Modbus 设备(利用 slave 模拟)
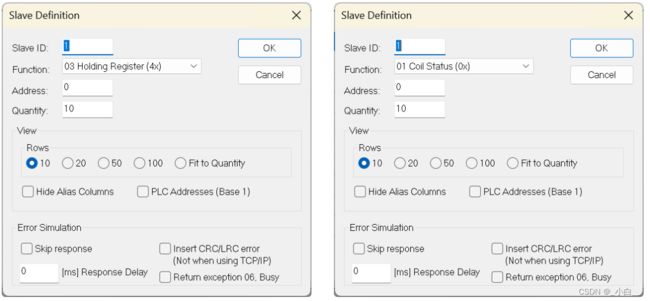
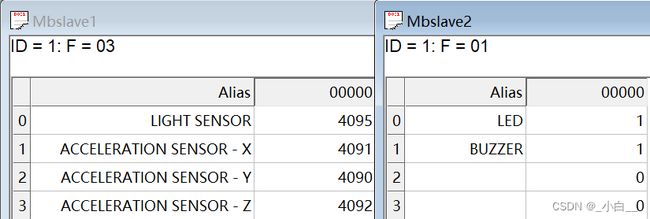
Modbus 采集程序
client.c
#include "client.h"
modbus_t *ctx;
key_t key_shm, key_msg;
int shmid, msgid;
struct shm *shm0;
struct msgbuf msg0;
void *collector(void *arg) {
struct shm *p = (struct shm *)arg;
while (1){
sleep(1);
if (modbus_read_registers(ctx, 0, 4, p->buf) < 0) {
perror("Failed to modbus_read_registers");
return NULL;
}
printf("LIGHT SENSOR: %d\n", p->buf[0]);
printf("ACCELERATION SENSOR-X: %d\n", p->buf[1]);
printf("ACCELERATION SENSOR-Y: %d\n", p->buf[2]);
printf("ACCELERATION SENSOR-Z: %d\n\n", p->buf[3]);
}
pthread_exit(0);
}
void *control(void *arg) {
while (1){
msgrcv(msgid, &msg0, sizeof(msg0)-sizeof(long), 250, 0);
printf("LED: %c\n", msg0.ctl[0]);
printf("BUZZER: %c\n\n", msg0.ctl[1]);
modbus_write_bit(ctx, msg0.ctl[0]-48, msg0.ctl[1]-48);
}
pthread_exit(0);
}
int main(int argc, char const *argv[])
{
if (argc != 3) {
printf("Please input %s . \n", argv[0]);
return -1;
}
init_modbus(&ctx, argv[1], atoi(argv[2]));
shm0 = init_shared_memory(&key_shm, &shmid);
printf("The key(shm): %#x\n", key_shm);
printf("The shmid: %d\n", shmid);
init_msg_queue(&key_msg, &msgid);
msg0.mtype = 250;
printf("The key(msg): %#x\n", key_msg);
printf("The msgid: %d\n", msgid);
pthread_t tid1, tid2;
if (pthread_create(&tid1, NULL, collector, shm0)) {
perror("Failed to create a thread named collector");
return -1;
}
if (pthread_create(&tid2, NULL, control, msg0.ctl)) {
perror("Failed to create a thread named input");
return -1;
}
pthread_join(tid1, NULL);
pthread_join(tid2, NULL);
modbus_close(ctx);
modbus_free(ctx);
return 0;
}
client.h
#ifndef __CLIENT_H_
#define __CLIENT_H_
#include
#include
#include
#include
#include
#include
#include
#include
#include
#include
#include
#define SHM_SIZE 64
struct shm {
uint16_t buf[4];
};
struct msgbuf {
long mtype;
uint8_t ctl[2];
};
int init_modbus(modbus_t **, const char *, int);
struct shm *init_shared_memory(key_t *, int *);
int init_msg_queue(key_t *, int *);
#endif
func.c
#include "client.h"
int init_modbus(modbus_t **ctx, const char *ip, int port) {
*ctx = modbus_new_tcp(ip, port);
if (*ctx == NULL) {
perror("Failed to modbus_new_tcp");
return -1;
}
if (modbus_set_slave(*ctx, 1) < 0) {
perror("Failed to modbus_set_slave");
return -2;
}
if (modbus_connect(*ctx) < 0) {
perror("Failed to modbus_connect");
return -3;
}
return 0;
}
struct shm *init_shared_memory(key_t *key, int *shmid) {
*key = ftok("./client.c", 'v');
if (key < 0) {
perror("Failed to ftok");
return NULL;
}
*shmid = shmget(*key, SHM_SIZE, IPC_CREAT | IPC_EXCL | 0666);
if (*shmid < 0) {
if (errno == EEXIST)
*shmid = shmget(*key, SHM_SIZE, 0666);
else {
perror("Failed to shmget");
return NULL;
}
}
struct shm *p = shmat(*shmid, NULL, 0);
if (p == (void *)-1) {
perror("Failed to shmat");
return NULL;
}
return p;
}
int init_msg_queue(key_t *key, int *msgid) {
*key = ftok("./client.h", 'v');
if (*key < 0){
perror("Failed to ftok");
return -4;
}
*msgid = msgget(*key, IPC_CREAT | IPC_EXCL | 0666);
if (*