XML定義:
XML(extensible Markup Language)即可扩展标记语言。
XML舉例:
<?
xml version="1.0" encoding="utf-8"
?>
<
Books
>
<
book
>
<
title
>
Beginning Visual C#
</
title
>
<
author
>
Karli Watson
</
author
>
<
code
>
7582
</
code
>
</
book
>
<
book
Pages
="1000"
>
<!--
This book is the book you are reading
-->
<
title
>
Beginning Visual C# 3rd Edition
</
title
>
<
author
>
Karli Watson et al
</
author
>
<
code
>
123456789
</
code
>
</
book
>
</
Books
>
XML主要結點類型:
結點類型在XML文件中對應如下:
XML操作的動作:
Xml操作的主要流程為:
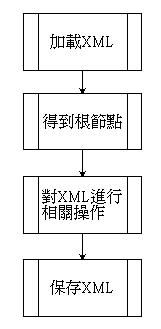
Xpath語言:
XPath是XML文檔的查詢語言。XML相關於數據庫,Xpath就是查詢數據的語言,即相當於SQL。詳細資料可參考(http://www.w3school.com.cn/xpath/index.asp)
Xpath常見的表達式如下:
表达式 |
描述 |
|
nodename |
选取此节点的所有子节点 |
|
/ |
从根节点选取 |
|
|
// |
从匹配选择的当前节点选择文档中的节点,而不考虑它们的位置 |
|
|
. |
选取当前节点 |
|
.. |
选取当前节点的父节点 |
|
* |
選擇任何節點 |
|
@ |
选取属性 |
|
@* |
選擇所有屬性 |
|
athor[2] |
按照索引選擇一個子節點,這裡選擇第二個author節點 |
|
text() |
選擇當前節點中所有文本節點 |
|
author/text() |
選擇當前節點的一個或多個子節點 |
|
//title |
在文檔中選擇帶有特定名稱的所有節點,在這裡是所有的title節點 |
|
//@author |
选取所有名为author的属性。 |
|
//book/title |
在文檔中選擇帶有特定和特定父節點的所有節點,在這裡父節點名稱是Book,節點名稱是title |
|
//book[author=’Jacob’] |
選擇滿足條件的節點,這是authoer節點為’Jacob’的值 |
|
//book[@pages=’10’] |
選擇屬性值滿足條件的節點,這裡是page屬性為10的值 |
|
//book/title | //book/ author |
选取所有 book 元素的 tilte 和 author元素。 |
|
例一、實現下圖功能。
using
System.Xml;
using
System.IO;
namespace
XmlOperate
{
public
partial
class
Form1 : Form
{
public
Form1()
{
InitializeComponent();
//
得到XML內容按鈕事件
btnGet.Click
+=
new
EventHandler(btnGet_Click);
//
創建Node按鈕事件
btnCreateNode.Click
+=
new
EventHandler(btnCreateNode_Click);
//
刪除節點按鈕事件
btnDeleteNode.Click
+=
new
EventHandler(btnDeleteNode_Click);
}
///
<summary>
///
得到XML內容按鈕事件方法
///
</summary>
///
<param name="sender"></param>
///
<param name="e"></param>
void
btnGet_Click(
object
sender, EventArgs e)
{
lbXmlValue.Items.Clear();
if
(File.Exists(
@"
D:\BegVCSharp\book.xml
"
))
{
//
加載XML文件
XmlDocument xdDocument
=
new
XmlDocument();
xdDocument.Load(
@"
D:\BegVCSharp\book.xml
"
);
//
得到主節點
XmlElement xeRoot
=
xdDocument.DocumentElement;
if
(xeRoot
!=
null
)
{
XmlNode xnNodeRoot
=
(XmlNode)xeRoot;
RecurseXmlDocument(xnNodeRoot,
0
);
}
}
}
///
<summary>
///
讀取XML
///
</summary>
///
<param name="aXnNode">
節點
</param>
///
<param name="aIndent">
縮進大小
</param>
private
void
RecurseXmlDocument(XmlNode aXnNode,
int
aIndent)
{
//
判斷結點中是否有內容
if
(aXnNode
==
null
)
{
return
;
}
//
節點是元素時
if
(aXnNode
is
XmlElement)
{
//
顯示根元素的名稱
lbXmlValue.Items.Add(aXnNode.Name.PadLeft(aXnNode.Name.Length
+
aIndent));
if
(aXnNode.Attributes
!=
null
)
{
//
得到屬性
foreach
(XmlAttribute xaAttribute
in
aXnNode.Attributes)
{
string
sText
=
""
;
sText
=
xaAttribute.Name;
lbXmlValue.Items.Add(sText.PadLeft(sText.Length
+
aIndent
+
2
));
sText
=
xaAttribute.Value;
lbXmlValue.Items.Add(sText.PadLeft(sText.Length
+
aIndent
+
4
));
}
}
//
根元素中是否有子元素
if
(aXnNode.HasChildNodes)
{
//
有子節點,遍歷子節點
RecurseXmlDocument(aXnNode.FirstChild, aIndent
+
2
);
}
//
判斷下個節點是為空
if
(aXnNode.NextSibling
!=
null
)
{
RecurseXmlDocument(aXnNode.NextSibling, aIndent);
}
}
else
if
(aXnNode
is
XmlText)
{
//
顯示節點中的內容
string
sText
=
((XmlText)aXnNode).Value;
lbXmlValue.Items.Add(sText.PadLeft(sText.Length
+
aIndent));
}
else
if
(aXnNode
is
XmlComment)
{
string
sText
=
aXnNode.Value;
lbXmlValue.Items.Add(sText.PadLeft(sText.Length
+
aIndent));
//
如果不加下邊的遍歷,資料只會得出備註中的內容,不會得出子節點內容
if
(aXnNode.HasChildNodes)
{
//
有子節點,遍歷子節點
RecurseXmlDocument(aXnNode.FirstChild, aIndent
+
2
);
}
if
(aXnNode.NextSibling
!=
null
)
{
RecurseXmlDocument(aXnNode.NextSibling, aIndent);
}
}
}
///
<summary>
///
創建Node按鈕事件方法
///
</summary>
///
<param name="sender"></param>
///
<param name="e"></param>
void
btnCreateNode_Click(
object
sender, EventArgs e)
{
//
加載XML文件
XmlDocument xdDocument
=
new
XmlDocument();
xdDocument.Load(
@"
D:\BegVCSharp\book.xml
"
);
//
得到主節點
XmlElement xeRoot
=
xdDocument.DocumentElement;
//
創建節點
XmlElement newBook
=
xdDocument.CreateElement(
"
book
"
);
XmlElement newTitle
=
xdDocument.CreateElement(
"
title
"
);
XmlElement newAuthor
=
xdDocument.CreateElement(
"
author
"
);
XmlElement newCode
=
xdDocument.CreateElement(
"
code
"
);
//
創建屬性
XmlAttribute xaNewAttribute
=
xdDocument.CreateAttribute(
"
Pages
"
);
xaNewAttribute.Value
=
"
1000
"
;
XmlText title
=
xdDocument.CreateTextNode(
"
Beginning Visual C# 3rd Edition
"
);
XmlText author
=
xdDocument.CreateTextNode(
"
Karli Watson et al
"
);
XmlText code
=
xdDocument.CreateTextNode(
"
123456789
"
);
//
創建備註
XmlComment comment
=
xdDocument.CreateComment(
"
This book is the book you are reading
"
);
//
元素插入XML樹中
newBook.AppendChild(comment);
newBook.Attributes.Append(xaNewAttribute);
newBook.AppendChild(newTitle);
newBook.AppendChild(newAuthor);
newBook.AppendChild(newCode);
newTitle.AppendChild(title);
newAuthor.AppendChild(author);
newCode.AppendChild(code);
//
插入某節點後邊
xeRoot.InsertAfter(newBook, xeRoot.FirstChild);
//
保存結果
xdDocument.Save(
@"
D:\BegVCSharp\book.xml
"
);
}
///
<summary>
///
刪除節點按鈕事件
///
</summary>
///
<param name="sender"></param>
///
<param name="e"></param>
void
btnDeleteNode_Click(
object
sender, EventArgs e)
{
//
加載XML文件
XmlDocument xdDocument
=
new
XmlDocument();
xdDocument.Load(
@"
D:\BegVCSharp\book.xml
"
);
//
得到主節點
XmlElement xeRoot
=
xdDocument.DocumentElement;
if
(xeRoot.HasChildNodes)
{
//
得到最後一個節點
XmlNode xnBook
=
xeRoot.LastChild;
//
刪除最後一個結點
xeRoot.RemoveChild(xnBook);
//
保存結果
xdDocument.Save(
@"
D:\BegVCSharp\book.xml
"
);
}
}
}
}
例二、用Xpath執行相關查詢
using
System.Xml;
namespace
XmlIoManage
{
public
partial
class
Form1 : Form
{
public
Form1()
{
InitializeComponent();
string
sXmlPath
=
@"
D:\BegVCSharp\XPathQuery.xml
"
;
XmlDocument xdDocument
=
new
XmlDocument();
xdDocument.Load(sXmlPath);
XmlNode xnNode
=
xdDocument.DocumentElement;
GetXmlListAttribute(xnNode,
"
//@pages
"
);
GetXmlElementList(xnNode,
"
//book
"
);
}
///
<summary>
///
得到指定結點的Text值
///
</summary>
///
<param name="aXnNode"></param>
///
<param name="asAttributeFilter"></param>
private
void
GetXmlElementList(XmlNode aXnNode,
string
asAttributeFilter)
{
List
<
string
>
lElementTextlist
=
new
List
<
string
>
();
if
(aXnNode
==
null
)
{
return
;
}
XmlNodeList xnlNodeList
=
aXnNode.SelectNodes(asAttributeFilter);
lElementTextlist
=
ReadXmlElementText(xnlNodeList);
}
///
<summary>
///
讀取節點Text值
///
</summary>
///
<param name="aXnlNodeList"></param>
///
<returns></returns>
private
List
<
string
>
ReadXmlElementText(XmlNodeList aXnlNodeList)
{
List
<
string
>
lElementTextlist
=
new
List
<
string
>
();
if
(aXnlNodeList
==
null
)
{
return
lElementTextlist;
}
foreach
(XmlNode xnNode
in
aXnlNodeList)
{
string
sText
=
RecurseXmlDocument(xnNode);
lElementTextlist.Add(sText);
}
return
lElementTextlist;
}
string
sNodeInderText
=
""
;
///
<summary>
///
返回第一個Text值
///
</summary>
///
<param name="aXnNode"></param>
///
<returns></returns>
private
string
RecurseXmlDocument(XmlNode aXnNode)
{
if
(aXnNode
==
null
)
{
return
""
;
}
if
(aXnNode
is
XmlElement)
{
if
(aXnNode.HasChildNodes)
{
RecurseXmlDocument(aXnNode.FirstChild);
}
}
else
if
(aXnNode
is
XmlText)
{
sNodeInderText
=
((XmlText)aXnNode).Value;
}
sNodeInderText
+=
sNodeInderText.Trim();
return
sNodeInderText;
}
///
<summary>
///
得到所有指定屬性的值
///
</summary>
///
<param name="aXnNode">
aXnNode
</param>
///
<param name="asAttributeFilter">
屬性過慮值
</param>
private
void
GetXmlListAttribute(XmlNode aXnNode,
string
asAttributeFilter)
{
//
存放得到屬性列表
List
<
string
>
lAttributeList
=
new
List
<
string
>
();
if
(aXnNode
==
null
)
{
return
;
}
XmlNodeList xnlNodeList
=
aXnNode.SelectNodes(asAttributeFilter);
lAttributeList
=
ReadXmlAttributeList(xnlNodeList);
}
///
<summary>
///
得到屬性列表
///
</summary>
///
<param name="aXaNodeList">
XmlNodeList
</param>
///
<returns>
得到的屬性列表
</returns>
private
List
<
string
>
ReadXmlAttributeList(XmlNodeList aXaNodeList)
{
//
存放得到屬性列表
List
<
string
>
lAttributeList
=
new
List
<
string
>
();
if
(aXaNodeList
==
null
)
{
return
lAttributeList;
}
foreach
(XmlNode xnNode
in
aXaNodeList)
{
if
(xnNode
is
XmlAttribute)
{
XmlAttribute xaAttribute
=
(XmlAttribute)xnNode;
lAttributeList.Add(xaAttribute.Value);
}
}
return
lAttributeList;
}
///
<summary>
///
選擇單個結點屬性
///
</summary>
///
<param name="aXnNode">
被查詢的Xml
</param>
///
<param name="asAttributeFilter">
Xpth條件
</param>
private
void
GetXmlSingleAttribute(XmlNode aXnNode,
string
asAttributeFilter)
{
if
(aXnNode
==
null
)
{
return
;
}
XmlNode xnSelectNode
=
aXnNode.SelectSingleNode(asAttributeFilter);
string
sResult
=
ReadXmlSingleAttribute(xnSelectNode);
}
///
<summary>
///
讀取XML單點的屬性方法
///
</summary>
///
<param name="aXnNode">
XmlNode
</param>
///
<returns>
得到的屬性值
</returns>
private
string
ReadXmlSingleAttribute(XmlNode aXnNode)
{
string
sRetrunValue
=
""
;
if
(aXnNode
==
null
)
{
return
sRetrunValue;
}
if
(aXnNode
is
XmlElement)
{
if
(aXnNode.Attributes
!=
null
)
{
foreach
(XmlAttribute xaAttribute
in
aXnNode.Attributes)
{
sRetrunValue
=
xaAttribute.Value;
}
}
}
return
sRetrunValue;
}
}
}