一、绘制背景
import pygame
from pygame.locals import *
import sys
import os
# 初始化
pygame.init()
# 背景大小设置
bg_size = (1200, 600)
# 设置屏幕背景大小
screen = pygame.display.set_mode(bg_size)
#设置屏幕标题
pygame.display.set_caption("植物大战僵尸")
# 设置图片路径
# rootpath = os.getcwd()
# imgpath = os.path.join(rootpath,background_path)
background_path = "material/images/background1.jpg"
sun_path = "material/images/SunBack.png"
# 加载背景图片
backgrounImg = pygame.image.load(background_path).convert()
sunImg = pygame.image.load(sun_path).convert()
# 设置文本
myfont = pygame.font.SysFont("arial",20)
txtImg = myfont.render("50",True,(255,255,0))
while True:
# 启动消息队列,获取消息并处理
for event in pygame.event.get():
if event.type == QUIT:
sys.exit()
# 绘制背景
screen.blit(backgrounImg, (0, 0))
# 绘制顶部太阳数量栏
screen.blit(sunImg, (250, 0))
screen.blit(txtImg,(320,6))
pygame.display.update()
二、添加豌豆射手
豌豆类
import pygame
class Peashooter:
def __init__(self):
self.images = [pygame.image.load('material/images/Peashooter_{:02d}.png'.format(i)).convert_alpha() for i in
range(0, 13)]
self.rect = self.images[0].get_rect()
self.rect.top = 100
self.rect.left = 250
import pygame
from pygame.locals import *
import sys
from Peashooter import Perashooter
# import os
# rootPath = os.getcwd()
# backgroundPath = os.path.join(rootPath, 'material/images/background2.jpg')
# sunbackPath = os.path.join(rootPath, 'material/images/SunBack.png')
# 初始化pygame
pygame.init()
size = (1200, 600)
# 设置屏幕宽高
screen = pygame.display.set_mode(size)
# 设置屏幕标题
pygame.display.set_caption("植物大战僵尸")
backgroundImg = pygame.image.load('material/images/background1.jpg').convert_alpha()
sunbackImg = pygame.image.load('material/images/SunBack.png').convert_alpha()
myfont = pygame.font.SysFont('arial', 30)
txtImg = myfont.render("1000", True, (0, 0, 0))
peashooter = Perashooter()
index = 0
clock = pygame.time.Clock()
while True:
if index > 100:
index = 0
clock.tick(15)
# 启动消息队列,获取消息并处理
for event in pygame.event.get():
if event.type == QUIT:
pygame.quit()
sys.exit()
screen.blit(backgroundImg, (0, 0))
screen.blit(sunbackImg, (250, 30))
screen.blit(txtImg, (300, 33))
screen.blit(peashooter.images[index % 13], peashooter.rect)
index += 1
pygame.display.update()
让豌豆射手动起来----豌豆射手类改为
帧率的概念
FPS:Frames Per Second
屏幕每秒刷新次数
用Pygame.time.Clock()控制帧速率
并不是向每个循环凌驾一个延迟,Pygame.time.Clock()会控制每个循环多长时间运行一次。这就像一个定时器在控制时间进程,指出“现在开始下一个循环”!现在开始下一个循环!……
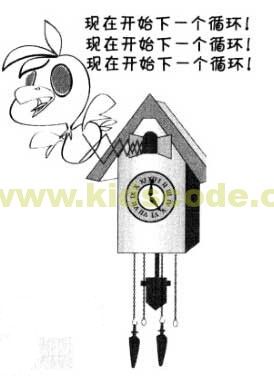
与孩子一起学编程-python教程
使用Pygame时钟之前,必须先创建Clock对象的一个实例,这与创建其他类的实例完全相同。
Clock= Pygame.time.Clock()
然后在主循环体中,只需要告诉时钟多久“滴答”一次-------也就是说,循环应该多长时间运行一次:clock.tick(60)
传入clock.tick()的数不是一个毫秒数。这是每秒内循环要运行的次数,所以这个循环应当每秒运行60次,在这里我只是说应当运行,因为循环只能按计算机能够保证的速度运行,每秒60个循环(或帧)时,每个循环需要1000/60=16.66ms(大约17ms)如果循环中的代码运行时间超过17ms,在clock指出下一次循环时当前循环将无法完成。
实际上,这说明对于图形运行的帧速率有一个限制,这个限制取次于图形的复杂程度、窗口大小以及运行这个程序的计算机的速度。对于一个特定的程序,计算机的运行速度可能是90fps,而较早的一个较慢的计算机也许只能以10fps的速度缓慢运行。
对于非常复杂的图形,大多数现代计算机都完全可以按20-30fps的速率运行Pygame程序。所以如果希望你的游戏在大多数计算机上都能以相同的速度运行,可以选择一个20-30fps(或者更低)的帧速率。这已经很快了,足以生成看上去流畅的运动。从现在开始,这本书中的例子都将使用clock.tick(30)
import pygame
# 创建类
class Perashooter(pygame.sprite.Sprite):
# 创建函数
def __init__(self):
super(Perashooter,self).__init__()
# 创建豌豆射手对象
self.image = pygame.image.load('material/images/Peashooter_00.png').convert_alpha()
# 创建动画
self.images = [pygame.image.load('material/images/Peashooter_{:02d}.png'.format(i)) for i in range(0, 13)]
# 利用坐标函数获得左边
self.rect = self.images[0].get_rect()
self.rect.top = 100
self.rect.left = 250
def update(self, *args):
self.image = self.images[args[0] % len(self.images)]
三、添加太阳花
太阳花类
import pygame
# 创建类
class SunFlower(pygame.sprite.Sprite):
# 创建函数
def __init__(self):
super(SunFlower,self).__init__()
# 创建豌豆射手对象
self.image = pygame.image.load('material/images/SunFlower_00.png').convert_alpha()
# 创建动画
self.images = [pygame.image.load('material/images/SunFlower_{:02d}.png'.format(i)) for i in range(0, 13)]
# 利用坐标函数获得左边
self.rect = self.images[0].get_rect()
self.rect.top = 180
self.rect.left = 230
def update(self, *args):
self.image = self.images[args[0] % len(self.images)]
import pygame
from pygame.locals import *
import sys
from Peashooter import Perashooter
from SunFlower import SunFlower
# import os
# rootPath = os.getcwd()
# backgroundPath = os.path.join(rootPath, 'material/images/background2.jpg')
# sunbackPath = os.path.join(rootPath, 'material/images/SunBack.png')
# 初始化pygame
pygame.init()
size = (1200, 600)
# 设置屏幕宽高
screen = pygame.display.set_mode(size)
# 设置屏幕标题
pygame.display.set_caption("植物大战僵尸")
backgroundImg = pygame.image.load('material/images/background1.jpg').convert_alpha()
sunbackImg = pygame.image.load('material/images/SunBack.png').convert_alpha()
myfont = pygame.font.SysFont('arial', 30)
txtImg = myfont.render("50", True, (0, 0, 0))
peashooter = Perashooter()
sunFlower = SunFlower()
index = 0
clock = pygame.time.Clock()
while True:
if index > 100:
index = 0
clock.tick(10)
# 启动消息队列,获取消息并处理
for event in pygame.event.get():
if event.type == QUIT:
pygame.quit()
sys.exit()
screen.blit(backgroundImg, (0, 0))
screen.blit(sunbackImg, (250, 30))
screen.blit(txtImg, (300, 33))
screen.blit(peashooter.images[index % 13], peashooter.rect)
index += 1
screen.blit(sunFlower.images[index % 13], sunFlower.rect)
index += 1
pygame.display.update()
四、添加坚果
坚果类
import pygame
# 创建类
class WallNut(pygame.sprite.Sprite):
# 创建函数
def __init__(self):
super(WallNut, self).__init__()
# 创建豌豆射手对象
self.image = pygame.image.load('material/images/WallNut_00.png').convert_alpha()
# 创建动画
self.images = [pygame.image.load('material/images/WallNut_{:02d}.png'.format(i)) for i in range(0, 13)]
# 利用坐标函数获得左边
self.rect = self.images[0].get_rect()
self.rect.top = 260
self.rect.left = 230
# 更新精灵的位置
def update(self, *args):
self.image = self.images[args[0] % len(self.images)]
import pygame
from pygame.locals import *
import sys
from Peashooter import Perashooter
from SunFlower import SunFlower
from WallNut import WallNut
# import os
# rootPath = os.getcwd()
# backgroundPath = os.path.join(rootPath, 'material/images/background2.jpg')
# sunbackPath = os.path.join(rootPath, 'material/images/SunBack.png')
# 初始化pygame
pygame.init()
size = (1200, 600)
# 设置屏幕宽高
screen = pygame.display.set_mode(size)
# 设置屏幕标题
pygame.display.set_caption("植物大战僵尸")
backgroundImg = pygame.image.load('material/images/background1.jpg').convert_alpha()
sunbackImg = pygame.image.load('material/images/SunBack.png').convert_alpha()
myfont = pygame.font.SysFont('arial', 30)
txtImg = myfont.render("50", True, (0, 0, 0))
peashooter = Perashooter()
sunFlower = SunFlower()
wallnut = WallNut()
index = 0
clock = pygame.time.Clock()
while True:
if index > 100:
index = 0
clock.tick(5)
# 启动消息队列,获取消息并处理
for event in pygame.event.get():
if event.type == QUIT:
pygame.quit()
sys.exit()
screen.blit(backgroundImg, (0, 0))
screen.blit(sunbackImg, (250, 30))
screen.blit(txtImg, (300, 33))
screen.blit(peashooter.images[index % 13], peashooter.rect)
index += 1
screen.blit(sunFlower.images[index % 13], sunFlower.rect)
index += 1
screen.blit(wallnut.images[index % 13], wallnut.rect)
index += 1
pygame.display.update()
五、添加太阳
太阳类
import pygame
import random
class Sun:
def __init__(self, rect):
self.images = [pygame.image.load('material/images/Sun_{}.png'.format(i)).convert_alpha() for i in
range(1, 18)]
self.rect = self.images[0].get_rect()
offsetTop = random.randint(-50, 50)
offsetLeft = random.randint(-50, 50)
self.rect.top = rect.top + offsetTop
self.rect.left = rect.left + offsetLeft
import pygame
from pygame.locals import *
import sys
from Peashooter import Perashooter
from SunFlower import SunFlower
from WallNut import WallNut
from Sun import Sun
# import os
# rootPath = os.getcwd()
# backgroundPath = os.path.join(rootPath, 'material/images/background2.jpg')
# sunbackPath = os.path.join(rootPath, 'material/images/SunBack.png')
# 初始化pygame
pygame.init()
size = (1200, 600)
# 设置屏幕宽高
screen = pygame.display.set_mode(size)
# 设置屏幕标题
pygame.display.set_caption("植物大战僵尸")
# 添加背景
backgroundImg = pygame.image.load('material/images/background1.jpg').convert_alpha()
sunbackImg = pygame.image.load('material/images/SunBack.png').convert_alpha()
# 创建字体
myfont = pygame.font.SysFont('arial', 30)
txtImg = myfont.render("50", True, (0, 0, 0))
# 创建类对象
peashooter = Perashooter()
sunFlower = SunFlower()
wallnut = WallNut()
sunList = pygame.sprite.Group()
sunList.add(peashooter)
sunList.add(sunFlower)
sunList.add(wallnut)
index = 0
clock = pygame.time.Clock()
while True:
if index > 100:
index = 0
clock.tick(10)
# 启动消息队列,获取消息并处理
for event in pygame.event.get():
if event.type == QUIT:
pygame.quit()
sys.exit()
screen.blit(backgroundImg, (0, 0))
screen.blit(sunbackImg, (250, 30))
screen.blit(txtImg, (300, 33))
# 屏幕显示动画效果
# screen.blit(peashooter.images[index % 13], peashooter.rect)
# screen.blit(sunFlower.images[index % 13], sunFlower.rect)
# screen.blit(wallnut.images[index % 13], wallnut.rect)
if index % 30 == 0:
# 找到太阳花定位
sun = Sun(sunFlower.rect)
# 向集合里存太阳花
sunList.add(sun)
# 遍历太阳花
sunList.update(index)
sunList.draw(screen)
index += 1
pygame.display.update()
六、添加僵尸
僵尸类
import pygame
import random
# 创建类 继承精灵类
class Zombie(pygame.sprite.Sprite):
# 创建函数
def __init__(self):
super(Zombie, self).__init__()
# 创建僵尸对象
self.image = pygame.image.load('material/images/Zombie_0.png').convert_alpha()
# 创建动画
self.images = [pygame.image.load('material/images/Zombie_{}.png'.format(i)) for i in range(1, 18)]
# 利用坐标函数获得左边
self.rect = self.images[0].get_rect()
self.rect.top = 50
self.rect.left = 1000
self.speed = 1
# 更新精灵的位置
def update(self, *args):
self.image = self.images[args[0] % len(self.images)]
if self.rect.left > 250:
self.rect.left -= self.speed
import pygame
from pygame.locals import *
import sys
from Peashooter import Perashooter
from SunFlower import SunFlower
from WallNut import WallNut
from Sun import Sun
# import os
# rootPath = os.getcwd()
# backgroundPath = os.path.join(rootPath, 'material/images/background2.jpg')
# sunbackPath = os.path.join(rootPath, 'material/images/SunBack.png')
# 初始化pygame
from Zombie import Zombie
pygame.init()
size = (1200, 600)
# 设置屏幕宽高
screen = pygame.display.set_mode(size)
# 设置屏幕标题
pygame.display.set_caption("植物大战僵尸")
# 添加背景
backgroundImg = pygame.image.load('material/images/background1.jpg').convert_alpha()
sunbackImg = pygame.image.load('material/images/SunBack.png').convert_alpha()
# 创建字体
myfont = pygame.font.SysFont('arial', 30)
txtImg = myfont.render("50", True, (0, 0, 0))
# 创建类对象
peashooter = Perashooter()
sunFlower = SunFlower()
wallnut = WallNut()
zombie = Zombie()
sunList = pygame.sprite.Group()
sunList.add(peashooter)
sunList.add(sunFlower)
sunList.add(wallnut)
sunList.add(zombie)
index = 0
clock = pygame.time.Clock()
while True:
if index > 100:
index = 0
clock.tick(10)
# 启动消息队列,获取消息并处理
for event in pygame.event.get():
if event.type == QUIT:
pygame.quit()
sys.exit()
screen.blit(backgroundImg, (0, 0))
screen.blit(sunbackImg, (250, 0))
screen.blit(txtImg, (300, 0))
# 屏幕显示动画效果
# screen.blit(peashooter.images[index % 13], peashooter.rect)
# screen.blit(sunFlower.images[index % 13], sunFlower.rect)
# screen.blit(wallnut.images[index % 13], wallnut.rect)
if index % 30 == 0:
# 找到太阳花定位
sun = Sun(sunFlower.rect)
# 向集合里存太阳花
sunList.add(sun)
# 遍历太阳花
sunList.update(index)
sunList.draw(screen)
index += 1
pygame.display.update()
七、豌豆发射子弹
子弹类
import pygame
# 创建类 继承精灵类
class Bullet(pygame.sprite.Sprite):
# 创建函数
def __init__(self, rect, bg_size):
super(Bullet, self).__init__()
# 创建子弹对象
self.image = pygame.image.load('material/images/Bullet_1.png').convert_alpha()
self.width,self.height = bg_size
# 利用坐标函数获得左边
self.rect = self.image.get_rect()
self.rect.top = rect.top
self.rect.left = rect.left + 50
self.speed = 10
# 更新精灵的位置
def update(self, *args):
if self.rect.left < self.width:
self.rect.left += self.speed
else:
self.kill()
import pygame
from pygame.locals import *
import sys
from Bullet import Bullet
from Peashooter import Peashooter
from Sun import Sun
from SunFlower import SunFlower
from WallNut import WallNut
# 初始化pygame
from Zombie import Zombie
pygame.init()
size = (1200, 600)
# 设置屏幕宽高
screen = pygame.display.set_mode(size)
# 设置屏幕标题
pygame.display.set_caption("植物大战僵尸")
backgroundImg = pygame.image.load('material/images/background1.jpg').convert_alpha()
sunbackImg = pygame.image.load('material/images/SunBack.png').convert_alpha()
myfont = pygame.font.SysFont('arial', 30)
txtImg = myfont.render("1000", True, (0, 0, 0))
peashooter = Peashooter()
sunFlower = SunFlower()
wallNut = WallNut()
zombie = Zombie()
sunList = pygame.sprite.Group()
sunList.add(peashooter)
sunList.add(sunFlower)
sunList.add(wallNut)
sunList.add(zombie)
index = 0
clock = pygame.time.Clock()
while True:
if index > 100:
index = 0
clock.tick(15)
# 启动消息队列,获取消息并处理
for event in pygame.event.get():
if event.type == QUIT:
pygame.quit()
sys.exit()
screen.blit(backgroundImg, (0, 0))
screen.blit(sunbackImg, (250, 30))
screen.blit(txtImg, (300, 33))
if index % 30 == 0:
bul = Bullet(peashooter.rect, size)
sunList.add(bul)
if index % 30 == 0:
sun = Sun(sunFlower.rect)
sunList.add(sun)
sunList.update(index)
sunList.draw(screen)
index += 1
pygame.display.update()
八、太阳花收集
import pygame
from pygame.locals import *
import sys
from Bullet import Bullet
from Peashooter import Perashooter
from SunFlower import SunFlower
from WallNut import WallNut
from Sun import Sun
# import os
# rootPath = os.getcwd()
# backgroundPath = os.path.join(rootPath, 'material/images/background2.jpg')
# sunbackPath = os.path.join(rootPath, 'material/images/SunBack.png')
# 初始化pygame
from Zombie import Zombie
pygame.init()
size = (1200, 600)
# 设置屏幕宽高
screen = pygame.display.set_mode(size)
# 设置屏幕标题
pygame.display.set_caption("植物大战僵尸")
# 添加背景
backgroundImg = pygame.image.load('material/images/background1.jpg').convert_alpha()
sunbackImg = pygame.image.load('material/images/SunBack.png').convert_alpha()
# 创建字体
score = '50'
myfont = pygame.font.SysFont('arial', 30)
txtImg = myfont.render("50", True, (0, 0, 0))
# 创建类对象
peashooter = Perashooter()
sunFlower = SunFlower()
wallnut = WallNut()
# zombie = Zombie()
spriteList = pygame.sprite.Group()
spriteList.add(peashooter)
spriteList.add(sunFlower)
spriteList.add(wallnut)
# spriteList.add(zombie)
zombieList = pygame.sprite.Group()
sunList = pygame.sprite.Group()
index = 0
clock = pygame.time.Clock()
# 自定义事件
GENERATOR_SUN_EVENT = pygame.USEREVENT + 1
pygame.time.set_timer(GENERATOR_SUN_EVENT, 2000)
GENERATOR_ZOMBIE_EVENT = pygame.USEREVENT + 2
pygame.time.set_timer(GENERATOR_ZOMBIE_EVENT, 5000)
while True:
clock.tick(10)
# 启动消息队列,获取消息并处理
for event in pygame.event.get():
if event.type == QUIT:
pygame.quit()
sys.exit()
if event.type == GENERATOR_SUN_EVENT:
sun = Sun(sunFlower.rect)
sunList.add(sun)
if event.type == GENERATOR_ZOMBIE_EVENT:
zombie = Zombie()
zombieList.add(zombie)
if event.type == MOUSEBUTTONDOWN:
mouse_pressed = pygame.mouse.get_pressed()
# 判断是否按下的事鼠标左键
if mouse_pressed[0]:
pos = pygame.mouse.get_pos()
for sun in sunList:
if sun.rect.collidepoint(pos):
# sunList.remove(sun)
sun.is_click = True
score = int(score) + 50
myfont = pygame.font.SysFont('arial', 30)
txtImg = myfont.render(str(score), True, (0, 0, 0))
screen.blit(backgroundImg, (0, 0))
screen.blit(sunbackImg, (250, 0))
screen.blit(txtImg, (300, 0))
# 屏幕显示动画效果
# screen.blit(peashooter.images[index % 13], peashooter.rect)
# screen.blit(sunFlower.images[index % 13], sunFlower.rect)
# screen.blit(wallnut.images[index % 13], wallnut.rect)
if index % 10 == 0:
bullet = Bullet(peashooter.rect, size)
spriteList.add(bullet)
# 遍历太阳花
spriteList.update(index)
spriteList.draw(screen)
sunList.update(index)
sunList.draw(screen)
zombieList.update(index)
zombieList.draw(screen)
# 遍历僵尸头部文字
for zombie in zombieList:
headStr = "哈哈"
yourfont = pygame.font.SysFont('simsunnsimsun', 30)
headpic = yourfont.render(headStr, True, (0, 0, 0))
screen.blit(headpic, (zombie.rect.left + 60, zombie.rect.top - 20))
index += 1
pygame.display.update()
太阳花奔向目标的同时,变小
import pygame
import random
# 创建类 继承精灵类
class Sun(pygame.sprite.Sprite):
# 创建函数
def __init__(self, rect):
super(Sun, self).__init__()
# 创建太阳花对象
self.image = pygame.image.load('material/images/Sun_1.png').convert_alpha()
# 创建动画
self.images = [pygame.image.load('material/images/Sun_{}.png'.format(i)) for i in range(1, 18)]
# 利用坐标函数获得左边
self.rect = self.images[0].get_rect()
# 定义变量 利用随机数使太阳花产生在上下50
offsetTop = random.randint(-50, 50)
# 定义变量 利用随机数使太阳花产生在左右50
offsetLeft = random.randint(-50, 50)
self.rect.top = rect.top + offsetTop
self.rect.left = rect.left + offsetLeft
self.is_click = False
self.times = 7
self.scale = 10
# 更新精灵的位置
def update(self, *args):
self.image = self.images[args[0] % len(self.images)]
if self.is_click:
self.rect.left -= (self.rect.left - 250) / self.times
self.rect.top -=(self.rect.top - 0) / self.times
self.image = pygame.transform.smoothscale(self.image, (self.rect.width // self.times * self.scale, self.rect.height // self.times * self.scale))
if self.scale > 1:
self.scale -= 1
if self.rect.left <= 250 and self.rect.top <= 30:
self.kill()
九、绘制顶部卡片
import pygame
from pygame.locals import *
import sys
from Bullet import Bullet
from Peashooter import Peashooter
from Sun import Sun
from SunFlower import SunFlower
from WallNut import WallNut
# 初始化pygame
from Zombie import Zombie
pygame.init()
for font in pygame.font.get_fonts():
print(font)
size = (1200, 600)
# 设置屏幕宽高
screen = pygame.display.set_mode(size)
# 设置屏幕标题
pygame.display.set_caption("植物大战僵尸")
backgroundImg = pygame.image.load('material/images/background1.jpg').convert_alpha()
sunbackImg = pygame.image.load('material/images/SeedBank.png').convert_alpha()
flower_seed = pygame.image.load("material/images/TwinSunflower.gif")
wallNut_seed = pygame.image.load("material/images/WallNut.gif")
peashooter_seed = pygame.image.load("material/images/Peashooter.gif")
score = '1000'
myfont = pygame.font.SysFont('arial', 20)
txtImg = myfont.render(score, True, (0, 0, 0))
peashooter = Peashooter()
sunFlower = SunFlower()
wallNut = WallNut()
# zombie = Zombie()
spriteList = pygame.sprite.Group()
spriteList.add(peashooter)
spriteList.add(sunFlower)
spriteList.add(wallNut)
# spriteList.add(zombie)
sunList = pygame.sprite.Group()
zombieList = pygame.sprite.Group()
index = 0
clock = pygame.time.Clock()
GENERATOR_SUN_EVENT = pygame.USEREVENT + 1
pygame.time.set_timer(GENERATOR_SUN_EVENT, 2000)
GENERATOR_ZOMBIE_EVENT = pygame.USEREVENT + 2
pygame.time.set_timer(GENERATOR_ZOMBIE_EVENT, 5000)
while True:
clock.tick(15)
# 启动消息队列,获取消息并处理
for event in pygame.event.get():
if event.type == QUIT:
pygame.quit()
sys.exit()
if event.type == GENERATOR_SUN_EVENT:
sun = Sun(sunFlower.rect)
sunList.add(sun)
if event.type == GENERATOR_ZOMBIE_EVENT:
zombie = Zombie()
zombieList.add(zombie)
if event.type == MOUSEBUTTONDOWN:
mouse_pressed = pygame.mouse.get_pressed()
# 判断是否按下的事鼠标左键
if mouse_pressed[0]:
pos = pygame.mouse.get_pos()
for sun in sunList:
if sun.rect.collidepoint(pos):
# sunList.remove(sun)
sun.is_click = True
score = int(score) + 50
myfont = pygame.font.SysFont('arial', 20)
txtImg = myfont.render(str(score), True, (0, 0, 0))
screen.blit(backgroundImg, (0, 0))
screen.blit(sunbackImg, (250, 0))
screen.blit(txtImg, (270, 60))
screen.blit(flower_seed, (330, 10))
screen.blit(wallNut_seed, (380, 10))
screen.blit(peashooter_seed, (430, 10))
if index % 10 == 0:
bullet = Bullet(peashooter.rect, size)
spriteList.add(bullet)
spriteList.update(index)
spriteList.draw(screen)
sunList.update(index)
sunList.draw(screen)
zombieList.update(index)
zombieList.draw(screen)
for zombie in zombieList:
headStr = '刘无敌'
yourfont = pygame.font.SysFont('simsunnsimsun', 30)
headpic = yourfont.render(headStr, True, (0, 0, 0))
screen.blit(headpic, (zombie.rect.left + 60, zombie.rect.top - 20))
index += 1
pygame.display.update()