1、构造初始化
#include
#include
using namespace std;
void PrintVector(vector<int>& v)
{
for (vector<int>::iterator it = v.begin(); it != v.end(); it++)
{
cout << *it << " ";
}
cout << endl;
}
void test01()
{
vector<int> v1;
int arr[] = { 10,20,30,40 };
vector<int> v2(arr, arr + sizeof(arr) / sizeof(int));
vector<int> v3(v2.begin(), v2.end());
vector<int> v4(v3);
PrintVector(v2);
PrintVector(v3);
PrintVector(v4);
}
int main()
{
test01();
return 0;
}
2、赋值操作
#include
#include
using namespace std;
void PrintVector(vector<int>& v)
{
for (vector<int>::iterator it = v.begin(); it != v.end(); it++)
{
cout << *it << " ";
}
cout << endl;
}
void test01()
{
int arr[] = { 10, 20, 30, 40 };
vector<int> v1(arr, arr + sizeof(arr) / sizeof(int));
vector<int> v2;
v2.assign(v1.begin(), v1.end());
vector<int> v3;
v3.assign(5, 1000);
vector<int> v4;
v4 = v3;
int array[] = { 100, 200, 300, 400 };
vector<int> v5(array, array + sizeof(array) / sizeof(int));
v5.swap(v1);
PrintVector(v1);
PrintVector(v2);
PrintVector(v3);
PrintVector(v4);
PrintVector(v5);
}
int main()
{
test01();
return 0;
}
3、容量和大小
#include
#include
using namespace std;
void PrintVector(vector<int>& v)
{
for (vector<int>::iterator it = v.begin(); it != v.end(); it++)
{
cout << *it << " ";
}
cout << endl;
}
void test01()
{
vector<int> v;
for (int i = 1; i <= 4; i++)
v.push_back(i * 10);
cout << "capacity: " << v.capacity() << endl;
cout << "size: " << v.size() << endl;
cout << "empty? " << v.empty() << endl;
PrintVector(v);
cout << "----------------------------------------" << endl;
v.resize(6);
PrintVector(v);
v.resize(2);
PrintVector(v);
cout << "----------------------------------------" << endl;
v.resize(4, 88);
PrintVector(v);
v.resize(2);
PrintVector(v);
}
int main()
{
test01();
return 0;
}
4、存取操作
#include
#include
using namespace std;
void PrintVector(vector<int>& v)
{
for (vector<int>::iterator it = v.begin(); it != v.end(); it++)
{
cout << *it << " ";
}
cout << endl;
}
void test01()
{
int array[] = { 100, 200, 300, 400 };
vector<int> v(array, array + sizeof(array) / sizeof(int));
for (int i = 0; i < v.size(); i++)
cout << v[i] << " ";
cout << endl;
cout << "------------" << endl;
for (int i = 0; i < v.size(); i++)
cout << v.at(i) << " ";
cout << endl;
cout << v.front() << endl;
cout << v.back() << endl;
}
int main()
{
test01();
return 0;
}
5、插入删除操作
#include
#include
using namespace std;
void PrintVector(vector<int>& v)
{
for (vector<int>::iterator it = v.begin(); it != v.end(); it++)
{
cout << *it << " ";
}
cout << endl;
}
void test01()
{
int array[] = { 10, 20, 30, 40 };
vector<int> v(array, array + sizeof(array) / sizeof(int));
v.insert(v.begin(), 336);
PrintVector(v);
v.insert(v.begin() + 2, 100);
PrintVector(v);
v.insert(v.begin() + 1, 3, 88);
PrintVector(v);
v.insert(v.begin(), array, array + sizeof(array) / sizeof(int));
PrintVector(v);
cout << "----------------------------------" << endl;
v.erase(v.begin());
PrintVector(v);
v.erase(v.begin()+2, v.begin() + 6);
PrintVector(v);
v.clear();
PrintVector(v);
cout << "size: " << v.size() << endl;
}
int main()
{
test01();
return 0;
}
6、利用swap缩减空间
#include
#include
using namespace std;
void PrintVector(vector<int>& v)
{
for (vector<int>::iterator it = v.begin(); it != v.end(); it++)
{
cout << *it << " ";
}
cout << endl;
}
void PrintVector(vector<int>&& v)
{
for (vector<int>::iterator it = v.begin(); it != v.end(); it++)
{
cout << *it << " ";
}
cout << endl;
}
void test01()
{
vector<int> v;
for (int i = 0; i < 100000; i++)
v.push_back(i);
cout << "size: " << v.size() << endl;
cout << "capacity: " << v.capacity() << endl;
cout << "--------------------------" << endl;
v.resize(10);
cout << "size: " << v.size() << endl;
cout << "capacity: " << v.capacity() << endl;
PrintVector(v);
cout << "------------缩减空间容量------------" << endl;
vector<int>(v).swap(v);
cout << "size: " << v.size() << endl;
cout << "capacity: " << v.capacity() << endl;
}
int main()
{
test01();
return 0;
}
7、reverse预留空间
#include
#include
using namespace std;
void PrintVector(vector<int>& v)
{
for (vector<int>::iterator it = v.begin(); it != v.end(); it++)
{
cout << *it << " ";
}
cout << endl;
}
void test01()
{
vector<int> v;
int count = 0;
int* p = NULL;
v.reserve(100000);
for (int i = 0; i < 100000; i++)
{
v.push_back(i);
if (p != &v[0])
{
p = &v[0];
count++;
}
}
cout << "count: " << count << endl;
}
int main()
{
test01();
return 0;
}
8、自动扩增
void test01()
{
vector<int> v;
cout << "capacity:" << v.capacity() << endl;
v.push_back(1);
cout << "capacity:" << v.capacity() << endl;
v.push_back(2);
cout << "capacity:" << v.capacity() << endl;
v.push_back(3);
cout << "capacity:" << v.capacity() << endl;
v.push_back(4);
cout << "capacity:" << v.capacity() << endl;
v.push_back(5);
cout << "capacity:" << v.capacity() << endl;
v.push_back(6);
cout << "capacity:" << v.capacity() << endl;
v.push_back(7);
cout << "capacity:" << v.capacity() << endl;
v.push_back(8);
cout << "capacity:" << v.capacity() << endl;
v.push_back(9);
cout << "capacity:" << v.capacity() << endl;
v.push_back(10);
cout << "capacity:" << v.capacity() << endl;
}
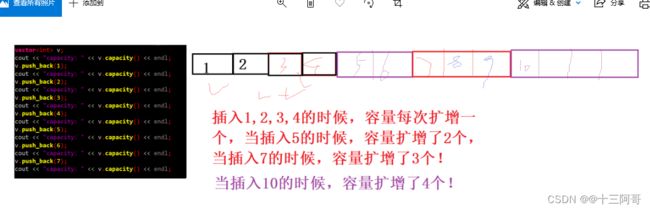
9、遍历
void test02()
{
vector<int> v;
for (int i = 0; i < 5; i++)
v.push_back(i);
cout << "-----------------iterator-------------------------" << endl;
for (vector<int>::iterator it = v.begin(); it != v.end(); it++)
cout << *it << " ";
cout << endl << "-----------------reverse_iterator-------------------------" << endl;
for (vector<int>::reverse_iterator rit = v.rbegin(); rit != v.rend(); rit++)
cout << *rit << " ";
cout << endl;
}
void test03()
{
cout << "普通对象 对应于 普通迭代器!" << endl;
cout << "常对象 对应于 常迭代器!" << endl;
cout << "--------------------普通对象下的迭代器--------------------" << endl;
vector<int> v1(5,3);
for (vector<int>::iterator it = v1.begin(); it != v1.end(); it++)
{
cout << *it << " ";
}
cout << endl << "---------------------常对象下的迭代器--------------------" << endl;
const vector<int> v2(5, 2);
for (vector<int>::const_iterator c_it = v2.begin(); c_it != v2.end(); c_it++)
{
cout << *c_it << " ";
}
cout << endl;
}