leetcode python刷题记录(十二)(111~120)
111. 二叉树的最小深度

class Solution:
def minDepth(self, root: Optional[TreeNode]) -> int:
if not root:
return 0
depth=[]
def dfs(node,d):
if not node.left and not node.right:
depth.append(d)
return
d=d+1
if node.left:
dfs(node.left,d)
if node.right:
dfs(node.right,d)
dfs(root,1)
return min(depth)
112. 路径总和

class Solution:
def hasPathSum(self, root: Optional[TreeNode], targetSum: int) -> bool:
if not root:
return False
path=[]
def dfs(root,sum):
if not root:
return
sum=sum+root.val
if not root.left and not root.right:
path.append(sum)
dfs(root.left,sum)
dfs(root.right,sum)
dfs(root,0)
if targetSum in path:
return True
else:
return False
113. 路径总和 II
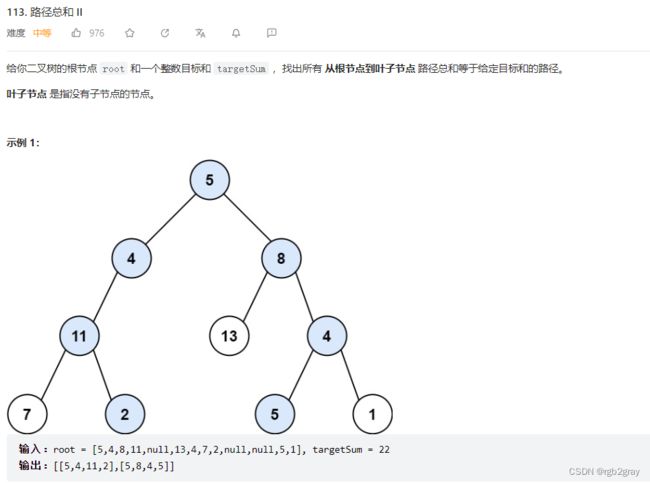
dfs:
class Solution:
def pathSum(self, root: Optional[TreeNode], targetSum: int) -> List[List[int]]:
path=[]
res=[]
def dfs(root,sum,path):
if not root:
return
if not root.left and not root.right:
if sum == root.val:
path=path+[root.val]
res.append(path)
dfs(root.left,sum-root.val,path+[root.val])
dfs(root.right,sum-root.val,path+[root.val])
dfs(root,targetSum,path)
return res
114. 二叉树展开为链表

class Solution:
def flatten(self, root: Optional[TreeNode]) -> None:
"""
Do not return anything, modify root in-place instead.
"""
preorderList = list()
def preorderTraversal(root: TreeNode):
if root:
preorderList.append(root)
preorderTraversal(root.left)
preorderTraversal(root.right)
preorderTraversal(root)
for i in range(1, len(preorderList)):
prev, curr = preorderList[i - 1], preorderList[i]
prev.left = None
prev.right = curr
115. 不同的子序列
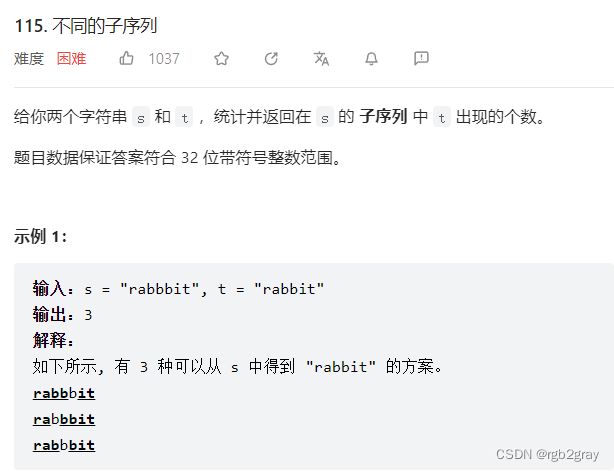
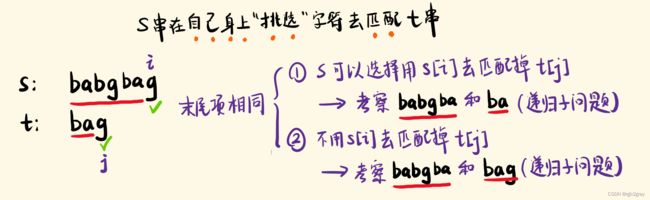

class Solution:
def numDistinct(self, s: str, t: str) -> int:
m,n=len(s),len(t)
dp=[[0]*(n+1) for _ in range(m+1)]
for i in range(m+1):
dp[i][0]=1
for i in range(1,m+1):
for j in range(1,n+1):
dp[i][j]=dp[i-1][j]
if s[i-1]==t[j-1]:
dp[i][j]=dp[i][j]+dp[i-1][j-1]
return dp[m][n]
116. 填充每个节点的下一个右侧节点指针


class Solution:
def connect(self, root: 'Optional[Node]') -> 'Optional[Node]':
if root:
if root.left:
root.left.next=root.right
if root.next:
root.right.next=root.next.left
self.connect(root.left)
self.connect(root.right)
return root
117. 填充每个节点的下一个右侧节点指针 II

class Solution:
def connect(self, root: 'Node') -> 'Node':
if not root:
return root
queue=[root]
while queue:
temp=queue
queue=[]
for x,y in pairwise(temp):
x.next=y
for node in temp:
if node.left:
queue.append(node.left)
if node.right:
queue.append(node.right)
return root
118. 杨辉三角
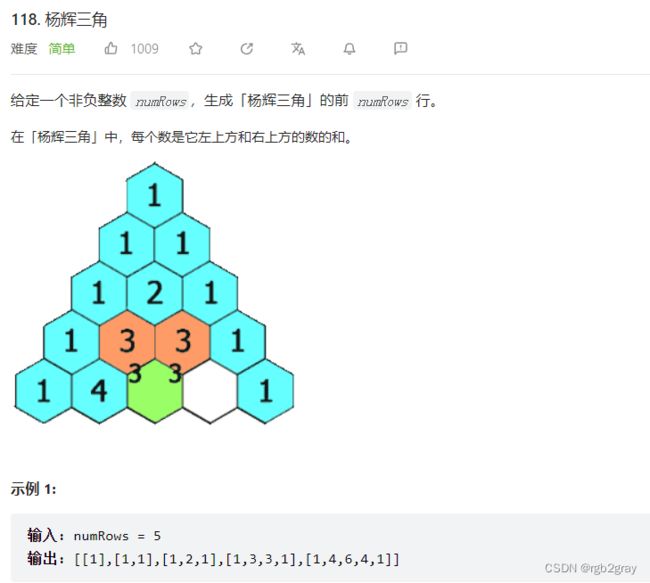
class Solution:
def generate(self, numRows: int) -> List[List[int]]:
res=[]
for i in range(numRows):
row=[]
for j in range(0,i+1):
if j==0 or j==i:
row.append(1)
else:
row.append(res[i-1][j]+res[i-1][j-1])
res.append(row)
return res
119. 杨辉三角 II
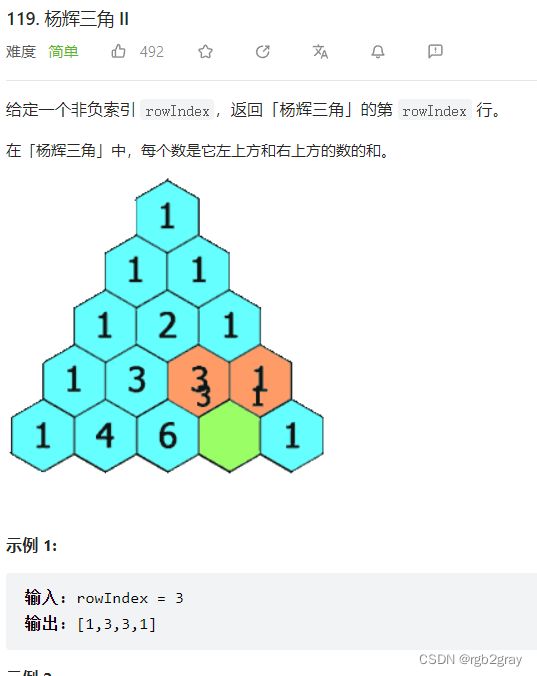
class Solution:
def getRow(self, rowIndex: int) -> List[int]:
res=[]
for i in range(rowIndex+1):
row=[]
for j in range(0,i+1):
if j==0 or i==j:
row.append(1)
else:
row.append(res[i-1][j]+res[i-1][j-1])
res.append(row)
return res[rowIndex]
120. 三角形最小路径和
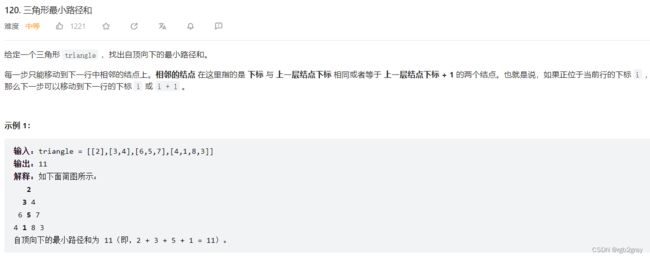
class Solution:
def minimumTotal(self, triangle: List[List[int]]) -> int:
triangle=triangle[::-1]
for i in range(1,len(triangle)):
for j in range(len(triangle[i])):
triangle[i][j]=triangle[i][j]+min(triangle[i-1][j],triangle[i-1][j+1])
return triangle[-1][-1]