图像特征检测与图像分割
1. 图像的角点检测
import numpy as np
from matplotlib import pylab as plt
from skimage.io import imread
from skimage.color import rgb2gray
from skimage.feature import corner_harris
image = imread('images/building.jpg')
plt.figure(figsize=(12,6))
plt.subplot(121)
plt.imshow(image)
plt.axis('off')
image_gray = rgb2gray(image)
coords = corner_harris(image_gray, k =0.001)
image[coords>0.01*coords.max()]=[255,0,255]
plt.subplot(122)
plt.imshow(image)
plt.axis('off')
plt.show()
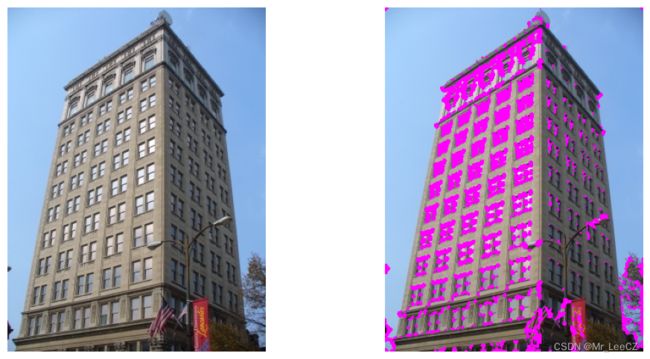
2. 图像分割
import matplotlib.pyplot as plt
import numpy as np
from skimage.io import imread
from skimage.segmentation import felzenszwalb, slic, quickshift, watershed
from skimage.segmentation import mark_boundaries, find_boundaries
from skimage.util import img_as_float
from matplotlib.colors import LinearSegmentedColormap
for imfile in ['images/eagle.png', 'images/horses.png', 'images/flowers.png', 'images/bisons.png']:
img = img_as_float(imread(imfile)[::2, ::2, :3])
plt.figure(figsize=(20,10))
segments_fz = felzenszwalb(img, scale=100, sigma=0.5, min_size=100)
borders = find_boundaries(segments_fz)
unique_colors = np.unique(segments_fz.ravel())
segments_fz[borders] = -1
colors = [np.zeros(3)]
for color in unique_colors:
colors.append(np.mean(img[segments_fz == color], axis=0))
cm = LinearSegmentedColormap.from_list('pallete', colors, N=len(colors))
plt.subplot(121)
plt.imshow(img)
plt.title('Original', size=20)
plt.axis('off')
plt.subplot(122)
plt.imshow(segments_fz, cmap=cm)
plt.title('Segmented with Felzenszwalbs\'s method', size=20)
plt.axis('off')
plt.tight_layout()
plt.show()
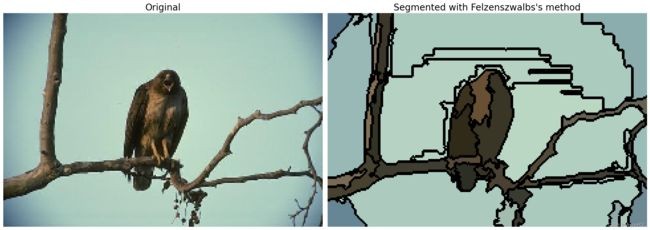
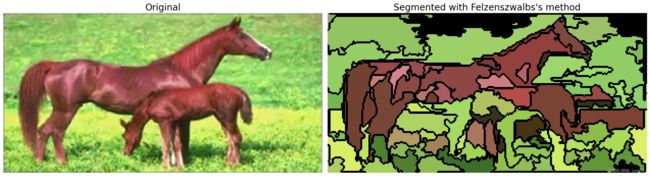
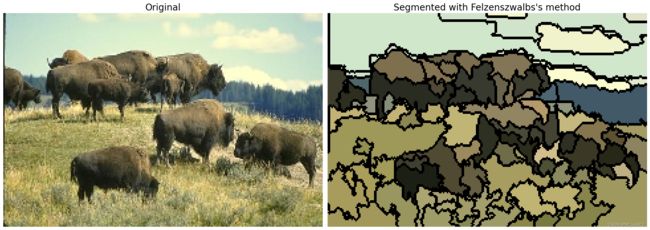