文章目录
-
- 一:赛车游戏动画
-
-
- `HTML`源码:
- `JS`源码:
- `CSS`源码:
-
- (1)`normalize.css`
- (2)`style.css`
- 二:吉普车动画演示
-
-
- `HTML`源码:
- `CSS`源码:
-
- (1)`reset.css`
- (2)`style.css`
一:赛车游戏动画
运行效果:键盘的方向键(上、下、左、右)分别控制赛车的前进、减速、向左和向右
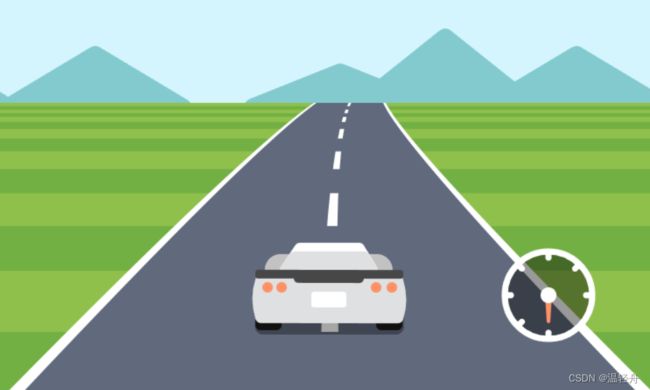
HTML
源码:
DOCTYPE html>
<html lang="zh">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge,chrome=1">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>赛车游戏动画title>
<link rel="stylesheet" type="text/css" href="css/normalize.css" />
<link rel="stylesheet" href="css/style.css">
head>
<body>
<canvas height="450" width="750">canvas>
<script type="text/javascript" src="js/main.js">script>
<div style="text-align:center;">
div>
body>
html>
JS
源码:
var $ = {
canvas: null,
ctx: null,
canvas2: null,
ctx2: null,
colors: {
sky: "#D4F5FE",
mountains: "#83CACE",
ground: "#8FC04C",
groundDark: "#73B043",
road: "#606a7c",
roadLine: "#FFF",
hud: "#FFF"
},
settings: {
fps: 60,
skySize: 120,
ground: {
size: 350,
min: 4,
max: 120
},
road: {
min: 76,
max: 700,
}
},
state: {
bgpos: 0,
offset: 0,
startDark: true,
curve: 0,
currentCurve: 0,
turn: 1,
speed: 27,
xpos: 0,
section: 50,
car: {
maxSpeed: 50,
friction: 0.4,
acc: 0.85,
deAcc: 0.5
},
keypress: {
up: false,
left: false,
right: false,
down: false
}
},
storage: {
bg: null
}
};
$.canvas = document.getElementsByTagName('canvas')[0];
$.ctx = $.canvas.getContext('2d');
$.canvas2 = document.createElement('canvas');
$.canvas2.width = $.canvas.width;
$.canvas2.height = $.canvas.height;
$.ctx2 = $.canvas2.getContext('2d');
window.addEventListener("keydown", keyDown, false);
window.addEventListener("keyup", keyUp, false);
drawBg();
draw();
function draw() {
setTimeout(function() {
calcMovement();
$.state.bgpos += ($.state.currentCurve * 0.02) * ($.state.speed * 0.2);
$.state.bgpos = $.state.bgpos % $.canvas.width;
$.ctx.putImageData($.storage.bg, $.state.bgpos, 5);
$.ctx.putImageData($.storage.bg, $.state.bgpos > 0 ? $.state.bgpos - $.canvas.width : $.state.bgpos + $.canvas.width, 5);
$.state.offset += $.state.speed * 0.05;
if($.state.offset > $.settings.ground.min) {
$.state.offset = $.settings.ground.min - $.state.offset;
$.state.startDark = !$.state.startDark;
}
drawGround($.ctx, $.state.offset, $.colors.ground, $.colors.groundDark, $.canvas.width);
drawRoad($.settings.road.min + 6, $.settings.road.max + 36, 10, $.colors.roadLine);
drawGround($.ctx2, $.state.offset, $.colors.roadLine, $.colors.road, $.canvas.width);
drawRoad($.settings.road.min, $.settings.road.max, 10, $.colors.road);
drawRoad(3, 24, 0, $.ctx.createPattern($.canvas2, 'repeat'));
drawCar();
drawHUD($.ctx, 630, 340, $.colors.hud);
requestAnimationFrame(draw);
}, 1000 / $.settings.fps);
}
function drawHUD(ctx, centerX, centerY, color) {
var radius = 50, tigs = [0, 90, 135, 180, 225, 270, 315],
angle = 90;
ctx.beginPath();
ctx.arc(centerX, centerY, radius, 0, 2 * Math.PI, false);
ctx.lineWidth = 7;
ctx.fillStyle = 'rgba(0, 0, 0, 0.4)';
ctx.fill();
ctx.strokeStyle = color;
ctx.stroke();
for (var i = 0; i < tigs.length; i++) {
drawTig(ctx, centerX, centerY, radius, tigs[i], 7);
}
angle = map($.state.speed, 0, $.state.car.maxSpeed, 90, 360);
drawPointer(ctx, color, 50, centerX, centerY, angle);
}
function drawPointer(ctx, color, radius, centerX, centerY, angle) {
var point = getCirclePoint(centerX, centerY, radius - 20, angle),
point2 = getCirclePoint(centerX, centerY, 2, angle + 90),
point3 = getCirclePoint(centerX, centerY, 2, angle - 90);
ctx.beginPath();
ctx.strokeStyle = "#FF9166";
ctx.lineCap = 'round';
ctx.lineWidth = 4;
ctx.moveTo(point2.x, point2.y);
ctx.lineTo(point.x, point.y);
ctx.lineTo(point3.x, point3.y);
ctx.stroke();
ctx.beginPath();
ctx.arc(centerX, centerY, 9, 0, 2 * Math.PI, false);
ctx.fillStyle = color;
ctx.fill();
}
function drawTig(ctx, x, y, radius, angle, size) {
var startPoint = getCirclePoint(x, y, radius - 4, angle),
endPoint = getCirclePoint(x, y, radius - size, angle)
ctx.beginPath();
ctx.lineCap = 'round';
ctx.moveTo(startPoint.x, startPoint.y);
ctx.lineTo(endPoint.x, endPoint.y);
ctx.stroke();
}
function getCirclePoint(x, y, radius, angle) {
var radian = (angle / 180) * Math.PI;
return {
x: x + radius * Math.cos(radian),
y: y + radius * Math.sin(radian)
}
}
function calcMovement() {
var move = $.state.speed * 0.01,
newCurve = 0;
if($.state.keypress.up) {
$.state.speed += $.state.car.acc - ($.state.speed * 0.015);
} else if ($.state.speed > 0) {
$.state.speed -= $.state.car.friction;
}
if($.state.keypress.down && $.state.speed > 0) {
$.state.speed -= 1;
}
$.state.xpos -= ($.state.currentCurve * $.state.speed) * 0.005;
if($.state.speed) {
if($.state.keypress.left) {
$.state.xpos += (Math.abs($.state.turn) + 7 + ($.state.speed > $.state.car.maxSpeed / 4 ? ($.state.car.maxSpeed - ($.state.speed / 2)) : $.state.speed)) * 0.2;
$.state.turn -= 1;
}
if($.state.keypress.right) {
$.state.xpos -= (Math.abs($.state.turn) + 7 + ($.state.speed > $.state.car.maxSpeed / 4 ? ($.state.car.maxSpeed - ($.state.speed / 2)) : $.state.speed)) * 0.2;
$.state.turn += 1;
}
if($.state.turn !== 0 && !$.state.keypress.left && !$.state.keypress.right) {
$.state.turn += $.state.turn > 0 ? -0.25 : 0.25;
}
}
$.state.turn = clamp($.state.turn, -5, 5);
$.state.speed = clamp($.state.speed, 0, $.state.car.maxSpeed);
$.state.section -= $.state.speed;
if($.state.section < 0) {
$.state.section = randomRange(1000, 9000);
newCurve = randomRange(-50, 50);
if(Math.abs($.state.curve - newCurve) < 20) {
newCurve = randomRange(-50, 50);
}
$.state.curve = newCurve;
}
if($.state.currentCurve < $.state.curve && move < Math.abs($.state.currentCurve - $.state.curve)) {
$.state.currentCurve += move;
} else if($.state.currentCurve > $.state.curve && move < Math.abs($.state.currentCurve - $.state.curve)) {
$.state.currentCurve -= move;
}
if(Math.abs($.state.xpos) > 550) {
$.state.speed *= 0.96;
}
$.state.xpos = clamp($.state.xpos, -650, 650);
}
function keyUp(e) {
move(e, false);
}
function keyDown(e) {
move(e, true);
}
function move(e, isKeyDown) {
if(e.keyCode >= 37 && e.keyCode <= 40) {
e.preventDefault();
}
if(e.keyCode === 37) {
$.state.keypress.left = isKeyDown;
}
if(e.keyCode === 38) {
$.state.keypress.up = isKeyDown;
}
if(e.keyCode === 39) {
$.state.keypress.right = isKeyDown;
}
if(e.keyCode === 40) {
$.state.keypress.down = isKeyDown;
}
}
function randomRange(min, max) {
return min + Math.random() * (max - min);
}
function norm(value, min, max) {
return (value - min) / (max - min);
}
function lerp(norm, min, max) {
return (max - min) * norm + min;
}
function map(value, sourceMin, sourceMax, destMin, destMax) {
return lerp(norm(value, sourceMin, sourceMax), destMin, destMax);
}
function clamp(value, min, max) {
return Math.min(Math.max(value, min), max);
}
function drawBg() {
$.ctx.fillStyle = $.colors.sky;
$.ctx.fillRect(0, 0, $.canvas.width, $.settings.skySize);
drawMountain(0, 60, 200);
drawMountain(280, 40, 200);
drawMountain(400, 80, 200);
drawMountain(550, 60, 200);
$.storage.bg = $.ctx.getImageData(0, 0, $.canvas.width, $.canvas.height);
}
function drawMountain(pos, height, width) {
$.ctx.fillStyle = $.colors.mountains;
$.ctx.strokeStyle = $.colors.mountains;
$.ctx.lineJoin = "round";
$.ctx.lineWidth = 20;
$.ctx.beginPath();
$.ctx.moveTo(pos, $.settings.skySize);
$.ctx.lineTo(pos + (width / 2), $.settings.skySize - height);
$.ctx.lineTo(pos + width, $.settings.skySize);
$.ctx.closePath();
$.ctx.stroke();
$.ctx.fill();
}
function drawSky() {
$.ctx.fillStyle = $.colors.sky;
$.ctx.fillRect(0, 0, $.canvas.width, $.settings.skySize);
}
function drawRoad(min, max, squishFactor, color) {
var basePos = $.canvas.width + $.state.xpos;
$.ctx.fillStyle = color;
$.ctx.beginPath();
$.ctx.moveTo(((basePos + min) / 2) - ($.state.currentCurve * 3), $.settings.skySize);
$.ctx.quadraticCurveTo((((basePos / 2) + min)) + ($.state.currentCurve / 3) + squishFactor, $.settings.skySize + 52, (basePos + max) / 2, $.canvas.height);
$.ctx.lineTo((basePos - max) / 2, $.canvas.height);
$.ctx.quadraticCurveTo((((basePos / 2) - min)) + ($.state.currentCurve / 3) - squishFactor, $.settings.skySize + 52, ((basePos - min) / 2) - ($.state.currentCurve * 3), $.settings.skySize);
$.ctx.closePath();
$.ctx.fill();
}
function drawCar() {
var carWidth = 160,
carHeight = 50,
carX = ($.canvas.width / 2) - (carWidth / 2),
carY = 320;
roundedRect($.ctx, "rgba(0, 0, 0, 0.35)", carX - 1 + $.state.turn, carY + (carHeight - 35), carWidth + 10, carHeight, 9);
roundedRect($.ctx, "#111", carX, carY + (carHeight - 30), 30, 40, 6);
roundedRect($.ctx, "#111", (carX - 22) + carWidth, carY + (carHeight - 30), 30, 40, 6);
drawCarBody($.ctx);
}
function drawCarBody(ctx) {
var startX = 299, startY = 311,
lights = [10, 26, 134, 152],
lightsY = 0;
roundedRect($.ctx, "#C2C2C2", startX + 6 + ($.state.turn * 1.1), startY - 18, 146, 40, 18);
ctx.beginPath();
ctx.lineWidth="12";
ctx.fillStyle="#FFFFFF";
ctx.strokeStyle="#FFFFFF";
ctx.moveTo(startX + 30, startY);
ctx.lineTo(startX + 46 + $.state.turn, startY - 25);
ctx.lineTo(startX + 114 + $.state.turn, startY - 25);
ctx.lineTo(startX + 130, startY);
ctx.fill();
ctx.stroke();
ctx.lineWidth="12";
ctx.lineCap = 'round';
ctx.beginPath();
ctx.fillStyle="#DEE0E2";
ctx.strokeStyle="#DEE0E2";
ctx.moveTo(startX + 2, startY + 12 + ($.state.turn * 0.2));
ctx.lineTo(startX + 159, startY + 12 + ($.state.turn * 0.2));
ctx.quadraticCurveTo(startX + 166, startY + 35, startX + 159, startY + 55 + ($.state.turn * 0.2));
ctx.lineTo(startX + 2, startY + 55 - ($.state.turn * 0.2));
ctx.quadraticCurveTo(startX - 5, startY + 32, startX + 2, startY + 12 - ($.state.turn * 0.2));
ctx.fill();
ctx.stroke();
ctx.beginPath();
ctx.lineWidth="12";
ctx.fillStyle="#DEE0E2";
ctx.strokeStyle="#DEE0E2";
ctx.moveTo(startX + 30, startY);
ctx.lineTo(startX + 40 + ($.state.turn * 0.7), startY - 15);
ctx.lineTo(startX + 120 + ($.state.turn * 0.7), startY - 15);
ctx.lineTo(startX + 130, startY);
ctx.fill();
ctx.stroke();
roundedRect(ctx, "#474747", startX - 4, startY, 169, 10, 3, true, 0.2);
roundedRect(ctx, "#474747", startX + 40, startY + 5, 80, 10, 5, true, 0.1);
ctx.fillStyle = "#FF9166";
lights.forEach(function(xPos) {
ctx.beginPath();
ctx.arc(startX + xPos, startY + 20 + lightsY, 6, 0, Math.PI*2, true);
ctx.closePath();
ctx.fill();
lightsY += $.state.turn * 0.05;
});
ctx.lineWidth="9";
ctx.fillStyle="#222222";
ctx.strokeStyle="#444";
roundedRect($.ctx, "#FFF", startX + 60, startY + 25, 40, 18, 3, true, 0.05);
}
function roundedRect(ctx, color, x, y, width, height, radius, turn, turneffect) {
var skew = turn === true ? $.state.turn * turneffect : 0;
ctx.fillStyle = color;
ctx.beginPath();
ctx.moveTo(x + radius, y - skew);
ctx.lineTo(x + width - radius, y + skew);
ctx.arcTo(x + width, y + skew, x + width, y + radius + skew, radius);
ctx.lineTo(x + width, y + radius + skew);
ctx.lineTo(x + width, (y + height + skew) - radius);
ctx.arcTo(x + width, y + height + skew, (x + width) - radius, y + height + skew, radius);
ctx.lineTo((x + width) - radius, y + height + skew);
ctx.lineTo(x + radius, y + height - skew);
ctx.arcTo(x, y + height - skew, x, (y + height - skew) - radius, radius);
ctx.lineTo(x, (y + height - skew) - radius);
ctx.lineTo(x, y + radius - skew);
ctx.arcTo(x, y - skew, x + radius, y - skew, radius);
ctx.lineTo(x + radius, y - skew);
ctx.fill();
}
function drawGround(ctx, offset, lightColor, darkColor, width) {
var pos = ($.settings.skySize - $.settings.ground.min) + offset, stepSize = 1, drawDark = $.state.startDark, firstRow = true;
ctx.fillStyle = lightColor;
ctx.fillRect(0, $.settings.skySize, width, $.settings.ground.size);
ctx.fillStyle = darkColor;
while(pos <= $.canvas.height) {
stepSize = norm(pos, $.settings.skySize, $.canvas.height) * $.settings.ground.max;
if(stepSize < $.settings.ground.min) {
stepSize = $.settings.ground.min;
}
if(drawDark) {
if(firstRow) {
ctx.fillRect(0, $.settings.skySize, width, stepSize - (offset > $.settings.ground.min ? $.settings.ground.min : $.settings.ground.min - offset));
} else {
ctx.fillRect(0, pos < $.settings.skySize ? $.settings.skySize : pos, width, stepSize);
}
}
firstRow = false;
pos += stepSize;
drawDark = !drawDark;
}
}
CSS
源码:
(1)normalize.css
article,aside,details,figcaption,figure,footer,header,hgroup,main,nav,section,summary{display:block;}audio,canvas,video{display:inline-block;}audio:not([controls]){display:none;height:0;}[hidden]{display:none;}html{font-family:sans-serif;-ms-text-size-adjust:100%;-webkit-text-size-adjust:100%;}body{margin:0;}a:focus{outline:thin dotted;}a:active,a:hover{outline:0;}h1{font-size:2em;margin:0.67em 0;}abbr[title]{border-bottom:1px dotted;}b,strong{font-weight:bold;}dfn{font-style:italic;}hr{-moz-box-sizing:content-box;box-sizing:content-box;height:0;}mark{background:#ff0;color:#000;}code,kbd,pre,samp{font-family:monospace,serif;font-size:1em;}pre{white-space:pre-wrap;}q{quotes:"\201C" "\201D" "\2018" "\2019";}small{font-size:80%;}sub,sup{font-size:75%;line-height:0;position:relative;vertical-align:baseline;}sup{top:-0.5em;}sub{bottom:-0.25em;}img{border:0;}svg:not(:root){overflow:hidden;}figure{margin:0;}fieldset{border:1px solid #c0c0c0;margin:0 2px;padding:0.35em 0.625em 0.75em;}legend{border:0;padding:0;}button,input,select,textarea{font-family:inherit;font-size:100%;margin:0;}button,input{line-height:normal;}button,select{text-transform:none;}button,html input[type="button"],input[type="reset"],input[type="submit"]{-webkit-appearance:button;cursor:pointer;}button[disabled],html input[disabled]{cursor:default;}input[type="checkbox"],input[type="radio"]{box-sizing:border-box;padding:0;}input[type="search"]{-webkit-appearance:textfield;-moz-box-sizing:content-box;-webkit-box-sizing:content-box;box-sizing:content-box;}input[type="search"]::-webkit-search-cancel-button,input[type="search"]::-webkit-search-decoration{-webkit-appearance:none;}button::-moz-focus-inner,input::-moz-focus-inner{border:0;padding:0;}textarea{overflow:auto;vertical-align:top;}table{border-collapse:collapse;border-spacing:0;}
(2)style.css
body {
background-color: #F8F3A9;
margin: 0;
display: -webkit-box;
display: -webkit-flex;
display: -ms-flexbox;
display: flex;
-webkit-box-align: center;
-webkit-align-items: center;
-ms-flex-align: center;
align-items: center;
-webkit-box-pack: center;
-webkit-justify-content: center;
-ms-flex-pack: center;
justify-content: center;
min-height: 100vh;
}
canvas {
border-radius: .4em;
}
二:吉普车动画演示
运行效果:动态动画演示
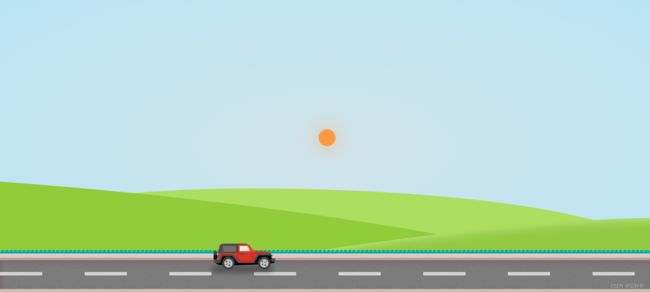
HTML
源码:
DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>吉普车动画演示title>
<style>
html,body,div,span,applet,object,iframe,h1,h2,h3,h4,h5,h6,p,blockquote,pre,a,abbr,acronym,address,big,cite,code,del,dfn,em,img,ins,kbd,q,s,samp,small,strike,strong,sub,sup,tt,var,b,u,i,center,dl,dt,dd,ol,ul,li,fieldset,form,label,legend,table,caption,tbody,tfoot,thead,tr,th,td,article,aside,canvas,details,embed,figure,figcaption,footer,header,hgroup,menu,nav,output,ruby,section,summary,time,mark,audio,video{margin:0;padding:0;border:0;font-size:100%;font:inherit;vertical-align:baseline}article,aside,details,figcaption,figure,footer,header,hgroup,menu,nav,section{display:block}body{line-height:1}ol,ul{list-style:none}blockquote,q{quotes:none}blockquote:before,blockquote:after,q:before,q:after{content:'';content:none}table{border-collapse:collapse;border-spacing:0}
style>
<style>
@charset "utf-8";
body, html {
height: 100%;
}
body {
background: rgb(209,228,234);
background: -moz-radial-gradient(center, ellipse cover, rgba(209,228,234,1) 0%, rgba(186,228,244,1) 100%);
background: -webkit-gradient(radial, center center, 0px, center center, 100%, color-stop(0%,rgba(209,228,234,1)), color-stop(100%,rgba(186,228,244,1)));
background: -webkit-radial-gradient(center, ellipse cover, rgba(209,228,234,1) 0%,rgba(186,228,244,1) 100%);
background: -o-radial-gradient(center, ellipse cover, rgba(209,228,234,1) 0%,rgba(186,228,244,1) 100%);
background: -ms-radial-gradient(center, ellipse cover, rgba(209,228,234,1) 0%,rgba(186,228,244,1) 100%);
background: radial-gradient(ellipse at center, rgba(209,228,234,1) 0%,rgba(186,228,244,1) 100%);
filter: progid:DXImageTransform.Microsoft.gradient( startColorstr='#d1e4ea', endColorstr='#bae4f4',GradientType=1 );
padding:0;
margin:0;
}
.country-wrap {
position:relative;
width:100%;
height:100%;
overflow:hidden;
}
.push-bottom {
position:absolute;
bottom:0;
height:100%;
}
.bottom-ground {
background:#8d773e;
width:102%;
left:-1%;
height:60px;
bottom:0;
position:absolute;
box-shadow:0 2px 3px rgba(0,0,0,0.2) inset;
}
.street {
background:#7a7a7a url(data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAADIAAAAyCAMAAAAp4XiDAAAAUVBMVEWFhYWDg4N3d3dtbW17e3t1dXWBgYGHh4d5eXlzc3OLi4ubm5uVlZWPj4+NjY19fX2JiYl/f39ra2uRkZGZmZlpaWmXl5dvb29xcXGTk5NnZ2c8TV1mAAAAG3RSTlNAQEBAQEBAQEBAQEBAQEBAQEBAQEBAQEBAQEAvEOwtAAAFVklEQVR4XpWWB67c2BUFb3g557T/hRo9/WUMZHlgr4Bg8Z4qQgQJlHI4A8SzFVrapvmTF9O7dmYRFZ60YiBhJRCgh1FYhiLAmdvX0CzTOpNE77ME0Zty/nWWzchDtiqrmQDeuv3powQ5ta2eN0FY0InkqDD73lT9c9lEzwUNqgFHs9VQce3TVClFCQrSTfOiYkVJQBmpbq2L6iZavPnAPcoU0dSw0SUTqz/GtrGuXfbyyBniKykOWQWGqwwMA7QiYAxi+IlPdqo+hYHnUt5ZPfnsHJyNiDtnpJyayNBkF6cWoYGAMY92U2hXHF/C1M8uP/ZtYdiuj26UdAdQQSXQErwSOMzt/XWRWAz5GuSBIkwG1H3FabJ2OsUOUhGC6tK4EMtJO0ttC6IBD3kM0ve0tJwMdSfjZo+EEISaeTr9P3wYrGjXqyC1krcKdhMpxEnt5JetoulscpyzhXN5FRpuPHvbeQaKxFAEB6EN+cYN6xD7RYGpXpNndMmZgM5Dcs3YSNFDHUo2LGfZuukSWyUYirJAdYbF3MfqEKmjM+I2EfhA94iG3L7uKrR+GdWD73ydlIB+6hgref1QTlmgmbM3/LeX5GI1Ux1RWpgxpLuZ2+I+IjzZ8wqE4nilvQdkUdfhzI5QDWy+kw5Wgg2pGpeEVeCCA7b85BO3F9DzxB3cdqvBzWcmzbyMiqhzuYqtHRVG2y4x+KOlnyqla8AoWWpuBoYRxzXrfKuILl6SfiWCbjxoZJUaCBj1CjH7GIaDbc9kqBY3W/Rgjda1iqQcOJu2WW+76pZC9QG7M00dffe9hNnseupFL53r8F7YHSwJWUKP2q+k7RdsxyOB11n0xtOvnW4irMMFNV4H0uqwS5ExsmP9AxbDTc9JwgneAT5vTiUSm1E7BSflSt3bfa1tv8Di3R8n3Af7MNWzs49hmauE2wP+ttrq+AsWpFG2awvsuOqbipWHgtuvuaAE+A1Z/7gC9hesnr+7wqCwG8c5yAg3AL1fm8T9AZtp/bbJGwl1pNrE7RuOX7PeMRUERVaPpEs+yqeoSmuOlokqw49pgomjLeh7icHNlG19yjs6XXOMedYm5xH2YxpV2tc0Ro2jJfxC50ApuxGob7lMsxfTbeUv07TyYxpeLucEH1gNd4IKH2LAg5TdVhlCafZvpskfncCfx8pOhJzd76bJWeYFnFciwcYfubRc12Ip/ppIhA1/mSZ/RxjFDrJC5xifFjJpY2Xl5zXdguFqYyTR1zSp1Y9p+tktDYYSNflcxI0iyO4TPBdlRcpeqjK/piF5bklq77VSEaA+z8qmJTFzIWiitbnzR794USKBUaT0NTEsVjZqLaFVqJoPN9ODG70IPbfBHKK+/q/AWR0tJzYHRULOa4MP+W/HfGadZUbfw177G7j/OGbIs8TahLyynl4X4RinF793Oz+BU0saXtUHrVBFT/DnA3ctNPoGbs4hRIjTok8i+algT1lTHi4SxFvONKNrgQFAq2/gFnWMXgwffgYMJpiKYkmW3tTg3ZQ9Jq+f8XN+A5eeUKHWvJWJ2sgJ1Sop+wwhqFVijqWaJhwtD8MNlSBeWNNWTa5Z5kPZw5+LbVT99wqTdx29lMUH4OIG/D86ruKEauBjvH5xy6um/Sfj7ei6UUVk4AIl3MyD4MSSTOFgSwsH/QJWaQ5as7ZcmgBZkzjjU1UrQ74ci1gWBCSGHtuV1H2mhSnO3Wp/3fEV5a+4wz//6qy8JxjZsmxxy5+4w9CDNJY09T072iKG0EnOS0arEYgXqYnXcYHwjTtUNAcMelOd4xpkoqiTYICWFq0JSiPfPDQdnt+4/wuqcXY47QILbgAAAABJRU5ErkJggg==);
height:80px;
width:102%;
position:absolute;
bottom:0;
box-shadow:0 1px 16px rgba(111, 35, 51, 0.4) inset;
}
.street:after {
content:'';
display:block;
position:absolute;
width:100%;
height:10px;
background:#cdbcb4;
bottom:0;
border-bottom:3px solid #72625a;
z-index:1;
}
.street:before {
content:'';
display:block;
position:absolute;
width:100%;
height:7px;
background:#c2a99d;
bottom:-7px;
z-index:1;
}
.street-stripe {
background:#d4d4d4;
height:8px;
width:100px;
position:absolute;
bottom:44px;
border-radius:2px;
box-shadow:200px 0 0 #d4d4d4, 400px 0 0 #d4d4d4 , 600px 0 0 #d4d4d4 , 800px 0 0 #d4d4d4 , 1000px 0 0 #d4d4d4 , 1200px 0 0 #d4d4d4 , 1400px 0 0 #d4d4d4 , 1600px 0 0 #d4d4d4 , 1800px 0 0 #d4d4d4 , 2000px 0 0 #d4d4d4;
}
.grass {
height: 40px;
width: 100%;
background-color: #dbcac2;
position:absolute;
bottom:60px;
}
.grass:before {
position: absolute;
content: '';
top: 14px;
left: 0;
height: 8px;
width: 100%;
background-color: #b99f93;
}
.grass:after {
position: absolute;
content: '';
top: -4px;
left: 0;
width: 100%;
height: 8px;
background-color: #0aa;
background: linear-gradient(135deg, transparent 25%, #0aa 25%);
background-size:10px 10px;
}
.sun {
background:#ff9944;
width:40px;
height:40px;
border-radius:50%;
box-shadow:0 0 50px rgba(255,153,68,0.7);
position:absolute;
left:49%;
bottom:350px;
}
.tree-1 {
position:absolute;
z-index:2;
bottom:210px;
right:10px;
width:50px;
height:100px;
}
.trunk {
width:20%;
height:30%;
background:brown;
}
.branch {
position:abslute;
width:60%;
height:30%;
background:green;
-moz-transform:rotate(45deg);
border-radius:0 0 100% 0;
}
.branch-1 {
border:50px solid;
border-bottom:90px solid;
border-color:transparent transparent #a5894a transparent;
height: 0;
width: 0;
position:absolute;
left:-50px;
top:-70px;
}
.branch-2 {
border-bottom: 40px solid #9d8346;
border-left: 30px solid transparent;
border-right: 30px solid transparent;
height: 0;
width: 100px;
position:absolute;
top:60px;
left:-80px;
}
.branch-3 {
border-bottom: 50px solid #90713b;
border-left: 40px solid transparent;
border-right: 40px solid transparent;
height: 0;
width: 100px;
position:absolute;
top:100px;
left:-90px;
}
.mountain-1 {
background:#cea392;
width:500px;
height:500px;
position:absolute;
-moz-transform:rotate(45deg);
-webkit-transform:rotate(45deg);
bottom:-150px;
border-radius:40px;
}
.mountain-2 {
background:#e4dbd2;
width:800px;
height:800px;
position:absolute;
-moz-transform:rotate(45deg);
-webkit-transform:rotate(45deg);
bottom:-350px;
border-radius:40px;
left:250px;
z-index:-1;
box-shadow: 0 0 25px #e4dbd2;
opacity:0.6;
}
.la {
position: absolute;
bottom: 200px;
width: 2px;
height: 50px;
background: $cd;
margin-right: 20px;
}
.la:before {
top: 5px;
left: -10px;
width: 22px;
height: 2px;
background: $cd;
}
.la:after {
bottom: 0;
left: -2px;
width: 6px;
height: 12px;
background: $cd;
}
.l {
position: absolute;
width: 5px;
height: 5px;
border-radius: 50%;
background: #fff;
box-shadow: 0 0 10px #fff, 0 0 25px #fff, 0 0 50px #fff;
}
.l:nth-child(1) { left: -10px; }
.l:nth-child(2) { right: -10px; }
.car {
position:absolute;
top:-35%;
z-index:10;
-moz-animation:myfirst 10s linear infinite;
-webkit-animation:myfirst 10s linear infinite;
}
@-moz-keyframes myfirst
{
0% {left:-25%;}
100% {left:100%;}
}
@-webkit-keyframes myfirst
{
0% {left:-25%;}
100% {left:100%;}
}
.tyre {
width:30px;
height:30px;
border-radius:50%;
background:#3f3f40;
position:absolute;
z-index:2;
left:9px;
top:20px;
-moz-animation:tyre-rotate 1s infinite linear;
-webkit-animation:tyre-rotate 1s infinite linear;
}
@-moz-keyframes tyre-rotate {
from{-moz-transform:rotate(-360deg);}
to{-moz-transform:rotate(0deg);}
}
@-webkit-keyframes tyre-rotate {
from{-webkit-transform:rotate(-360deg);}
to{-webkit-transform:rotate(0deg);}
}
.tyre:before {
content:'';
width:20px;
height:20px;
border-radius:50%;
background:#bdc2bd;
position:absolute;
top:5px;
left:5px;
}
.gap {
background:#3f3f40;
width:2px;
height:4px;
position:absolute;
left:14px;
top:8px;
box-shadow:0 9px 0 #3f3f40;
}
.gap:before {
content:'';
display:block;
width:2px;
height:4px;
position:absolute;
background:#3f3f40;
box-shadow:0 12px 0 #3f3f40;
-webkit-transform:rotate(-90deg);
-webkit-transform-origin:0 7px;
-moz-transform:rotate(-90deg);
-moz-transform-origin:0 7px;
z-index:3;
}
.car-base {
position:absolute;
display:block;
width: 125px;
height: 30px;
background:#000000;
border-radius: 10% 10% 50% 50% / 60% 100% 20% 10%;
-webkit-transform:rotate(-2deg);
-moz-transform:rotate(-2deg);
border:solid;
border-color:#000000;
}
.back-bonet {
background: #4c4b4b;
border-radius: 54% 25% 0 0;
height: 22px;
left: 11px;
position: absolute;
top: 8px;
width: 40px;
}
.tyre.front {
left:94px;
}
.car-body {
border-bottom: 24px solid #d1352b;
height: 0;
top:10px;
width: 120px;
position:relative;
}
.car-body:before {
content:'';
display:inline-block;
width:30px;
height:24px;
position:absolute;
right:-5px;
background:#d1352b;
border-top-right-radius:4px;
z-index:1;
}
.car-body:after {
content:'';
display:inline-block;
width:121px;
border-bottom: 1px solid #942b25;
border-right: 2px solid transparent;
height: 0;
z-index:2;
position:absolute;
}
.car-gate {
width:32px;
height:20px;
background:#d1352b;
border-radius:0 0 2px 8px / 0 0 2px 8px;
box-shadow:0 0 0 1px #892924;
position:absolute;
left:48px;
}
.car-gate:before {
content:'';
width:8px;
height:2px;
background:#4c4b4b;
position:absolute;
top:2px;
left:4px;
box-shadow:1px 1px 1px rgba(0,0,0,0.1);
}
.car-top-back {
background: none repeat scroll 0 0 #4C4B4B;
border-radius: 5px 0 0 0;
height: 20px;
left: 4px;
position: absolute;
top: -20px;
width: 58px;
}
.car-top-back:before {
width:30px;
height:15px;
background:#736f6f;
content:'';
position:absolute;
top:3px;
left:8px;
border-radius:2px;
}
.car-top-back:after {
content:'';
background:#4c4b4b;
border-radius: 30%;
height: 5px;
left: 3px;
position: absolute;
top: -1px;
width: 62px;
}
.car-top-front {
top:-19px;
position:absolute;
left:47px;
width:20px;
height:20px;
background:#dc4630;
border-left: 1px solid #892924;
border-radius: 2px 0 0 0;
}
.car-top-front:after {
width:26px;
height:20px;
-webkit-transform:skew(37deg);
-moz-transform:skew(37deg);
background:#dc4630;
content:'';
position:absolute;
top:0;
left:6px;
border-radius:4px 0 4px 4px;
}
.car-top-front:before {
width:12px;
height:5px;
background:#dc4630;
content:'';
position:absolute;
top:14px;
left:28px;
z-index:1;
border:solid #a82e27;
border-width:0 1px 1px 0;
}
.wind-sheild {
top:3px;
left:3px;
position:absolute;
z-index:3;
width:18px;
height:12px;
background:#f5e7e7;
border-radius:0 3px 0 0;
}
.wind-sheild:after {
width:12px;
height:12px;
-webkit-transform:skew(25deg);
-moz-transform:skew(25deg);
background:#f5e7e7;
content:'';
position:absolute;
top:0;
left:10px;
border-radius:3px;
}
.boundary-tyre-cover {
position:absolute;
top:14px; left:10px;
border-bottom: 20px solid #4c4b4b;
border-right: 10px solid transparent;
height:0;
width:20px;
}
.boundary-tyre-cover:before {
content:'';
position:absolute;
display:inline-block;
background:#4c4b4b;
height:20px;
width:15px;
-webkit-transform:skewX(-20deg);
-moz-transform:skewX(-20deg);
border-radius:3px;
left:-6px;
top:0;
}
.boundary-tyre-cover:after {
content:'';
position:absolute;
display:inline-block;
background:#4c4b4b;
height:20px;
width:20px;
-webkit-transform:skewx(40deg);
-moz-transform:skewX(40deg);
border-radius:3px;
right:-14px;
top:0;
}
.boundary-tyre-cover-inner {
position:absolute;
top:4px; left:4px;
border-bottom: 16px solid black;
border-right: 10px solid transparent;
height:0;
width:15px;
z-index:2;
}
.boundary-tyre-cover-inner:before {
content:'';
position:absolute;
display:inline-block;
background:black;
height:16px;
width:15px;
-webkit-transform:skewX(-20deg);
-moz-transform:skewX(-20deg);
border-radius:3px 3px 0 0;
left:-6px;
top:0;
}
.boundary-tyre-cover-inner:after {
content:'';
position:absolute;
display:inline-block;
background:black;
height:16px;
width:20px;
-webkit-transform:skewx(40deg);
-moz-transform:skewX(40deg);
border-radius:3px 3px 0 0;
right:-11px;
top:0;
}
.boundary-tyre-cover-back-bottom {
position: absolute;
width: 14px;
height: 8px;
background: #4c4b4b;
top: 12px;
left: -19px;
}
.bonet-front {
background: #d1352b;
border-radius: 5px 258px 0 38px / 36px 50px 0 0;
height: 4px;
position: absolute;
right: 0;
top: -4px;
width: 40px;
z-index: 0;
}
.back-curve {
background: none repeat scroll 0 0 #4C4B4B;
border-radius: 960% 100% 0 0;
height: 20px;
left: -3px;
position: absolute;
top: 1px;
transform: rotate(6deg);
width: 5px;
}
.stepney {
height: 6px;
left: -4px;
position: absolute;
top: 6px;
width: 8px;
z-index: -1;
background:#3f3f40;
}
.stepney:before {
width:8px;
height:12px;
background:#3f3f40;
content:'';
position:absolute;
top:-10px;
left:-7px;
border-radius:3px 3px 0 0;
}
.stepney:after {
width:8px;
height:12px;
background:#0d0c0d;
content:'';
position:absolute;
top:0px;
left:-7px;
border-radius:0 0 3px 3px;
}
.tyre-cover-front {
background:#4c4b4b;
height: 4px;
left: 97px;
position: absolute;
top: 13px;
width: 22px;
z-index: 1;
}
.tyre-cover-front:before {
background: none repeat scroll 0 0 #4c4b4b;
content: "";
display: inline-block;
height: 21px;
left: -10px;
position: absolute;
top: 0;
transform: skewX(-30deg);
width: 10px;
z-index: 6;
border-radius:4px 0 0 0;
}
.tyre-cover-front:after {
background: none repeat scroll 0 0 #4c4b4b;
content: "";
display: inline-block;
height: 6px;
left: 14px;
position: absolute;
top: 0;
transform: skewX(30deg);
width: 17px;
z-index: 6;
border-radius:0 4px 2px 0;
}
.boundary-tyre-cover-inner-front {
position:absolute;
top:4px; left:4px;
border-bottom: 16px solid black;
border-right: 10px solid transparent;
height:0;
width:15px;
z-index:7;
}
.boundary-tyre-cover-inner-front:before {
background: none repeat scroll 0 0 #000000;
border-radius: 3px 3px 0 0;
content: "";
display: inline-block;
height: 17px;
left: -10px;
position: absolute;
top: 0;
transform: skewX(-30deg);
width: 15px;
}
.boundary-tyre-cover-inner-front:after {
content:'';
position:absolute;
display:inline-block;
background:black;
height:16px;
width:20px;
-webkit-transform:skewx(25deg);
-moz-transform:skewX(25deg);
border-radius:3px 3px 0 0;
right:-12px;
top:0;
}
.base-axcel {
background: none repeat scroll 0 0 black;
bottom: -15px;
height: 10px;
left: 30px;
position: absolute;
transform: rotate(-2deg);
width: 70px;
z-index:-1;
}
.base-axcel:before {
background: none repeat scroll 0 0 black;
border-radius: 0 0 0 10px / 0 0 0 5px;
content: "";
height: 10px;
left: -35px;
position: absolute;
top: -2px;
transform: rotate(6deg);
width: 30px;
}
.base-axcel:after {
background: none repeat scroll 0 0 black;
border-radius: 0 0 0 10px / 0 0 0 5px;
content: "";
height: 10px;
right: -33px;
position: absolute;
top: -1px;
transform: rotate(-4deg);
width: 40px;
border-radius:0 10px 5px 0;
}
.front-bumper {
background: none repeat scroll 0 0 #4c4b4b;
border-radius: 0 2px 2px 0;
height: 8px;
position: absolute;
right: -15px;
width: 11px;
z-index: 1;
-moz-transform:rotate(-5deg);
-webkit-transform:rotate(-5deg);
}
.front-bumper:before {
background: none repeat scroll 0 0 #000000;
content: "";
height: 10px;
left: -7px;
position: absolute;
transform: rotate(-22deg);
width: 9px;
z-index: 1;
}
.car-shadow {
background: none repeat scroll 0 0 rgba(0, 0, 0, 0);
bottom: -15px;
box-shadow: -5px 10px 15px 3px #000000;
left: -7px;
position: absolute;
width: 136px;
}
.noise {
position: relative;
z-index: 1;
}
.noise:before, .body-noise:before {
content: "";
position: absolute;
z-index: -1;
top:0;
bottom:0;
left:0;
right:0;
background-image: url(data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAADIAAAAyCAMAAAAp4XiDAAAAUVBMVEWFhYWDg4N3d3dtbW17e3t1dXWBgYGHh4d5eXlzc3OLi4ubm5uVlZWPj4+NjY19fX2JiYl/f39ra2uRkZGZmZlpaWmXl5dvb29xcXGTk5NnZ2c8TV1mAAAAG3RSTlNAQEBAQEBAQEBAQEBAQEBAQEBAQEBAQEBAQEAvEOwtAAAFVklEQVR4XpWWB67c2BUFb3g557T/hRo9/WUMZHlgr4Bg8Z4qQgQJlHI4A8SzFVrapvmTF9O7dmYRFZ60YiBhJRCgh1FYhiLAmdvX0CzTOpNE77ME0Zty/nWWzchDtiqrmQDeuv3powQ5ta2eN0FY0InkqDD73lT9c9lEzwUNqgFHs9VQce3TVClFCQrSTfOiYkVJQBmpbq2L6iZavPnAPcoU0dSw0SUTqz/GtrGuXfbyyBniKykOWQWGqwwMA7QiYAxi+IlPdqo+hYHnUt5ZPfnsHJyNiDtnpJyayNBkF6cWoYGAMY92U2hXHF/C1M8uP/ZtYdiuj26UdAdQQSXQErwSOMzt/XWRWAz5GuSBIkwG1H3FabJ2OsUOUhGC6tK4EMtJO0ttC6IBD3kM0ve0tJwMdSfjZo+EEISaeTr9P3wYrGjXqyC1krcKdhMpxEnt5JetoulscpyzhXN5FRpuPHvbeQaKxFAEB6EN+cYN6xD7RYGpXpNndMmZgM5Dcs3YSNFDHUo2LGfZuukSWyUYirJAdYbF3MfqEKmjM+I2EfhA94iG3L7uKrR+GdWD73ydlIB+6hgref1QTlmgmbM3/LeX5GI1Ux1RWpgxpLuZ2+I+IjzZ8wqE4nilvQdkUdfhzI5QDWy+kw5Wgg2pGpeEVeCCA7b85BO3F9DzxB3cdqvBzWcmzbyMiqhzuYqtHRVG2y4x+KOlnyqla8AoWWpuBoYRxzXrfKuILl6SfiWCbjxoZJUaCBj1CjH7GIaDbc9kqBY3W/Rgjda1iqQcOJu2WW+76pZC9QG7M00dffe9hNnseupFL53r8F7YHSwJWUKP2q+k7RdsxyOB11n0xtOvnW4irMMFNV4H0uqwS5ExsmP9AxbDTc9JwgneAT5vTiUSm1E7BSflSt3bfa1tv8Di3R8n3Af7MNWzs49hmauE2wP+ttrq+AsWpFG2awvsuOqbipWHgtuvuaAE+A1Z/7gC9hesnr+7wqCwG8c5yAg3AL1fm8T9AZtp/bbJGwl1pNrE7RuOX7PeMRUERVaPpEs+yqeoSmuOlokqw49pgomjLeh7icHNlG19yjs6XXOMedYm5xH2YxpV2tc0Ro2jJfxC50ApuxGob7lMsxfTbeUv07TyYxpeLucEH1gNd4IKH2LAg5TdVhlCafZvpskfncCfx8pOhJzd76bJWeYFnFciwcYfubRc12Ip/ppIhA1/mSZ/RxjFDrJC5xifFjJpY2Xl5zXdguFqYyTR1zSp1Y9p+tktDYYSNflcxI0iyO4TPBdlRcpeqjK/piF5bklq77VSEaA+z8qmJTFzIWiitbnzR794USKBUaT0NTEsVjZqLaFVqJoPN9ODG70IPbfBHKK+/q/AWR0tJzYHRULOa4MP+W/HfGadZUbfw177G7j/OGbIs8TahLyynl4X4RinF793Oz+BU0saXtUHrVBFT/DnA3ctNPoGbs4hRIjTok8i+algT1lTHi4SxFvONKNrgQFAq2/gFnWMXgwffgYMJpiKYkmW3tTg3ZQ9Jq+f8XN+A5eeUKHWvJWJ2sgJ1Sop+wwhqFVijqWaJhwtD8MNlSBeWNNWTa5Z5kPZw5+LbVT99wqTdx29lMUH4OIG/D86ruKEauBjvH5xy6um/Sfj7ei6UUVk4AIl3MyD4MSSTOFgSwsH/QJWaQ5as7ZcmgBZkzjjU1UrQ74ci1gWBCSGHtuV1H2mhSnO3Wp/3fEV5a+4wz//6qy8JxjZsmxxy5+4w9CDNJY09T072iKG0EnOS0arEYgXqYnXcYHwjTtUNAcMelOd4xpkoqiTYICWFq0JSiPfPDQdnt+4/wuqcXY47QILbgAAAABJRU5ErkJggg==);
}
.hill {
position: absolute;
bottom: 0;
right: 0;
width: 100%;
height: 250px;
border-top-right-radius: 160%;
border-top-left-radius: 100%;
background-color: #adde60;
z-index:-1;
}
.hill:after {
content: '';
position: absolute;
bottom: -100px;
right: -400px;
width: 120%;
height: 110%;
border-top-right-radius: 100%;
border-top-left-radius: 0%;
background-color: #94c943;
-moz-transform:rotate(-12deg);
-webkit-transform:rotate(-12deg);
box-shadow:0 0 25px #cbf191 inset;
}
.hill:before {
background-color: #93cc3a;
border-top-left-radius: 0;
border-top-right-radius: 100%;
bottom: -70px;
content: "";
height: 110%;
left: -54px;
position: absolute;
transform: rotate(4deg);
width: 120%;
}
.cloud {
background:#fff;
width:150px;
height:50px;
border-radius:50px;
position:absolute;
left:450px;
top:150px;
}
.cloud:before {
width:100px;
height:100px;
content:'';
position:absolute;
bottom:0;
left:-15px;
border-radius:50px;
box-shadow:100px 0 0 #fff;
background:#fff;
}
style>
<script src="js/prefixfree.min.js">script>
head>
<body>
<div class="country-wrap">
<div style="text-align:center;clear:both">
<script src="/gg_bd_ad_720x90.js" type="text/javascript">script>
<script src="/follow.js" type="text/javascript">script>
div>
<div class="sun">div>
<div class="grass">div>
<div class="street">
<div class="car">
<div class="car-body">
<div class="car-top-back">
<div class="back-curve">div>
div>
<div class="car-gate">div>
<div class="car-top-front">
<div class="wind-sheild">div>
div>
<div class="bonet-front">div>
<div class="stepney">div>
div>
<div class="boundary-tyre-cover">
<div class="boundary-tyre-cover-back-bottom">div>
<div class="boundary-tyre-cover-inner">div>
div>
<div class="tyre-cover-front">
<div class="boundary-tyre-cover-inner-front">div>
div>
<div class="base-axcel">
div>
<div class="front-bumper">div>
<div class="tyre">
<div class="gap">div>
div>
<div class="tyre front">
<div class="gap">div>
div>
<div class="car-shadow">div>
div>
div>
<div class="street-stripe">div>
<div class="hill">
div>
div>
<script src='js/jquery.js'>script>
body>
html>
CSS
源码:
(1)reset.css
html,body,div,span,applet,object,iframe,h1,h2,h3,h4,h5,h6,p,blockquote,pre,a,abbr,acronym,address,big,cite,code,del,dfn,em,img,ins,kbd,q,s,samp,small,strike,strong,sub,sup,tt,var,b,u,i,center,dl,dt,dd,ol,ul,li,fieldset,form,label,legend,table,caption,tbody,tfoot,thead,tr,th,td,article,aside,canvas,details,embed,figure,figcaption,footer,header,hgroup,menu,nav,output,ruby,section,summary,time,mark,audio,video{margin:0;padding:0;border:0;font-size:100%;font:inherit;vertical-align:baseline}article,aside,details,figcaption,figure,footer,header,hgroup,menu,nav,section{display:block}body{line-height:1}ol,ul{list-style:none}blockquote,q{quotes:none}blockquote:before,blockquote:after,q:before,q:after{content:'';content:none}table{border-collapse:collapse;border-spacing:0}
(2)style.css
@charset "utf-8";
body, html {
height: 100%;
}
body {
background: rgb(209,228,234);
background: -moz-radial-gradient(center, ellipse cover, rgba(209,228,234,1) 0%, rgba(186,228,244,1) 100%);
background: -webkit-gradient(radial, center center, 0px, center center, 100%, color-stop(0%,rgba(209,228,234,1)), color-stop(100%,rgba(186,228,244,1)));
background: -webkit-radial-gradient(center, ellipse cover, rgba(209,228,234,1) 0%,rgba(186,228,244,1) 100%);
background: -o-radial-gradient(center, ellipse cover, rgba(209,228,234,1) 0%,rgba(186,228,244,1) 100%);
background: -ms-radial-gradient(center, ellipse cover, rgba(209,228,234,1) 0%,rgba(186,228,244,1) 100%);
background: radial-gradient(ellipse at center, rgba(209,228,234,1) 0%,rgba(186,228,244,1) 100%);
filter: progid:DXImageTransform.Microsoft.gradient( startColorstr='#d1e4ea', endColorstr='#bae4f4',GradientType=1 );
padding:0;
margin:0;
}
.country-wrap {
position:relative;
width:100%;
height:100%;
overflow:hidden;
}
.push-bottom {
position:absolute;
bottom:0;
height:100%;
}
.bottom-ground {
background:#8d773e;
width:102%;
left:-1%;
height:60px;
bottom:0;
position:absolute;
box-shadow:0 2px 3px rgba(0,0,0,0.2) inset;
}
.street {
background:#7a7a7a url(data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAADIAAAAyCAMAAAAp4XiDAAAAUVBMVEWFhYWDg4N3d3dtbW17e3t1dXWBgYGHh4d5eXlzc3OLi4ubm5uVlZWPj4+NjY19fX2JiYl/f39ra2uRkZGZmZlpaWmXl5dvb29xcXGTk5NnZ2c8TV1mAAAAG3RSTlNAQEBAQEBAQEBAQEBAQEBAQEBAQEBAQEBAQEAvEOwtAAAFVklEQVR4XpWWB67c2BUFb3g557T/hRo9/WUMZHlgr4Bg8Z4qQgQJlHI4A8SzFVrapvmTF9O7dmYRFZ60YiBhJRCgh1FYhiLAmdvX0CzTOpNE77ME0Zty/nWWzchDtiqrmQDeuv3powQ5ta2eN0FY0InkqDD73lT9c9lEzwUNqgFHs9VQce3TVClFCQrSTfOiYkVJQBmpbq2L6iZavPnAPcoU0dSw0SUTqz/GtrGuXfbyyBniKykOWQWGqwwMA7QiYAxi+IlPdqo+hYHnUt5ZPfnsHJyNiDtnpJyayNBkF6cWoYGAMY92U2hXHF/C1M8uP/ZtYdiuj26UdAdQQSXQErwSOMzt/XWRWAz5GuSBIkwG1H3FabJ2OsUOUhGC6tK4EMtJO0ttC6IBD3kM0ve0tJwMdSfjZo+EEISaeTr9P3wYrGjXqyC1krcKdhMpxEnt5JetoulscpyzhXN5FRpuPHvbeQaKxFAEB6EN+cYN6xD7RYGpXpNndMmZgM5Dcs3YSNFDHUo2LGfZuukSWyUYirJAdYbF3MfqEKmjM+I2EfhA94iG3L7uKrR+GdWD73ydlIB+6hgref1QTlmgmbM3/LeX5GI1Ux1RWpgxpLuZ2+I+IjzZ8wqE4nilvQdkUdfhzI5QDWy+kw5Wgg2pGpeEVeCCA7b85BO3F9DzxB3cdqvBzWcmzbyMiqhzuYqtHRVG2y4x+KOlnyqla8AoWWpuBoYRxzXrfKuILl6SfiWCbjxoZJUaCBj1CjH7GIaDbc9kqBY3W/Rgjda1iqQcOJu2WW+76pZC9QG7M00dffe9hNnseupFL53r8F7YHSwJWUKP2q+k7RdsxyOB11n0xtOvnW4irMMFNV4H0uqwS5ExsmP9AxbDTc9JwgneAT5vTiUSm1E7BSflSt3bfa1tv8Di3R8n3Af7MNWzs49hmauE2wP+ttrq+AsWpFG2awvsuOqbipWHgtuvuaAE+A1Z/7gC9hesnr+7wqCwG8c5yAg3AL1fm8T9AZtp/bbJGwl1pNrE7RuOX7PeMRUERVaPpEs+yqeoSmuOlokqw49pgomjLeh7icHNlG19yjs6XXOMedYm5xH2YxpV2tc0Ro2jJfxC50ApuxGob7lMsxfTbeUv07TyYxpeLucEH1gNd4IKH2LAg5TdVhlCafZvpskfncCfx8pOhJzd76bJWeYFnFciwcYfubRc12Ip/ppIhA1/mSZ/RxjFDrJC5xifFjJpY2Xl5zXdguFqYyTR1zSp1Y9p+tktDYYSNflcxI0iyO4TPBdlRcpeqjK/piF5bklq77VSEaA+z8qmJTFzIWiitbnzR794USKBUaT0NTEsVjZqLaFVqJoPN9ODG70IPbfBHKK+/q/AWR0tJzYHRULOa4MP+W/HfGadZUbfw177G7j/OGbIs8TahLyynl4X4RinF793Oz+BU0saXtUHrVBFT/DnA3ctNPoGbs4hRIjTok8i+algT1lTHi4SxFvONKNrgQFAq2/gFnWMXgwffgYMJpiKYkmW3tTg3ZQ9Jq+f8XN+A5eeUKHWvJWJ2sgJ1Sop+wwhqFVijqWaJhwtD8MNlSBeWNNWTa5Z5kPZw5+LbVT99wqTdx29lMUH4OIG/D86ruKEauBjvH5xy6um/Sfj7ei6UUVk4AIl3MyD4MSSTOFgSwsH/QJWaQ5as7ZcmgBZkzjjU1UrQ74ci1gWBCSGHtuV1H2mhSnO3Wp/3fEV5a+4wz//6qy8JxjZsmxxy5+4w9CDNJY09T072iKG0EnOS0arEYgXqYnXcYHwjTtUNAcMelOd4xpkoqiTYICWFq0JSiPfPDQdnt+4/wuqcXY47QILbgAAAABJRU5ErkJggg==);
height:80px;
width:102%;
position:absolute;
bottom:0;
box-shadow:0 1px 16px rgba(111, 35, 51, 0.4) inset;
}
.street:after {
content:'';
display:block;
position:absolute;
width:100%;
height:10px;
background:#cdbcb4;
bottom:0;
border-bottom:3px solid #72625a;
z-index:1;
}
.street:before {
content:'';
display:block;
position:absolute;
width:100%;
height:7px;
background:#c2a99d;
bottom:-7px;
z-index:1;
}
.street-stripe {
background:#d4d4d4;
height:8px;
width:100px;
position:absolute;
bottom:44px;
border-radius:2px;
box-shadow:200px 0 0 #d4d4d4, 400px 0 0 #d4d4d4 , 600px 0 0 #d4d4d4 , 800px 0 0 #d4d4d4 , 1000px 0 0 #d4d4d4 , 1200px 0 0 #d4d4d4 , 1400px 0 0 #d4d4d4 , 1600px 0 0 #d4d4d4 , 1800px 0 0 #d4d4d4 , 2000px 0 0 #d4d4d4;
}
.grass {
height: 40px;
width: 100%;
background-color: #dbcac2;
position:absolute;
bottom:60px;
}
.grass:before {
position: absolute;
content: '';
top: 14px;
left: 0;
height: 8px;
width: 100%;
background-color: #b99f93;
}
.grass:after {
position: absolute;
content: '';
top: -4px;
left: 0;
width: 100%;
height: 8px;
background-color: #0aa;
background: linear-gradient(135deg, transparent 25%, #0aa 25%);
background-size:10px 10px;
}
.sun {
background:#ff9944;
width:40px;
height:40px;
border-radius:50%;
box-shadow:0 0 50px rgba(255,153,68,0.7);
position:absolute;
left:49%;
bottom:350px;
}
.tree-1 {
position:absolute;
z-index:2;
bottom:210px;
right:10px;
width:50px;
height:100px;
}
.trunk {
width:20%;
height:30%;
background:brown;
}
.branch {
position:abslute;
width:60%;
height:30%;
background:green;
-moz-transform:rotate(45deg);
border-radius:0 0 100% 0;
}
.branch-1 {
border:50px solid;
border-bottom:90px solid;
border-color:transparent transparent #a5894a transparent;
height: 0;
width: 0;
position:absolute;
left:-50px;
top:-70px;
}
.branch-2 {
border-bottom: 40px solid #9d8346;
border-left: 30px solid transparent;
border-right: 30px solid transparent;
height: 0;
width: 100px;
position:absolute;
top:60px;
left:-80px;
}
.branch-3 {
border-bottom: 50px solid #90713b;
border-left: 40px solid transparent;
border-right: 40px solid transparent;
height: 0;
width: 100px;
position:absolute;
top:100px;
left:-90px;
}
.mountain-1 {
background:#cea392;
width:500px;
height:500px;
position:absolute;
-moz-transform:rotate(45deg);
-webkit-transform:rotate(45deg);
bottom:-150px;
border-radius:40px;
}
.mountain-2 {
background:#e4dbd2;
width:800px;
height:800px;
position:absolute;
-moz-transform:rotate(45deg);
-webkit-transform:rotate(45deg);
bottom:-350px;
border-radius:40px;
left:250px;
z-index:-1;
box-shadow: 0 0 25px #e4dbd2;
opacity:0.6;
}
.la {
position: absolute;
bottom: 200px;
width: 2px;
height: 50px;
background: $cd;
margin-right: 20px;
}
.la:before {
top: 5px;
left: -10px;
width: 22px;
height: 2px;
background: $cd;
}
.la:after {
bottom: 0;
left: -2px;
width: 6px;
height: 12px;
background: $cd;
}
.l {
position: absolute;
width: 5px;
height: 5px;
border-radius: 50%;
background: #fff;
box-shadow: 0 0 10px #fff, 0 0 25px #fff, 0 0 50px #fff;
}
.l:nth-child(1) { left: -10px; }
.l:nth-child(2) { right: -10px; }
.car {
position:absolute;
top:-35%;
z-index:10;
-moz-animation:myfirst 10s linear infinite;
-webkit-animation:myfirst 10s linear infinite;
}
@-moz-keyframes myfirst
{
0% {left:-25%;}
100% {left:100%;}
}
@-webkit-keyframes myfirst
{
0% {left:-25%;}
100% {left:100%;}
}
.tyre {
width:30px;
height:30px;
border-radius:50%;
background:#3f3f40;
position:absolute;
z-index:2;
left:9px;
top:20px;
-moz-animation:tyre-rotate 1s infinite linear;
-webkit-animation:tyre-rotate 1s infinite linear;
}
@-moz-keyframes tyre-rotate {
from{-moz-transform:rotate(-360deg);}
to{-moz-transform:rotate(0deg);}
}
@-webkit-keyframes tyre-rotate {
from{-webkit-transform:rotate(-360deg);}
to{-webkit-transform:rotate(0deg);}
}
.tyre:before {
content:'';
width:20px;
height:20px;
border-radius:50%;
background:#bdc2bd;
position:absolute;
top:5px;
left:5px;
}
.gap {
background:#3f3f40;
width:2px;
height:4px;
position:absolute;
left:14px;
top:8px;
box-shadow:0 9px 0 #3f3f40;
}
.gap:before {
content:'';
display:block;
width:2px;
height:4px;
position:absolute;
background:#3f3f40;
box-shadow:0 12px 0 #3f3f40;
-webkit-transform:rotate(-90deg);
-webkit-transform-origin:0 7px;
-moz-transform:rotate(-90deg);
-moz-transform-origin:0 7px;
z-index:3;
}
.car-base {
position:absolute;
display:block;
width: 125px;
height: 30px;
background:#000000;
border-radius: 10% 10% 50% 50% / 60% 100% 20% 10%;
-webkit-transform:rotate(-2deg);
-moz-transform:rotate(-2deg);
border:solid;
border-color:#000000;
}
.back-bonet {
background: #4c4b4b;
border-radius: 54% 25% 0 0;
height: 22px;
left: 11px;
position: absolute;
top: 8px;
width: 40px;
}
.tyre.front {
left:94px;
}
.car-body {
border-bottom: 24px solid #d1352b;
height: 0;
top:10px;
width: 120px;
position:relative;
}
.car-body:before {
content:'';
display:inline-block;
width:30px;
height:24px;
position:absolute;
right:-5px;
background:#d1352b;
border-top-right-radius:4px;
z-index:1;
}
.car-body:after {
content:'';
display:inline-block;
width:121px;
border-bottom: 1px solid #942b25;
border-right: 2px solid transparent;
height: 0;
z-index:2;
position:absolute;
}
.car-gate {
width:32px;
height:20px;
background:#d1352b;
border-radius:0 0 2px 8px / 0 0 2px 8px;
box-shadow:0 0 0 1px #892924;
position:absolute;
left:48px;
}
.car-gate:before {
content:'';
width:8px;
height:2px;
background:#4c4b4b;
position:absolute;
top:2px;
left:4px;
box-shadow:1px 1px 1px rgba(0,0,0,0.1);
}
.car-top-back {
background: none repeat scroll 0 0 #4C4B4B;
border-radius: 5px 0 0 0;
height: 20px;
left: 4px;
position: absolute;
top: -20px;
width: 58px;
}
.car-top-back:before {
width:30px;
height:15px;
background:#736f6f;
content:'';
position:absolute;
top:3px;
left:8px;
border-radius:2px;
}
.car-top-back:after {
content:'';
background:#4c4b4b;
border-radius: 30%;
height: 5px;
left: 3px;
position: absolute;
top: -1px;
width: 62px;
}
.car-top-front {
top:-19px;
position:absolute;
left:47px;
width:20px;
height:20px;
background:#dc4630;
border-left: 1px solid #892924;
border-radius: 2px 0 0 0;
}
.car-top-front:after {
width:26px;
height:20px;
-webkit-transform:skew(37deg);
-moz-transform:skew(37deg);
background:#dc4630;
content:'';
position:absolute;
top:0;
left:6px;
border-radius:4px 0 4px 4px;
}
.car-top-front:before {
width:12px;
height:5px;
background:#dc4630;
content:'';
position:absolute;
top:14px;
left:28px;
z-index:1;
border:solid #a82e27;
border-width:0 1px 1px 0;
}
.wind-sheild {
top:3px;
left:3px;
position:absolute;
z-index:3;
width:18px;
height:12px;
background:#f5e7e7;
border-radius:0 3px 0 0;
}
.wind-sheild:after {
width:12px;
height:12px;
-webkit-transform:skew(25deg);
-moz-transform:skew(25deg);
background:#f5e7e7;
content:'';
position:absolute;
top:0;
left:10px;
border-radius:3px;
}
.boundary-tyre-cover {
position:absolute;
top:14px; left:10px;
border-bottom: 20px solid #4c4b4b;
border-right: 10px solid transparent;
height:0;
width:20px;
}
.boundary-tyre-cover:before {
content:'';
position:absolute;
display:inline-block;
background:#4c4b4b;
height:20px;
width:15px;
-webkit-transform:skewX(-20deg);
-moz-transform:skewX(-20deg);
border-radius:3px;
left:-6px;
top:0;
}
.boundary-tyre-cover:after {
content:'';
position:absolute;
display:inline-block;
background:#4c4b4b;
height:20px;
width:20px;
-webkit-transform:skewx(40deg);
-moz-transform:skewX(40deg);
border-radius:3px;
right:-14px;
top:0;
}
.boundary-tyre-cover-inner {
position:absolute;
top:4px; left:4px;
border-bottom: 16px solid black;
border-right: 10px solid transparent;
height:0;
width:15px;
z-index:2;
}
.boundary-tyre-cover-inner:before {
content:'';
position:absolute;
display:inline-block;
background:black;
height:16px;
width:15px;
-webkit-transform:skewX(-20deg);
-moz-transform:skewX(-20deg);
border-radius:3px 3px 0 0;
left:-6px;
top:0;
}
.boundary-tyre-cover-inner:after {
content:'';
position:absolute;
display:inline-block;
background:black;
height:16px;
width:20px;
-webkit-transform:skewx(40deg);
-moz-transform:skewX(40deg);
border-radius:3px 3px 0 0;
right:-11px;
top:0;
}
.boundary-tyre-cover-back-bottom {
position: absolute;
width: 14px;
height: 8px;
background: #4c4b4b;
top: 12px;
left: -19px;
}
.bonet-front {
background: #d1352b;
border-radius: 5px 258px 0 38px / 36px 50px 0 0;
height: 4px;
position: absolute;
right: 0;
top: -4px;
width: 40px;
z-index: 0;
}
.back-curve {
background: none repeat scroll 0 0 #4C4B4B;
border-radius: 960% 100% 0 0;
height: 20px;
left: -3px;
position: absolute;
top: 1px;
transform: rotate(6deg);
width: 5px;
}
.stepney {
height: 6px;
left: -4px;
position: absolute;
top: 6px;
width: 8px;
z-index: -1;
background:#3f3f40;
}
.stepney:before {
width:8px;
height:12px;
background:#3f3f40;
content:'';
position:absolute;
top:-10px;
left:-7px;
border-radius:3px 3px 0 0;
}
.stepney:after {
width:8px;
height:12px;
background:#0d0c0d;
content:'';
position:absolute;
top:0px;
left:-7px;
border-radius:0 0 3px 3px;
}
.tyre-cover-front {
background:#4c4b4b;
height: 4px;
left: 97px;
position: absolute;
top: 13px;
width: 22px;
z-index: 1;
}
.tyre-cover-front:before {
background: none repeat scroll 0 0 #4c4b4b;
content: "";
display: inline-block;
height: 21px;
left: -10px;
position: absolute;
top: 0;
transform: skewX(-30deg);
width: 10px;
z-index: 6;
border-radius:4px 0 0 0;
}
.tyre-cover-front:after {
background: none repeat scroll 0 0 #4c4b4b;
content: "";
display: inline-block;
height: 6px;
left: 14px;
position: absolute;
top: 0;
transform: skewX(30deg);
width: 17px;
z-index: 6;
border-radius:0 4px 2px 0;
}
.boundary-tyre-cover-inner-front {
position:absolute;
top:4px; left:4px;
border-bottom: 16px solid black;
border-right: 10px solid transparent;
height:0;
width:15px;
z-index:7;
}
.boundary-tyre-cover-inner-front:before {
background: none repeat scroll 0 0 #000000;
border-radius: 3px 3px 0 0;
content: "";
display: inline-block;
height: 17px;
left: -10px;
position: absolute;
top: 0;
transform: skewX(-30deg);
width: 15px;
}
.boundary-tyre-cover-inner-front:after {
content:'';
position:absolute;
display:inline-block;
background:black;
height:16px;
width:20px;
-webkit-transform:skewx(25deg);
-moz-transform:skewX(25deg);
border-radius:3px 3px 0 0;
right:-12px;
top:0;
}
.base-axcel {
background: none repeat scroll 0 0 black;
bottom: -15px;
height: 10px;
left: 30px;
position: absolute;
transform: rotate(-2deg);
width: 70px;
z-index:-1;
}
.base-axcel:before {
background: none repeat scroll 0 0 black;
border-radius: 0 0 0 10px / 0 0 0 5px;
content: "";
height: 10px;
left: -35px;
position: absolute;
top: -2px;
transform: rotate(6deg);
width: 30px;
}
.base-axcel:after {
background: none repeat scroll 0 0 black;
border-radius: 0 0 0 10px / 0 0 0 5px;
content: "";
height: 10px;
right: -33px;
position: absolute;
top: -1px;
transform: rotate(-4deg);
width: 40px;
border-radius:0 10px 5px 0;
}
.front-bumper {
background: none repeat scroll 0 0 #4c4b4b;
border-radius: 0 2px 2px 0;
height: 8px;
position: absolute;
right: -15px;
width: 11px;
z-index: 1;
-moz-transform:rotate(-5deg);
-webkit-transform:rotate(-5deg);
}
.front-bumper:before {
background: none repeat scroll 0 0 #000000;
content: "";
height: 10px;
left: -7px;
position: absolute;
transform: rotate(-22deg);
width: 9px;
z-index: 1;
}
.car-shadow {
background: none repeat scroll 0 0 rgba(0, 0, 0, 0);
bottom: -15px;
box-shadow: -5px 10px 15px 3px #000000;
left: -7px;
position: absolute;
width: 136px;
}
.noise {
position: relative;
z-index: 1;
}
.noise:before, .body-noise:before {
content: "";
position: absolute;
z-index: -1;
top:0;
bottom:0;
left:0;
right:0;
background-image: url(data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAADIAAAAyCAMAAAAp4XiDAAAAUVBMVEWFhYWDg4N3d3dtbW17e3t1dXWBgYGHh4d5eXlzc3OLi4ubm5uVlZWPj4+NjY19fX2JiYl/f39ra2uRkZGZmZlpaWmXl5dvb29xcXGTk5NnZ2c8TV1mAAAAG3RSTlNAQEBAQEBAQEBAQEBAQEBAQEBAQEBAQEBAQEAvEOwtAAAFVklEQVR4XpWWB67c2BUFb3g557T/hRo9/WUMZHlgr4Bg8Z4qQgQJlHI4A8SzFVrapvmTF9O7dmYRFZ60YiBhJRCgh1FYhiLAmdvX0CzTOpNE77ME0Zty/nWWzchDtiqrmQDeuv3powQ5ta2eN0FY0InkqDD73lT9c9lEzwUNqgFHs9VQce3TVClFCQrSTfOiYkVJQBmpbq2L6iZavPnAPcoU0dSw0SUTqz/GtrGuXfbyyBniKykOWQWGqwwMA7QiYAxi+IlPdqo+hYHnUt5ZPfnsHJyNiDtnpJyayNBkF6cWoYGAMY92U2hXHF/C1M8uP/ZtYdiuj26UdAdQQSXQErwSOMzt/XWRWAz5GuSBIkwG1H3FabJ2OsUOUhGC6tK4EMtJO0ttC6IBD3kM0ve0tJwMdSfjZo+EEISaeTr9P3wYrGjXqyC1krcKdhMpxEnt5JetoulscpyzhXN5FRpuPHvbeQaKxFAEB6EN+cYN6xD7RYGpXpNndMmZgM5Dcs3YSNFDHUo2LGfZuukSWyUYirJAdYbF3MfqEKmjM+I2EfhA94iG3L7uKrR+GdWD73ydlIB+6hgref1QTlmgmbM3/LeX5GI1Ux1RWpgxpLuZ2+I+IjzZ8wqE4nilvQdkUdfhzI5QDWy+kw5Wgg2pGpeEVeCCA7b85BO3F9DzxB3cdqvBzWcmzbyMiqhzuYqtHRVG2y4x+KOlnyqla8AoWWpuBoYRxzXrfKuILl6SfiWCbjxoZJUaCBj1CjH7GIaDbc9kqBY3W/Rgjda1iqQcOJu2WW+76pZC9QG7M00dffe9hNnseupFL53r8F7YHSwJWUKP2q+k7RdsxyOB11n0xtOvnW4irMMFNV4H0uqwS5ExsmP9AxbDTc9JwgneAT5vTiUSm1E7BSflSt3bfa1tv8Di3R8n3Af7MNWzs49hmauE2wP+ttrq+AsWpFG2awvsuOqbipWHgtuvuaAE+A1Z/7gC9hesnr+7wqCwG8c5yAg3AL1fm8T9AZtp/bbJGwl1pNrE7RuOX7PeMRUERVaPpEs+yqeoSmuOlokqw49pgomjLeh7icHNlG19yjs6XXOMedYm5xH2YxpV2tc0Ro2jJfxC50ApuxGob7lMsxfTbeUv07TyYxpeLucEH1gNd4IKH2LAg5TdVhlCafZvpskfncCfx8pOhJzd76bJWeYFnFciwcYfubRc12Ip/ppIhA1/mSZ/RxjFDrJC5xifFjJpY2Xl5zXdguFqYyTR1zSp1Y9p+tktDYYSNflcxI0iyO4TPBdlRcpeqjK/piF5bklq77VSEaA+z8qmJTFzIWiitbnzR794USKBUaT0NTEsVjZqLaFVqJoPN9ODG70IPbfBHKK+/q/AWR0tJzYHRULOa4MP+W/HfGadZUbfw177G7j/OGbIs8TahLyynl4X4RinF793Oz+BU0saXtUHrVBFT/DnA3ctNPoGbs4hRIjTok8i+algT1lTHi4SxFvONKNrgQFAq2/gFnWMXgwffgYMJpiKYkmW3tTg3ZQ9Jq+f8XN+A5eeUKHWvJWJ2sgJ1Sop+wwhqFVijqWaJhwtD8MNlSBeWNNWTa5Z5kPZw5+LbVT99wqTdx29lMUH4OIG/D86ruKEauBjvH5xy6um/Sfj7ei6UUVk4AIl3MyD4MSSTOFgSwsH/QJWaQ5as7ZcmgBZkzjjU1UrQ74ci1gWBCSGHtuV1H2mhSnO3Wp/3fEV5a+4wz//6qy8JxjZsmxxy5+4w9CDNJY09T072iKG0EnOS0arEYgXqYnXcYHwjTtUNAcMelOd4xpkoqiTYICWFq0JSiPfPDQdnt+4/wuqcXY47QILbgAAAABJRU5ErkJggg==);
}
.hill {
position: absolute;
bottom: 0;
right: 0;
width: 100%;
height: 250px;
border-top-right-radius: 160%;
border-top-left-radius: 100%;
background-color: #adde60;
z-index:-1;
}
.hill:after {
content: '';
position: absolute;
bottom: -100px;
right: -400px;
width: 120%;
height: 110%;
border-top-right-radius: 100%;
border-top-left-radius: 0%;
background-color: #94c943;
-moz-transform:rotate(-12deg);
-webkit-transform:rotate(-12deg);
box-shadow:0 0 25px #cbf191 inset;
}
.hill:before {
background-color: #93cc3a;
border-top-left-radius: 0;
border-top-right-radius: 100%;
bottom: -70px;
content: "";
height: 110%;
left: -54px;
position: absolute;
transform: rotate(4deg);
width: 120%;
}
.cloud {
background:#fff;
width:150px;
height:50px;
border-radius:50px;
position:absolute;
left:450px;
top:150px;
}
.cloud:before {
width:100px;
height:100px;
content:'';
position:absolute;
bottom:0;
left:-15px;
border-radius:50px;
box-shadow:100px 0 0 #fff;
background:#fff;
}