DFS
1.排列数字
dfs和递归差不多,具体图示如下图:
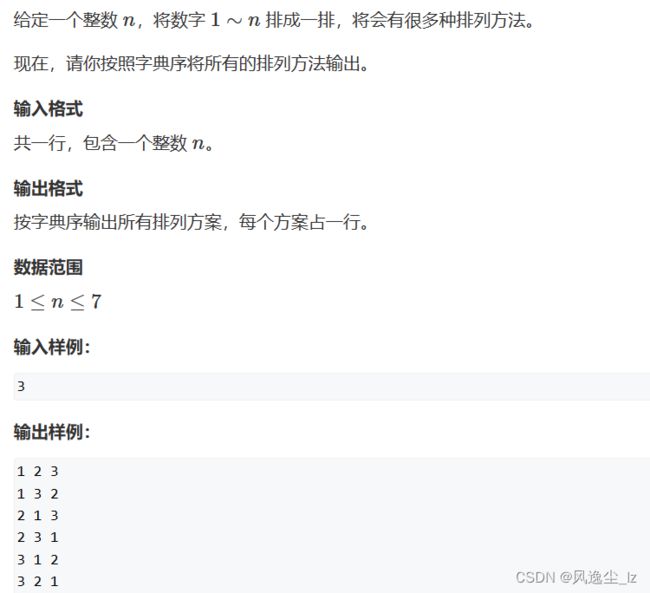
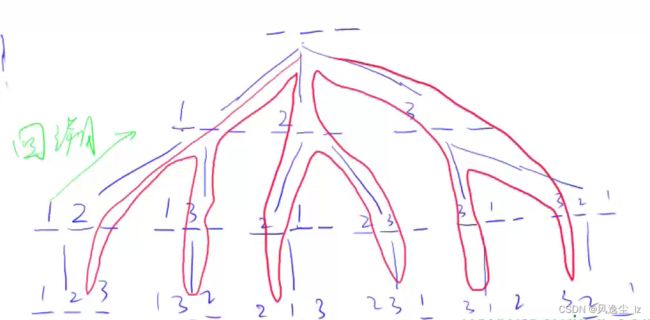
N=10
path=[0]*N
state=[False]*N
def dfs(u):
if u==n:
for i in range(n):
print(path[i],end=' ')
print()
for i in range(n):
if state[i]==False:
path[u]=i+1
state[i]=True
dfs(u+1)
path[u]=0
state[i]=False
n=int(input())
dfs(0)
2.n皇后问题
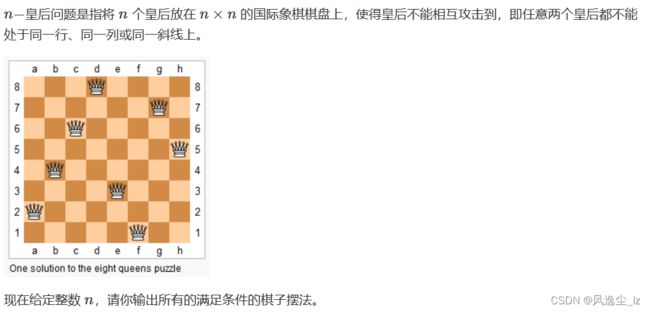
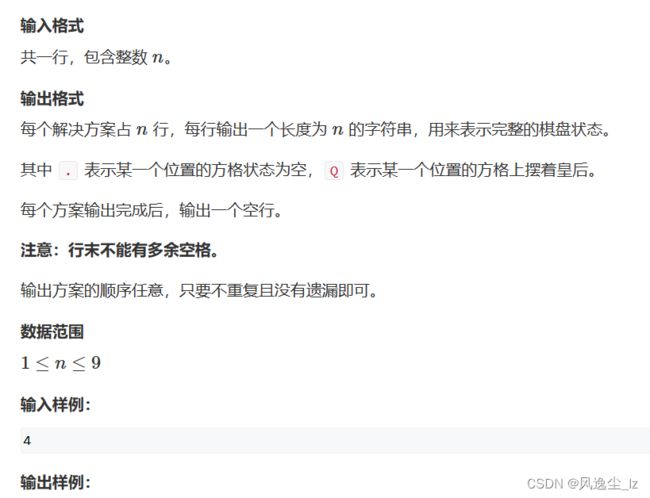
.Q..
...Q
Q...
..Q.
..Q.
Q...
...Q
.Q..
def dfs(x,y,queue):
if y==n:
x,y=x+1,0
if x==n:
if queue==n:
for i in range(n):
for j in range(n):
print(chessboard[i][j],end='')
print()
print()
return
if not row[x] and not col[y] and not dg[x+y] and not udg[y-x+n]:
chessboard[x][y]='Q'
row[x],col[y],dg[x+y],udg[y-x+n]=1,1,1,1
dfs(x,y+1,queue+1)
row[x],col[y],dg[x+y],udg[y-x+n],chessboard[x][y]=0,0,0,0,'.'
dfs(x,y+1,queue)
if __name__=='__main__':
N=10
n=int(input())
chessboard=[['.' for _ in range(N)] for _ in range(N)]
row,col,dg,udg=[0]*N,[0]*N,[0]*2*N,[0]*2*N
dfs(0,0,0)
BFS
走迷宫
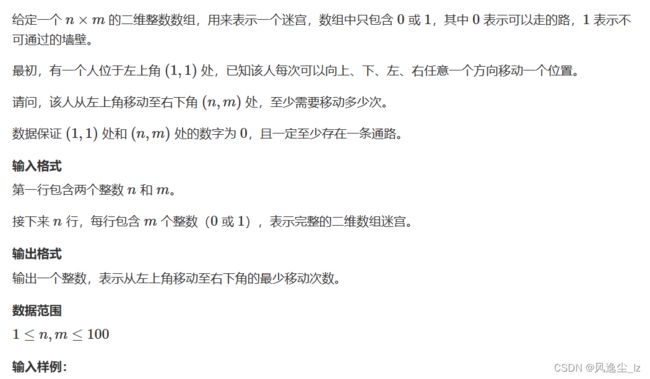
输入样例:
5 5
0 1 0 0 0
0 1 0 1 0
0 0 0 0 0
0 1 1 1 0
0 0 0 1 0
输出样例:
8
def bfs():
dx=[-1,0,1,0]
dy=[0,1,0,-1]
queue=[(0,0)]
trace[0][0]=0
while queue:
x,y=queue.pop(0)
if (x,y)==(n-1,m-1):
return trace[x][y]
for i in range(4):
a=x+dx[i]
b=y+dy[i]
if 0<=a<n and 0<=b<m and graph[a][b]!=1 and trace[a][b]==-1:
queue.append((a,b))
trace[a][b]=trace[x][y]+1
N=101
n,m=map(int,input().split())
graph=[[0]*N for _ in range(N)]
trace=[[-1]*N for _ in range(N)]
for i in range(n):
graph[i][0:m]=list(map(int,input().split()))
print(bfs())
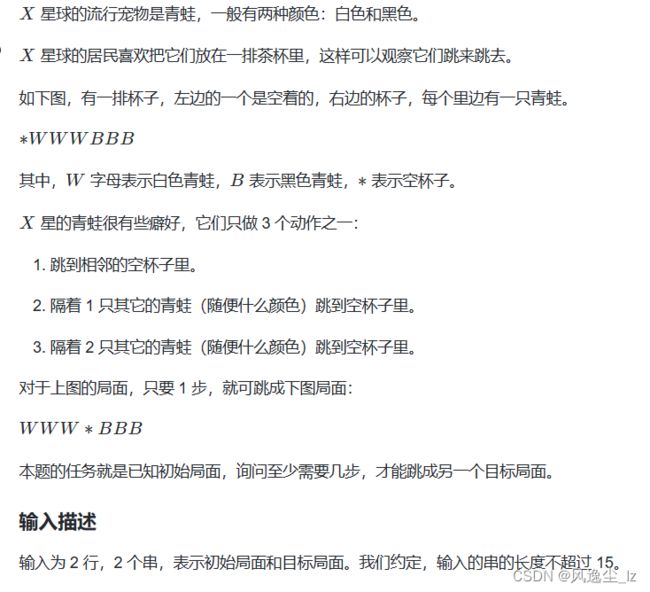
青蛙跳杯子
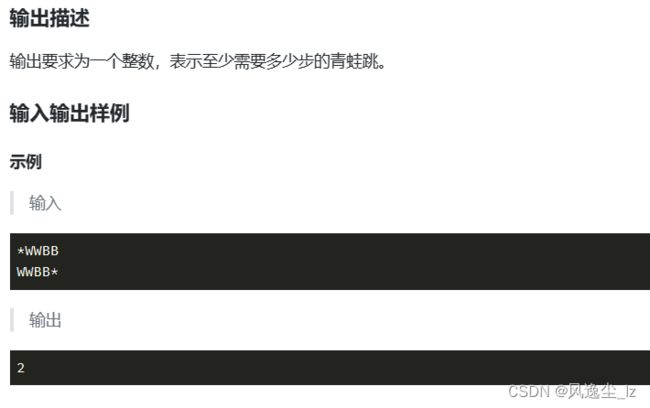
x=input()
y=input()
move=[1,-1,2,-2,3,-3]
aset={x}
def bfs():
Q=[(x,0)]
while Q:
old=Q.pop(0)
for i in move:
a=list(old[0])
b=old[1]
c=a.index('*')
d=c+i
if 0<=d<len(x):
a[c]=a[d]
a[d]='*'
e=''.join(a)
b+=1
if e==y:
print(b)
return
if e not in aset:
aset.add(e)
Q.append((e,b))
bfs()