spring注解方式
-
- 代码整体布局:
- 代码如下:
-
- pom.xml:
- spring-1.xml:
- Student:
- Test:
- Test2:
- spring-2.xml:
- Car:
- Driver:
- Test:
- spring-3.xml:
- IA:
- ClsA1:
- ClsA2:
- MyCls:
- MyCls2:
- Dog:
- Test:
- Test2:
代码整体布局:

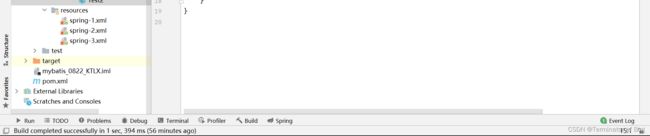
代码如下:
pom.xml:
4.0.0
org.example
mybatis_0822_KTLX
1.0-SNAPSHOT
8
8
org.springframework
spring-context
5.3.18
spring-1.xml:
Student:
package com.test;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
//@Component:用于告诉spring,当前类的对象需要spring来管理。
// 里面的名字就相当于bean标签的id,作为对象的唯一标识
@Component("stu")
public class Student {
private String name;
private String sex;
private Integer age;
public Student() {
System.out.println("=====Student 无参构造=====");
}
public Student(String name, String sex, Integer age) {
this.name = name;
this.sex = sex;
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
System.out.println("========setName========:"+name);
this.name = name;
}
public String getSex() {
return sex;
}
public void setSex(String sex) {
this.sex = sex;
}
public Integer getAge() {
return age;
}
public void setAge(Integer age) {
this.age = age;
}
@Override
public String toString() {
return "Student{" +
"name='" + name + '\'' +
", sex='" + sex + '\'' +
", age=" + age +
'}';
}
}
Test:
package com.test;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import javax.annotation.Resource;
public class Test {
@Resource
private Student stu;
public static void main(String[] args) {
//创建spring容器
ApplicationContext ctx=new ClassPathXmlApplicationContext("spring-1.xml"); //运行结果:=====Student 无参构造===== ========setName========:张三
}
}
Test2:
package com.test;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class Test2 {
public static void main(String[] args) {
//通过注解从spring容器中获取对象
//1.在spring配置文件中,配置包扫描
//2.在需要被spring管理的类上加特定注解
ApplicationContext ctx=new ClassPathXmlApplicationContext("spring-1.xml");
Student stu=ctx.getBean(Student.class);
System.out.println(stu); //运行结果:=====Student 无参构造===== ========setName========:张三 Student{name='张三', sex='男', age=20}
Student stu1=(Student)ctx.getBean("stu");
System.out.println(stu1); //运行结果: Student{name='张三', sex='男', age=20}
}
}
spring-2.xml:
Car:
package com.test2;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
import org.springframework.stereotype.Repository;
import org.springframework.stereotype.Service;
@Component("mycar") //通过注释,可以用在任意对象上
//@Service //本质就是@Component这个注释的别名,用于Service层的对象
//@Repository //本质就是@Component这个注释的别名,用于数据访问层的对象
public class Car {
//品牌
@Value("宝马")
private String brand;
//价格
@Value("2000")
private int price;
//时速
@Value("120")
private int space;
public Car() {
}
public Car(String brand, int price, int space) {
this.brand = brand;
this.price = price;
this.space = space;
}
public void run(){
System.out.println(this.brand+"在跑");
}
public String getBrand() {
return brand;
}
public void setBrand(String brand) {
this.brand = brand;
}
public int getPrice() {
return price;
}
public void setPrice(int price) {
this.price = price;
}
public int getSpace() {
return space;
}
public void setSpace(int space) {
this.space = space;
}
@Override
public String toString() {
return "Car{" +
"brand='" + brand + '\'' +
", price=" + price +
", space=" + space +
'}';
}
}
Driver:
package com.test2;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
import javax.annotation.Resource;
@Component //当注释没有指定id名字的时候,spring自动用类名小驼峰做对象的id名
public class Driver {
//@Value只用于注入普通值
@Value("张三")
private String name;
@Value("20")
private Integer age;
//@Autowired 用于注入对象类型的值(按照类型注入对象,前提是spring容器中有该类型的对象)
// 该注释属于spring框架下的注释。特征是按照类型注入对象
//@Autowired
@Resource
private Car car;
public Driver() {
}
public Driver(String name, Integer age, Car car) {
this.name = name;
this.age = age;
this.car = car;
}
//开车
public void drive(){
System.out.println(this.name+"开车");
this.car.run();
}
public String getName() {
return name;
}
public void setName(String name) {
//注解方式没有调用setter方法
System.out.println("==========setName==============:"+name);
this.name = name;
}
public Integer getAge() {
return age;
}
public void setAge(Integer age) {
this.age = age;
}
public Car getCar() {
return car;
}
public void setCar(Car car) {
this.car = car;
}
@Override
public String toString() {
return "Driver{" +
"name='" + name + '\'' +
", age=" + age +
", car=" + car +
'}';
}
}
Test:
package com.test2;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class Test {
public static void main(String[] args) {
ApplicationContext ctx=new ClassPathXmlApplicationContext("spring-2.xml");
//获取容器中的司机对象
Driver driver=ctx.getBean(Driver.class);
//调用开车的方法
driver.drive(); //运行结果:张三开车 宝马在跑
Car car=ctx.getBean(Car.class);
System.out.println(car); //运行结果:Car{brand='宝马', price=2000, space=120}
Car mycar=(Car)ctx.getBean("mycar");
System.out.println(mycar); //运行结果:Car{brand='宝马', price=2000, space=120}
}
}
spring-3.xml:
IA:
package com.test3;
public interface IA {
void test();
}
ClsA1:
package com.test3;
import org.springframework.stereotype.Component;
@Component
public class ClsA1 implements IA{
@Override
public void test() {
System.out.println("这是A1测试");
}
}
ClsA2:
package com.test3;
import org.springframework.stereotype.Component;
@Component
public class ClsA2 implements IA{
@Override
public void test() {
System.out.println("这是A2测试");
}
}
MyCls:
package com.test3;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.stereotype.Component;
import javax.annotation.Resource;
@Component //当注释没有指定id名字的时候,spring自动用类名小驼峰做对象的id名
public class MyCls {
// @Autowired
// private IA a; //运行结果:ERROR:expected single matching bean but found 2: clsA1,clsA2
// //@Autowired先按类型,再按照名字注入
// @Autowired
// private IA clsA1; //指定名字注入。 ClsA1类名小写clsA1 。
//
// public void show(){
// this.clsA1.test();
// } //运行结果:这是A1测试
//@Autowired先按类型,再按照名字注入
@Autowired
@Qualifier("clsA1") //按名字注入对象a
private IA a;
public void show(){
this.a.test();
} //运行结果:这是A1测试
}
MyCls2:
package com.test3;
import org.springframework.stereotype.Component;
import javax.annotation.Resource;
@Component
public class MyCls2 {
// @Resource(name = "clsA1")
// private IA a;
//
// public void show(){
// this.a.test();
// } //运行结果:这是A1测试
// @Resource
// private IA clsA1; //指定名字注入
//
// public void show(){
// this.clsA1.test();
// } //运行结果:这是A1测试
// //先按名字,再按类型注入
// @Resource
// private IA a;
//
// public void show(){
// this.a.test();
// } //运行结果:BeanNotOfRequiredTypeException: Bean named 'a' is expected to be of type 'com.test3.IA' but was actually of type 'com.test3.Dog'
//先按名字,再按类型注入
@Resource
private IA a;
public void show(){
this.a.test();
} //运行结果:NoUniqueBeanDefinitionException: No qualifying bean of type 'com.test3.IA' available: expected single matching bean but found 2: clsA1,clsA2
}
Dog:
package com.test3;
import org.springframework.stereotype.Component;
//@Component("a")
@Component("dog")
public class Dog {
}
Test:
package com.test3;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class Test {
public static void main(String[] args) {
ApplicationContext ac=new ClassPathXmlApplicationContext("spring-3.xml");
MyCls myCls=ac.getBean(MyCls.class);
// ac.getBean(MyCls.class);
myCls.show();
}
}
Test2:
package com.test3;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class Test2 {
public static void main(String[] args) {
ApplicationContext ac=new ClassPathXmlApplicationContext("spring-3.xml");
MyCls2 myCls2=ac.getBean(MyCls2.class);
myCls2.show();
}
}
// A code block
var foo = 'bar';
// A code block
var foo = 'bar';
// A code block
var foo = 'bar';
// A code block
var foo = 'bar';
// A code block
var foo = 'bar';