问题描述
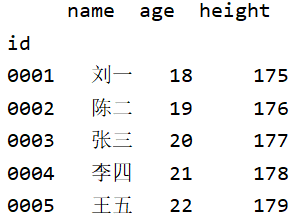
import pandas as pd
data = {
'name': ['刘一', '陈二', '张三', '李四', '王五'],
'age': [18, 19, 20, 21, 22],
'height': [175, 176, 177, 178, 179],
}
index = ['0001', '0002', '0003', '0004', '0005']
df = pd.DataFrame(data=data, index=index)
df.index.name = 'id'
print(df)
按行遍历
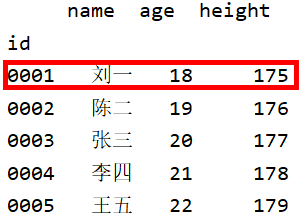
import pandas as pd
data = {
'name': ['刘一', '陈二', '张三', '李四', '王五'],
'age': [18, 19, 20, 21, 22],
'height': [175, 176, 177, 178, 179],
}
index = ['0001', '0002', '0003', '0004', '0005']
df = pd.DataFrame(data=data, index=index)
df.index.name = 'id'
for index, x in df.iterrows():
print(index, x['name'], x['age'], x['height'])
print()
columns = df.columns
for index, x in df.iterrows():
print(index, end=' ')
for column in columns:
print(x[column], end=' ')
print()
print()
for index, x in df.iterrows():
print(index, x.to_dict())
print({index: x.to_dict() for index, x in df.iterrows()})
按列遍历
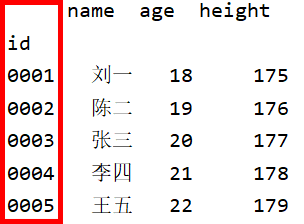
import pandas as pd
data = {
'name': ['刘一', '陈二', '张三', '李四', '王五'],
'age': [18, 19, 20, 21, 22],
'height': [175, 176, 177, 178, 179],
}
index = ['0001', '0002', '0003', '0004', '0005']
df = pd.DataFrame(data=data, index=index)
df.index.name = 'id'
print(df.index.name, end=' ')
for index in df.index:
print(index, end=' ')
print()
print()
for column, x in df.items():
print(column, end=' ')
for i in x:
print(i, end=' ')
print()
print()
for column in df.columns:
print(column, end=' ')
for i in df[column]:
print(i, end=' ')
print()
print()
for column, x in df.items():
print(f'index_{column}_map', x.to_dict())
参考文献
- pandas.DataFrame Documentation
- 10分钟了解Pandas基础知识
- Pandas index column title or name