文章目录
-
- 一、鼠标事件
- 二、键盘事件
-
- 2.1、按下键盘键(keydown)
- 2.2、抬起键盘键(keyup)
- 2.3、按下有效键(keypress)
- 2.4、常见键盘码(了解)
- 三、焦点事件
-
- 3.1、聚焦(focus)
- 3.2、失焦(blur)
- 3.3、扩展(focusin、focusout)
- 四、加载事件
-
- 4.1、加载完成(onload)
- 4.2、加载失败(onerror)
- 4.3、窗口变化(onresize)
一、鼠标事件
点击事件 |
描述(指鼠标左键) |
click |
鼠标点击事件 |
dblclick |
鼠标双击事件 |
mousedown |
鼠标按下事件 |
mouseup |
鼠标抬起事件 |
鼠标点击事件触发顺序是:
1. mousedown 首先触发 2. mouseup 接着触发
3. click 之后触发 4. dblclick 最后触发
移动事件 |
描述 |
mousemove |
当鼠标在一个节点内部移动时触发 |
mouseout |
鼠标离开一个节点时触发,离开父节点也会触发这个事件 |
mouseover |
鼠标进入一个节点时触发,进入子节点会再一次触发这个事件 |
mouseleave |
鼠标离开一个节点时触发,离开父节点不会触发这个事件 |
mouseenter |
鼠标进入一个节点时触发,进入子节点不会触发这个事件 |
其他事件 |
描述 |
contextmenu |
按下鼠标右键时(上下文菜单出现前)触发 |
wheel |
滚动鼠标的滚轮时触发,该事件继承的是WheelEvent接口 |
二、键盘事件
事件 |
描述 |
keydown |
键盘按下事件 |
keypress |
按下有值的键时触发,即按下 Ctrl、Alt、Shift、Meta 这样无值的键,这个事件不会触发 |
keyup |
键盘抬起事件 |
鼠标点击事件触发顺序是:
1. keydown 首先触发 2. keypress 接着触发 3. keyup 最后触发
2.1、按下键盘键(keydown)
DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>title>
head>
<body>
<input id="input" type="text">
body>
<script>
const input = document.getElementById("input");
input.addEventListener(
"keydown",
(event) => {
console.log(event.code)
},
true,
);
script>
head>
html>
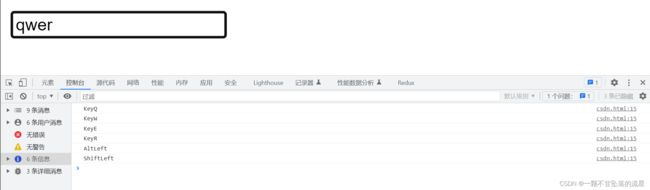
2.2、抬起键盘键(keyup)
DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>title>
head>
<body>
<input id="input" type="text">
body>
<script>
const input = document.getElementById("input");
input.addEventListener(
"keyup",
(event) => {
console.log(event.code)
},
true,
);
script>
head>
html>
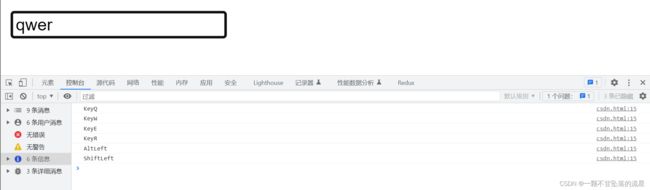
2.3、按下有效键(keypress)
已弃用: 不再推荐使用该特性。虽然一些浏览器仍然支持它,但也许已从相关的 web 标准中移除,也许正准备移除或出于兼容性而保留。请尽量不要使用该特性,并更新现有的代码;
2.4、常见键盘码(了解)
function showChar(e) {
console.log('当前按下的键是: ' + e.code);
}
document.body.addEventListener('keydown', showChar, false);
按键 |
按键码 |
数字键0 - 9 |
Digit0 - Digit9 |
字母键A - z |
KeyA - KeyZ(不区分大小写) |
功能键F1 - F12 |
F1 - F12 |
方向键 |
ArrowDown、ArrowUp、ArrowLeft、ArrowRight |
Alt 键 |
AltLeft 或 AltRight |
Shift 键 |
ShiftLeft 或 ShiftRight |
Ctrl 键 |
ControlLeft或ControlRight |
删除键 |
Delete |
制表符 |
Tab |
回车键 |
Enter |
空格键 |
Space |
Esc 键 |
Escape |
三、焦点事件
焦点事件发生在元素节点和document对象上面,与获得或失去焦点相关。它主要包括以下四个事件。
事件 |
描述 |
是否冒泡 |
focus |
元素节点获得焦点后触发 |
否 |
blur |
元素节点失去焦点后触发 |
否 |
focusin |
元素节点将要获得焦点时触发,发生在focus事件之前 |
是 |
focusout |
元素节点将要失去焦点时触发,发生在blur事件之前 |
是 |
- 由于
focus
和blur
事件不会冒泡,只能在捕获阶段触发,所以addEventListener
方法的第三个参数需要设为true
。
const form = document.getElementById("form");
form.addEventListener('focus', function (event) {
event.target.style.background = 'pink';
}, true);
form.addEventListener('blur', function (event) {
event.target.style.background = '';
}, true);
3.1、聚焦(focus)
DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>title>
head>
<body>
<form id="form">
<input type="text" placeholder="text input" />
<input type="password" placeholder="password" />
form>
body>
<script>
const form = document.getElementById("form");
form.addEventListener(
"focus",
(event) => {
event.target.style.background = "pink";
},
true,
);
script>
head>
html>

3.2、失焦(blur)
blur
事件:当一个元素失去焦点的时候 blur 事件被触发
DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>title>
head>
<body>
<form id="form">
<input type="text" placeholder="text input" />
<input type="password" placeholder="password" />
form>
body>
<script>
const form = document.getElementById("form");
form.addEventListener(
"blur",
(event) => {
event.target.style.background = "green";
},
true,
);
script>
head>
html>

3.3、扩展(focusin、focusout)
- 当元素即将获取焦点时,focusin 事件被触发。focusin 事件和 focus 事件之间的主要区别在于后者不会冒泡。
- 当元素即将失去焦点时,focusout 事件被触发。focusout 事件和 blur 事件之间的主要区别在于后者不会冒泡。
四、加载事件
4.1、加载完成(onload)
- load事件在页面或某个资源加载成功时触发。
- 注意,页面或资源从浏览器缓存加载,并不会触发load事件。
- 在 html 页面中把 js 写在 head 里面
<html>
<head>
<meta charset="UTF-8" />
<script>
window.onload = function () {
}
script>
head>
<body>
<div>div>
body>
html>
- 在 html 页面中把 js 写在 body 最后面
<html>
<head>
<meta charset="UTF-8" />
head>
<body>
<div>div>
<script>
window.onload = function () {
}
script>
body>
html>
4.2、加载失败(onerror)
onerror
:比如加载图像时发生错误,则会触发该事件
DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>title>
head>
<body>
<img src="image.gif" onerror="imgError()">
<p>这里我们引用了不存在的图像,因此发生了 onerror 事件。p>
body>
<script>
function imgError() {
console.log('无法加载图像。');
}
script>
head>
html>
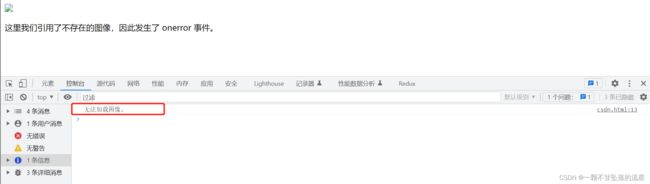
4.3、窗口变化(onresize)
onresize
:文档尺寸发生改变时就会触发改事件
DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>title>
head>
<body onresize="myFunction()">
<p>请尝试调整浏览器窗口的大小。p>
body>
<script>
function myFunction() {
var w = window.outerWidth;
var h = window.outerHeight;
console.log("当前窗口大小: width=" + w + ", height=" + h)
}
script>
head>
html>
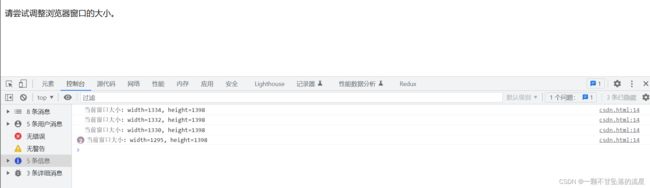