安装maatwebsite/excel
composer require maatwebsite/excel
$grid->tools(function (Grid\Tools $tools) {
// excle 导入
$tools->append(new ExcelAdd());
});
2.创建按钮文件
;
use Throwable;
use Encore\Admin\Admin;
use App\Imports\DataExcel;
use Encore\Admin\Actions\Action;
use Encore\Admin\Actions\Response;
use Maatwebsite\Excel\Facades\Excel;
use Maatwebsite\Excel\Validators\ValidationException;
class ExcelAdd extends Action
{
protected $selector = '.import-template';
public function handle()
{
try {
Excel::import(new DataExcel(time()), request()->file('file'));
} catch (ValidationException $validationException) {
return Response::withException($validationException);
} catch (Throwable $throwable) {
$this->response()->status = false;
return $this->response()->swal()->error($throwable->getMessage());
}
return $this->response()->success('上传成功')->refresh();
}
// 按钮样式
public function html()
{
return <<class="btn btn-sm btn-default import-template">上传简历</a>
HTML;
}
// 上传表单
public function form()
{
$this->file('file', '上传简历')->rules('required', ['required' => '文件不能为空']);
}
/**
* @return string
* 上传效果
*/
public function handleActionPromise()
{
$resolve = <<<'SCRIPT'
var actionResolverss = function (data) {
$('.modal-footer').show()
$('.tips').remove()
var response = data[0];
var target = data[1];
if (typeof response !== 'object') {
return $.admin.swal({type: 'error', title: 'Oops!'});
}
var then = function (then) {
if (then.action == 'refresh') {
$.admin.reload();
}
if (then.action == 'download') {
window.open(then.value, '_blank');
}
if (then.action == 'redirect') {
$.admin.redirect(then.value);
}
};
if (typeof response.html === 'string') {
target.html(response.html);
}
if (typeof response.swal === 'object') {
$.admin.swal(response.swal);
}
if (typeof response.toastr === 'object') {
$.admin.toastr[response.toastr.type](response.toastr.content, '', response.toastr.options);
}
if (response.then) {
then(response.then);
}
};
var actionCatcherss = function (request) {
$('.modal-footer').show()
$('.tips').remove()
if (request && typeof request.responseJSON === 'object') {
$.admin.toastr.error(request.responseJSON.message, '', {positionClass:"toast-bottom-center", timeOut: 10000}).css("width","500px")
}
};
SCRIPT;
Admin::script($resolve);
return <<<'SCRIPT'
$('.modal-footer').hide()
let html = `class='tips' style='color: red;font-size: 18px;'>导入时间取决于数据量,请耐心等待结果不要关闭窗口!
"data:image/gif;base64,R0lGODlhEAAQAPQAAP///1VVVfr6+np6eqysrFhYWG5ubuPj48TExGNjY6Ojo5iYmOzs7Lq6utjY2ISEhI6OjgAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAACH/C05FVFNDQVBFMi4wAwEAAAAh/hpDcmVhdGVkIHdpdGggYWpheGxvYWQuaW5mbwAh+QQJCgAAACwAAAAAEAAQAAAFUCAgjmRpnqUwFGwhKoRgqq2YFMaRGjWA8AbZiIBbjQQ8AmmFUJEQhQGJhaKOrCksgEla+KIkYvC6SJKQOISoNSYdeIk1ayA8ExTyeR3F749CACH5BAkKAAAALAAAAAAQABAAAAVoICCKR9KMaCoaxeCoqEAkRX3AwMHWxQIIjJSAZWgUEgzBwCBAEQpMwIDwY1FHgwJCtOW2UDWYIDyqNVVkUbYr6CK+o2eUMKgWrqKhj0FrEM8jQQALPFA3MAc8CQSAMA5ZBjgqDQmHIyEAIfkECQoAAAAsAAAAABAAEAAABWAgII4j85Ao2hRIKgrEUBQJLaSHMe8zgQo6Q8sxS7RIhILhBkgumCTZsXkACBC+0cwF2GoLLoFXREDcDlkAojBICRaFLDCOQtQKjmsQSubtDFU/NXcDBHwkaw1cKQ8MiyEAIfkECQoAAAAsAAAAABAAEAAABVIgII5kaZ6AIJQCMRTFQKiDQx4GrBfGa4uCnAEhQuRgPwCBtwK+kCNFgjh6QlFYgGO7baJ2CxIioSDpwqNggWCGDVVGphly3BkOpXDrKfNm/4AhACH5BAkKAAAALAAAAAAQABAAAAVgICCOZGmeqEAMRTEQwskYbV0Yx7kYSIzQhtgoBxCKBDQCIOcoLBimRiFhSABYU5gIgW01pLUBYkRItAYAqrlhYiwKjiWAcDMWY8QjsCf4DewiBzQ2N1AmKlgvgCiMjSQhACH5BAkKAAAALAAAAAAQABAAAAVfICCOZGmeqEgUxUAIpkA0AMKyxkEiSZEIsJqhYAg+boUFSTAkiBiNHks3sg1ILAfBiS10gyqCg0UaFBCkwy3RYKiIYMAC+RAxiQgYsJdAjw5DN2gILzEEZgVcKYuMJiEAOwAAAAAAAAAAAA=="><\/div>`
$('.modal-header').append(html)
process.then(actionResolverss).catch(actionCatcherss);
SCRIPT;
}
}
3、获取 excel 中第一个 文件 sheet 中的信息
namespace App\Imports;
use Maatwebsite\Excel\Concerns\WithMultipleSheets;
class DataExcel implements WithMultipleSheets
{
private $round;
public function __construct(int $round)
{
$this->round = $round;
}
public function sheets(): array
{
return [
new FirstSheetImport($this->round),
];
}
}
4、获取信息进行导入数据库
;
use App\Models\Data;
use App\Models\Data as DataModel;
use Illuminate\Support\Collection;
use Illuminate\Database\Eloquent\Model;
use Maatwebsite\Excel\Concerns\ToModel;
use Maatwebsite\Excel\Concerns\ToCollection;
use Maatwebsite\Excel\Concerns\WithHeadingRow;
use Maatwebsite\Excel\Concerns\WithBatchInserts;
use Maatwebsite\Excel\Concerns\WithChunkReading;
use Maatwebsite\Excel\Imports\HeadingRowFormatter;
HeadingRowFormatter::
default('none');
class FirstSheetImport implements ToCollection, WithBatchInserts, WithChunkReading, WithHeadingRow, ToModel
{
private $round;
public function __construct(int $round)
{
$this->round = $round;
}
/**
* @param array $row
*
* @return Model|Model[]|null
*/
public function model(array $row)
{
// 断数据是否
$user = Data::where('mobile', '=', $row['手机'])->first();
if ($user) {
// 存在返回 null
return null;
}
// 数据库对应的字段
return new DataModel([
'name' => $row['姓名'],
'gender' => $row['性别'],
]);
}
public function collection(Collection $rows)
{
//
}
//批量导入1000条
public function batchSize(): int
{
return 1000;
}
//以1000条数据基准切割数据
public function chunkSize(): int
{
return 1000;
}
}
注意需要在models加上
protected $fillable = ['img', 'content','static','username'];
这段来源
Dcat admin
Dcat Admin是一个基于laravel-admin二次开发而成的后台系统构建工具,只需极少的代码即可快速构建出一个功能完善的高颜值后台系统。支持页面一键生成CURD代码,内置丰富的后台常用组件,开箱即用,让开发者告别冗杂的HTML代码,对后端开发者非常友好。
效果图

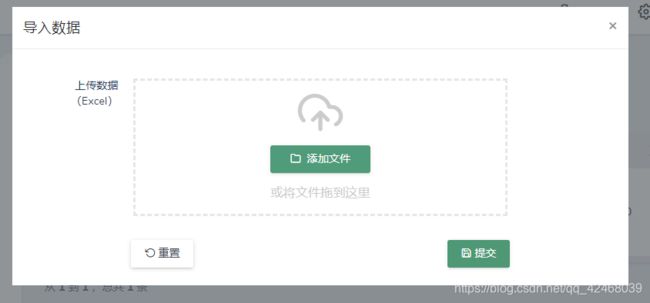
- 创建按钮
$grid->tools(function (Grid\Tools $tools) {
// excle 导入
$tools->append(new Reast());
});
2.app/admin/actions/grid 下创建 Reast.php
namespace App\Admin\Actions\Grid;
use App\Admin\Actions\Form\Import;
use Dcat\Admin\Admin;
use Dcat\Admin\Actions\Response;
use Dcat\Admin\Grid\Tools\AbstractTool;
use Dcat\Admin\Traits\HasPermissions;
use Illuminate\Contracts\Auth\Authenticatable;
use Illuminate\Database\Eloquent\Model;
use Illuminate\Http\Request;
class Reast extends AbstractTool
{
/**
* @return string
*/
protected $title = 'Title';
public function render()
{
$id = "reset-pwd-{$this->getKey()}";
// 模态窗
$this->modal($id);
return <<<HTML
">
HTML;
}
protected function modal($id)
{
$form = new Import();
Admin::script('Dcat.onPjaxComplete(function () {
$(".modal-backdrop").remove();
$("body").removeClass("modal-open");
}, true)');
// 通过 Admin::html 方法设置模态窗HTML
Admin::html(
<<<HTML
<div class="modal fade" id="{$id}">
<div class="modal-dialog modal-lg" role="document">
<div class="modal-content">
<div class="modal-header">
<h4 class="modal-title">导入数据</h4>
<button type="button" class="close" data-dismiss="modal" aria-label="Close"><span aria-hidden="true">×</span></button>
</div>
<div class="modal-body">
{$form->render()}
</div>
</div>
</div>
</div>
HTML
);
}
/**
* @param Model|Authenticatable|HasPermissions|null $user
*
* @return bool
*/
protected function authorize($user): bool
{
return true;
}
/**
* @return array
*/
protected function parameters()
{
return [];
}
}
3.在Actions/form下创建Import.php
namespace App\Admin\Actions\Form;
use Dcat\Admin\Models\Administrator;
use Dcat\Admin\Widgets\Form;
use Symfony\Component\HttpFoundation\Response;
use App\Imports\DataExcel;
use Maatwebsite\Excel\Facades\Excel;
use Maatwebsite\Excel\Validators\ValidationException;
class Import extends Form
{
public function handle(array $input)
{
$file = env('APP_URL').'/upload/'.$input['file'];
try {
Excel::import(new DataExcel(time()), $input['file'],'public');
//dcat-2.0版本写法
return $this->response()
->success('导入成功')
->redirect('/');
//dcat-1.7
//return $this->success('导入成功');
} catch (ValidationException $validationException) {
return Response::withException($validationException);
} catch (Throwable $throwable) {
//dcat 2.0写法
$this->response()->status = false;
return $this->response()->error('上传失败')->refresh();
//dcat 1.7
//return $this->error('上传失败')->refresh();
}
}
public function form()
{
$this->file('file', '上传数据(Excel)')->rules('required', ['required' => '文件不能为空']);
}
}
再重复上一标题下的3.4方法