection |
|
类型 |
介绍 |
Collection |
Collection与另一个Collection取并集的结果 |
source = [1, 2, 3, 4, 5]
other = [6, 7, 8, 9, 10]
collection1 = Collection(0, source)
collection2 = Collection(0, other)
result = collection1 | collection2
print(result)
>>>>[1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
or(self, other)函数
参数名 |
类型 |
介绍 |
默认值 |
other |
Collection |
取交集的Collection |
|
类型 |
介绍 |
Collection |
Collection与另一个Collection取并集的结果 |
source = [1, 2, 3, 4, 5]
other = [6, 7, 8, 9, 10]
collection1 = Collection(0, source)
collection2 = Collection(0, other)
result = collection1 & collection2
print(result)
>>>>[]
contains(self, item)函数
参数名 |
类型 |
介绍 |
默认值 |
item |
object |
item元素是否在当前Collection中 |
|
类型 |
介绍 |
bool |
item是否在当前Collection中 |
source = [1, 2, 3, 4, 5]
collection = Collection(0, source)
print(3 in collection)
>>>>True
delitem(self, key)函数
参数名 |
类型 |
介绍 |
默认值 |
key |
int |
删除Collection的index位置的元素 |
|
source = [1, 2, 3, 4, 5]
collection = Collection(0, source)
del collection[0]
print(collection)
>>>>[2, 3, 4, 5]
isub(self, other)函数
参数名 |
类型 |
介绍 |
默认值 |
other |
object |
Collection中需要删除的对象 |
|
source = [1, 2, 3, 4, 5]
collection = Collection(0, source)
collection -= 5
print(collection)
>>>>[1, 2, 3, 4]
iadd(self, other)函数
参数名 |
类型 |
介绍 |
默认值 |
other |
object |
Collection中需要增加的对象 |
|
source = [1, 2, 3, 4, 5]
collection = Collection(0, source)
collection += 20
print(collection)
>>>>[1, 2, 3, 4, 5, 20]
getitem(self, item)函数
参数名 |
类型 |
介绍 |
默认值 |
item |
int |
获取需要在Collection中item的位置 |
|
source = [1, 2, 3, 4, 5]
collection = Collection(0, source)
print(collection[0])
>>>>1
setitem(self, key, value)函数
参数名 |
类型 |
介绍 |
默认值 |
key |
int |
获取需要在Collection中item的位置 |
|
value |
object |
修改的值 |
|
source = [1, 2, 3, 4, 5]
collection = Collection(0, source)
collection[0] = 20
print(collection)
>>>>[20, 2, 3, 4, 5]
bool(self)函数
类型 |
介绍 |
bool |
Collection的元素数量是否为0 |
source = [1, 2, 3, 4, 5]
collection = Collection(0, source)
print(collection == True)
>>>>False
copy(self)函数
类型 |
介绍 |
Collection |
返回这个元素的复制 |
source = [1, 2, 3, 4, 5]
collection = Collection(0, source)
result = copy.copy(collection)
print(result)
>>>>[1, 2, 3, 4, 5]
成员函数
isnumber(self)函数
source = [1, 2, 3, 4, 5]
collection = Collection(0, source)
print(collection.isnumber())
>>>>True
count(self)函数
类型 |
介绍 |
int |
Collection中的元素的数量 |
source = [1, 2, 3, 4, 5]
collection = Collection(0, source)
print(collection.count())
>>>>5
add(self, item)函数
参数名 |
类型 |
介绍 |
默认值 |
item |
object |
待添加进Collection的元素 |
|
source = [1, 2, 3, 4, 5]
collection = Collection(0, source)
collection.add(5)
print(collection)
>>>>[1, 2, 3, 4, 5, 5]
source = [1, 2, 3, 4, 5]
collection = Collection(0, source)
collection.add('A')
print(collection)
>>>>Traceback (most recent call last):
File "DBCollection.py", line 345, in <module>
collection.add('A')
File "DBCollection.py", line 94, in add
raise Exception("Type Error")
Exception: Type Error
insert(self, index, item)函数
参数名 |
类型 |
介绍 |
默认值 |
index |
int |
待插入元素位置 |
|
item |
object |
待插入元素 |
|
source = [1, 2, 3, 4, 5]
collection = Collection(0, source)
collection.insert(0, 30)
print(collection)
>>>>[30, 1, 2, 3, 4, 5]
addrange(self, items)函数
参数名 |
类型 |
介绍 |
默认值 |
items |
__iter__ |
待插入的元素集合 |
|
source = [1, 2, 3, 4, 5]
collection = Collection(0, source)
collection.addrange([10, 20, 30, 40])
print(collection)
>>>>[1, 2, 3, 4, 5, 10, 20, 30, 40]
remove(self, item)函数
参数名 |
类型 |
介绍 |
默认值 |
item |
object |
待移除的对象 |
|
source = [1, 2, 3, 4, 5]
collection = Collection(0, source)
collection.remove(5)
print(collection)
>>>>[1, 2, 3, 4]
removeat(self, index)函数
参数名 |
类型 |
介绍 |
默认值 |
item |
object |
待移除的对象 |
|
source = [1, 2, 3, 4, 5]
collection = Collection(0, source)
collection.removeat(0)
print(collection)
>>>>[2, 3, 4, 5]
copy(self)函数
类型 |
介绍 |
Collection |
返回这个元素的复制 |
source = [1, 2, 3, 4, 5]
collection = Collection(0, source)
result = collection.copy()
print(result)
>>>>[1, 2, 3, 4, 5]
indexof(self, item)函数
参数名 |
类型 |
介绍 |
默认值 |
item |
object |
查询位置的对象 |
|
source = [1, 2, 3, 4, 5]
collection = Collection(0, source)
print(collection.indexof(3))
>>>>2
reverse(self)函数
将Collection中的元素反序
source = [1, 2, 3, 4, 5]
collection = Collection(0, source)
collection.reverse()
print(collection)
>>>>[5, 4, 3, 2, 1]
sort(self, action=None)函数
参数名 |
类型 |
介绍 |
默认值 |
action |
function |
对元素变换的函数 |
None |
source = [
['Alice', 15],
['Mace', 17],
['Alade', 15],
['Pak', 27],
['Vu', 10]
]
collection = Collection([], source)
collection.sort(lambda x: x[1])
print(collection)
>>>>[['Vu', 10], ['Alice', 15], ['Alade', 15], ['Mace', 17], ['Pak', 27]]
random(self, count, dicts=None)函数
参数名 |
类型 |
介绍 |
默认值 |
count |
int |
填充Collection的长度 |
|
dicts |
__getitem__ |
填充Collection所用字典 |
None |
collection = Collection()
collection.random(12)
collection.sort()
print(collection)
>>>>[-39526, -23267, -14011, -9193, 21789, 28951, 32123, 34779, 41263, 44166, 45688, 54137]
single(self, item)函数
参数名 |
类型 |
介绍 |
默认值 |
item |
object |
需要检查的对象 |
|
类型 |
介绍 |
bool |
是否是该Collection中唯一存在 |
datas = [1, 2, 3, 4, 5]
collection = Collection(0,datas)
print(collection.single(5))
>>>>True
counter(self, item)函数
参数名 |
类型 |
介绍 |
默认值 |
item |
object |
需要检查的对象 |
|
类型 |
介绍 |
int |
该对象在该Collection中出现的次数 |
datas = [1, 2, 3, 4, 5, 5, 5]
collection = Collection(0,datas)
print(collection.counter(5))
>>>>3
max(self, action=None)函数
参数名 |
类型 |
介绍 |
默认值 |
action |
function |
对象映射 |
None |
类型 |
介绍 |
int |
该Collection在映射下,最大的一个元素值 |
datas = [1, 2, 3, 4, 5, 5, 5]
collection = Collection(0,datas)
print(collection.max())
>>>>5
min(self, action=None)函数
参数名 |
类型 |
介绍 |
默认值 |
action |
function |
对象映射 |
None |
类型 |
介绍 |
int |
该Collection在映射下,最小的一个元素值 |
datas = [1, 2, 3, 4, 5, 5, 5]
collection = Collection(0,datas)
print(collection.max())
>>>>1
where(self, expression)
函数
参数名 |
类型 |
介绍 |
默认值 |
expression |
function |
对象选取条件 |
|
类型 |
介绍 |
Collection |
符合条件的对象组成的Collection映射 |
class people:
def __init__(self, name, age):
self.name = name
self.age = age
datas = [
people('Alice', 15),
people('Bob', 17),
people('Anda', 15),
people('Wide', 20),
people('Jake', 13)
]
collection = Collection()
for item in datas:
collection += item
result = collection.where(lambda x: x.age >= 15 and len(x.name) > 3)
for item in result:
print(item.name, item.age)
>>>>
Alice 15
Anda 15
Wide 20
delete(self, expression)函数
参数名 |
类型 |
介绍 |
默认值 |
expression |
function |
对象选取条件 |
|
collection = Collection()
for item in datas:
collection += item
collection.delete(lambda x: x.age <= 15)
for item in collection:
print(item.name, item.age)
>>>>
Bob 17
Wide 20
all(self, expression)函数
参数名 |
类型 |
介绍 |
默认值 |
expression |
function |
对象验证条件 |
|
datas = [1, 2, 5, 7, 10, 12, 13]
collection = Collection(0, datas)
print(collection.all(lambda x: x > 5))
>>>>False
firstordefault(self, default=None)函数
参数名 |
类型 |
介绍 |
默认值 |
default |
object |
当Collection为空的时候,函数返回默认值 |
None |
类型 |
介绍 |
object |
该Collection中第一个元素 |
datas = [1, 2, 5, 7, 10, 12, 13]
collection = Collection(0, datas)
print(collection.firstordefault())
>>>>1
first(self)函数
类型 |
介绍 |
object |
该Collection中第一个元素 |
datas = [1, 2, 5, 7, 10, 12, 13]
collection = Collection(0, datas)
print(collection.first())
>>>>1
foreach(self, action)函数
参数名 |
类型 |
介绍 |
默认值 |
action |
function |
元素映射函数 |
|
类型 |
介绍 |
Collection |
映射后的Collection |
datas = [1, 2, 5, 7, 10, 12, 13]
collection = Collection(0, datas)
print(collection.foreach(lambda x: x*x))
>>>>[1, 4, 25, 49, 100, 144, 169]
Collection存在的意义
为该ORM框架添加一个数据集合,能更好的完成查找的过程