Plugins文件夹里放这三个
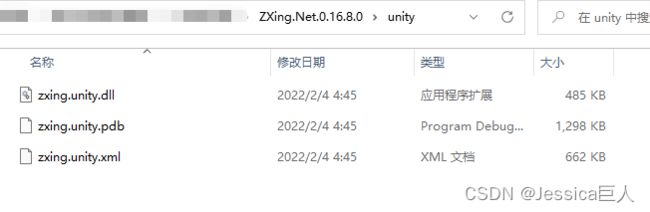
using System.IO;
public class CameraTextureSave : MonoBehaviour
{
public static void SaveOne(WebCamTexture t,int frame,int w,int h)
{
Texture2D t2d= new Texture2D(w, h, TextureFormat.ARGB32, true);
Vector2 offset = new Vector2((t.width - w) / 2, (t.height - h) / 2);
t2d.SetPixels(t.GetPixels((int)offset.x, (int)offset.y, w, h));
t2d.Apply();
byte[] imageTytes=t2d.EncodeToJPG();
File.WriteAllBytes(@"Assets\Resources\myTexture1.jpg", imageTytes);
}
public static void SaveTwo(WebCamTexture t,int frame,int w=850,int h=550)
{
Texture2D texture=new Texture2D(w,h, TextureFormat.ARGB32,true);
Vector2 offset=new Vector2((t.width-w)/2,(t.height-h)/2);
texture.SetPixels(t.GetPixels((int)offset.x,(int)offset.y,w,h));
texture.Apply();
byte[] imageTytes = texture.EncodeToJPG();
File.WriteAllBytes(@"Assets\Resources\myTexture2.jpg", imageTytes);
}
}
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
using ZXing;
using System.IO;
public class TestQRCodeScanning : MonoBehaviour
{
[Header("摄像机检测界面")]
public RawImage cameraTexture;
private WebCamTexture webCamTexture;
public int framecount = 0;
public int framecount1 = 0;
BarcodeReader barcodeReader;
public int CameraRequestedWidth = 1024;
public int CameraRequestedHeight = 1024;
void DeviceInit()
{
WebCamDevice[] devices = WebCamTexture.devices;
string deviceName = devices[0].name;
webCamTexture = new WebCamTexture(deviceName, 1024, 1024);
cameraTexture.texture = webCamTexture;
CameraRequestedWidth = webCamTexture.requestedWidth;
CameraRequestedHeight = webCamTexture.requestedHeight;
webCamTexture.Play();
barcodeReader = new BarcodeReader();
}
Color32[] data;
void ScanQRCode()
{
data = webCamTexture.GetPixels32();
Result result=barcodeReader.Decode(data,webCamTexture.width,webCamTexture.height);
if (result!=null)
{
Debug.Log(result.Text);
if (result.Text== "二维码")
{
CameraTextureSave.SaveOne(webCamTexture, framecount,850,550);
FishOneCreate();
framecount++;
}
else if (result.Text == "第一个")
{
CameraTextureSave.SaveTwo(webCamTexture, framecount1);
FishTwoCreate();
framecount1++;
}
}
}
private void Start()
{
DeviceInit();
IsScanning = true;
}
bool IsScanning = false;
float interval = 5;
[SerializeField]
Button scanningButton;
void ScanningButtonClick()
{
}
private void Update()
{
if (IsScanning)
{
interval += Time.deltaTime;
if (interval>=5)
{
interval = 0;
ScanQRCode();
}
}
}
public void FishOneCreate()
{
float x = Random.Range(-3.0f, 0);
float y = Random.Range(-3.0f, 0);
float z = Random.Range(-3.0f, 0);
Vector3 pos = new Vector3(x, y, z);
GameObject obj = Resources.Load<GameObject>("yu_1");
byte[] imgData = File.ReadAllBytes(@"Assets\Resources\myTexture1.jpg");
Texture mTexture = ByteToTex2d(imgData);
GameObject a = Instantiate(obj, pos, Quaternion.identity);
a.transform.Find("yu_1").GetComponent<Renderer>().material.mainTexture = mTexture;
}
public void FishTwoCreate()
{
float x = Random.Range(-3.0f, 0);
float y = Random.Range(-3.0f, 0);
float z = Random.Range(-3.0f, 0);
Vector3 pos = new Vector3(x, y, z);
GameObject obj = Resources.Load<GameObject>("yu_2");
byte[] imgData = File.ReadAllBytes(@"Assets\Resources\myTexture2.jpg");
Texture mTexture = ByteToTex2d(imgData);
GameObject a = Instantiate(obj, pos, Quaternion.identity);
a.transform.Find("yu_2").GetComponent<Renderer>().material.mainTexture = mTexture;
}
public static Texture2D ByteToTex2d(byte[] bytes, int w = 1024, int h = 1024)
{
Texture2D tex = new Texture2D(w, h);
tex.LoadImage(bytes);
return tex;
}
}
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
using ZXing;
using ZXing.QrCode;
public class TestQRCodeDraw : MonoBehaviour
{
[Header("绘制好的二维码显示界面")]
public RawImage QRCode;
BarcodeWriter barcodeWriter;
[SerializeField]
Button drawbutton;
Color32[] GeneQRCode(string formatStr, int width, int height)
{
QrCodeEncodingOptions options = new QrCodeEncodingOptions();
options.CharacterSet = "UTF-8";
options.Width= width;
options.Height= height;
options.Margin = 1;
barcodeWriter=new BarcodeWriter { Format = BarcodeFormat.QR_CODE,Options = options};
return barcodeWriter.Write(formatStr);
}
Texture2D showQRCode(string str,int width,int height)
{
Texture2D t=new Texture2D(width,height);
Color32[] col32=GeneQRCode(str,width,height);
t.SetPixels32(col32);
t.Apply();
return t;
}
void DrawQRCode(string formatStr)
{
Texture2D t=showQRCode(formatStr,256,256);
QRCode .texture = t;
}
public string QRCodeText = "二维码";
void DrawButtonClick()
{
DrawQRCode(QRCodeText);
}
private void Start()
{
drawbutton.onClick.AddListener(DrawButtonClick);
}
}
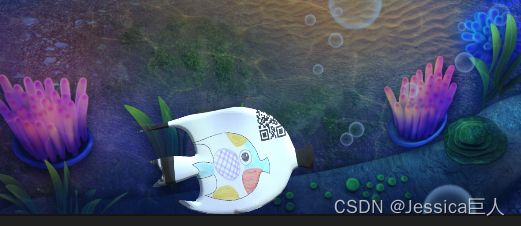