文章目录
- 一【题目类别】
- 二【题目难度】
- 三【题目编号】
- 四【题目描述】
- 五【题目示例】
- 六【题目提示】
- 七【解题思路】
- 八【时间频度】
- 九【代码实现】
- 十【提交结果】
一【题目类别】
二【题目难度】
三【题目编号】
四【题目描述】
- 给定一个 m x n 二维字符网格 board 和一个单词(字符串)列表 words,找出所有同时在二维网格和字典中出现的单词。
- 单词必须按照字母顺序,通过 相邻的单元格 内的字母构成,其中“相邻”单元格是那些水平相邻或垂直相邻的单元格。同一个单元格内的字母在一个单词中不允许被重复使用。
五【题目示例】
- 示例 1:
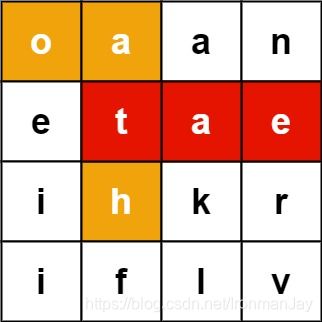
输入:board = [[“o”,“a”,“a”,“n”],[“e”,“t”,“a”,“e”],[“i”,“h”,“k”,“r”],[“i”,“f”,“l”,“v”]], words = [“oath”,“pea”,“eat”,“rain”]
输出:[“eat”,“oath”]
- 示例 2:
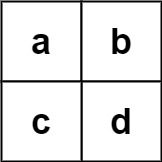
输入:board = [[“a”,“b”],[“c”,“d”]], words = [“abcb”]
输出:[]
六【题目提示】
- m = = b o a r d . l e n g t h m == board.length m==board.length
- n = = b o a r d [ i ] . l e n g t h n == board[i].length n==board[i].length
- 1 < = m , n < = 12 1 <= m, n <= 12 1<=m,n<=12
- b o a r d [ i ] [ j ] 是 一 个 小 写 英 文 字 母 board[i][j] 是一个小写英文字母 board[i][j]是一个小写英文字母
- 1 < = w o r d s . l e n g t h < = 3 ∗ 1 0 4 1 <= words.length <= 3 * 10^4 1<=words.length<=3∗104
- 1 < = w o r d s [ i ] . l e n g t h < = 10 1 <= words[i].length <= 10 1<=words[i].length<=10
- w o r d s [ i ] 由 小 写 英 文 字 母 组 成 words[i] 由小写英文字母组成 words[i]由小写英文字母组成
- w o r d s 中 的 所 有 字 符 串 互 不 相 同 words 中的所有字符串互不相同 words中的所有字符串互不相同
七【解题思路】
- 和79.单词搜索一样,就是加了一个for循环遍历每一个word和一个List存放结果
八【时间频度】
- 时间复杂度: O ( S ∗ M ∗ N ∗ 3 L ) O(S*M*N*3^L) O(S∗M∗N∗3L),其中 M 和 N 分别为行数和列数,L是单词的最大长度,S为二维网格中的单元格数
九【代码实现】
- Java语言版
package ToFlashBack;
import java.util.ArrayList;
import java.util.List;
public class p212_WordSearchII {
private static int[][] dires = {{0, 1}, {1, 0}, {0, -1}, {-1, 0}};
private int row, col;
private boolean hasFind;
private boolean[][] visited;
public static void main(String[] args) {
p212_WordSearchII p_212_wordSearchII = new p212_WordSearchII();
char[][] board = {
{'o', 'a', 'a', 'n'},
{'e', 't', 'a', 'e'},
{'i', 'h', 'k', 'r'},
{'i', 'f', 'l', 'v'}
};
String[] words = {"oath", "pea", "eat", "rain"};
List<String> res = p_212_wordSearchII.findWords(board, words);
System.out.println("res = " + res);
}
public List<String> findWords(char[][] board, String[] words) {
List list = new ArrayList();
for (int i = 0; i < words.length; i++) {
String word = words[i];
if (exist(board, word)) {
list.add(word);
}
}
return list;
}
public boolean exist(char[][] board, String word) {
row = board.length;
col = board[0].length;
hasFind = false;
if (row * col < word.length()) {
return false;
}
visited = new boolean[row][col];
char[] chars = word.toCharArray();
for (int i = 0; i < row; i++) {
for (int j = 0; j < col; j++) {
if (board[i][j] == chars[0]) {
backTrack(board, chars, 1, i, j);
if (hasFind) {
return true;
}
}
}
}
return false;
}
private void backTrack(char[][] board, char[] word, int curIndex, int x, int y) {
if (hasFind) {
return;
}
if (curIndex == word.length) {
hasFind = true;
return;
}
visited[x][y] = true;
for (int[] dire : dires) {
int newX = x + dire[0];
int newY = y + dire[1];
if (isIn(newX, newY) && !visited[newX][newY] && board[newX][newY] == word[curIndex]) {
backTrack(board, word, curIndex + 1, newX, newY);
}
}
visited[x][y] = false;
}
private boolean isIn(int x, int y) {
return x >= 0 && x < row && y >= 0 && y < col;
}
}
- C语言版
#include
#include
#include
#include
bool p212_WordSearchII_backTrack(char** board, int boardSize, int boardColSize, char* word, int wordIndex, bool** visited, int x, int y)
{
if (x < 0 || x >= boardSize || y < 0 || y >= boardColSize || visited[x][y] || board[x][y] != word[wordIndex])
{
return false;
}
if (wordIndex + 1 == strlen(word))
{
return true;
}
visited[x][y] = 1;
if (p212_WordSearchII_backTrack(board, boardSize, boardColSize, word, wordIndex + 1, visited, x + 1, y))
{
return true;
}
else if (p212_WordSearchII_backTrack(board, boardSize, boardColSize, word, wordIndex + 1, visited, x, y + 1))
{
return true;
}
else if (p212_WordSearchII_backTrack(board, boardSize, boardColSize, word, wordIndex + 1, visited, x - 1, y))
{
return true;
}
else if (p212_WordSearchII_backTrack(board, boardSize, boardColSize, word, wordIndex + 1, visited, x, y - 1))
{
return true;
}
else
{
visited[x][y] = 0;
return false;
}
}
bool p212_WordSearchII_exist(char** board, int boardSize, int boardColSize, char* word, int wordIndex, bool** visited)
{
for (int x = 0; x < boardSize; x++)
{
for (int y = 0; y < boardColSize; y++)
{
if (p212_WordSearchII_backTrack(board, boardSize, boardColSize, word, wordIndex, visited, x, y))
{
return true;
}
}
}
return false;
}
char ** findWords(char** board, int boardSize, int* boardColSize, char ** words, int wordsSize, int* returnSize)
{
char** res = (char**)malloc(sizeof(char*) * 1000);
int resIndex = 0;
int** visited = (int**)malloc(sizeof(int*)*boardSize);
for (int i = 0; i < wordsSize; i++)
{
for (int j = 0; j < boardSize; j++)
{
visited[j] = (int*)calloc(boardColSize[0], sizeof(int));
}
if (p212_WordSearchII_exist(board, boardSize, boardColSize[0], words[i], 0, visited))
{
res[resIndex++] = words[i];
}
}
*returnSize = resIndex;
return res;
}
十【提交结果】
-
Java语言版
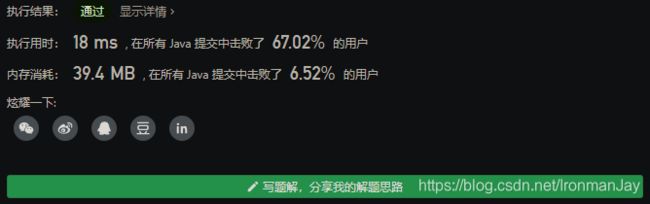
-
C语言版
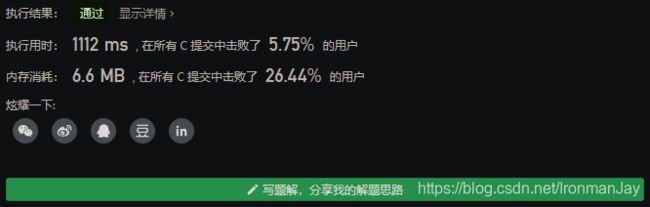