吃金币案例
创建金币逻辑
- 之前的MyActor_One.cpp,直接添加几个资源拿着就用
UPROPERTY(VisibleAnywhere, BlueprintReadOnly)
class UStaticMeshComponent* StaticMesh;
UPROPERTY(VisibleAnywhere, BlueprintReadWrite)
class USphereComponent* TriggerVolume;
UPROPERTY(EditAnywhere, BlueprintReadWrite, Category = "Interoperable|Particle")
class UParticleSystemComponent* ParticleEffectsComponent;
UPROPERTY(EditAnywhere, BlueprintReadWrite, Category = "Interoperable|Particle")
class UParticleSystem* Particle;
UPROPERTY(EditAnywhere, BlueprintReadWrite, Category = "Interoperable|Sounds")
class USoundCue* Sound;
UPROPERTY(EditAnywhere, BlueprintReadWrite, Category = "Interoperable Item|Properties")
bool bRotate = true;
UPROPERTY(EditAnywhere, BlueprintReadWrite, Category = "Interoperable Item|Properties")
float RotationRate = 45.f;
#include "MyActor_One.h"
#include "Engine/Engine.h"
#include "UObject/ConstructorHelpers.h"
#include "Components/StaticMeshComponent.h"
#include "Components/SphereComponent.h"
#include "Particles/ParticleSystemComponent.h"
#include "MyObjectUE5/MyCharacters/MyCharacter.h"
#include "Kismet/GamePlayStatics.h"
#include "Sound/SoundCue.h"
AMyActor_One::AMyActor_One()
{
PrimaryActorTick.bCanEverTick = true;
StaticMesh = CreateDefaultSubobject<UStaticMeshComponent>(TEXT("StaticMesh"));
RootComponent = StaticMesh;
TriggerVolume = CreateDefaultSubobject<USphereComponent>(TEXT("TriggerVolume"));
TriggerVolume->SetupAttachment(GetRootComponent());
ParticleEffectsComponent = CreateDefaultSubobject<UParticleSystemComponent>(TEXT("ParticleEffects"));
ParticleEffectsComponent->SetupAttachment(GetRootComponent());
TriggerVolume->SetCollisionEnabled(ECollisionEnabled::QueryOnly);
TriggerVolume->SetCollisionObjectType(ECollisionChannel::ECC_WorldStatic);
TriggerVolume->SetCollisionResponseToAllChannels(ECollisionResponse::ECR_Ignore);
TriggerVolume->SetCollisionResponseToChannel(ECollisionChannel::ECC_Pawn, ECollisionResponse::ECR_Overlap);
}
void AMyActor_One::BeginPlay()
{
Super::BeginPlay();
TriggerVolume->OnComponentBeginOverlap.AddDynamic(this, &AMyActor_One::OnOverlapBegin);
TriggerVolume->OnComponentEndOverlap.AddDynamic(this, &AMyActor_One::OnOverlapEnd);
}
void AMyActor_One::OnOverlapBegin(UPrimitiveComponent* OverlappedComponent, AActor* OtherActor, UPrimitiveComponent* OtherComp, int32 OtherBodyIndex, bool bFromSweep, const FHitResult& SweepResult)
{
if (OtherActor)
{
AMyCharacter* Player = Cast<AMyCharacter>(OtherActor);
if (Player)
{
if (Particle)
{
UGameplayStatics::SpawnEmitterAtLocation(this, Particle, GetActorLocation(), FRotator(0.f), true);
}
if (Sound)
{
UGameplayStatics::PlaySound2D(this, Sound);
}
Destroy();
}
}
}
void AMyActor_One::OnOverlapEnd(UPrimitiveComponent* OverlappedComponent, AActor* OtherActor, UPrimitiveComponent* OtherComp, int32 OtherBodyIndex)
{
}
void AMyActor_One::Tick(float DeltaTime)
{
Super::Tick(DeltaTime);
if (bRotate)
{
FRotator rotator = GetActorRotation();
rotator.Yaw += RotationRate * DeltaTime;
SetActorRotation(rotator);
}
}
角色吃到金币逻辑
UPROPERTY(EditAnywhere, BlueprintReadOnly, Category = "Coin")
int Coin = 0;
- 将金币的Actor类碰撞处理函数测试一下是否能捡到金币
void AMyActor_One::OnOverlapBegin(UPrimitiveComponent* OverlappedComponent, AActor* OtherActor, UPrimitiveComponent* OtherComp, int32 OtherBodyIndex, bool bFromSweep, const FHitResult& SweepResult)
{
if (OtherActor)
{
AMyCharacter* Player = Cast<AMyCharacter>(OtherActor);
if (Player)
{
if (Particle)
{
UGameplayStatics::SpawnEmitterAtLocation(this, Particle, GetActorLocation(), FRotator(0.f), true);
}
if (Sound)
{
UGameplayStatics::PlaySound2D(this, Sound);
}
Player->Coin++;
GEngine->AddOnScreenDebugMessage(2, 10, FColor::Red, FString::Printf(TEXT("%d"), Player->Coin));
Destroy();
}
}
}
UE5中的UI控件
- 新建一个UI控件蓝图后,UE5中的控件蓝图默认是什么都没有要添加一个画布面板之后才能添加控件在蓝图上
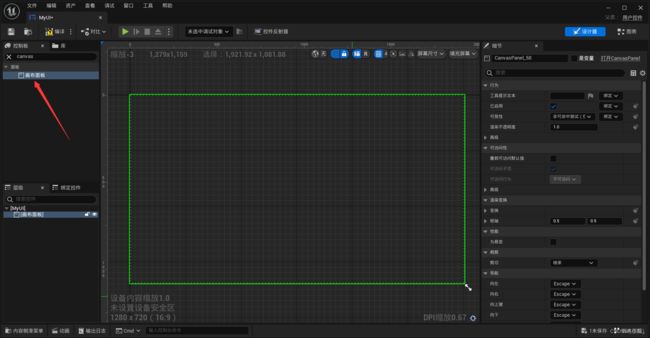
- 获取控件就得把控件提升为变量就可以在蓝图中调用了
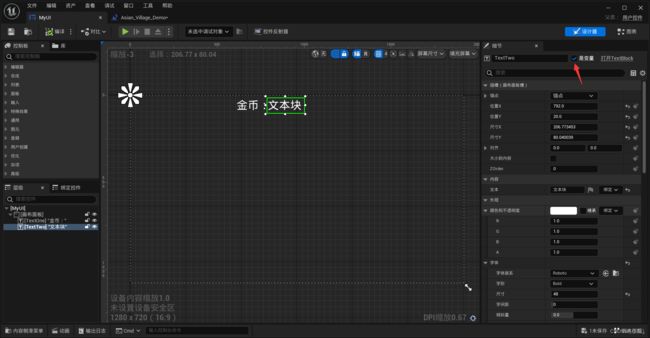
- 编写获取金币逻辑
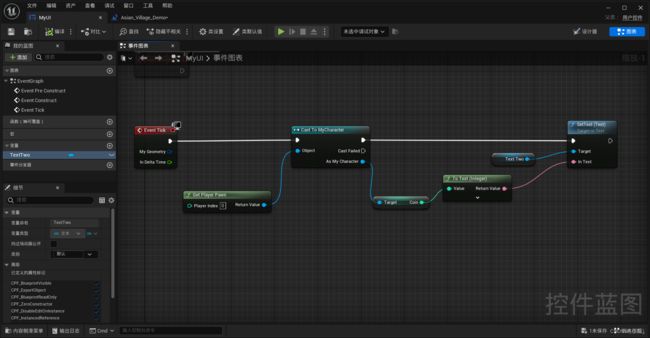
- 在关卡蓝图中创建自己的UI就完成啦
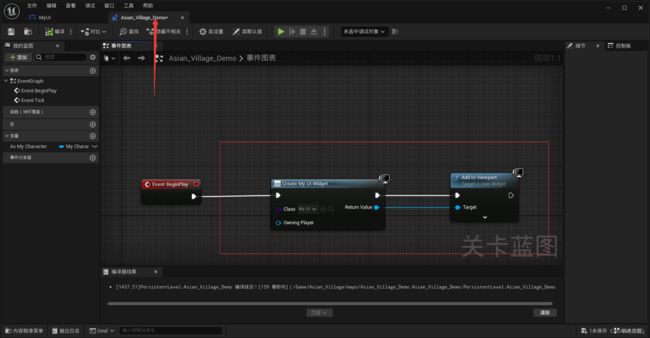
- 运行效果
MyCharacter.h
#pragma once
#include "CoreMinimal.h"
#include "GameFramework/Character.h"
#include "InputActionValue.h"
#include "MyCharacter.generated.h"
UCLASS()
class MYOBJECTUE5_API AMyCharacter : public ACharacter
{
GENERATED_BODY()
public:
AMyCharacter();
UPROPERTY(VisibleAnywhere, BlueprintReadOnly, Category = "Camera", meta = (AllPrivateAccess = "true"))
class USpringArmComponent* SpringArm;
UPROPERTY(VisibleAnywhere, BlueprintReadOnly, Category = "Camera", meta = (AllPrivateAccess = "true"))
class UCameraComponent* MyCamera;
UPROPERTY(EditAnywhere, BlueprintReadOnly, Category = "Input", meta = (AllPrivateAccess = "true"))
class UInputMappingContext* DefaultMappingContext;
UPROPERTY(EditAnywhere, BlueprintReadOnly, Category = "Input", meta = (AllPrivateAccess = "true"))
class UInputAction* MoveAction;
UPROPERTY(EditAnywhere, BlueprintReadOnly, Category = "Input", meta = (AllPrivateAccess = "true"))
class UInputAction* LookAction;
UPROPERTY(EditAnywhere, BlueprintReadOnly, Category = "Coin")
int Coin = 0;
protected:
virtual void BeginPlay() override;
void CharacterMove(const FInputActionValue& value);
void CharacterLook(const FInputActionValue& value);
public:
virtual void Tick(float DeltaTime) override;
virtual void SetupPlayerInputComponent(class UInputComponent* PlayerInputComponent) override;
};
MyCharacter.cpp
#include "MyCharacter.h"
#include "Camera/CameraComponent.h"
#include "GameFramework/SpringArmComponent.h"
#include "GameFramework/CharacterMovementComponent.h"
#include "EnhancedInputComponent.h"
#include "EnhancedInputSubsystems.h"
#include "Engine/Engine.h"
#include "MyObjectUE5/MyActors/MyActor_One.h"
AMyCharacter::AMyCharacter()
{
PrimaryActorTick.bCanEverTick = true;
GetCharacterMovement()->bOrientRotationToMovement = true;
GetCharacterMovement()->RotationRate = FRotator(0.f, 500.f, 0.f);
GetCharacterMovement()->MaxWalkSpeed = 500.f;
GetCharacterMovement()->MinAnalogWalkSpeed = 20.f;
GetCharacterMovement()->BrakingDecelerationWalking = 2000.f;
SpringArm = CreateDefaultSubobject<USpringArmComponent>(TEXT("SpringArm"));
SpringArm->SetupAttachment(GetRootComponent());
SpringArm->TargetArmLength = 400.f;
SpringArm->bUsePawnControlRotation = true;
MyCamera = CreateDefaultSubobject<UCameraComponent>(TEXT("MyCamera"));
MyCamera->SetupAttachment(SpringArm, USpringArmComponent::SocketName);
MyCamera->bUsePawnControlRotation = false;
}
void AMyCharacter::BeginPlay()
{
Super::BeginPlay();
APlayerController* PlayerController = Cast<APlayerController>(Controller);
if (PlayerController)
{
UEnhancedInputLocalPlayerSubsystem* Subsystem =
ULocalPlayer::GetSubsystem<UEnhancedInputLocalPlayerSubsystem>(PlayerController->GetLocalPlayer());
if (Subsystem)
{
Subsystem->AddMappingContext(DefaultMappingContext, 0);
}
}
}
void AMyCharacter::CharacterMove(const FInputActionValue& value)
{
FVector2D MovementVector = value.Get<FVector2D>();
if (Controller!=nullptr)
{
FRotator Rotation = Controller->GetControlRotation();
FRotator YawRotation = FRotator(0, Rotation.Yaw, 0);
FVector ForwardDirection = FRotationMatrix(YawRotation).GetUnitAxis(EAxis::X);
FVector RightDirection = FRotationMatrix(YawRotation).GetUnitAxis(EAxis::Y);
AddMovementInput(ForwardDirection, MovementVector.Y);
AddMovementInput(RightDirection, MovementVector.X);
}
}
void AMyCharacter::CharacterLook(const FInputActionValue& value)
{
FVector2D LookAxisVector = value.Get<FVector2D>();
if (Controller != nullptr)
{
AddControllerYawInput(LookAxisVector.X);
if (GetControlRotation().Pitch < 270.f && GetControlRotation().Pitch>180.f && LookAxisVector.Y > 0.f)
{
return;
}
if (GetControlRotation().Pitch < 180.f && GetControlRotation().Pitch>45.f && LookAxisVector.Y < 0.f)
{
return;
}
AddControllerPitchInput(LookAxisVector.Y);
}
}
void AMyCharacter::Tick(float DeltaTime)
{
Super::Tick(DeltaTime);
}
void AMyCharacter::SetupPlayerInputComponent(UInputComponent* PlayerInputComponent)
{
Super::SetupPlayerInputComponent(PlayerInputComponent);
UEnhancedInputComponent* EnhancedInputComponent = Cast<UEnhancedInputComponent>(PlayerInputComponent);
if (EnhancedInputComponent)
{
EnhancedInputComponent->BindAction(MoveAction, ETriggerEvent::Triggered, this, &AMyCharacter::CharacterMove);
EnhancedInputComponent->BindAction(LookAction, ETriggerEvent::Triggered, this, &AMyCharacter::CharacterLook);
}
}
MyActor_One.h
#pragma once
#include "CoreMinimal.h"
#include "GameFramework/Actor.h"
#include "MyActor_One.generated.h"
UCLASS()
class MYOBJECTUE5_API AMyActor_One : public AActor
{
GENERATED_BODY()
public:
AMyActor_One();
UPROPERTY(VisibleAnywhere, BlueprintReadOnly)
class UStaticMeshComponent* StaticMesh;
UPROPERTY(VisibleAnywhere, BlueprintReadWrite)
class USphereComponent* TriggerVolume;
UPROPERTY(EditAnywhere, BlueprintReadWrite, Category = "Interoperable|Particle")
class UParticleSystemComponent* ParticleEffectsComponent;
UPROPERTY(EditAnywhere, BlueprintReadWrite, Category = "Interoperable|Particle")
class UParticleSystem* Particle;
UPROPERTY(EditAnywhere, BlueprintReadWrite, Category = "Interoperable|Sounds")
class USoundCue* Sound;
UPROPERTY(EditAnywhere, BlueprintReadWrite, Category = "Interoperable Item|Properties")
bool bRotate = true;
UPROPERTY(EditAnywhere, BlueprintReadWrite, Category = "Interoperable Item|Properties")
float RotationRate = 45.f;
protected:
virtual void BeginPlay() override;
UFUNCTION()
void OnOverlapBegin(UPrimitiveComponent* OverlappedComponent, AActor* OtherActor, UPrimitiveComponent* OtherComp, int32 OtherBodyIndex, bool bFromSweep, const FHitResult& SweepResult);
UFUNCTION()
void OnOverlapEnd(UPrimitiveComponent* OverlappedComponent, AActor* OtherActor, UPrimitiveComponent* OtherComp, int32 OtherBodyIndex);
public:
virtual void Tick(float DeltaTime) override;
};
MyActor_One.cpp
#include "MyActor_One.h"
#include "Engine/Engine.h"
#include "UObject/ConstructorHelpers.h"
#include "Components/StaticMeshComponent.h"
#include "Components/SphereComponent.h"
#include "Particles/ParticleSystemComponent.h"
#include "MyObjectUE5/MyCharacters/MyCharacter.h"
#include "Kismet/GamePlayStatics.h"
#include "Sound/SoundCue.h"
AMyActor_One::AMyActor_One()
{
PrimaryActorTick.bCanEverTick = true;
StaticMesh = CreateDefaultSubobject<UStaticMeshComponent>(TEXT("StaticMesh"));
RootComponent = StaticMesh;
TriggerVolume = CreateDefaultSubobject<USphereComponent>(TEXT("TriggerVolume"));
TriggerVolume->SetupAttachment(GetRootComponent());
ParticleEffectsComponent = CreateDefaultSubobject<UParticleSystemComponent>(TEXT("ParticleEffects"));
ParticleEffectsComponent->SetupAttachment(GetRootComponent());
TriggerVolume->SetCollisionEnabled(ECollisionEnabled::QueryOnly);
TriggerVolume->SetCollisionObjectType(ECollisionChannel::ECC_WorldStatic);
TriggerVolume->SetCollisionResponseToAllChannels(ECollisionResponse::ECR_Ignore);
TriggerVolume->SetCollisionResponseToChannel(ECollisionChannel::ECC_Pawn, ECollisionResponse::ECR_Overlap);
}
void AMyActor_One::BeginPlay()
{
Super::BeginPlay();
TriggerVolume->OnComponentBeginOverlap.AddDynamic(this, &AMyActor_One::OnOverlapBegin);
TriggerVolume->OnComponentEndOverlap.AddDynamic(this, &AMyActor_One::OnOverlapEnd);
}
void AMyActor_One::OnOverlapBegin(UPrimitiveComponent* OverlappedComponent, AActor* OtherActor, UPrimitiveComponent* OtherComp, int32 OtherBodyIndex, bool bFromSweep, const FHitResult& SweepResult)
{
if (OtherActor)
{
AMyCharacter* Player = Cast<AMyCharacter>(OtherActor);
if (Player)
{
if (Particle)
{
UGameplayStatics::SpawnEmitterAtLocation(this, Particle, GetActorLocation(), FRotator(0.f), true);
}
if (Sound)
{
UGameplayStatics::PlaySound2D(this, Sound);
}
Player->Coin++;
GEngine->AddOnScreenDebugMessage(2, 10, FColor::Red, FString::Printf(TEXT("%d"), Player->Coin));
Destroy();
}
}
}
void AMyActor_One::OnOverlapEnd(UPrimitiveComponent* OverlappedComponent, AActor* OtherActor, UPrimitiveComponent* OtherComp, int32 OtherBodyIndex)
{
}
void AMyActor_One::Tick(float DeltaTime)
{
Super::Tick(DeltaTime);
if (bRotate)
{
FRotator rotator = GetActorRotation();
rotator.Yaw += RotationRate * DeltaTime;
SetActorRotation(rotator);
}
}