一、Redis入门
1.1、Redis简介
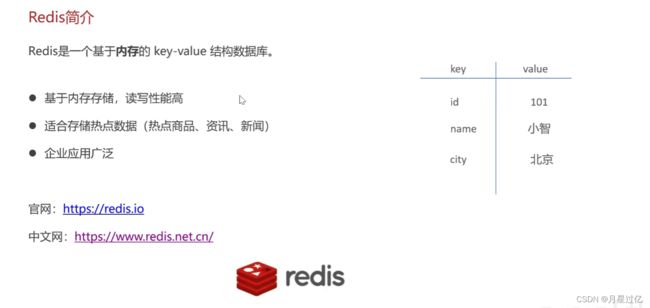
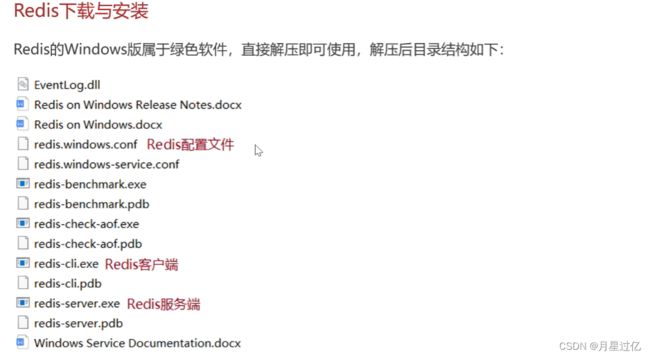
1.2 、redis启动
redis-server redis.windows.conf
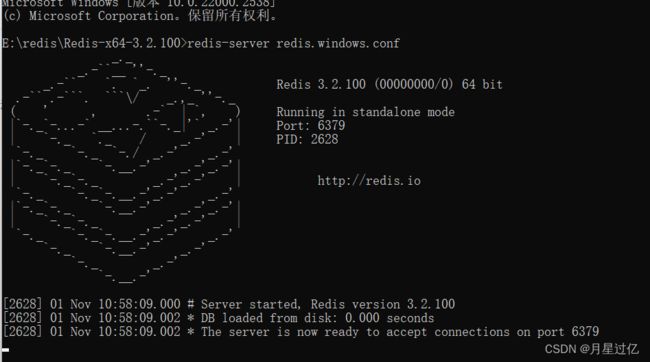
1.3、redis连接
redis-cli.exe -h localhost -p 6379 -a 731206
二、redis数据类型
2.1、redis的五种数据类型
2.2、redis的各种数据类型的特点
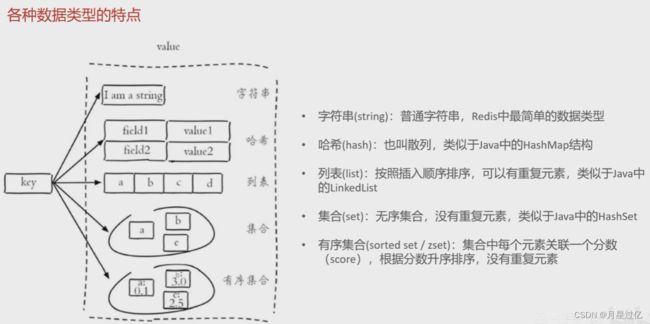
三、Redis的常用命令
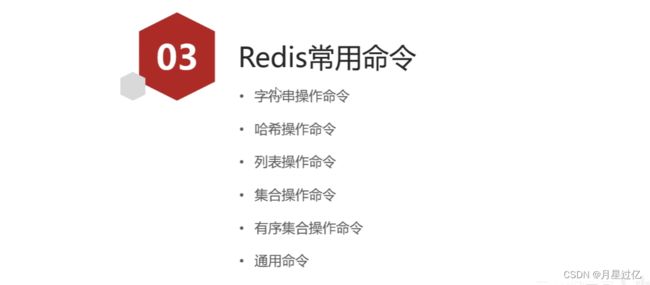
3.1、字符串操作命令
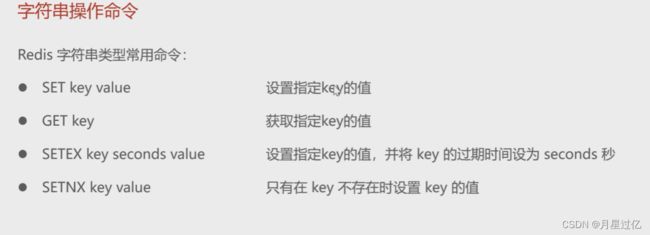
3.2、哈希操作命令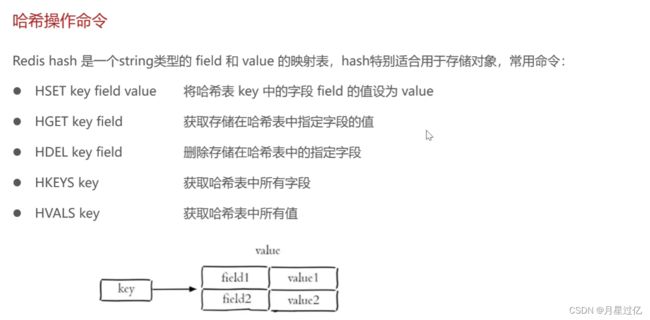
3.3、列表操作命令
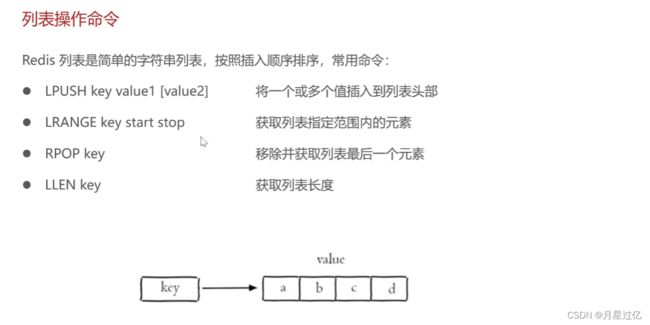
3.4、集合操作命令
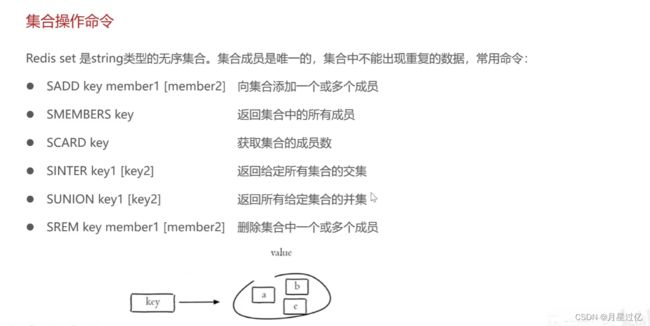
3.5、有序集合操作命令
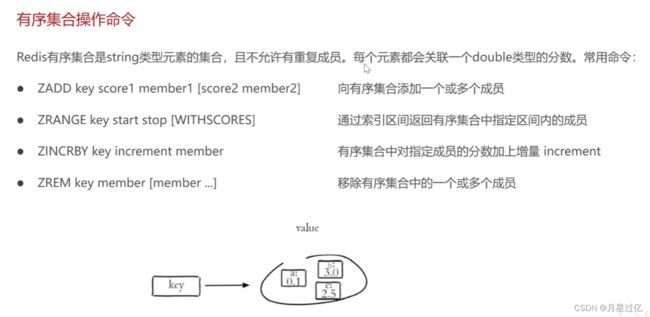
3.6、通用命令
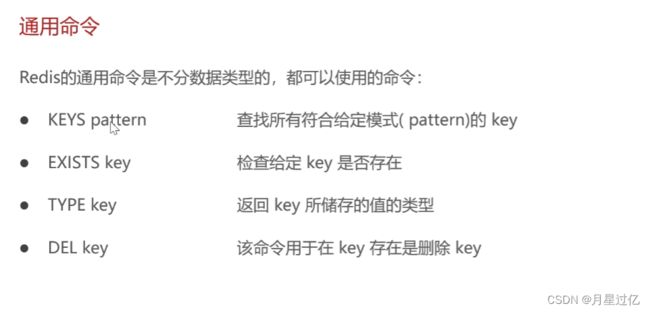
四、在Java程序中操作Redis
4.1、操作步骤说明
4.1.1、Redis的Java客户端
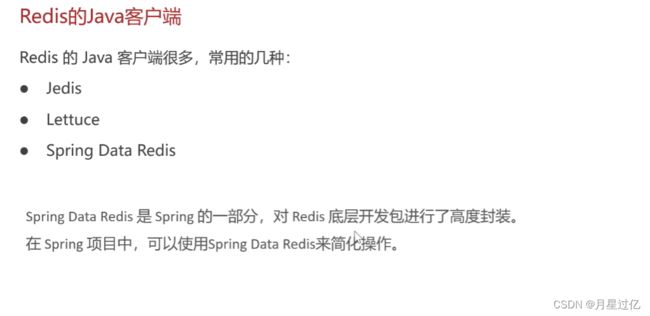
4.2.2、Spring Data Redis 使用方式
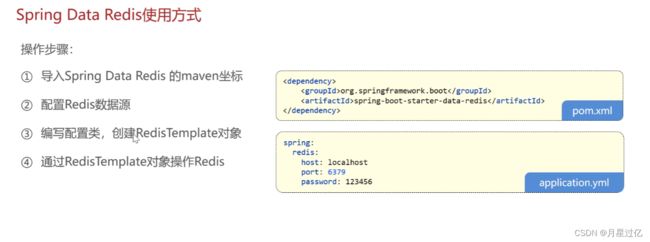
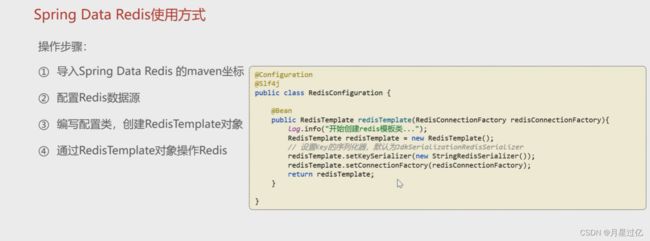
org.springframework.boot
spring-boot-starter-data-redis
import lombok.extern.slf4j.Slf4j;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.data.redis.RedisConnectionFailureException;
import org.springframework.data.redis.connection.RedisConnectionFactory;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.data.redis.serializer.StringRedisSerializer;
@Configuration
@Slf4j
public class RedisConfiguration {
@Bean
public RedisTemplate redisTemplate(RedisConnectionFactory redisConnectionFactory){
log.info ( "开始创建redis模板对象" );
RedisTemplate redisTemplate = new RedisTemplate ();
//设置Redis的连接工厂对象
redisTemplate.setConnectionFactory ( redisConnectionFactory );
//设置Redis key的序列化器
redisTemplate.setKeySerializer ( new StringRedisSerializer () );
return redisTemplate;
}
}
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.data.redis.connection.DataType;
import org.springframework.data.redis.core.*;
import java.util.List;
import java.util.Set;
import java.util.concurrent.TimeUnit;
@SpringBootTest
public class SpringDataRedisTest {
@Autowired
private RedisTemplate redisTemplate;
@Test
public void testRedisTemplate(){
System.out.println ( redisTemplate );
//操作字符串对象
ValueOperations valueOperations = redisTemplate.opsForValue ();
//操作哈希
HashOperations hashOperations = redisTemplate.opsForHash ();
//操作列表
ListOperations listOperations = redisTemplate.opsForList ();
//操作无序集合
SetOperations setOperations = redisTemplate.opsForSet ();
//操作有序集合
ZSetOperations zSetOperations = redisTemplate.opsForZSet ();
}
/**
* 操作字符串类型的数据
*/
@Test
public void testString(){
//set get setex setnx
redisTemplate.opsForValue ().set ( "city","北京" );
String city = (String) redisTemplate.opsForValue ().get ( "city" );
System.out.println (city);
redisTemplate.opsForValue ().set ( "code","1234",3, TimeUnit.MINUTES );
redisTemplate.opsForValue ().setIfAbsent ( "lock",1 );//setex
redisTemplate.opsForValue ().setIfAbsent ( "lock",2 );
}
/**
* 操作哈希类数据
*/
@Test
public void testHash(){
//hset hget hdel hkeys hvals
HashOperations hashOperations = redisTemplate.opsForHash ();
hashOperations.put ( "100","name","tom" );
hashOperations.put ( "100","age","20" );
String name = (String) hashOperations.get ( "100", "name" );
System.out.println ( name );
Set keys = hashOperations.keys ( "100" );
System.out.println ( keys );
List values = hashOperations.values ( "100" );
System.out.println ( values );
hashOperations.delete ( "100","age" );
}
/**
* 操作列表类型数据
*/
@Test
public void testList(){
//lpush lrange rpop llen
ListOperations listOperations = redisTemplate.opsForList ();
listOperations.leftPushAll ( "mylist","a","b","c" );
listOperations.leftPush ( "mylist","d" );
List mylist = listOperations.range ( "mylist", 0, -1 );
System.out.println ( mylist );
listOperations.rightPop ( "mylist" );
Long size = listOperations.size ( "mylist" );
System.out.println ( size );
}
/**
* 无序集合类型数据
*/
@Test
public void testSet(){
//sadd smember scard sinsert sunion srem
SetOperations setOperations = redisTemplate.opsForSet ();
setOperations.add ( "set1","a","b","c","d" );
setOperations.add ( "set2","a","b","x","y" );
Set members = setOperations.members ( "set1" );
System.out.println ( members );
Long size = setOperations.size ( "set1" );
System.out.println ( size );
Set intersect = setOperations.intersect ( "set1", "set2" );
System.out.println ( intersect );
Set union = setOperations.union ( "set1", "set2" );
System.out.println ( union );
setOperations.remove ( "set1","a","b" );
}
/**
* 操作有序集合
*/
@Test
public void testZset(){
//zadd zrange zincrby zrem
ZSetOperations zSetOperations = redisTemplate.opsForZSet ();
zSetOperations.add ( "zset1","a",10 );
zSetOperations.add ( "zset1","b",12 );
zSetOperations.add( "zest1","c",9);
Set zset1 = zSetOperations.range ( "zset1", 0, -1 );
System.out.println ( zset1 );
zSetOperations.incrementScore ( "zset1","c",10 );
zSetOperations.remove ( "zset1","a","b" );
}
/**
* 通用命令
*/
@Test
public void testCommom(){
//keys exists del
Set keys = redisTemplate.keys ( "*" );
System.out.println ( keys );
Boolean name = redisTemplate.hasKey ( "name" );
Boolean set1 = redisTemplate.hasKey ( "set1" );
for (Object key : keys) {
DataType type = redisTemplate.type ( key );
System.out.println ( type.name () );
}
redisTemplate.delete ( "mylist" );
}
}
五、店铺营业状态设置
5.1、需求分析设计
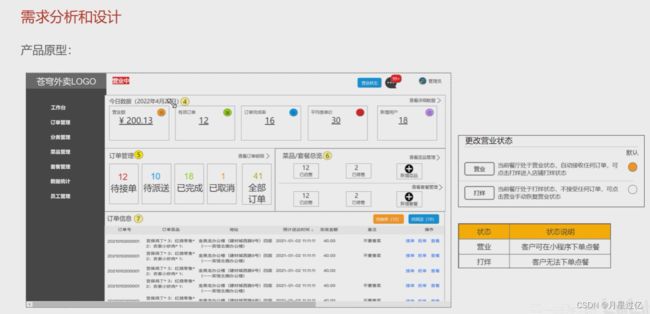
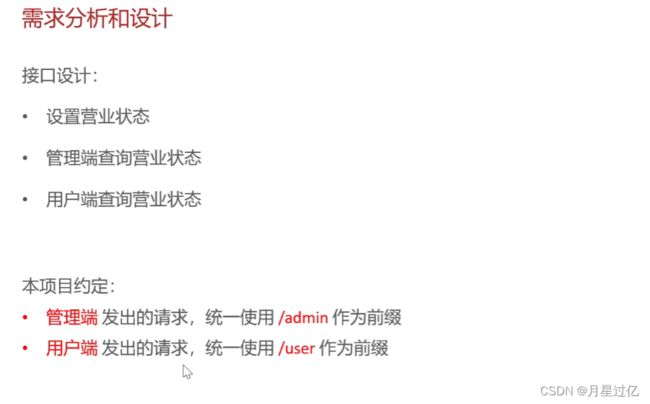
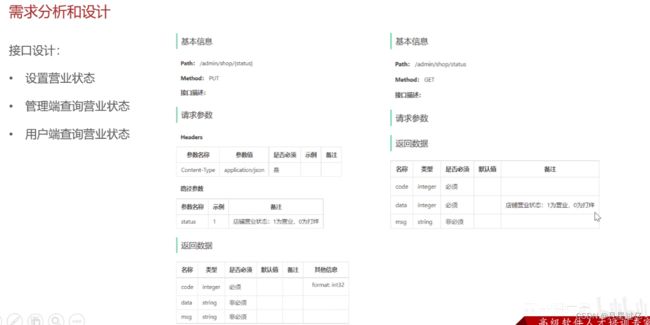
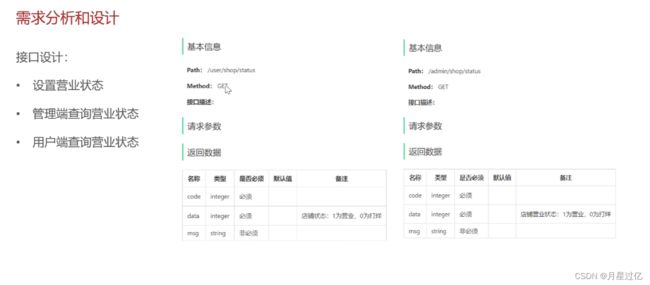
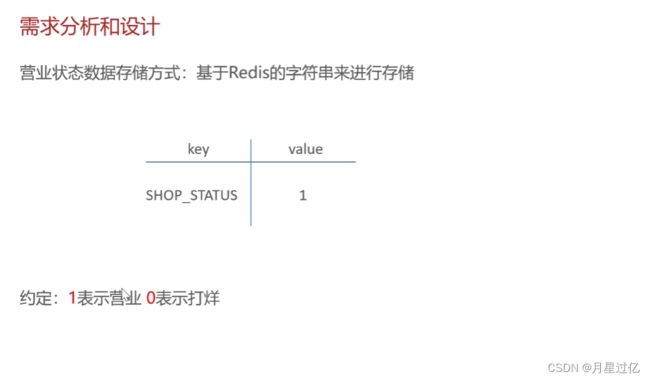
5.2、代码开发
import com.sky.result.Result;
import io.swagger.annotations.Api;
import io.swagger.annotations.ApiOperation;
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.web.bind.annotation.*;
@RestController("adminShopController")
@RequestMapping("/admin/shop")
@Api(tags = "店铺相关接口")
@Slf4j
public class ShopController {
public static final String KEY="SHOP_STATUS";
@Autowired
private RedisTemplate redisTemplate;
@ApiOperation ( "设置店铺的营业状态" )
@PutMapping("/{status}")
public Result setStatus(@PathVariable Integer status){
log.info ( "设置店铺的营业状态,{}",status==1?"营业中":"打烊中" );
redisTemplate.opsForValue ().set ( KEY,status );
return Result.success ();
}
/**
* 获取店铺营业状态
* @return
*/
@GetMapping("/status")
@ApiOperation ( "获取店铺营业状态" )
public Result getStatus(){
Integer shop_status = (Integer) redisTemplate.opsForValue ().get ( KEY );
log.info ( "获取店铺营业状态,{}",shop_status==1?"营业中":"打烊中");
return Result.success (shop_status);
}
}