开心消消乐
文章目录
- 开心消消乐
-
- 消消乐游戏需求
- 游戏展示
-
- 代码实现
-
- 熊类
- 鸟类
- 狐狸类
- 青蛙类
- 元素类
- 图片类
- 游戏窗口
消消乐游戏需求
- 所参与的角色:熊、鸟、狐狸、青蛙
- 功能:
- 由系统随机长成元素并显示在窗口中(8行6列),保证不能有可消元素
- 选中两个元素,若相邻则交换,而后判断:
- 若不可消(不连3),则换回去
- 若可消(连3及以上),则:
- 爆破后删除元素
- 重新生成新的元素,若还是可消则重复2.2步骤,直到没有可消元素为止
游戏展示
初始界面
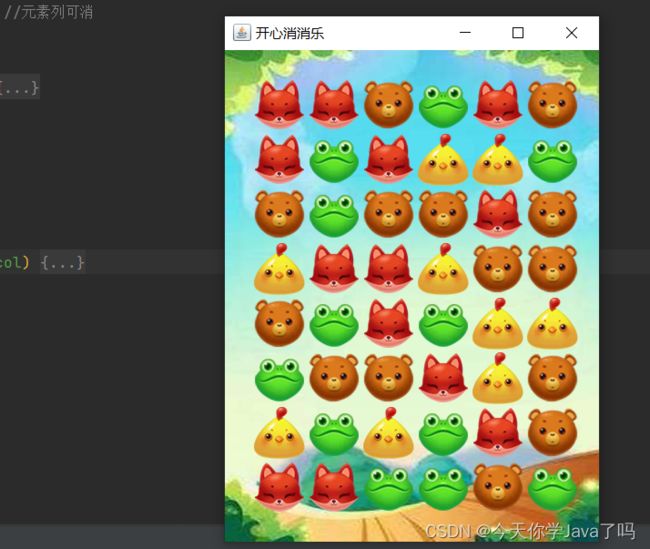
爆炸展示
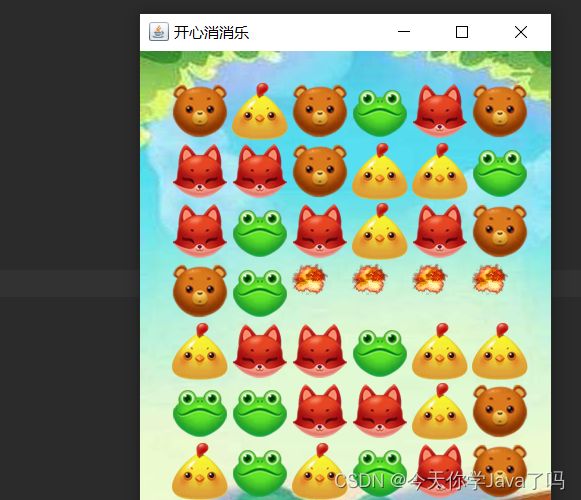
代码实现
熊类
public class Bear extends Element{
public Bear(int x, int y) {
super(x, y);
}
@Override
public ImageIcon getImage() {
return Images.bear;
}
}
鸟类
public class Bird extends Element{
public Bird(int x, int y) {
super(x, y);
}
@Override
public ImageIcon getImage() {
return Images.bird;
}
}
狐狸类
public class Fox extends Element {
public Fox(int x, int y) {
super(x, y);
}
@Override
public ImageIcon getImage() {
return Images.fox;
}
}
青蛙类
public class Frog extends Element {
public Frog(int x, int y) {
super(x, y);
}
@Override
public ImageIcon getImage() {
return Images.frog;
}
}
元素类
public abstract class Element {
private int x, y;
private boolean selected;
private boolean eliminated;
private int eliminatedIndex;
public Element(int x, int y) {
this.x = x;
this.y = y;
this.selected = false;
this.eliminated = false;
this.eliminatedIndex = 0;
}
public abstract ImageIcon getImage();
public void paintElement(Graphics g) {
if (isSelected()) {
g.setColor(Color.GREEN);
g.fillRect(x, y, World.ELEMENT_SIZE, World.ELEMENT_SIZE);
this.getImage().paintIcon(null, g, this.x, this.y);
} else if (isEliminated()) {
if (eliminatedIndex < Images.bombs.length) {
Images.bombs[eliminatedIndex++].paintIcon(null, g, x, y);
}
} else {
this.getImage().paintIcon(null, g, this.x, this.y);
}
}
boolean isSelected() {
return selected;
}
public void setSelected(boolean selected) {
this.selected = selected;
}
boolean isEliminated() {
return eliminated;
}
public void setEliminated(boolean eliminated) {
this.eliminated = eliminated;
}
public int getX() {
return x;
}
public int getY() {
return y;
}
public void setX(int x) {
this.x = x;
}
public void setY(int y) {
this.y = y;
}
}
图片类
public class Images {
public static ImageIcon background;
public static ImageIcon bird;
public static ImageIcon bear;
public static ImageIcon fox;
public static ImageIcon frog;
public static ImageIcon[] bombs;
static {
background = new ImageIcon("HappyEliminate/src/com/liner/img/background.png");
bird = new ImageIcon("HappyEliminate/src/com/liner/img/bird.png");
bear = new ImageIcon("HappyEliminate/src/com/liner/img/bear.png");
fox = new ImageIcon("HappyEliminate/src/com/liner/img/fox.png");
frog = new ImageIcon("HappyEliminate/src/com/liner/img/frog.png");
bombs = new ImageIcon[4];
for (int i = 0; i < bombs.length; i++) {
bombs[i] = new ImageIcon("HappyEliminate/src/com/liner/img/bom" + (i + 1) + ".png");
}
}
public static void main(String[] args) {
System.out.println(background.getImageLoadStatus());
System.out.println(bird.getImageLoadStatus());
System.out.println(frog.getImageLoadStatus());
System.out.println(fox.getImageLoadStatus());
System.out.println(bear.getImageLoadStatus());
for (int i = 0; i < bombs.length ; i++) {
System.out.println(bombs[i].getImageLoadStatus());
}
}
}
游戏窗口
public class World extends JPanel {
public static final int WIDTH = 429;
public static final int HEIGHT = 570;
public static final int ROWS = 8;
public static final int COLS = 6;
public static final int ELEMENT_SIZE = 60;
public static final int OFFSET = 30;
private Element[][] elements = new Element[ROWS][COLS];
private boolean canInteractive = true;
private int selectedNumber = 0;
private int firstRow = 0;
private int firstCol = 0;
private int secondRow = 0;
private int secondCol = 0;
public static void main(String[] args) {
JFrame jFrame = new JFrame();
World world = new World();
world.setFocusable(true);
jFrame.add(world);
jFrame.setTitle("开心消消乐");
jFrame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
jFrame.setSize(WIDTH, HEIGHT + 17);
jFrame.setVisible(true);
world.start();
}
private void start() {
fillAllElement();
MouseAdapter mouseAdapter = new MouseAdapter() {
@Override
public void mouseClicked(MouseEvent e) {
if (!canInteractive) {
return;
}
canInteractive = false;
int row = (e.getY() - OFFSET) / ELEMENT_SIZE;
int col = (e.getX() - OFFSET) / ELEMENT_SIZE;
selectedNumber++;
if (selectedNumber == 1) {
firstRow = row;
firstCol = col;
elements[firstRow][firstCol].setSelected(true);
canInteractive = true;
} else if (selectedNumber == 2) {
secondRow = row;
secondCol = col;
elements[secondRow][secondCol].setSelected(true);
if (isAdjacent()) {
new Thread(() -> {
elements[firstRow][firstCol].setSelected(false);
elements[secondRow][secondCol].setSelected(false);
moveElement();
exchangeElement();
if (eliminateElement()) {
do {
dropElement();
try {
Thread.sleep(10);
} catch (InterruptedException ex) {
ex.printStackTrace();
}
}while (eliminateElement());
} else {
moveElement();
exchangeElement();
}
canInteractive = true;
}).start();
} else {
elements[firstRow][firstCol].setSelected(false);
elements[secondRow][secondCol].setSelected(false);
canInteractive = true;
}
canInteractive = true;
selectedNumber = 0;
}
repaint();
}
};
this.addMouseListener(mouseAdapter);
}
private void dropElement() {
for (int row = ROWS - 1; row >= 0; row--) {
while (true) {
int[] nullCols = {};
for (int col = COLS - 1; col >= 0; col--) {
Element element = elements[row][col];
if (element == null) {
nullCols = Arrays.copyOf(nullCols, nullCols.length + 1);
nullCols[nullCols.length - 1] = col;
}
}
if (nullCols.length > 0) {
for (int count = 0; count < 15; count++) {
for (int i = 0; i < nullCols.length; i++) {
int nullCol = nullCols[i];
for (int dr = row - 1; dr >= 0; dr--) {
Element element = elements[dr][nullCol];
if (element != null) {
element.setY(element.getY() + 4);
}
}
}
try {
Thread.sleep(10);
} catch (InterruptedException e) {
e.printStackTrace();
}
repaint();
}
for (int i = 0; i < nullCols.length; i++) {
int nullCol = nullCols[i];
for (int nr = row; nr > 0; nr--) {
elements[nr][nullCol] = elements[nr - 1][nullCol];
}
elements[0][nullCol] = createElement(0,nullCol);
}
} else {
break;
}
}
}
}
private boolean eliminateElement() {
boolean haveEliminated = false;
for (int row = ROWS - 1; row >= 0; row--) {
for (int col = COLS - 1; col >= 0; col--) {
Element element = elements[row][col];
if (element == null) {
continue;
}
int colRepeat = 0;
for (int pc = col - 1; pc >= 0; pc--) {
if (elements[row][pc] == null) {
break;
}
if (elements[row][pc].getClass() == element.getClass()) {
colRepeat++;
} else {
break;
}
}
int rowRepeat = 0;
for (int pr = row - 1; pr >= 0; pr--) {
if (elements[pr][col] == null) {
break;
}
if (elements[pr][col].getClass() == element.getClass()) {
rowRepeat++;
} else {
break;
}
}
if (colRepeat >= 2) {
elements[row][col].setEliminated(true);
for (int i = 1; i <= colRepeat; i++) {
elements[row][col - i].setEliminated(true);
}
}
if (rowRepeat >= 2) {
elements[row][col].setEliminated(true);
for (int i = 1; i <= rowRepeat; i++) {
elements[row - i][col].setEliminated(true);
}
}
if (colRepeat >= 2 || rowRepeat >= 2) {
for (int i = 0; i < Images.bombs.length; i++) {
repaint();
try {
Thread.sleep(50);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
if (colRepeat >= 2) {
elements[row][col] = null;
for (int i = 1; i <= colRepeat; i++) {
elements[row][col - i] = null;
}
haveEliminated = true;
}
if (rowRepeat >= 2) {
elements[row][col] = null;
for (int i = 1; i <= rowRepeat; i++) {
elements[row - i][col] = null;
}
haveEliminated = true;
}
}
}
return haveEliminated;
}
private void exchangeElement() {
Element element1 = elements[firstRow][firstCol];
Element element2 = elements[secondRow][secondCol];
elements[firstRow][firstCol] = element2;
elements[secondRow][secondCol] = element1;
}
private void moveElement() {
if (firstRow == secondRow) {
int firstX = OFFSET + firstCol * ELEMENT_SIZE;
int secondX = OFFSET + secondCol * ELEMENT_SIZE;
int step = firstX < secondX ? 4 : -4;
for (int i = 0; i < 15; i++) {
try {
Thread.sleep(10);
} catch (InterruptedException e) {
e.printStackTrace();
}
firstX += step;
secondX -= step;
elements[firstRow][firstCol].setX(firstX);
elements[secondRow][secondCol].setX(secondX);
repaint();
}
}
if (firstCol == secondCol) {
int firstY = OFFSET + firstRow * ELEMENT_SIZE;
int secondY = OFFSET + secondRow * ELEMENT_SIZE;
int step = firstY < secondY ? 4 : -4;
for (int i = 0; i < 15; i++) {
try {
Thread.sleep(10);
} catch (InterruptedException e) {
e.printStackTrace();
}
firstY += step;
secondY -= step;
elements[firstRow][firstCol].setY(firstY);
elements[secondRow][secondCol].setY(secondY);
repaint();
}
}
}
public static final int ELEMENT_TYPE_BEAR = 0;
public static final int ELEMENT_TYPE_BIRD = 1;
public static final int ELEMENT_TYPE_FOX = 2;
public static final int ELEMENT_TYPE_FROG = 3;
public static final int ELIMINATE_NONE = 0;
public static final int ELIMINATE_ROW = 1;
public static final int ELIMINATE_COL = 2;
public int canEliminate(int row, int col) {
Element element = elements[row][col];
if (row >= 2) {
Element element1 = elements[row - 1][col];
Element element2 = elements[row - 2][col];
if (element1 != null && element2 != null && element != null) {
if (element.getClass().equals(element1.getClass()) && element.getClass().equals(element2.getClass())) {
return ELIMINATE_COL;
}
}
}
if (col >= 2) {
Element element1 = elements[row][col - 1];
Element element2 = elements[row][col - 2];
if (element1 != null && element2 != null && element != null) {
if (element.getClass().equals(element1.getClass()) && element.getClass().equals(element2.getClass())) {
return ELIMINATE_ROW;
}
}
}
return ELIMINATE_NONE;
}
public boolean isAdjacent() {
if ((Math.abs(firstRow - secondRow) == 1 && firstCol == secondCol) || (Math.abs(firstCol - secondCol) == 1 && firstRow == secondRow)) {
return true;
} else {
return false;
}
}
public Element createElement(int row, int col) {
int x = OFFSET + col * ELEMENT_SIZE;
int y = OFFSET + row * ELEMENT_SIZE;
Random random = new Random();
int type = random.nextInt(4);
switch (type) {
case ELEMENT_TYPE_BEAR:
return new Bear(x, y);
case ELEMENT_TYPE_BIRD:
return new Bird(x, y);
case ELEMENT_TYPE_FOX:
return new Fox(x, y);
default:
return new Frog(x, y);
}
}
public void fillAllElement() {
for (int row = 0; row < ROWS; row++) {
for (int col = 0; col < COLS; col++) {
do {
Element element = createElement(row, col);
elements[row][col] = element;
} while (canEliminate(row, col) != ELIMINATE_NONE);
}
}
}
@Override
public void paint(Graphics g) {
Images.background.paintIcon(null, g, 0, 0);
for (int row = 0; row < ROWS; row++) {
for (int col = 0; col < COLS; col++) {
Element element = elements[row][col];
if (element != null) {
element.paintElement(g);
}
}
}
}
}