一、动态渲染表格
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
<style>
table {
width: 300px;
text-align: center;
}
</style>
</head>
<body>
<table border="1" cellspacing="0">
<thead>
<tr>
<th>ID</th>
<th>姓名</th>
<th>性别</th>
</tr>
</thead>
<tbody>
<!-- JS 渲染 -->
</tbody>
</table>
<script>
var users = [
{ id: 1, name: '前端小灰狼', age: 18 },
{ id: 2, name: '公羊无衣', age: 22 },
{ id: 3, name: '古艺散人', age: 30 }
]
var tbody = document.querySelector('tbody')
users.forEach(function (item) {
console.log(item)
var tr = document.createElement('tr')
for (var key in item) {
var td = document.createElement('td')
td.innerHTML = item[key]
tr.appendChild(td)
}
tbody.appendChild(tr)
})
</script>
</body>
</html>
二、事件上
1.事件绑定
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
<style>
div {
width: 200px;
height: 200px;
background-color: pink;
}
</style>
</head>
<body>
<div></div>
<script>
var div = document.querySelector('div')
div.onclick = function () {
console.log('啊 ! 救命啊 !!')
}
</script>
</body>
</html>
2.事件对象
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
<style>
div {
width: 200px;
height: 200px;
background-color: pink;
}
</style>
</head>
<body>
<div></div>
<script>
var div = document.querySelector('div')
div.onclick = function (e) {
console.log(e)
}
</script>
</body>
</html>
三、案列
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
<style>
img {
width: 50px;
height: 50px;
position: fixed;
left: 0;
top: 0;
}
</style>
</head>
<body>
<img src="./a.png" alt="">
<script>
var imgBox = document.querySelector('img')
document.onmousemove = function (e) {
var x = e.clientX
var y = e.clientY
imgBox.style.left = x + 5 + 'px'
imgBox.style.top = y + 5 + 'px'
}
</script>
</body>
</html>
下图案例图片
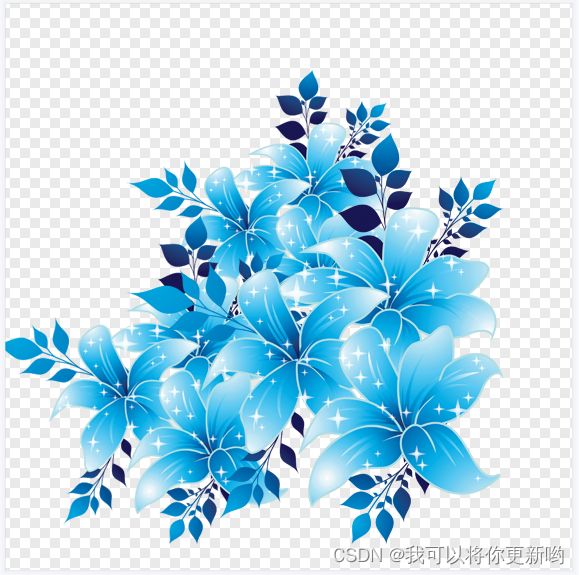
四、事件下
1.事件传播
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
<style>
* {
margin: 0;
padding: 0;
}
.outer {
width: 500px;
height: 500px;
display: flex;
justify-content: center;
align-items: center;
background-color: pink;
position: absolute;
left: 0;
top: 0;
right: 0;
bottom: 0;
margin: auto;
}
.center {
width: 300px;
height: 300px;
display: flex;
justify-content: center;
align-items: center;
background-color: skyblue;
}
.inner {
width: 100px;
height: 100px;
background-color: red;
}
</style>
</head>
<body>
<div class="outer">
<div class="center">
<div class="inner"></div>
</div>
</div>
<script>
var outer = document.querySelector('.outer')
var center = document.querySelector('.center')
var inner = document.querySelector('.inner')
outer.onclick = function () { console.log('我是 outer 元素, 我被点击了') }
center.onclick = function () { console.log('我是 center 元素, 我被点击了') }
inner.onclick = function () { console.log('我是 inner 元素, 我被点击了') }
</script>
</body>
</html>
2.阻止事件传播
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
<style>
* {
margin: 0;
padding: 0;
}
.outer {
width: 500px;
height: 500px;
display: flex;
justify-content: center;
align-items: center;
background-color: pink;
position: absolute;
left: 0;
top: 0;
right: 0;
bottom: 0;
margin: auto;
}
.center {
width: 300px;
height: 300px;
display: flex;
justify-content: center;
align-items: center;
background-color: skyblue;
}
.inner {
width: 100px;
height: 100px;
background-color: red;
}
</style>
</head>
<body>
<div class="outer">
<div class="center">
<div class="inner"></div>
</div>
</div>
<script>
var outer = document.querySelector('.outer')
var center = document.querySelector('.center')
var inner = document.querySelector('.inner')
outer.onclick = function () { console.log('我是 outer 元素, 我被点击了') }
center.onclick = function () { console.log('我是 center 元素, 我被点击了') }
inner.onclick = function (e) {
e.stopPropagation()
console.log('我是 inner 元素, 我被点击了')
}
</script>
</body>
</html>
3.事件委托
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
<style>
* {
margin: 0;
padding: 0;
}
ul, li {
list-style: none;
}
ul {
width: 800px;
height: 600px;
background-color: skyblue;
margin: 50px;
overflow: hidden;
}
ul > li {
float: left;
width: 100px;
height: 100px;
margin: 20px;
background-color: pink;
color: #fff;
display: flex;
justify-content: center;
align-items: center;
font-size: 20px;
cursor: pointer;
}
</style>
</head>
<body>
<ul>
<li>1</li>
<li>2</li>
<li>3</li>
</ul>
<script>
var ul = document.querySelector('ul')
ul.onclick = function (e) {
if (e.target.tagName === 'LI') {
console.log('你点击的是 li')
}
}
</script>
</body>
</html>
五、轮播图
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
<style>
* {
margin: 0;
padding: 0;
}
ul, ol, li {
list-style: none;
}
img {
width: 100%;
height: 100%;
display: block;
}
.banner {
width: 100%;
height: 500px;
position: relative;
margin: 50px 0;
}
.banner > ul {
width: 100%;
height: 100%;
position: relative;
}
.banner > ul > li {
width: 100%;
height: 100%;
position: absolute;
left: 0;
top: 0;
opacity: 0;
transition: opacity .5s linear;
}
.banner > ul > li.active {
opacity: 1;
}
.banner > ol {
width: 200px;
height: 30px;
position: absolute;
left: 30px;
bottom: 30px;
background-color: rgba(0, 0, 0, .5);
display: flex;
justify-content: space-around;
align-items: center;
border-radius: 15px;
}
.banner > ol > li {
width: 20px;
height: 20px;
background-color: #fff;
border-radius: 50%;
cursor: pointer;
}
.banner > ol > li.active {
background-color: orange;
}
.banner > div {
width: 40px;
height: 60px;
position: absolute;
top: 50%;
transform: translateY(-50%);
background-color: rgba(0, 0, 0, .5);
display: flex;
justify-content: center;
align-items: center;
font-size: 30px;
color: #fff;
cursor: pointer;
}
.banner > div.left {
left: 0;
}
.banner > div.right {
right: 0;
}
</style>
</head>
<body>
<div class="banner">
<!-- 图片区域 -->
<ul class="imgBox">
<li class="active"><img src="https://img.zcool.cn/community/018add5cd53d9ea80121416837a1b6.jpg@1280w_1l_2o_100sh.jpg" alt=""></li>
<li><img src="https://img.zcool.cn/community/0143625aed952ca801207fa1daee09.JPG@1280w_1l_2o_100sh.jpg" alt=""></li>
<li><img src="https://img.zcool.cn/community/01e99a5821e425a84a0d304fbecf48.jpg@1280w_1l_2o_100sh.jpg" alt=""></li>
<li><img src="https://img.zcool.cn/community/[email protected]" alt=""></li>
</ul>
<!-- 焦点区域 -->
<ol>
<li data-i="0" data-name="point" class="active"></li>
<li data-i="1" data-name="point"></li>
<li data-i="2" data-name="point"></li>
<li data-i="3" data-name="point"></li>
</ol>
<!-- 左右切换按钮 -->
<div class="left"><</div>
<div class="right">></div>
</div>
<script>
var imgs = document.querySelectorAll('ul > li')
var points = document.querySelectorAll('ol > li')
var banner = document.querySelector('.banner')
var index = 0
function changeOne(type){
imgs[index].className = '';
points[index].className = '';
if(type === true){
index++;
} else if(type === false){
index--;
}else{
index = type;
}
if(index >= imgs.length){
index=0;
}
if(index<0){
index=imgs.length-1;
}
imgs[index].className = 'active';
points[index].className = 'active';
}
banner.onclick = function(e){
if(e.target.className === 'left'){
console.log('点击的是左按钮');
changeOne(false);
}
if(e.target.className === 'right'){
console.log('点击的是右按钮');
changeOne(true);
}
if(e.target.dataset.name === 'point'){
console.log('点击的是焦点盒子');
var i = e.target.dataset.i-0;
changeOne(i);
}
}
setInterval(function () {
changeOne(true);
}, 3000);
</script>
</body>
</html>