一、vue 中 引入 mapbox (同一页面进行多次切换操作)
1.mapbox的 底图用天地图
安装 @mapbox/mapbox-gl-geocoder
npm install --save @mapbox/mapbox-gl-geocoder
安装 mapbox-gl
npm install --save mapbox-gl
2.图片展示
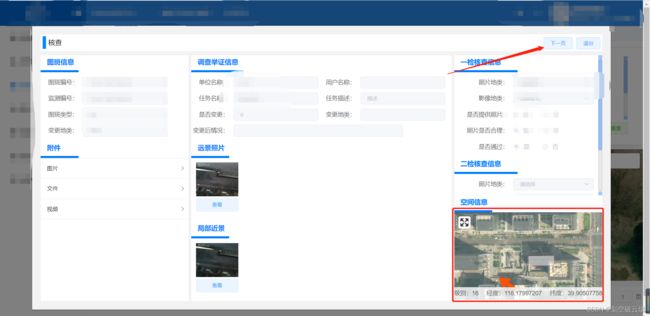
3.代码
<template>
<div class="map-box-inspect">
<div
id="inspectMap"
:style="{ height: height + 'px', width: width + 'px' }"
>
</div>
<div class="calculation-box">
<div class="bottom">
<div>级别:{{ currentZoom }}</div>
<div>经度:{{ lng }}</div>
<div>纬度:{{ lat }}</div>
</div>
</div>
</div>
</template>
<script>
import "mapbox-gl/dist/mapbox-gl.css";
import MapboxLanguage from "@mapbox/mapbox-gl-language";
import MapboxGeocoder from "@mapbox/mapbox-gl-geocoder";
import "@mapbox/mapbox-gl-geocoder/dist/mapbox-gl-geocoder.css";
import { createApp, ref, h } from "vue";
import pop from "./pop.vue";
const mapboxgl = require("mapbox-gl");
export default {
name: "inspectMap",
props: {
height: {
type: Number,
default: null,
},
width: {
type: Number,
default: null,
},
mapData: {
type: Object,
default: () => {},
},
},
data() {
return {
map: null,
center: [116.177794023, 39.905043954],
tbfwData: "",
arrowData: [],
featuresData: [],
flyCoordinates: [],
picCoordinates: [],
tbObj: {},
layers: [],
lng: "",
lat: "",
currentZoom: "",
flyZoom: 16,
};
},
mounted() {
},
beforeDestroy() {
this.map.remove();
},
watch: {
mapData: {
immediate: false,
deep: true,
handler: function (newVal, oldVal) {
if (newVal !== null) {
this.tbfwData = newVal.tbfw;
this.tbObj = JSON.parse(this.tbfwData);
this.flyCoordinates = this.tbObj.coordinates[0][0][0];
let arr1 = [];
this.arrowData = newVal.arrow;
this.arrowData.forEach((item, index) => {
let obj = {
type: "Feature",
properties: {
description: "箭头的信息",
Name: "拍摄角度1",
icon: "arrow-r",
rotate: 60,
},
geometry: {
type: "Point",
coordinates: [116.17383, 39.9014],
},
};
obj.properties.rotate = item.psjd;
obj.geometry.coordinates = [item.longitude, item.latitude];
obj.properties.fjmc = item.fjmc;
arr1.push(obj);
return arr1;
});
this.featuresData = arr1;
this.featuresData.forEach((item, index) => {
item.properties.icon = "arrow-r";
if (item.properties.fjmc === newVal.currentFjmc) {
item.properties.icon = "arrow-g";
this.picCoordinates = item.geometry.coordinates;
this.flyCoordinates = this.picCoordinates;
}
});
if (this.picCoordinates[0] === 0 || this.picCoordinates[1] === 0) {
this.$message.error(`该照片没有经纬度`);
} else {
this.$nextTick(() => {
this.initMap();
});
}
}
},
},
},
methods: {
initMap() {
if (this.map) {
this.clearArrowLayerFn();
this.loadArrowLayersFn();
this.clearTbLayerFn();
this.loadTbLayersFn();
this.flyTo();
} else {
mapboxgl.accessToken =
"pk.eyJ1IjoiY2FvcnVpYmluIiwiYSI6ImNsYWR3MjEwMjA5b2UzcW85dmZlbTVtMTAifQ.4v81PyG-oZ6TVL7IuyCbrg";
this.map = new mapboxgl.Map({
container: "inspectMap",
style: {
version: 8,
sources: {
"tian-satellite1": {
type: "raster",
tiles: [
"http://t0.tianditu.gov.cn/img_w/wmts?tk=1883a2da124fe27b3c281f9d65356e82&SERVICE=WMTS&REQUEST=GetTile&VERSION=1.0.0&LAYER=img&STYLE=default&TILEMATRIXSET=w&TILEMATRIX={z}&TILEROW={y}&TILECOL={x}&FORMAT=tiles",
],
tileSize: 256,
minzoom: 0,
maxzoom: 18,
},
},
layers: [
{
id: "tian-satellite1",
type: "raster",
source: "tian-satellite1",
layout: {
visibility: "none",
},
minzoom: 0,
maxzoom: 22,
},
],
glyphs: "mapbox://fonts/mapbox/{fontstack}/{range}.pbf",
},
center: [116.177794023, 39.905043954],
zoom: 10,
});
this.fullscreenControl = new mapboxgl.FullscreenControl();
this.map.addControl(this.fullscreenControl, "top-left");
this.loadArrowImg();
this.map.on("load", (e) => {
this.map.setFog({});
this.map.setLayoutProperty(
"tian-satellite1",
"visibility",
"visible"
);
this.map.on("mousemove", (e) => {
this.lng = e.lngLat.lng.toFixed(8);
this.lat = e.lngLat.lat.toFixed(8);
});
this.currentZoom = this.flyZoom;
this.map.on("wheel", (e) => {
let zoom = this.map.getZoom();
this.currentZoom = parseInt(zoom);
});
this.map.on("click", "tbLayer", (e) => {
console.log("@eeeee", e.features[0]);
});
this.loadArrowLayersFn();
this.loadTbLayersFn();
this.flyTo();
});
}
},
flyTo() {
this.map.flyTo({
center: this.flyCoordinates,
zoom: this.flyZoom,
});
},
loadTbLayersFn() {
this.map.addSource("tbSource", {
type: "geojson",
data: this.tbObj,
});
this.map.addLayer({
id: "tbLayer",
type: "fill",
source: "tbSource",
layout: {},
paint: {
"fill-color": "#fff",
"fill-opacity": 0.5,
"fill-outline-color": "red",
},
});
this.map.on("mouseenter", "tbLayer", (e) => {
this.map.getCanvas().style.cursor = "pointer";
});
this.map.on("mouseleave", "tbLayer", () => {
this.map.getCanvas().style.cursor = "";
});
},
loadArrowImg() {
let url = "./resource/arrow-g.png";
let url1 = "./resource/arrow-r.png";
this.map.loadImage(url, (error, image) => {
this.map.addImage("arrow-g", image);
});
this.map.loadImage(url1, (error, image) => {
this.map.addImage("arrow-r", image);
});
},
loadArrowLayersFn() {
if (this.arrowData.length > 0) {
this.map.addSource("arrowSource", {
type: "geojson",
data: {
type: "FeatureCollection",
name: "拍摄角度",
features: this.featuresData,
},
});
this.map.addLayer({
id: "arrowLayer",
type: "symbol",
source: "arrowSource",
layout: {
"text-font": ["Open Sans Semibold", "Arial Unicode MS Bold"],
"icon-image": ["get", "icon"],
"text-field": ["get", "Name"],
"icon-ignore-placement": true,
"text-offset": [0, 1.25],
"text-anchor": "top",
"icon-size": 0.4,
"icon-rotation-alignment": "map",
"icon-rotate": ["get", "rotate"],
"text-size": 10,
},
paint: {
"text-halo-color": "rgb(255,255,255)",
"text-halo-width": 0.5,
},
});
this.map.on("click", "arrowLayer", (e) => {
console.log("@eeeee", e.features[0]);
});
this.map.on("mouseenter", "arrowLayer", () => {
this.map.getCanvas().style.cursor = "pointer";
});
this.map.on("mouseleave", "arrowLayer", () => {
this.map.getCanvas().style.cursor = "";
});
}
},
clearTbLayerFn() {
if (this.map && this.map.getLayer("tbLayer")) {
this.map.removeLayer("tbLayer");
}
if (this.map && this.map.getSource("tbSource")) {
this.map.removeSource("tbSource");
}
},
clearArrowLayerFn() {
if (this.map && this.map.getLayer("arrowLayer")) {
this.map.removeLayer("arrowLayer");
}
if (this.map && this.map.getSource("arrowSource")) {
this.map.removeSource("arrowSource");
}
},
clearLayerFn() {
this.clearTbLayerFn();
this.clearArrowLayerFn();
},
mountDomFn(value) {
let app = createApp(
h(pop, {
objes: value || "传过去的值是 6666",
})
);
const parent = document.createElement("div");
const instance = app.mount(parent);
return instance;
},
},
};
</script>
<style lang="less" scoped>
.map-box-inspect {
position: relative;
width: 100%;
height: 100%;
#inspectMap {
width: 100%;
height: 100%;
/deep/ .mapboxgl-canvas {
}
/deep/ .mapboxgl-control-container {
.mapboxgl-ctrl-bottom-left {
.mapboxgl-ctrl {
.mapboxgl-ctrl-logo {
display: none;
}
}
}
.mapboxgl-ctrl-bottom-right {
.mapboxgl-ctrl-attrib-inner {
display: none;
}
.mapboxgl-compact {
display: none;
}
}
}
}
.calculation-box {
height: 30px;
width: 100%;
position: absolute;
bottom: 0px;
left: 0px;
background-color: rgba(255, 255, 255, 0.8);
text-align: center;
.bottom {
line-height: 30px;
display: flex;
justify-content: space-between;
}
}
}
</style>
4.模拟地图所需的数据
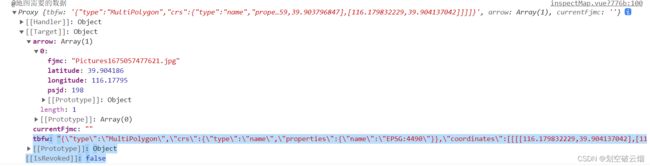
{
"tbfw": "{\"type\":\"MultiPolygon\",\"crs\":{\"type\":\"name\",\"properties\":{\"name\":\"EPSG:4490\"}},\"coordinates\":[[[[116.179832229,39.904137042],[116.180285516,39.904165596],[116.180306946,39.903825399],[116.179853659,39.903796847],[116.179832229,39.904137042]]]]}",
"arrow": [
{
"latitude": 39.904186,
"longitude": 116.17795,
"psjd": 198,
"fjmc": "Pictures1675057477621.jpg"
}
],
"currentFjmc": ""
}