- Hutool TreeUtil快速构建树形数据结构
yifanghub
工具类java
在管理菜单、部门结构等场景时,我们经常需要将数据库中的层级数据转换为树形结构。本文将通过Hutool的TreeUtil工具类,实现零递归快速构建树形结构。一、环境准备JDK1.8+SpringBoot2.xHutool5.8.16MySQL8.0二、数据准备--创建部门表CREATETABLE`sys_dept`(`id`intNOTNULLAUTO_INCREMENT,`dept_name`va
- 4.服务注册发现:微服务的神经系统
在微服务架构中,服务之间不再是固定连接,而是高度动态、短暂存在的。如何让每个服务准确找到彼此,是分布式系统治理的核心问题之一。服务注册发现机制,正如神经系统之于人体,承担着连接、协调、感知变化的关键角色。本文将围绕Netflix开源的服务注册发现组件Eureka展开,深入剖析其原理,并以SpringCloud实战为导向,帮助你掌握服务治理的第一步。一、为什么需要服务注册发现?在单体架构中,服务调用
- 2.Spring Cloud生态全景解析:核心组件、能力边界与定位
碎风影
SpringCloud深度解析springcloudspring后端
导语:SpringCloud并非单一框架,而是基于SpringBoot构建的分布式系统工具集。它通过标准化封装,将服务发现、配置管理、熔断限流等复杂基础设施转化为开箱即用的组件,让开发者聚焦业务逻辑。本文将系统解析其核心组成、与SpringBoot的共生关系,并客观审视其能力边界,助您构建清晰的微服务技术选型地图。一、核心基石:SpringBoot与SpringCloud的共生关系关键结论:Spr
- SpringBoot3+JPA+MySQL实现多数据源的读写分离(基于EntityManagerFactory)
没刮胡子
java软件开发技术实战专栏SpringBoot3JPAMySQL多数据源读写分离
1、简介在SpringBoot中配置多个数据源并实现自动切换EntityManager,这里我编写了一个RoutingEntityManagerFactory和AOP(面向切面编程)的方式来实现。这里我配置了两个数据源:primary和secondary,其中primary主数据源用来写入数据,secondary从数据源用来读取数据。注意1:使用Springboot3的读写分离,首先要保证主库和从
- 微服务世界的“导航仪”!Spring Cloud五大注册中心选型指南,从此不再迷路!
码农技术栈
微服务微服务springcloud架构springbootjava后端
引言:为什么微服务需要“导航仪”?想象一下,你走进一座巨大的迷宫(微服务集群),里面有成百上千个房间(服务实例),每个房间都在动态变化位置(服务扩缩容)。注册中心就像迷宫里的导航仪,实时记录所有房间的位置,告诉你怎么最快找到目标。没有它?你可能会永远迷失在“服务调用”的迷宫里!注册中心的核心作用服务注册:服务启动时,主动上报自己的地址和状态。服务发现:调用方通过注册中心查询目标服务的位置。健康监测
- Java基础学习笔记2
qichi333
学习笔记javaeclipse
今天是Java基础学习第二天,加油!!!下面是我今天记的一些笔记。(有点懒惰了,爬虫今天没学,因为赖床了(bushi),但我会勤奋起来的^_^,一定一定!明天不能偷懒了天!!)一、运算符例子:inta=10;intb=20;intc=a+b;其中,“+”是运算符,且是算术运算符;“a+b”是表达式,且是算术表达式。1.算术运算符例1:publicclassdemo3{publicstaticvoi
- spring-data-jpa+spring+hibernate+druid配置
参考链接:http://doc.okbase.net/liuyitian/archive/109276.htmlhttp://my.oschina.net/u/1859292/blog/312188最新公司的web项目需要用到spring-data-jpa作为JPA的实现框架,同时使用阿里巴巴的开源数据库连接池druid。关于这两种框架的介绍我在这里就不多赘述。直接进入配置页面:spring的配置
- 自己动手写编译器
JeffWoodNo.1
编译器hexcompilerfunctionbrancheclipse
这里不再仅仅是简单的记录一下……直接上手环境目标1:在elicpse平台上使用ant构建ANTLR目标2:在elicpse平台上使用antlride编写ANTLR语法编写实用的C解析器背景调查到底还需不需要自己动手写一个编译器这就是“工具哲学”所谓bootstrap的编译器构造方式。C的核心子集Appendix直接上手环境antlr-for-eclipse,即antlride-2.0-rc4版,在
- Spring Data Jpa +alibaba druid+query dsl 实现多数据源
下海揽月
springdatajpajava
SpringDataJpa+alibabadruid+querydsl实现多数据源,主要通过配置来实现多个数据源的操作,无需动态切换1.maven配置org.springframework.bootspring-boot-starter-data-jpa2.3.12.RELEASEcom.alibabadruid-spring-boot-starter1.1.24com.querydslquery
- Spring Boot + Spring JPA + JDBC + Druid实现动态数据源切换
Apr01Chell
代码片段springjava数据库
SpringBoot+SpringJPA+JDBC+Druid实现动态数据源切换目录SpringBoot+SpringJPA+JDBC+Druid实现动态数据源切换AbstractRoutingDataSource源码分析需求代码实现DynamicDataSourceDBContextHolderDruidDbConfigDataSourcePropertiesAllDataSourcesExec
- Spring框架中的Component与Bean注解
SpringBoot中的@Bean与@Component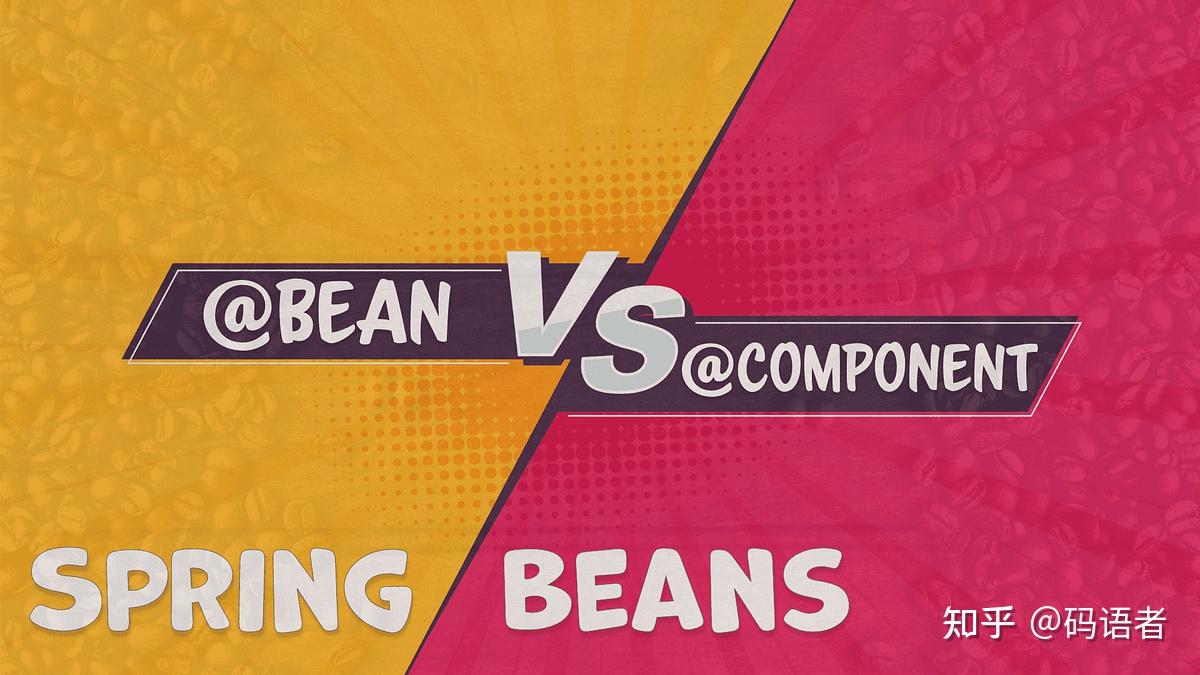Spring的@Component和@Bean注解的关键区别在于:@Bean注解可用于暴露您自己编写的JavaBeans,而@Component注解可用于暴露源代码由他人维护的JavaBeans。Sprin
- Flex与Spring集成
hkmw
Flex配置springflexapplicationdependenciescomponentsaccess
Flex与Spring集成UsingFlexwithSpringUPDATE(1/12/2007):IputtogetheraTomcat-basedTestDriveServerthatincludesthesamplesdescribedbelowrunningout-of-thebox.Readthispostformoreinfo.WhatisSpring?Springisoneofthe
- 【视频观看系统】- 技术与架构选型
✅项目技术选型方案一、整体架构风格项目层级技术选型说明架构风格微服务架构(SpringCloud)独立部署、易扩展、易维护服务通信HTTP(RestTemplate或Feign)+RocketMQ同步调用+异步事件注册中心Nacos服务注册、发现、配置中心配置中心Nacos配置管理多服务统一配置API网关SpringCloudGateway路由转发、权限验证、限流服务监控SpringBootAdm
- SpringBoot ThreadLocal 全局动态变量设置
xdscode
springbootjavaThreadLocal
需求说明:现有一个游戏后台管理系统,该系统可管理多个大区的数据,但是需要使用大区id实现数据隔离,并且提供了大区选择功能,先择大区后展示对应的数据。需要实现一下几点:1.前端请求时,area_id是必传的1.数据隔离,包括查询及增删改:使用mybatis拦截器实现2.多个用户同时操作互不影响3.非前端调用场景的处理:定时任务、mq1.前端决定area_id为了解决多个用户可以互不影响的使用不同的a
- 实操 SpringBoot+MCP!
清风孤客
springboot后端java人工智能
引言随着人工智能的飞速发展,大语言模型(LLM)正在革命性地重塑用户与软件的交互范式。想象一下这样的场景:用户无需钻研复杂的API文档或者在繁琐的表单间来回切换,只需通过自然语言直接与系统对话——“帮我查找所有2023年出版的图书”、“创建一个新用户叫张三,邮箱是
[email protected]”。这种直观、流畅的交互方式不仅能显著降低新用户的学习曲线,更能大幅削减B端系统的培训成本和实施
- 【SpringBoot】Spring Boot 高并发优化终极指南,涵盖线程模型、JVM 调优、数据库访问、缓存策略等 15+ 核心模块
夜雨hiyeyu.com
javaspringbootjvmspringjava后端性能优化系统架构
SpringBoot高并发优化终极指南,涵盖线程模型、JVM调优、数据库访问、缓存策略等15+核心模块一、线程模型深度调优(核心瓶颈突破)1.Tomcat线程池原子级配置2.异步任务线程池隔离策略二、JVM层终极调参(G1GC深度优化)1.内存分配策略2.GC日志分析技巧三、缓存策略原子级优化1.三级缓存架构实现2.缓存穿透/雪崩防护四、数据库访问极致优化1.连接池死亡参数配置2.分页查询深度优化
- SpringBoot+AOP+自定义注解,实现日志记录
一.定义自定义注解importjava.lang.annotation.*;/***@authorawen*定义注解目的想让他当作切点*/@Target({ElementType.METHOD})@Retention(RetentionPolicy.RUNTIME)//.java.class字节码@Documentedpublic@interfaceLog{/***处理类型**@return{@l
- spring boot使用mybatis-plus实现分页功能
kong@react
springbootmybatis后端
使用MyBatis-Plus实现分页功能MyBatis-Plus提供了内置的分页插件,可以轻松实现分页查询功能。以下是实现分页的具体方法:配置分页插件在SpringBoot项目中,需要在配置类中注册分页插件:@ConfigurationpublicclassMybatisPlusConfig{@BeanpublicMybatisPlusInterceptormybatisPlusIntercept
- springboot通过aop实现全局日志(是否自定义注解都可以)
甜无能
springbootjava#aopspringbootjavaaop全局日志自定义注解
内容参考自以下两个链接1、springboot中使用AOP切面完成全局日志_aop全局日志_邹飞鸣的博客-CSDN博客使用AOP记录日志_aop日志_trusause的博客-CSDN博客第一个链接思路很清晰,讲的也很详细,第二个链接讲了自定义注解为了便于自己理解做了以下整理目录1.aspectj基本概念2.添加aop依赖3.进行切面处理(1)切面类(2)自定义注解(3)controller和ser
- SpringBoot AOP+注解 全局日志记录
xdscode
springbootjavaAOP
一、需求描述如何优雅地记录用户操作日志?网站后台,功能开发完成后,新增了一个需求,即需要记录用户的各种操作记录。由于是在开发后期,如果针对每一个功能都去添加一段记录日志的代码,工作量较大、代码侵入性太强,因此采用AOP+注解的方式实现。可读性大大提高,且便于维护和扩展。AOP:面向切面编程,在不修改现有逻辑代码的情况下,增强功能,恰好体现了spring的理念:无入侵式自定义注解:当被注解的方法执行
- Spring Bean 生命周期 SmartLifecycle接口介绍和使用场景 和 Lifecycle对比
极光雨雨
#Spring全家springjava
在SpringBoot中,SmartLifecycle是org.springframework.context.Lifecycle接口的一个扩展接口,它提供了更细粒度的控制生命周期的方法。Spring容器管理Bean的生命周期时,可以通过实现SmartLifecycle接口来定义自定义的启动和关闭逻辑。一、使用前提需要在Spring容器启动完成后执行某些初始化操作。需要在应用关闭前做一些清理工作(
- Java程序设计(二十七):基于SSM框架的OA办公自动化管理平台的设计与实现
人工智能_SYBH
2025年java程序设计java数据挖掘开发语言vue.js后端人工智能springboot
1.项目概述办公自动化(OA,OfficeAutomation)管理平台是企业实现内部管理信息化的重要工具。本文提出并实现了一个基于Java的OA办公自动化管理平台。该平台基于SSM架构(Spring+SpringMVC+MyBatis),数据库采用MySQL,并通过HTML、CSS、JavaScript等技术实现用户界面。1.1平台功能简介平台提供了管理员、普通用户和部门三类角色,分别具有不同的
- 【人工智能】Spring AI Alibaba,一个面向 Java 开发者的开源框架,它旨在简化将人工智能(AI)功能集成到应用程序中的过程。
本本本添哥
A-AIGC人工智能大模型人工智能javaspring
一、SpringAIAlibaba介绍SpringAIAlibaba是一个面向Java开发者的开源框架,它旨在简化将人工智能(AI)功能集成到应用程序中的过程。该项目基于SpringAI构建,并且是阿里云通义系列模型及服务在JavaAI应用开发领域的最佳实践。SpringAIAlibaba的目标是为开发者提供一套高层次的AIAPI抽象以及与云原生基础设施的深度集成方案,从而帮助他们快速构建智能应用
- SpringAI Alibaba 正式版发布!四个问题让你彻底拿捏它
小付爱coding
人工智能
SpringAIAlibaba正式版发布!四个问题让你彻底拿捏它作者:XXX|发布时间:2025年4月最近,SpringAIAlibaba正式版重磅上线了!作为一个Java开发者,如果你还没听说过它,那你可能真的要掉队了。别急,今天我就用最通俗的方式带你搞懂这玩意儿到底是个啥、为啥要学它、学什么、能干啥!一、SpringAIAlibaba到底是个啥?一句话总结:SpringAIAlibaba是一个
- Spring Data Neo4j 与后端人工智能算法的数据交互
AI大模型应用实战
springneo4j人工智能ai
SpringDataNeo4j与后端人工智能算法的数据交互关键词:SpringDataNeo4j、图数据库、人工智能算法、数据交互、知识图谱、图神经网络、数据集成摘要:本文深入探讨了如何利用SpringDataNeo4j框架实现后端人工智能算法与图数据库的高效数据交互。文章首先介绍了图数据库和人工智能算法的基本概念,然后详细解析了SpringDataNeo4j的核心架构和原理。接着,通过实际代码示
- SpringBoot+Mybatis+MySQL+Vue+ElementUI前后端分离版:整体布局、架构调整(二)
喜欢敲代码的程序员
前后端分离SpringBootSpringspringbootmybatismysqlvue.jselementui
目录一、前言二、后端调整1.实体类调整2.菜单相关接口3.用户相关接口4.新增工具类5.新增菜单树返回类6.配置类、拦截器三、前端调整1.请求调整2.页面布局、样式调整1.user.vue2.index.vue3.请求拦截四、开发过程中的问题五、附:源码1.源码下载地址六、结语一、前言此文章在上次的基础上进行了部分调整,并根据用户体验(我自己)确认了页面整体布局和数据呈现,暂定就先这样,后续有需要
- Spring Security:认证与授权的实现原理及实践
SpringSecurity是Spring生态中强大的安全框架,用于为Java应用提供认证(Authentication)和授权(Authorization)功能。根据2024年StackOverflow开发者调查,SpringBoot是Java开发者中最流行的框架,约60%的Java开发者使用它构建微服务,而SpringSecurity是其首选安全解决方案。本文深入剖析SpringSecurit
- 创建 TransactionStatus
悟能不能悟
log4jjava开发语言
在Spring框架中,TransactionStatus是一个接口,通常由事务管理器(如PlatformTransactionManager)在开启事务时自动创建,而不是由开发者直接实例化。如果你需要在代码中操作事务状态,应通过以下标准方式:正确获取TransactionStatus的步骤:注入事务管理器在SpringBean中注入PlatformTransactionManager(如DataS
- [3-02-01].第14节:三方整合 - SpringData整合Redis集群
1.01^1000
阶段03:企业框架springboot
Redis大纲一、SpringBoot整合主从架构的Redis:1.1.问题说明:1.在Sentinel集群监管下的Redis哨兵架构中,其节点会因为自动故障转移而发生变化,Redis的客户端必须感知这种变化,及时更新连接信息2.SpringBoot中的RedisTemplate底层利用lettuce实现了节点的感知和自动切换,我们需要进行配置才可以实现这种动态上下线的情况。下面,我们通过一个测试
- IDEA Maven报错 无法解析 com.taobao:parent:pom:1.0.1【100%解决 此类型问题】
Dolphin_Home
私有_案例分析生产环境_场景抽象Debugintellij-ideamavenjava
IDEAMaven报错无法解析com.taobao:parent:pom:1.0.1【100%解决此类型问题】报错日志PSD:\Learn_Materials\IDEA_WorkSpace\Demo\spring_test_demo>mvncleaninstall-U[INFO]Scanningforprojects...[WARNING][WARNING]Someproblemswereenco
- Nginx负载均衡
510888780
nginx应用服务器
Nginx负载均衡一些基础知识:
nginx 的 upstream目前支持 4 种方式的分配
1)、轮询(默认)
每个请求按时间顺序逐一分配到不同的后端服务器,如果后端服务器down掉,能自动剔除。
2)、weight
指定轮询几率,weight和访问比率成正比
- RedHat 6.4 安装 rabbitmq
bylijinnan
erlangrabbitmqredhat
在 linux 下安装软件就是折腾,首先是测试机不能上外网要找运维开通,开通后发现测试机的 yum 不能使用于是又要配置 yum 源,最后安装 rabbitmq 时也尝试了两种方法最后才安装成功
机器版本:
[root@redhat1 rabbitmq]# lsb_release
LSB Version: :base-4.0-amd64:base-4.0-noarch:core
- FilenameUtils工具类
eksliang
FilenameUtilscommon-io
转载请出自出处:http://eksliang.iteye.com/blog/2217081 一、概述
这是一个Java操作文件的常用库,是Apache对java的IO包的封装,这里面有两个非常核心的类FilenameUtils跟FileUtils,其中FilenameUtils是对文件名操作的封装;FileUtils是文件封装,开发中对文件的操作,几乎都可以在这个框架里面找到。 非常的好用。
- xml文件解析SAX
不懂事的小屁孩
xml
xml文件解析:xml文件解析有四种方式,
1.DOM生成和解析XML文档(SAX是基于事件流的解析)
2.SAX生成和解析XML文档(基于XML文档树结构的解析)
3.DOM4J生成和解析XML文档
4.JDOM生成和解析XML
本文章用第一种方法进行解析,使用android常用的DefaultHandler
import org.xml.sax.Attributes;
- 通过定时任务执行mysql的定期删除和新建分区,此处是按日分区
酷的飞上天空
mysql
使用python脚本作为命令脚本,linux的定时任务来每天定时执行
#!/usr/bin/python
# -*- coding: utf8 -*-
import pymysql
import datetime
import calendar
#要分区的表
table_name = 'my_table'
#连接数据库的信息
host,user,passwd,db =
- 如何搭建数据湖架构?听听专家的意见
蓝儿唯美
架构
Edo Interactive在几年前遇到一个大问题:公司使用交易数据来帮助零售商和餐馆进行个性化促销,但其数据仓库没有足够时间去处理所有的信用卡和借记卡交易数据
“我们要花费27小时来处理每日的数据量,”Edo主管基础设施和信息系统的高级副总裁Tim Garnto说道:“所以在2013年,我们放弃了现有的基于PostgreSQL的关系型数据库系统,使用了Hadoop集群作为公司的数
- spring学习——控制反转与依赖注入
a-john
spring
控制反转(Inversion of Control,英文缩写为IoC)是一个重要的面向对象编程的法则来削减计算机程序的耦合问题,也是轻量级的Spring框架的核心。 控制反转一般分为两种类型,依赖注入(Dependency Injection,简称DI)和依赖查找(Dependency Lookup)。依赖注入应用比较广泛。
- 用spool+unixshell生成文本文件的方法
aijuans
xshell
例如我们把scott.dept表生成文本文件的语句写成dept.sql,内容如下:
set pages 50000;
set lines 200;
set trims on;
set heading off;
spool /oracle_backup/log/test/dept.lst;
select deptno||','||dname||','||loc
- 1、基础--名词解析(OOA/OOD/OOP)
asia007
学习基础知识
OOA:Object-Oriented Analysis(面向对象分析方法)
是在一个系统的开发过程中进行了系统业务调查以后,按照面向对象的思想来分析问题。OOA与结构化分析有较大的区别。OOA所强调的是在系统调查资料的基础上,针对OO方法所需要的素材进行的归类分析和整理,而不是对管理业务现状和方法的分析。
OOA(面向对象的分析)模型由5个层次(主题层、对象类层、结构层、属性层和服务层)
- 浅谈java转成json编码格式技术
百合不是茶
json编码java转成json编码
json编码;是一个轻量级的数据存储和传输的语言
在java中需要引入json相关的包,引包方式在工程的lib下就可以了
JSON与JAVA数据的转换(JSON 即 JavaScript Object Natation,它是一种轻量级的数据交换格式,非
常适合于服务器与 JavaScript 之间的数据的交
- web.xml之Spring配置(基于Spring+Struts+Ibatis)
bijian1013
javaweb.xmlSSIspring配置
指定Spring配置文件位置
<context-param>
<param-name>contextConfigLocation</param-name>
<param-value>
/WEB-INF/spring-dao-bean.xml,/WEB-INF/spring-resources.xml,
/WEB-INF/
- Installing SonarQube(Fail to download libraries from server)
sunjing
InstallSonar
1. Download and unzip the SonarQube distribution
2. Starting the Web Server
The default port is "9000" and the context path is "/". These values can be changed in &l
- 【MongoDB学习笔记十一】Mongo副本集基本的增删查
bit1129
mongodb
一、创建复本集
假设mongod,mongo已经配置在系统路径变量上,启动三个命令行窗口,分别执行如下命令:
mongod --port 27017 --dbpath data1 --replSet rs0
mongod --port 27018 --dbpath data2 --replSet rs0
mongod --port 27019 -
- Anychart图表系列二之执行Flash和HTML5渲染
白糖_
Flash
今天介绍Anychart的Flash和HTML5渲染功能
HTML5
Anychart从6.0第一个版本起,已经逐渐开始支持各种图的HTML5渲染效果了,也就是说即使你没有安装Flash插件,只要浏览器支持HTML5,也能看到Anychart的图形(不过这些是需要做一些配置的)。
这里要提醒下大家,Anychart6.0版本对HTML5的支持还不算很成熟,目前还处于
- Laravel版本更新异常4.2.8-> 4.2.9 Declaration of ... CompilerEngine ... should be compa
bozch
laravel
昨天在为了把laravel升级到最新的版本,突然之间就出现了如下错误:
ErrorException thrown with message "Declaration of Illuminate\View\Engines\CompilerEngine::handleViewException() should be compatible with Illuminate\View\Eng
- 编程之美-NIM游戏分析-石头总数为奇数时如何保证先动手者必胜
bylijinnan
编程之美
import java.util.Arrays;
import java.util.Random;
public class Nim {
/**编程之美 NIM游戏分析
问题:
有N块石头和两个玩家A和B,玩家A先将石头随机分成若干堆,然后按照BABA...的顺序不断轮流取石头,
能将剩下的石头一次取光的玩家获胜,每次取石头时,每个玩家只能从若干堆石头中任选一堆,
- lunce创建索引及简单查询
chengxuyuancsdn
查询创建索引lunce
import java.io.File;
import java.io.IOException;
import org.apache.lucene.analysis.Analyzer;
import org.apache.lucene.analysis.standard.StandardAnalyzer;
import org.apache.lucene.document.Docume
- [IT与投资]坚持独立自主的研究核心技术
comsci
it
和别人合作开发某项产品....如果互相之间的技术水平不同,那么这种合作很难进行,一般都会成为强者控制弱者的方法和手段.....
所以弱者,在遇到技术难题的时候,最好不要一开始就去寻求强者的帮助,因为在我们这颗星球上,生物都有一种控制其
- flashback transaction闪回事务查询
daizj
oraclesql闪回事务
闪回事务查询有别于闪回查询的特点有以下3个:
(1)其正常工作不但需要利用撤销数据,还需要事先启用最小补充日志。
(2)返回的结果不是以前的“旧”数据,而是能够将当前数据修改为以前的样子的撤销SQL(Undo SQL)语句。
(3)集中地在名为flashback_transaction_query表上查询,而不是在各个表上通过“as of”或“vers
- Java I/O之FilenameFilter类列举出指定路径下某个扩展名的文件
游其是你
FilenameFilter
这是一个FilenameFilter类用法的例子,实现的列举出“c:\\folder“路径下所有以“.jpg”扩展名的文件。 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
- C语言学习五函数,函数的前置声明以及如何在软件开发中合理的设计函数来解决实际问题
dcj3sjt126com
c
# include <stdio.h>
int f(void) //括号中的void表示该函数不能接受数据,int表示返回的类型为int类型
{
return 10; //向主调函数返回10
}
void g(void) //函数名前面的void表示该函数没有返回值
{
//return 10; //error 与第8行行首的void相矛盾
}
in
- 今天在测试环境使用yum安装,遇到一个问题: Error: Cannot retrieve metalink for repository: epel. Pl
dcj3sjt126com
centos
今天在测试环境使用yum安装,遇到一个问题:
Error: Cannot retrieve metalink for repository: epel. Please verify its path and try again
处理很简单,修改文件“/etc/yum.repos.d/epel.repo”, 将baseurl的注释取消, mirrorlist注释掉。即可。
&n
- 单例模式
shuizhaosi888
单例模式
单例模式 懒汉式
public class RunMain {
/**
* 私有构造
*/
private RunMain() {
}
/**
* 内部类,用于占位,只有
*/
private static class SingletonRunMain {
priv
- Spring Security(09)——Filter
234390216
Spring Security
Filter
目录
1.1 Filter顺序
1.2 添加Filter到FilterChain
1.3 DelegatingFilterProxy
1.4 FilterChainProxy
1.5
- 公司项目NODEJS实践0.1
逐行分析JS源代码
mongodbnginxubuntunodejs
一、前言
前端如何独立用nodeJs实现一个简单的注册、登录功能,是不是只用nodejs+sql就可以了?其实是可以实现,但离实际应用还有距离,那要怎么做才是实际可用的。
网上有很多nod
- java.lang.Math
liuhaibo_ljf
javaMathlang
System.out.println(Math.PI);
System.out.println(Math.abs(1.2));
System.out.println(Math.abs(1.2));
System.out.println(Math.abs(1));
System.out.println(Math.abs(111111111));
System.out.println(Mat
- linux下时间同步
nonobaba
ntp
今天在linux下做hbase集群的时候,发现hmaster启动成功了,但是用hbase命令进入shell的时候报了一个错误 PleaseHoldException: Master is initializing,查看了日志,大致意思是说master和slave时间不同步,没办法,只好找一种手动同步一下,后来发现一共部署了10来台机器,手动同步偏差又比较大,所以还是从网上找现成的解决方
- ZooKeeper3.4.6的集群部署
roadrunners
zookeeper集群部署
ZooKeeper是Apache的一个开源项目,在分布式服务中应用比较广泛。它主要用来解决分布式应用中经常遇到的一些数据管理问题,如:统一命名服务、状态同步、集群管理、配置文件管理、同步锁、队列等。这里主要讲集群中ZooKeeper的部署。
1、准备工作
我们准备3台机器做ZooKeeper集群,分别在3台机器上创建ZooKeeper需要的目录。
数据存储目录
- Java高效读取大文件
tomcat_oracle
java
读取文件行的标准方式是在内存中读取,Guava 和Apache Commons IO都提供了如下所示快速读取文件行的方法: Files.readLines(new File(path), Charsets.UTF_8); FileUtils.readLines(new File(path)); 这种方法带来的问题是文件的所有行都被存放在内存中,当文件足够大时很快就会导致
- 微信支付api返回的xml转换为Map的方法
xu3508620
xmlmap微信api
举例如下:
<xml>
<return_code><![CDATA[SUCCESS]]></return_code>
<return_msg><![CDATA[OK]]></return_msg>
<appid><