查找
返回匹配特定键的元素数量
std::map::count
函数
size_type count( const Key& key ) const;
返回拥有关键比较等价于指定参数的元素数,因为此容器不允许重复故为 1 或 0。
参数
返回值
拥有比较等价于 key
的关键的元素数,对于 (1) 为 1 或 0。
复杂度
与容器大小成对数。
示例
cout << "countTest() begin" << endl;
map> map1{{1, "A"}, {2, "B"}, {3, "C"}, {4, "D"}, {5, "E"}};
cout << "map1.count(1): " << map1.count(1) << endl;
cout << "map1.count(6): " << map1.count(6) << endl;
cout << "countTest() end" << endl;
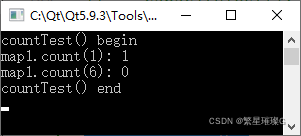
寻找带有特定键的元素
std::map::find
函数
iterator find( const Key& key ); (1)
const_iterator find( const Key& key ) const; (2)
1,2) 寻找键等于 key
的的元素。
参数
返回值
指向键等于 key
的元素的迭代器。若找不到这种元素,则返回尾后(见 end() )迭代器。
复杂度
与容器大小成对数。
示例
cout << "countTest() begin" << endl;
map> map1{{1, "A"}, {2, "B"}, {3, "C"}, {4, "D"}, {5, "E"}};
map::const_iterator it = map1.find(1);
cout << "map1.find(1): " << "key: " << (it == map1.end() ? "find no" : it->second) << endl;
auto it2 = map1.find(6);
cout << "map1.find(6): " << "key: " << (it2 == map1.end() ? "find no" : it2->second) << endl;
cout << "countTest() end" << endl;
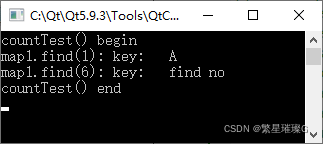
返回匹配特定键的元素范围
std::map::equal_range
函数
std::pair equal_range( const Key& key ); (1)
std::pair equal_range( const Key& key ) const; (2)
返回容器中所有拥有给定关键的元素范围。范围以二个迭代器定义,一个指向首个不小于 key
的元素,另一个指向首个大于 key
的元素。首个迭代器可以换用 lower_bound() 获得,而第二迭代器可换用 upper_bound() 获得。
1,2) 比较关键与 key
。
参数
key |
- |
要比较元素的关键值 |
x |
- |
能与 Key 比较的替用值 |
返回值
含一对定义所需范围的迭代器的 std::pair :第一个指向首个不小于 key
的元素,第二个指向首个大于 key
的元素。
若无元素不小于 key
,则将尾后(见 end() )迭代器作为第一元素返回。类似地,若无元素大于 key
,则将尾后迭代器作为第二元素返回。
复杂度
与容器大小成对数。
示例
cout << "equal_rangeTest() begin" << endl;
map> map1{{1, "A"}, {2, "B"}, {3, "C"}, {4, "D"}, {5, "E"}};
std::pair
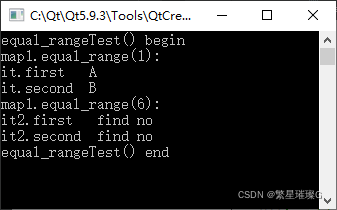
返回指向首个不小于给定键的元素的迭代器
std::map::lower_bound
函数
iterator lower_bound( const Key& key ); (1)
const_iterator lower_bound( const Key& key ) const; (1)
1) 返回指向首个不小于 key
的元素的迭代器。
参数
返回值
指向首个不小于 key
的元素的迭代器。若找不到这种元素,则返回尾后迭代器(见 end() )。
复杂度
与容器大小成对数。
示例
cout << "lower_boundTest() begin" << endl;
map> map1{{1, "A"}, {2, "B"}, {3, "C"}, {4, "D"}, {5, "E"}};
map::iterator it = map1.lower_bound(1);
cout << "map1.lower_bound(1): " << "key: " << (it == map1.end() ? "find no" : it->second) << endl;
auto it1 = map1.lower_bound(6);
cout << "map1.lower_bound(6): " << "key: " << (it1 == map1.end() ? "find no" : it1->second) << endl;
cout << "lower_boundTest() end" << endl;
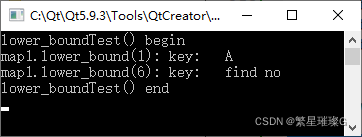
返回指向首个大于给定键的元素的迭代器
std::map::upper_bound
函数
iterator upper_bound( const Key& key ); (1)
const_iterator upper_bound( const Key& key ) const; (1)
1) 返回指向首个大于 key
的元素的迭代器。
参数
返回值
指向首个大于 key
的元素的迭代器。若找不到这种元素,则返回尾后(见 end() )迭代器。
复杂度
与容器大小成对数。
示例
cout << "upper_boundTest() begin" << endl;
map> map1{{1, "A"}, {2, "B"}, {3, "C"}, {4, "D"}, {5, "E"}};
map::iterator it = map1.upper_bound(1);
cout << "map1.upper_bound(1): " << "key: " << (it == map1.end() ? "find no" : it->second) << endl;
auto it1 = map1.upper_bound(6);
cout << "map1.upper_bound(6): " << "key: " << (it1 == map1.end() ? "find no" : it1->second) << endl;
cout << "upper_boundTest() end" << endl;
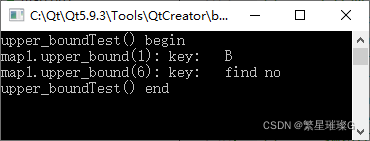
代码汇总
#include
#include