1.录制视频并保存
import datetime
import cv2
fourcc = cv2.VideoWriter_fourcc(*'DIVX')
vw = cv2.VideoWriter(".\\out1.mp4",fourcc,25,(640,480))
cv2.namedWindow('video',cv2.WINDOW_NORMAL)
cv2.resizeWindow('video',640,480)
cap = cv2.VideoCapture(0)
flag = 0
while cap.isOpened():
ret,frame = cap.read()
if ret ==True :
timer = cv2.getTickCount()
fps = cv2.getTickFrequency() / (cv2.getTickCount() - timer)
cv2.putText(frame, "FPS : " + str(int(fps)), (100, 50), cv2.FONT_HERSHEY_SIMPLEX, 0.85, (150, 170, 50), 2, cv2.LINE_AA)
datet = str(datetime.datetime.now())[0:19]
cv2.putText(frame, "Time:" + datet, (100, 20), cv2.FONT_HERSHEY_SIMPLEX, 0.85,(0, 255, 255), 2, cv2.LINE_AA)
if flag ==0:
cv2.putText(frame, "image collecting~" , (100, 80), cv2.FONT_HERSHEY_SIMPLEX, 0.85,(0, 255, ), 2, cv2.LINE_AA)
cv2.imshow('video',frame)
cv2.resizeWindow('video',640,480)
key = cv2.waitKey(1)
if flag ==1:
cv2.putText(frame, "image saving~" , (100, 80), cv2.FONT_HERSHEY_SIMPLEX, 0.85,(0, 255, 0), 2, cv2.LINE_AA)
cv2.imshow('video',frame)
cv2.resizeWindow('video',640,480)
cv2.waitKey(1 )
vw.write(frame)
if key == 32:
vw.write(frame)
flag = 1
elif (key &0xFF == ord('s')):
flag =0
print('停止保存图像')
elif(key & 0xFF == ord('q')):
print('结束采集图像')
break
else:
break
cap.release()
vw.release()
cv2.destroyAllWindows()
2.读取保存的视频
from openni import openni2
import numpy as np
import cv2
cv2.namedWindow('video',cv2.WINDOW_NORMAL)
video = cv2.VideoCapture('.\\out1.mp4')
while video.isOpened():
open,frame = video.read()
if open:
cv2.imshow('video',frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
else:
break
video.release()
cv2.destroyAllWindows()
录制效果如下:
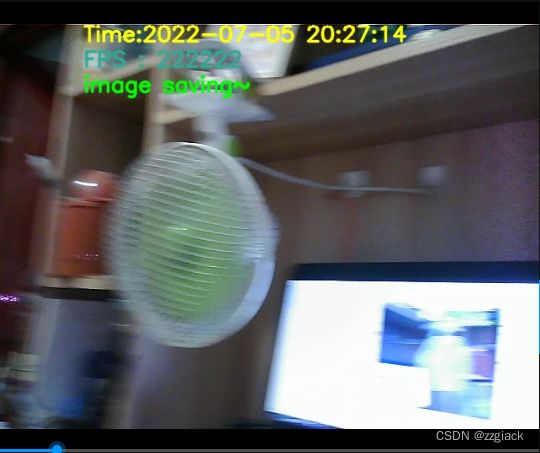