曾经被IPhone用手指在屏幕上滑来滑去拖动图片的操作方式吸引吗?在Android里,这并不是什么难事,只要用到Gallery即可。它通常用在设计相册、图片类型的选择器上。
在开始之前,先了解一下什么是Context以及Android.Widget命名空间下的BaseAdapter,在Activity当中,Context就如同是Canvas画布,随时等着被处理或覆盖。还记得先前介绍Activity之间的传递时,做过Intent以及Context的应用吗?当中Intent是Android.Content命名空间下的一个类,而Context也是一样。本范例在Layout里布局一个Gallery对象,再通过BaseAdapter容器存放Gallery所需要的图片。先将你要显示的图片放到Drawable文件夹下,然后编译一下工程,使其能在代码中使用。
Main.axml的结构很简单,只要放上一个Gallery即可:
- <?xml version="1.0" encoding="utf-8"?>
- <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
- android:orientation="vertical"
- android:layout_width="fill_parent"
- android:layout_height="fill_parent"
- >
- <Gallery android:id="@+id/gallery" android:layout_height="fill_parent" android:layout_width="fill_parent"></Gallery>
- </LinearLayout>
本范例的另一个重点,就是如何设置Gallery图片的宽高以及放置图片Layout的大小,在此我们新建一个ImageAdapter类来继承BaseAdapter容器来存放图片,通过ImageView的SetScaleType方法来改变图片的显示,再通过LayoutParameters属性类改变Layout的宽高。
我们先在Values目录下新建一个attrs.xml文件,这是一个自制Layout元素的用法,在其中定义<declare-styleable>标签,目的是自定义layout的背景风格,并且通过Android.Content.Res.TypedArray类的特性,让相同的Layout元素可以重复用于每一张图片。
- <?xml version="1.0" encoding="utf-8"?>
- <resources>
- <declare-styleable name="Gallery">
- <attr name="android:galleryItemBackground" />
- </declare-styleable>
- </resources>
ImageAdapter类的结构如下:
- public class ImageAdapter : BaseAdapter
- {
- private Context ctx;
- int mGalleryItemBackground;
- private int[] images =
- {
- Resource.Drawable.btn1,
- Resource.Drawable.btn1_b,
- Resource.Drawable.btn2,
- Resource.Drawable.btn2_b,
- Resource.Drawable.btn3,
- Resource.Drawable.btn3_b,
- Resource.Drawable.btn4,
- Resource.Drawable.btn4_b
- };
- public ImageAdapter(Context ctx)
- {
- try
- {
- this.ctx = ctx;
- Android.Content.Res.TypedArray a = ctx.ObtainStyledAttributes(Resource.Styleable.Gallery);
- mGalleryItemBackground = a.GetResourceId(Resource.Styleable.Gallery_android_galleryItemBackground, Android.Graphics.Color.Azure);
- a.Recycle();
- }
- catch (System.Exception ex)
- {
- MessageBox.ShowErrorMessage(ctx, ex);
- }
- }
- public override int Count
- {
- get
- {
- return images.Length;
- }
- }
- public override Java.Lang.Object GetItem(int position)
- {
- return position;
- }
- public override long GetItemId(int position)
- {
- return position;
- }
- public override View GetView(int position, View convertView, ViewGroup parent)
- {
- try
- {
- ImageView v = new ImageView(this.ctx);
- v.SetImageResource(this.images[position]);
- v.SetScaleType(ImageView.ScaleType.FitXy);
- v.LayoutParameters = new Gallery.LayoutParams(100, 50);
- v.SetBackgroundResource(mGalleryItemBackground);
- return v;
- }
- catch (System.Exception ex)
- {
- MessageBox.ShowErrorMessage(this.ctx, ex);
- return null;
- }
- }
- }
Activity1.cs的代码:
- [Activity(Label = "MonoDroidTest", MainLauncher = true)]
- public class Activity1 : Activity
- {
- protected override void OnCreate(Bundle bundle)
- {
- base.OnCreate(bundle);
- SetContentView(Resource.Layout.Main);
- try
- {
- Gallery mGallery = FindViewById<Gallery>(Resource.Id.gallery);
- mGallery.Adapter = new ImageAdapter(this);
- }
- catch (System.Exception ex)
- {
- MessageBox.ShowErrorMessage(this, ex);
- }
- }
- }
运行程序,效果如下:
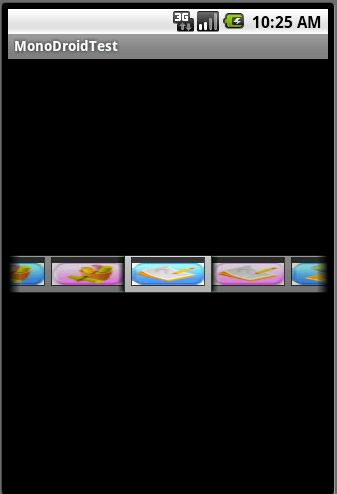
接下来的问题就是,我们能不能读取我们SD卡上的图片,而不是直接把图片作为资源打到包里啊?这当然是可以的,下面我们把ImageAdapter改写一下,从下面的例子,我们还可以学到如何读取SD卡的文件(记得using Java.IO;):
- public class ImageAdapter : BaseAdapter
- {
- private Context ctx;
- int mGalleryItemBackground;
- File[] files;
- int width, height;
- public ImageAdapter(Context ctx)
- {
- try
- {
- this.ctx = ctx;
- DisplayMetrics dm = new DisplayMetrics();
- ((Activity)this.ctx).WindowManager.DefaultDisplay.GetMetrics(dm);
- width = dm.WidthPixels;
- height = dm.HeightPixels;
- if (Android.OS.Environment.ExternalStorageState.Equals(Android.OS.Environment.MediaMounted))
- {
- File path = Android.OS.Environment.ExternalStorageDirectory;
- File file = new File(path.Path + "/Pictures/Camera");
- if (file.Exists())
- files = file.ListFiles();
- else
- throw new System.Exception("SD卡中不存在此路径");
- }
- else if (Android.OS.Environment.ExternalStorageState.Equals(Android.OS.Environment.MediaRemoved))
- throw new System.Exception("没有SD卡");
- Android.Content.Res.TypedArray a = ctx.ObtainStyledAttributes(Resource.Styleable.Gallery);
- mGalleryItemBackground = a.GetResourceId(Resource.Styleable.Gallery_android_galleryItemBackground, Android.Graphics.Color.Azure);
- a.Recycle();
- }
- catch (System.Exception ex)
- {
- MessageBox.ShowErrorMessage(ctx, ex);
- }
- }
- public override int Count
- {
- get
- {
- if (files == null)
- return 0;
- return this.files.Length;
- }
- }
- public override Java.Lang.Object GetItem(int position)
- {
- return position;
- }
- public override long GetItemId(int position)
- {
- return position;
- }
- public override View GetView(int position, View convertView, ViewGroup parent)
- {
- try
- {
- ImageView v = new ImageView(this.ctx);
- Bitmap bmp = BitmapFactory.DecodeFile(files[position].Path);
- v.SetImageBitmap(bmp);
- v.SetScaleType(ImageView.ScaleType.FitXy);
- v.LayoutParameters = new Gallery.LayoutParams(width, height);
- v.SetBackgroundResource(mGalleryItemBackground);
- return v;
- }
- catch (System.Exception ex)
- {
- MessageBox.ShowErrorMessage(this.ctx, ex);
- return null;
- }
- }
- }
然后我们在增加一点小功能,就是在点击图片的时候,弹出个小提示,提示你点击的是哪张图片。这里用到了Toast对象,其实Toast对象我们在第四篇文章介绍布局的时候就已经用过了,但没有具体介绍过它,Toast是Android专属的提示小对象,它的使用方法相当简单,但用途却很广泛。基本上,Toast就是一个简短的小信息,将要告诉用户的信息以一个浮动在最上层的View显示,显示Toast之后,静待几秒就会自动消失,最常见的应用就是音量大小的调整。当单击音量调整按钮之后,会看见跳出的音量指示Toast对象,等待调整完之后便会消失。
- Gallery mGallery = FindViewById<Gallery>(Resource.Id.gallery);
- mGallery.Adapter = new ImageAdapter(this);
- mGallery.ItemClick += (sender, e) =>
- {
- Toast.MakeText(this, string.Format("你点击的是{0}号图片", e.Position), ToastLength.Short).Show();
- };
运行后的效果:
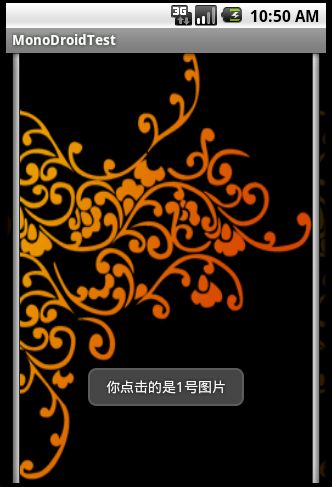
Toast显示后会在一定时间内消失,在Toast.MakeText方法的第三个参数是一个ToastLength枚举,颗选择Long或Short,前者时间较长,后者较短。
当然,你也可以使用重写Toast对象的方法,自定义Toast显示的Layout,以不同于系统内置的方式显示Toast对象,如要在Toast里显示图片,方式如下:
- Gallery mGallery = FindViewById<Gallery>(Resource.Id.gallery);
- mGallery.Adapter = new ImageAdapter(this);
- mGallery.ItemClick += (sender, e) =>
- {
- Toast t = new Toast(this);
- ImageView img = new ImageView(this);
- img.SetImageResource(Android.Resource.Drawable.IcMenuCamera);
- t.View = img;
- t.Show();
-
- };
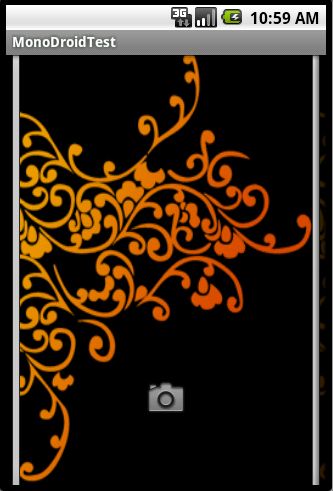
最后说一下BitmapFactory(位于Android.Graphics命名空间下),它是Android API提供的对象,可以将图片文件转换成Bitmap对象,范例中使用的DecodeFile()方法可以将手机系统中的图片文件转换成为Bitmap对象。另外,BitmapFactory也提供了其他的方法,如DecodeResource可以将预先存入的图片文件转换成Bitmap对象,DecodeStream方法则可以将InputStream转换成Bitmap对象。