import subprocess
import requests
import time
import json
class MacInfo:
def __init__(self):
self.strSerial_Number = ""
self.strChip = ""
self.strProcessor_Name = ""
self.strProcessor_Speed = ""
self.valueNumber_of_Processors = ""
self.valueTotal_Number_of_Cores = ""
self.strMemory = ""
self.strHardware_UUID = ""
def getModel_Identifier(self):
cmd = "system_profiler SPHardwareDataType | grep 'Model Identifier'| awk '{print $3}'"
result = subprocess.run(cmd, stdout=subprocess.PIPE, shell=True, check=True)
model_identifier = result.stdout.strip()
s = str(model_identifier, 'utf-8')
return s
'''
def getProcessor_Nmae(self):
cmd = "system_profiler SPHardwareDataType | grep 'Processor Name' | awk '{print $3$4$5$6}'"
result = subprocess.run(cmd, stdout=subprocess.PIPE, shell=True, check=True)
processor_nmae = result.stdout.strip()
return processor_nmae'''
def getChip(self):
cmd = "system_profiler SPHardwareDataType | grep 'Chip' | awk '{print $2$3$4$5$6}'"
result = subprocess.run(cmd, stdout=subprocess.PIPE, shell=True, check=True)
chip = result.stdout.strip()
return chip
def getProcessor_Speed(self):
cmd = "system_profiler SPHardwareDataType | grep 'Processor Speed' | awk '{print $3$4}'"
result = subprocess.run(cmd, stdout=subprocess.PIPE, shell=True, check=True)
processor_speed = result.stdout.strip()
return processor_speed
def getNumber_of_Processors(self):
cmd = "system_profiler SPHardwareDataType | grep 'Number of Processors' | awk '{print $4}'"
result = subprocess.run(cmd, stdout=subprocess.PIPE, shell=True, check=True)
number_of_processors = result.stdout.strip()
return number_of_processors
def getTotal_Number_of_Cores(self):
cmd = "system_profiler SPHardwareDataType | grep 'Total Number of Cores' | awk '{print $5}'"
result = subprocess.run(cmd, stdout=subprocess.PIPE, shell=True, check=True)
total_number_of_cores = result.stdout.strip()
return total_number_of_cores
def getMemory(self):
cmd = "system_profiler SPHardwareDataType | grep 'Memory' | awk '{print $2$3}'"
result = subprocess.run(cmd, stdout=subprocess.PIPE, shell=True, check=True)
memory = result.stdout.strip()
s = str(memory, 'utf-8')
return s
def getSerial_Number(self):
cmd = "system_profiler SPHardwareDataType | grep 'Serial Number' | awk '{print $4}'"
result = subprocess.run(cmd, stdout=subprocess.PIPE, shell=True, check=True)
serial_number = result.stdout.strip()
s = str(serial_number, 'utf-8')
return s
def get_CUP(self):
cmd = 'sysctl -n machdep.cpu.brand_string'
result = subprocess.run(cmd, stdout=subprocess.PIPE, shell=True, check=True)
data = result.stdout.split()
m = []
for i in data:
s = str(i, 'utf-8')
m.append(s)
CUP = "".join(m)
return CUP
def get_MAC(self):
cmd = 'ifconfig en0'
result = subprocess.run(cmd, stdout=subprocess.PIPE, shell=True, check=True)
ipMAC = result.stdout.split()[6]
s = str(ipMAC, 'utf-8')
return s
def get_IP(self):
cmd = 'ifconfig en0'
result = subprocess.run(cmd, stdout=subprocess.PIPE, shell=True, check=True)
IP = result.stdout.split()[15]
s = str(IP, 'UTF-8')
return s
def get_disk(self):
cmd = 'diskutil list'
result = subprocess.run(cmd, stdout=subprocess.PIPE, shell=True, check=True)
disk = result.stdout.split()[10]
s = str(disk, 'utf-8').replace("*", '') + " GB"
if self.getModel_Identifier().startswith('Intel'):
return s
else:
cmd1 = 'diskutil list'
result = subprocess.run(cmd1, stdout=subprocess.PIPE, shell=True, check=True)
disk = result.stdout.split()[9]
s1 = str(disk, "utf-8")
return s1
def Webhook(self):
url = "这里写自己企业微信创建的机器人的webhook地址"
headers = {'Content-Type': 'application/json;charset=utf-8'}
data_text = {
"msgtype": "markdown",
"markdown": {
"content": f"MAC电脑配置信息请相关同事注意。\n>"
f"Model Identifier:{self.getModel_Identifier()}"
f"\n> Serial Number:"
f"{self.getSerial_Number()}\n> Chip:{self.get_CUP()}"
f"\n Memory:{self.getMemory()}"
f"\n disk:{self.get_disk()}"
f"\n ip:{self.get_IP()}"
f"\n mac:{self.get_MAC()}"
}
}
r = requests.post(url, data=json.dumps(data_text), headers=headers)
if __name__ == '__main__':
objHardware = MacInfo()
print("Model Identifier:{}".format(objHardware.getModel_Identifier()))
print("Serial Number:{}".format(objHardware.getSerial_Number()))
print('Chip:{}'.format(objHardware.get_CUP()))
print("Memory:{}".format(objHardware.getMemory()))
print('disk:{}'.format(objHardware.get_disk()))
print('ip:{}'.format(objHardware.get_IP()))
print('mac:{}'.format(objHardware.get_MAC()))
objHardware.Webhook()
time.sleep(200)
input('')
接下来看效果
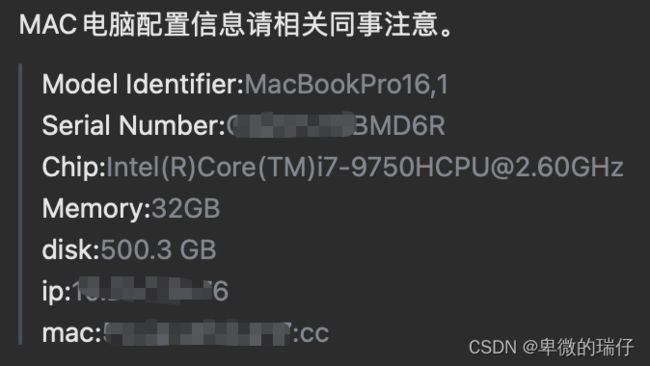