- win10 无法访问samba文件,提示SMB1是不安全协议解决方案
wellnw
Openwrt
方式一:修改win10,增加SMB1支持https://blog.csdn.net/maxzero/article/details/81410620方式二:修改samba服务配置ssh登录到路由后台,执行sed-i's/security=share/security=user/'/etc/samba/smb.conf.template/etc/init.d/sambarestartwin10不开启
- 从数据到情感:全维度解析哪吒2的212亿票房之战
数据分析
综合目前的数据来看,我分析一下哪吒2的最终票房和冲击第一名可能性。当前态势:票房现状说明目前票房:110亿国内贡献:90%以上(约108亿)海外表现:仅2300万已上映:春节档15天左右三条预测路径分析(含日均计算)A.基础预测线(160-170亿)目标缺口:50-60亿时间周期:45天具体路径:第一阶段(15天)日均要求:2亿阶段贡献:30亿工作日表现:1.5亿/天周末表现:3亿/天第二阶段(1
- 揭秘!100 个 Python 常用易错知识点的避坑指南
tekin
PythonpythonPython易错点Python编程避坑Python知识总结Python基础与进阶Python代码优化Python常见错误解析
目录简介1.类方法命名中的下划线2.函数形参中的*和**3.函数实参中的*4.变量作用域5.浅拷贝和深拷贝6.默认参数的陷阱7.迭代器和生成器相关迭代器使用后耗尽生成器表达式和列表推导式混淆8.异常处理相关捕获异常范围过大异常处理中的finally子句9.多线程和多进程相关全局解释器锁(GIL)误解多线程性能提升多进程中的资源共享问题10.字符串编码问题编码和解码错误11.模块导入相关循环导入问题
- yolov8(8.2.10)+deepsort(demo)
fengsongdehappy
YOLO
只需要训练好yolov8的检测模型然后调用:results=model.track(frame,persist=True)#执行跟踪,persist=True表示持续跟踪。保持同一个人在多帧画面的id一就可以完整代码:importcv2importnumpyasnpfromultralyticsimportYOLOfromcollectionsimportdefaultdict#框的中心点的历史轨
- vue-plugin-hiprint (vue2
_未知_开摆
vue.jsjavascriptecmascript
页面效果{{type}}自定义纸张宽X高(mm):x确定旋转纸张(宽高互换)清空纸张浏览器打印直接打印(需要连接客户端){{(scaleValue*100).toFixed(0)}}%默认样式自定义样式import{hiprint}from"vue-plugin-hiprint"import{provider1}from"./provider1"import{provider2}from"./pr
- Deepseek与doubao|tongyi|wenxin三个大模型对比编写数据处理脚本
AI技术老狗(QA)
Deepseek大模型AI编写脚本
DeepSeek在编写脚本方面的能力非常强大,尤其在编程、推理和数学计算方面展现出了超越普通AI的能力。DeepSeek的核心优势在于其编程能力的显著提高,能够轻松应对前端脚本和后端逻辑的编写,大大降低了程序员编写代码的难度。今天我们就对比下deepseek、豆包、通义千问、文心一言这四个进行一下对比,对比的题目为:《帮我写一个处理excel数据的python脚本,要求:100万条数据,去除重
- Windows环境下使用Ollama搭建本地AI大模型教程
冰山上的柯莱
人工智能windows人工智能ollamadeepseekcuda
注:Ollama仅支持Windows10及以上版本。安装Ollama去ollama官网下载对应平台及OS的安装包。运行安装包,点击“安装”按钮即可开始安装。Ollama会自动安装到你的C:\Users\\AppData\Local\Programs\Ollama目录上。安装完成后,Ollama会自动启动。启动时任务栏中图标显示如下:当Ollama未启动时,可以通过点击开始菜单Ollama快捷方式或
- Github 2024-06-20 开源项目日报 Top10
老孙正经胡说
github开源Github趋势分析开源项目PythonGolang
根据GithubTrendings的统计,今日(2024-06-20统计)共有10个项目上榜。根据开发语言中项目的数量,汇总情况如下:开发语言项目数量Python项目4TypeScript项目4Rust项目2JavaScript项目1Dart项目1Java项目1Go项目1RustDesk:用Rust编写的开源远程桌面软件创建周期:1218天开发语言:Rust,Dart协议类型:GNUAfferoG
- 打家劫舍----算法C语言
weixin_44799641
数据结构和算法算法c语言java
intbuff[20]={0,1,2,3,4,5,6,7,8,9,10,11,12,13,14,15,16,17,18,19};//计算两个数中的较大值/******************************************************************函数功能:取2个房子中的最大金额输入参数:Aamount:A房子的金额数Bamount:B房子的金额数输出参数:无
- Springboot使用Thumbnailator压缩图片上传到阿里云OSS(无损压缩)
一勺菠萝丶
Java#OSS#SpringBoot
前提:图片的压缩大致有两种,一种是将图片的尺寸压缩小,另一种是尺寸不变,将压缩质量,一般对于项目我们需要第一种,即用户上传一张分辨率为3840 × 2160的图片,通过上传图片接口后上传到OSS上的图片分辨率会变成1920×1080(如3840 × 2160的图片大小为11.4M,上传后的图片大概会为1.9M),此时上传后到OSS的图片和原图质量上一致,也就是说看上去只的大小的区别,清晰度上没有任
- android jetpack,Android Jetpack应用指南
comparethecloud
androidjetpack
第1章初识Jetpack11.1Android应用程序架构设计标准的缺失概论11.2什么是Jetpack21.3Jetpack与AndroidX31.4迁移至AndroidX41.5新建项目默认支持AndroidX61.6总结8第2章LifeCycle92.1LifeCycle的诞生92.2使用LifeCycle解耦页面与组件102.2.1案例分析102.2.2LifeCycle的原理112.2.
- 嵌入式玩具--无人机字幕
无数碎片寻妳
不花钱计划玩游戏
day0101-无人机-组成结构-上哎,好,各位,那现在呢我们一起来看一下,就是咱们接下来要做的这个小项目啊。呃,当然这个名字有很多啊,就是这种飞行器有管,它叫四旋翼飞行器的,也有叫四轴飞行器的啊OK啊,好了,这个应该都能理解,对吧?哎,好,我就不再多说了。呃,其实它的机械结构是非常非常简单的,对不对?一共就是一个飞机,然后四个螺旋桨,对吧?四个电机啊,只要这四个电机转,那它就能够是不是提供向上的
- 利用java编写一个简单计算器(实现加减乘除)
嘵奇
Java小程序java
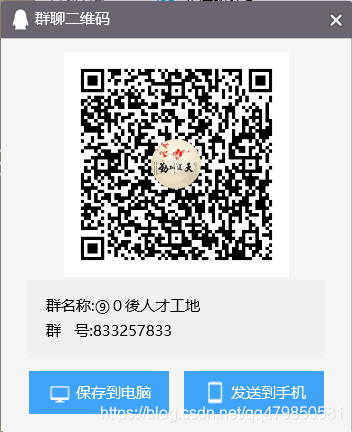与-all(完整版)两种发行包类型,支持通过官网、腾讯云镜像、阿里云镜像多通道下载。发行版类型说明特性-bin版本(二进制版)-all版本(完整版)包含内容核心运行时(二进制文件/基础库/脚本)全部-bin内容+文档/示例/源代码体积较小(~100MB)较大(~200
- 【Elasticsearch】分片与副本机制:优化数据存储与查询性能
程风破~
ElasticsearchElasticsearch实战elasticsearch大数据搜索引擎
博主简介:CSDN博客专家,历代文学网(PC端可以访问:https://literature.sinhy.com/#/?__c=1000,移动端可微信小程序搜索“历代文学”)总架构师,15年工作经验,精通Java编程,高并发设计,Springboot和微服务,熟悉Linux,ESXI虚拟化以及云原生Docker和K8s,热衷于探索科技的边界,并将理论知识转化为实际应用。保持对新技术的好奇心,乐于分
- 2025清华DeepSeek从入门到精通
京漂的人
ai
DeepSeek火爆全球,网上各类教程层出不穷,质量却参差不齐。近日,清华大学新闻与传播学院-新媒体研究中心-元宇宙文化实验室团队出了一版DeepSeek详细使用手册,长达104页。该教程堪称国产AI工具DeepSeek深度使用的标杆指南,既适合新手快速掌握基础操作,也为进阶用户提供系统性方法论https://download.csdn.net/download/gyc800/90360007
- usb闪存驱动器_如何为Windows 10、8或7创建USB闪存驱动器安装程序
culunxun2863
javapythonlinuxwindows数据库
usb闪存驱动器Ifyou’dliketoinstallWindowsbutdon’thaveaDVDdrive,it’seasyenoughtocreateabootableUSBflashdrivewiththerightinstallationmedia.Here’showtogetitdoneforWindows10,8,or7.如果您想安装Windows但没有DVD驱动器,那么使用正确的
- 第一章:Matlab 基础入门
正是读书时
《邂逅matlab
第一章:Matlab基础入门1.1基本数据类型MATLAB支持多种数据类型,包括数值型、字符型、逻辑型等。了解这些数据类型是使用MATLAB进行编程的基础。1.1.1数值型数据整数:表示整数值,例如1,-5,100等。浮点数:表示带有小数部分的数值,例如3.14,-0.001,2.71828等。代码示例:%定义整数a=10;b=-5;%定义浮点数c=3.14159;d=-0.001;%显示变量类型
- python字符串怎么转换成字典_用python将字符串转换成字典
weixin_39777018
Iknowthatthisquestionsoundaduplicate,butit'snot,atleastlookedforawhileandIcouldn'tfinenothingformyspecificproblem.Ihavethefollowingstring:"{first:{name:'test',value:100},second:{name:'test2',value:50}
- python-将字符串转换为字典
weixin_30505751
pythonjson
json越来越流行,通过python获取到json格式的字符串后,可以通过eval函数转换成dict格式:>>>a='{"name":"yct","age":10}'>>>eval(a){'age':10,'name':'yct'}转载于:https://www.cnblogs.com/gy-ph/p/8087372.html
- GMSL 明星产品之 MAX96724
iMr_Stone
硬件工程arm开发驱动开发嵌入式硬件
上一篇文章中,我们介绍了摄像头侧GMSL加串器MAX96717.今天我们来介绍下GMSL解串器明星产品MAX96724:可将四路GMSL™2/1输入转换为1路、2路或4路MIPID-PHY或C-PHY输出。该器件支持通过符合GMSL通道规范的50Ω同轴电缆或100Ω屏蔽双绞线(STP)电缆进行同时双向传输。每条GMSL2串行链路正向以3Gbps或6Gbps的固定速率运行,反向则以187.5Mbps
- EXCEL和DBC互转工具
劉小帅
自动化工具dbccanqt
excel转dbc使用方法见https://blog.csdn.net/m0_56315547/article/details/121164420?spm=1001.2014.3001.5501此工具只作为参考,作者不对生成的数据进行负责,生成完毕后,请自行校对,如果因生成的数据造成损失,与作者无关本工具主要针对与can和canfd。dbc转excel当dbc为J1939时请自行校对版本记录1.0
- 中电联协议对接互联互通实现充电桩小程序成熟搭建
玉阳软件yuyangdev_cn
小程序中电联互联互通充电
ICS35.240.60L73T/CEC中国电力企业联合会标准T/CEC102.3—2016电动汽车充换电服务信息交换部分:业务信息交换规范InteractiveofchargingandbatteryswapserviceinformationforelectricvehiclesPart3:Businessinformationexchangespecification2016-10-21发布
- PAT乙级真题 — 1087 有多少不同的值(java)
黄昏岭
算法数据结构
当自然数n依次取1、2、3、……、N时,算式⌊n/2⌋+⌊n/3⌋+⌊n/5⌋有多少个不同的值?(注:⌊x⌋为取整函数,表示不超过x的最大自然数,即x的整数部分。)输入格式:输入给出一个正整数N(2≤N≤104)。输出格式:在一行中输出题面中算式取到的不同值的个数。输入样例:2017输出样例:1480思路:先找最大值——10000/2+10000/3+10000/5=10335,然后定义10335
- Github 2025-02-13Go开源项目日报 Top10
老孙正经胡说
github开源Github趋势分析开源项目PythonGolang
根据GithubTrendings的统计,今日(2025-02-13统计)共有10个项目上榜。根据开发语言中项目的数量,汇总情况如下:开发语言项目数量Go项目10TypeScript项目1InnoSetup项目1Kubernetes:容器化应用程序管理系统创建周期:3618天开发语言:Go协议类型:ApacheLicense2.0Star数量:106913个Fork数量:38445次关注人数:10
- Bash 中的运算方式
躺不平的理查德
#bash开发语言
目录概述:1.(())运算符2.let命令3.expr命令4.$[]直接运算5.bc(计算器,支持浮点数)6.awk(强大的文本处理工具,也可计算)概述:Bash本身只支持整数运算,但可以结合bc和awk进行浮点运算。以下是常见的计算方法:1.(())运算符(())是Bash的整数计算语法,支持算术运算符、逻辑运算符,并且可以直接操作变量。echo$((2+3))#输出5echo$((10/3))
- JD短视频带货项目详解 | 普通素人月入过万的新机会
沐凡资源
全文检索
一、项目本质JD短视频带货是京东近几年重点扶持的「内容电商」项目,创作者通过发布商品种草短视频,用户点击视频中的商品链接下单后,即可获得佣金分成。核心逻辑:用短视频内容激活京东站内流量,对标抖音的「兴趣电商」,但竞争小得多。二、为什么现在入场是红利期?平台疯狂撒钱:JD2023年拿出10亿现金补贴,新人发5条视频就送50元流量缺口大:JD用户习惯搜索购物,短视频内容占比不足5%,官方急需创作者补位
- 小程序二:利用Python编写一个简单的计算器(实现加减乘除)
嘵奇
Python小程序python
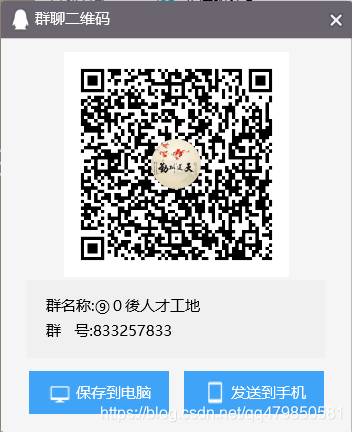共有10个项目上榜。根据开发语言中项目的数量,汇总情况如下:开发语言项目数量Rust项目10TypeScript项目1Zed:由Atom和Tree-sitter的创建者开发的高性能多人代码编辑器创建周期:1071天开发语言:Rust协议类型:OtherStar数量:9436个Fork数量:261次关注人数:9436人贡献人数
- Pix2PixHD代码小白解读(3)——Pix2PixHD_model.py
咖啡百怪
Pix2PixHD代码解读深度学习机器学习人工智能python
上两期:Pix2PixHD代码小白注释(1)——train.pyhttps://blog.csdn.net/qq_73991479/article/details/134757142?spm=1001.2014.3001.5501Pix2PixHD代码小白注释(2)——BaseModel.pyhttps://blog.csdn.net/qq_73991479/article/details/134
- java封装继承多态等
麦田的设计者
javaeclipsejvmcencapsulatopn
最近一段时间看了很多的视频却忘记总结了,现在只能想到什么写什么了,希望能起到一个回忆巩固的作用。
1、final关键字
译为:最终的
&
- F5与集群的区别
bijian1013
weblogic集群F5
http请求配置不是通过集群,而是F5;集群是weblogic容器的,如果是ejb接口是通过集群。
F5同集群的差别,主要还是会话复制的问题,F5一把是分发http请求用的,因为http都是无状态的服务,无需关注会话问题,类似
- LeetCode[Math] - #7 Reverse Integer
Cwind
java题解MathLeetCodeAlgorithm
原题链接:#7 Reverse Integer
要求:
按位反转输入的数字
例1: 输入 x = 123, 返回 321
例2: 输入 x = -123, 返回 -321
难度:简单
分析:
对于一般情况,首先保存输入数字的符号,然后每次取输入的末位(x%10)作为输出的高位(result = result*10 + x%10)即可。但
- BufferedOutputStream
周凡杨
首先说一下这个大批量,是指有上千万的数据量。
例子:
有一张短信历史表,其数据有上千万条数据,要进行数据备份到文本文件,就是执行如下SQL然后将结果集写入到文件中!
select t.msisd
- linux下模拟按键输入和鼠标
被触发
linux
查看/dev/input/eventX是什么类型的事件, cat /proc/bus/input/devices
设备有着自己特殊的按键键码,我需要将一些标准的按键,比如0-9,X-Z等模拟成标准按键,比如KEY_0,KEY-Z等,所以需要用到按键 模拟,具体方法就是操作/dev/input/event1文件,向它写入个input_event结构体就可以模拟按键的输入了。
linux/in
- ContentProvider初体验
肆无忌惮_
ContentProvider
ContentProvider在安卓开发中非常重要。与Activity,Service,BroadcastReceiver并称安卓组件四大天王。
在android中的作用是用来对外共享数据。因为安卓程序的数据库文件存放在data/data/packagename里面,这里面的文件默认都是私有的,别的程序无法访问。
如果QQ游戏想访问手机QQ的帐号信息一键登录,那么就需要使用内容提供者COnte
- 关于Spring MVC项目(maven)中通过fileupload上传文件
843977358
mybatisspring mvc修改头像上传文件upload
Spring MVC 中通过fileupload上传文件,其中项目使用maven管理。
1.上传文件首先需要的是导入相关支持jar包:commons-fileupload.jar,commons-io.jar
因为我是用的maven管理项目,所以要在pom文件中配置(每个人的jar包位置根据实际情况定)
<!-- 文件上传 start by zhangyd-c --&g
- 使用svnkit api,纯java操作svn,实现svn提交,更新等操作
aigo
svnkit
原文:http://blog.csdn.net/hardwin/article/details/7963318
import java.io.File;
import org.apache.log4j.Logger;
import org.tmatesoft.svn.core.SVNCommitInfo;
import org.tmateso
- 对比浏览器,casperjs,httpclient的Header信息
alleni123
爬虫crawlerheader
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse res) throws ServletException, IOException
{
String type=req.getParameter("type");
Enumeration es=re
- java.io操作 DataInputStream和DataOutputStream基本数据流
百合不是茶
java流
1,java中如果不保存整个对象,只保存类中的属性,那么我们可以使用本篇文章中的方法,如果要保存整个对象 先将类实例化 后面的文章将详细写到
2,DataInputStream 是java.io包中一个数据输入流允许应用程序以与机器无关方式从底层输入流中读取基本 Java 数据类型。应用程序可以使用数据输出流写入稍后由数据输入流读取的数据。
- 车辆保险理赔案例
bijian1013
车险
理赔案例:
一货运车,运输公司为车辆购买了机动车商业险和交强险,也买了安全生产责任险,运输一车烟花爆竹,在行驶途中发生爆炸,出现车毁、货损、司机亡、炸死一路人、炸毁一间民宅等惨剧,针对这几种情况,该如何赔付。
赔付建议和方案:
客户所买交强险在这里不起作用,因为交强险的赔付前提是:“机动车发生道路交通意外事故”;
如果是交通意外事故引发的爆炸,则优先适用交强险条款进行赔付,不足的部分由商业
- 学习Spring必学的Java基础知识(5)—注解
bijian1013
javaspring
文章来源:http://www.iteye.com/topic/1123823,整理在我的博客有两个目的:一个是原文确实很不错,通俗易懂,督促自已将博主的这一系列关于Spring文章都学完;另一个原因是为免原文被博主删除,在此记录,方便以后查找阅读。
有必要对
- 【Struts2一】Struts2 Hello World
bit1129
Hello world
Struts2 Hello World应用的基本步骤
创建Struts2的Hello World应用,包括如下几步:
1.配置web.xml
2.创建Action
3.创建struts.xml,配置Action
4.启动web server,通过浏览器访问
配置web.xml
<?xml version="1.0" encoding="
- 【Avro二】Avro RPC框架
bit1129
rpc
1. Avro RPC简介 1.1. RPC
RPC逻辑上分为二层,一是传输层,负责网络通信;二是协议层,将数据按照一定协议格式打包和解包
从序列化方式来看,Apache Thrift 和Google的Protocol Buffers和Avro应该是属于同一个级别的框架,都能跨语言,性能优秀,数据精简,但是Avro的动态模式(不用生成代码,而且性能很好)这个特点让人非常喜欢,比较适合R
- lua set get cookie
ronin47
lua cookie
lua:
local access_token = ngx.var.cookie_SGAccessToken
if access_token then
ngx.header["Set-Cookie"] = "SGAccessToken="..access_token.."; path=/;Max-Age=3000"
end
- java-打印不大于N的质数
bylijinnan
java
public class PrimeNumber {
/**
* 寻找不大于N的质数
*/
public static void main(String[] args) {
int n=100;
PrimeNumber pn=new PrimeNumber();
pn.printPrimeNumber(n);
System.out.print
- Spring源码学习-PropertyPlaceholderHelper
bylijinnan
javaspring
今天在看Spring 3.0.0.RELEASE的源码,发现PropertyPlaceholderHelper的一个bug
当时觉得奇怪,上网一搜,果然是个bug,不过早就有人发现了,且已经修复:
详见:
http://forum.spring.io/forum/spring-projects/container/88107-propertyplaceholderhelper-bug
- [逻辑与拓扑]布尔逻辑与拓扑结构的结合会产生什么?
comsci
拓扑
如果我们已经在一个工作流的节点中嵌入了可以进行逻辑推理的代码,那么成百上千个这样的节点如果组成一个拓扑网络,而这个网络是可以自动遍历的,非线性的拓扑计算模型和节点内部的布尔逻辑处理的结合,会产生什么样的结果呢?
是否可以形成一种新的模糊语言识别和处理模型呢? 大家有兴趣可以试试,用软件搞这些有个好处,就是花钱比较少,就算不成
- ITEYE 都换百度推广了
cuisuqiang
GoogleAdSense百度推广广告外快
以前ITEYE的广告都是谷歌的Google AdSense,现在都换成百度推广了。
为什么个人博客设置里面还是Google AdSense呢?
都知道Google AdSense不好申请,这在ITEYE上也不是讨论了一两天了,强烈建议ITEYE换掉Google AdSense。至少,用一个好申请的吧。
什么时候能从ITEYE上来点外快,哪怕少点
- 新浪微博技术架构分析
dalan_123
新浪微博架构
新浪微博在短短一年时间内从零发展到五千万用户,我们的基层架构也发展了几个版本。第一版就是是非常快的,我们可以非常快的实现我们的模块。我们看一下技术特点,微博这个产品从架构上来分析,它需要解决的是发表和订阅的问题。我们第一版采用的是推的消息模式,假如说我们一个明星用户他有10万个粉丝,那就是说用户发表一条微博的时候,我们把这个微博消息攒成10万份,这样就是很简单了,第一版的架构实际上就是这两行字。第
- 玩转ARP攻击
dcj3sjt126com
r
我写这片文章只是想让你明白深刻理解某一协议的好处。高手免看。如果有人利用这片文章所做的一切事情,盖不负责。 网上关于ARP的资料已经很多了,就不用我都说了。 用某一位高手的话来说,“我们能做的事情很多,唯一受限制的是我们的创造力和想象力”。 ARP也是如此。 以下讨论的机子有 一个要攻击的机子:10.5.4.178 硬件地址:52:54:4C:98
- PHP编码规范
dcj3sjt126com
编码规范
一、文件格式
1. 对于只含有 php 代码的文件,我们将在文件结尾处忽略掉 "?>" 。这是为了防止多余的空格或者其它字符影响到代码。例如:<?php$foo = 'foo';2. 缩进应该能够反映出代码的逻辑结果,尽量使用四个空格,禁止使用制表符TAB,因为这样能够保证有跨客户端编程器软件的灵活性。例
- linux 脱机管理(nohup)
eksliang
linux nohupnohup
脱机管理 nohup
转载请出自出处:http://eksliang.iteye.com/blog/2166699
nohup可以让你在脱机或者注销系统后,还能够让工作继续进行。他的语法如下
nohup [命令与参数] --在终端机前台工作
nohup [命令与参数] & --在终端机后台工作
但是这个命令需要注意的是,nohup并不支持bash的内置命令,所
- BusinessObjects Enterprise Java SDK
greemranqq
javaBOSAPCrystal Reports
最近项目用到oracle_ADF 从SAP/BO 上调用 水晶报表,资料比较少,我做一个简单的分享,给和我一样的新手 提供更多的便利。
首先,我是尝试用JAVA JSP 去访问的。
官方API:http://devlibrary.businessobjects.com/BusinessObjectsxi/en/en/BOE_SDK/boesdk_ja
- 系统负载剧变下的管控策略
iamzhongyong
高并发
假如目前的系统有100台机器,能够支撑每天1亿的点击量(这个就简单比喻一下),然后系统流量剧变了要,我如何应对,系统有那些策略可以处理,这里总结了一下之前的一些做法。
1、水平扩展
这个最容易理解,加机器,这样的话对于系统刚刚开始的伸缩性设计要求比较高,能够非常灵活的添加机器,来应对流量的变化。
2、系统分组
假如系统服务的业务不同,有优先级高的,有优先级低的,那就让不同的业务调用提前分组
- BitTorrent DHT 协议中文翻译
justjavac
bit
前言
做了一个磁力链接和BT种子的搜索引擎 {Magnet & Torrent},因此把 DHT 协议重新看了一遍。
BEP: 5Title: DHT ProtocolVersion: 3dec52cb3ae103ce22358e3894b31cad47a6f22bLast-Modified: Tue Apr 2 16:51:45 2013 -070
- Ubuntu下Java环境的搭建
macroli
java工作ubuntu
配置命令:
$sudo apt-get install ubuntu-restricted-extras
再运行如下命令:
$sudo apt-get install sun-java6-jdk
待安装完毕后选择默认Java.
$sudo update- alternatives --config java
安装过程提示选择,输入“2”即可,然后按回车键确定。
- js字符串转日期(兼容IE所有版本)
qiaolevip
TODateStringIE
/**
* 字符串转时间(yyyy-MM-dd HH:mm:ss)
* result (分钟)
*/
stringToDate : function(fDate){
var fullDate = fDate.split(" ")[0].split("-");
var fullTime = fDate.split("
- 【数据挖掘学习】关联规则算法Apriori的学习与SQL简单实现购物篮分析
superlxw1234
sql数据挖掘关联规则
关联规则挖掘用于寻找给定数据集中项之间的有趣的关联或相关关系。
关联规则揭示了数据项间的未知的依赖关系,根据所挖掘的关联关系,可以从一个数据对象的信息来推断另一个数据对象的信息。
例如购物篮分析。牛奶 ⇒ 面包 [支持度:3%,置信度:40%] 支持度3%:意味3%顾客同时购买牛奶和面包。 置信度40%:意味购买牛奶的顾客40%也购买面包。 规则的支持度和置信度是两个规则兴
- Spring 5.0 的系统需求,期待你的反馈
wiselyman
spring
Spring 5.0将在2016年发布。Spring5.0将支持JDK 9。
Spring 5.0的特性计划还在工作中,请保持关注,所以作者希望从使用者得到关于Spring 5.0系统需求方面的反馈。