OpenCV Python 理解SVM 代码示意
【代码】
简单线性
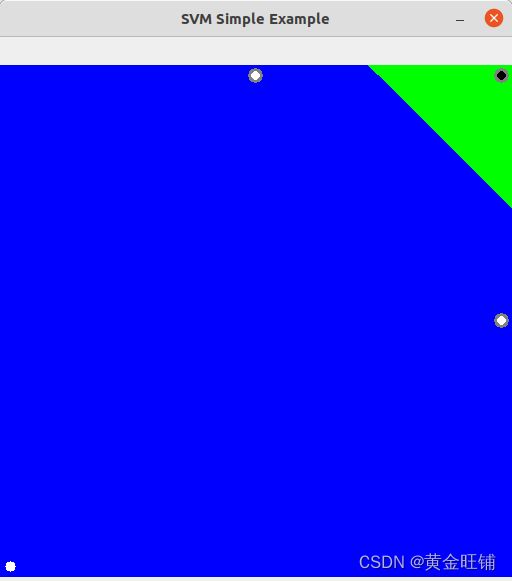
import cv2
import numpy as np
width = 512
height = 512
image = np.zeros((height, width, 3), dtype=np.uint8)
labels = np.array([1, -1, -1, -1])
trainingData = np.matrix(
[[501, 10], [255, 10], [501, 255], [10, 501]], dtype=np.float32)
svm = cv2.ml.SVM_create()
svm.setType(cv2.ml.SVM_C_SVC)
svm.setKernel(cv2.ml.SVM_LINEAR)
svm.setTermCriteria((cv2.TERM_CRITERIA_MAX_ITER, 100, 1e-6))
svm.train(trainingData, cv2.ml.ROW_SAMPLE, labels)
green = (0, 255, 0)
blue = (255, 0, 0)
for i in range(image.shape[0]):
for j in range(image.shape[1]):
sampleMat = np.matrix([[j, i]], dtype=np.float32)
response = svm.predict(sampleMat)[1]
if response == 1:
image[i, j] = green
elif response == -1:
image[i, j] = blue
thickness = -1
cv2.circle(image, (501, 10), 5, (0, 0, 0), thickness)
cv2.circle(image, (255, 10), 5, (255, 255, 255), thickness)
cv2.circle(image, (501, 255), 5, (255, 255, 255), thickness)
cv2.circle(image, (10, 501), 5, (255, 255, 255), thickness)
thickness = 2
sv = svm.getUncompressedSupportVectors()
for i in range(sv.shape[0]):
cv2.circle(image, (int(sv[i, 0]), int(sv[i, 1])),
6, (128, 128, 128), thickness)
cv2.imshow('SVM Simple Example', image)
cv2.waitKey()
cv2.destroyAllWindows()
非线性数据
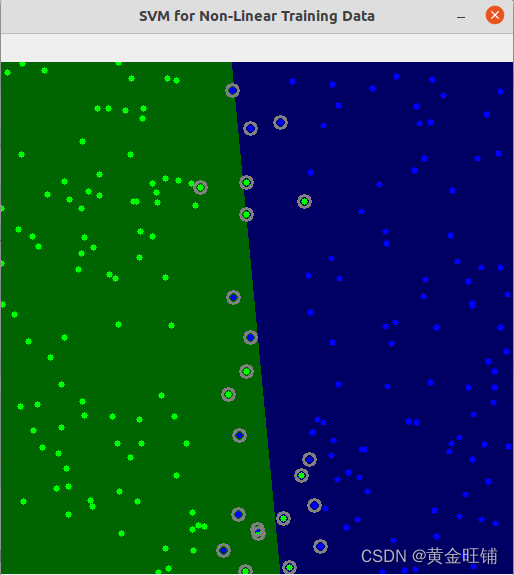
import cv2
import numpy as np
import random as rng
NTRAINING_SAMPLES = 100
FRAC_LINEAR_SEP = 0.9
WIDTH = 512
HEIGHT = 512
I = np.zeros((HEIGHT, WIDTH, 3), dtype=np.uint8)
trainData = np.empty((2*NTRAINING_SAMPLES, 2), dtype=np.float32)
labels = np.empty((2*NTRAINING_SAMPLES, 1), dtype=np.int32)
rng.seed(100)
nLinearSamples = int(FRAC_LINEAR_SEP * NTRAINING_SAMPLES)
trainClass = trainData[0:nLinearSamples,:]
c = trainClass[:,0:1]
c[:] = np.random.uniform(0.0, 0.4 * WIDTH, c.shape)
c = trainClass[:,1:2]
c[:] = np.random.uniform(0.0, HEIGHT, c.shape)
trainClass = trainData[2*NTRAINING_SAMPLES-nLinearSamples:2*NTRAINING_SAMPLES,:]
c = trainClass[:,0:1]
c[:] = np.random.uniform(0.6*WIDTH, WIDTH, c.shape)
c = trainClass[:,1:2]
c[:] = np.random.uniform(0.0, HEIGHT, c.shape)
trainClass = trainData[nLinearSamples:2*NTRAINING_SAMPLES-nLinearSamples,:]
c = trainClass[:,0:1]
c[:] = np.random.uniform(0.4*WIDTH, 0.6*WIDTH, c.shape)
c = trainClass[:,1:2]
c[:] = np.random.uniform(0.0, HEIGHT, c.shape)
labels[0:NTRAINING_SAMPLES,:] = 1
labels[NTRAINING_SAMPLES:2*NTRAINING_SAMPLES,:] = 2
print('Starting training process')
svm = cv2.ml.SVM_create()
svm.setType(cv2.ml.SVM_C_SVC)
svm.setC(0.1)
svm.setKernel(cv2.ml.SVM_LINEAR)
svm.setTermCriteria((cv2.TERM_CRITERIA_MAX_ITER, int(1e7), 1e-6))
svm.train(trainData, cv2.ml.ROW_SAMPLE, labels)
print('Finished training process')
green = (0,100,0)
blue = (100,0,0)
for i in range(I.shape[0]):
for j in range(I.shape[1]):
sampleMat = np.matrix([[j,i]], dtype=np.float32)
response = svm.predict(sampleMat)[1]
if response == 1:
I[i,j] = green
elif response == 2:
I[i,j] = blue
thick = -1
for i in range(NTRAINING_SAMPLES):
px = trainData[i,0]
py = trainData[i,1]
cv2.circle(I, (int(px), int(py)), 3, (0, 255, 0), thick)
for i in range(NTRAINING_SAMPLES, 2*NTRAINING_SAMPLES):
px = trainData[i,0]
py = trainData[i,1]
cv2.circle(I, (int(px), int(py)), 3, (255, 0, 0), thick)
thick = 2
sv = svm.getUncompressedSupportVectors()
for i in range(sv.shape[0]):
cv2.circle(I, (int(sv[i,0]), int(sv[i,1])), 6, (128, 128, 128), thick)
cv2.imshow('SVM for Non-Linear Training Data', I)
cv2.waitKey()
cv2.destroyAllWindows()
【参考】
- Introduction to Support Vector Machines
- Support Vector Machines for Non-Linearly Separable Data
- 理解SVM