类的定义和使用
使用对象组织数据
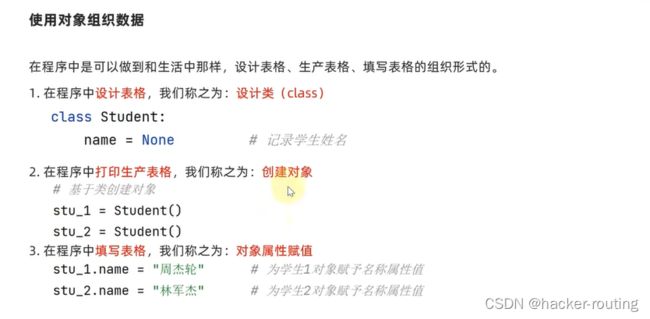
#设计一个类(设计一张登记表)
class student:
name = None
gender = None
nationality = None
native_place = None
age = None
#创建一个对象(打印一张登记表)
stu_1 = student()
#对象属性进行赋值(填写表单)
stu_1.name = "林俊杰"
stu_1.gender = "男"
stu_1.nationality = "中国"
stu_1.native_place = "山东省"
stu_1.age = "31"
#获取对象中记录的信息
print(stu_1.name)
print(stu_1.gender)
print(stu_1.nationality)
print(stu_1.native_place)
print(stu_1.age)
实例:定义闹钟并工作
import winsound
class Clock:
def __init__(self, id, price):
self.id = id
self.price = price
def ring(self):
winsound.Beep(2000, 200)
clock1 = Clock("001", 19.99)
print(f"闹钟的id是: {clock1.id}, 价格是: {clock1.price}")
clock1.ring()
clock2 = Clock("002", 29.99)
print(f"闹钟的id是: {clock2.id}, 价格是: {clock2.price}")
clock2.ring()
构造方法
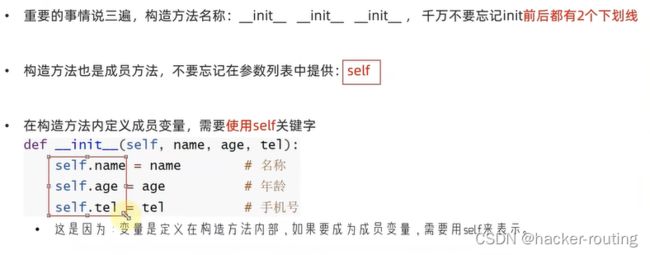
class student:
def __init__(self,name,age,tel):
self.name = name
self.age = age
self.tel = tel
print("student类创建了一个类对象")
stu = student("周杰伦",31,"10192837190")
print(stu.name)
print(stu.age)
print(stu.tel)
题目:
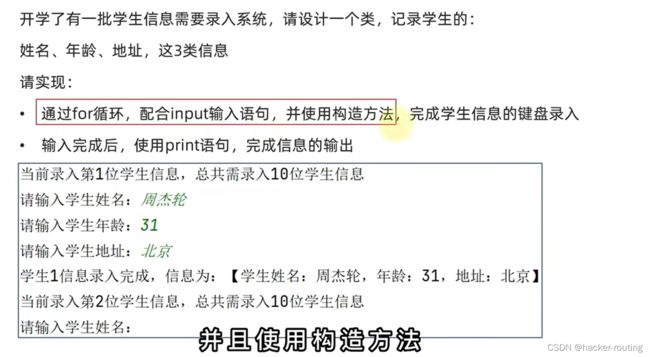
class Student:
def __init__(self, name, age, address):
self.name = name
self.age = age
self.address = address
# 创建一个空的学生表
students = []
# 通过for循环和input语句,逐个录入学生信息
for i in range(10):
print(f"请输入第{i+1}个学生的信息")
name = input("姓名:")
age = input("年龄:")
address = input("地址:")
# 实例化一个学生对象并添加到学生列表中
student = Student(name, age, address)
students.append(student)
# 使用print语句输出所有学生信息
print("学生信息如下:")
for i, student in enumerate(students):
print(f"第{i+1}个学生:")
print(f"姓名:{student.name}")
print(f"年龄:{student.age}")
print(f"地址:{student.address}")
print()
魔术方法
lt用于小于和大于
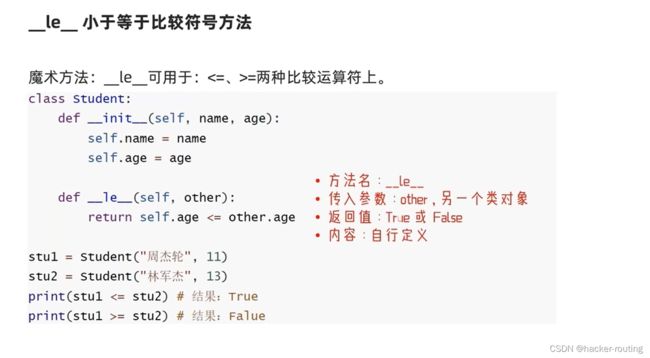
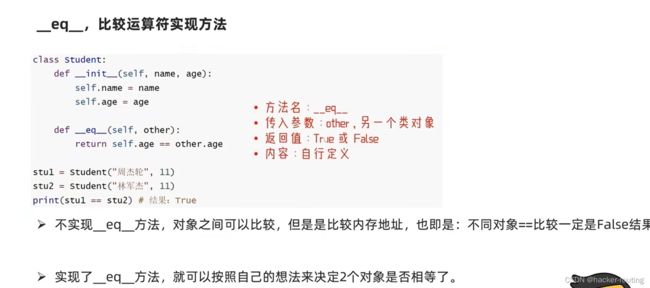
构造函数 __init__ 用于初始化学生的姓名和年龄属性。
__str__ 方法返回一个以适当格式表示学生对象的字符串。
__lt__ 方法是一个特殊方法,用于比较两个学生对象的年龄属性。在该方法中,我们使用 < 运算符来比较年龄的大小。
__le__ 方法是一个特殊方法,用于比较两个学生对象的姓名属性。在该方法中,我们使用 <= 运算符来比较姓名的大小。
__eq__ 方法是一个特殊方法,用于比较两个学生对象的姓名属性是否相等。在该方法中,我们使用 == 运算符来比较姓名的相等性。
class student:
def __init__(self, name, age):
self.name = name
self.age = age
def __str__(self):
return f"student的类对象:name{self.name},age{self.age}"
def __lt__(self, other):
return self.age < other.age
def __le__(self, other):
return self.name <= other.name
def __eq__(self, other):
return self.name == other.name
stu1 = student("周杰伦", 31)
stu2 = student("周杰伦", 31)
print(stu1 == stu2)
print(stu1 > stu2)
封装
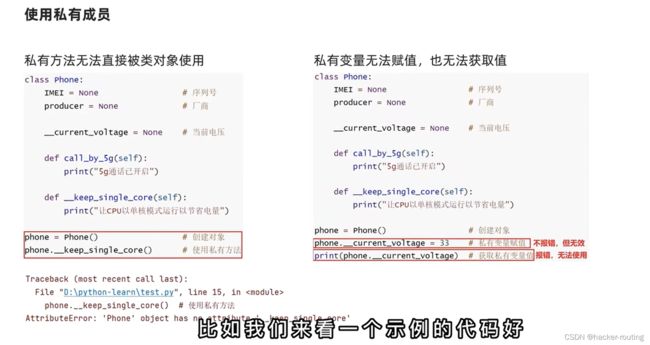
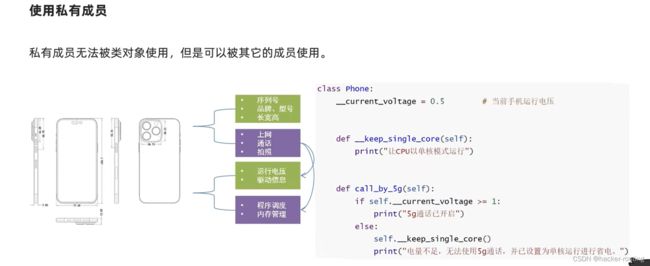
#定义一个类
from ast import If
class phone:
__current_voltage = 1 #当前手机电压
def __keep_single_core(self):
print("让cpu以单核模式运行")
def call_by_5g(self):
if self.__current_voltage >= 1:
print("5G模式已开启")
else:
self.__keep_single_core()
print("电量不足,无法使用5G通话,并设置为单核运行进行省电")
phone = phone()
phone.call_by_5g()
题目:
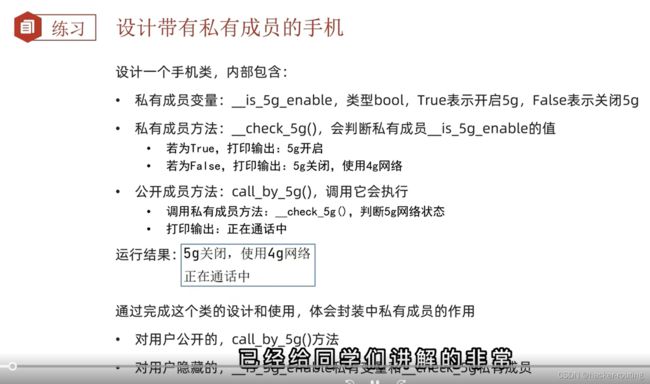
#设计一个类,用来描述手机
class phone:
#提供私有成员变量:__is_5g_enable
__is_5g_enable = False #5g关闭
#提供私有成员方法:__check_5g()
def __check_5g(self):
if self.__is_5g_enable:
print("5g开启")
else:
print("5g关闭,使用4g")
#提供公开成员方法:call_by_5g()
def call_by_5g(self):
self.__check_5g()
print("正在通话中")
phone = phone()
phone.call_by_5g()