创建新图层
public static void CreateLayer(string LayerName)
{
Document acDoc = Application.DocumentManager.MdiActiveDocument;
Database acCurDb = acDoc.Database;
using (Transaction acTrans = acCurDb.TransactionManager.StartTransaction())
{
LayerTable acLyrTbl = acTrans.GetObject(acCurDb.LayerTableId, OpenMode.ForRead) as LayerTable;
string sLayerName = LayerName;
if (acLyrTbl.Has(sLayerName) == false)
{
using (LayerTableRecord acLyrTblRec = new LayerTableRecord())
{
acLyrTblRec.Name = sLayerName;
acLyrTbl.UpgradeOpen();
acLyrTbl.Add(acLyrTblRec);
acTrans.AddNewlyCreatedDBObject(acLyrTblRec, true);
}
}
acTrans.Commit();
}
}
镜像CAD实体对象
public static Entity MirrorEntity(this ObjectId entId, Point3d point1, Point3d point2, bool isEraseSource)
{
Entity entR;
Matrix3d mt = Matrix3d.Mirroring(new Line3d(point1, point2));
using (Transaction trans = entId.Database.TransactionManager.StartTransaction())
{
if (isEraseSource)
{
Entity ent = (Entity)trans.GetObject(entId, OpenMode.ForWrite);
ent.TransformBy(mt);
entR = ent;
}
else
{
Entity ent = (Entity)trans.GetObject(entId, OpenMode.ForRead);
entR = ent.GetTransformedCopy(mt);
}
return entR;
}
}
public static Entity MirrorEntity(this Entity ent, Point3d point1, Point3d point2, bool isEraseSource)
{
Entity entR;
if (ent.IsNewObject == true)
{
Matrix3d mt = Matrix3d.Mirroring(new Line3d(point1, point2));
entR = ent.GetTransformedCopy(mt);
}
else
{
entR = ent.ObjectId.MirrorEntity(point1, point2, isEraseSource);
}
return entR;
}
CAD ObjectId转换为实体对象
public static DBObject GetDBObject(ObjectId id)
{
Document doc = MgdAcApplication.DocumentManager.MdiActiveDocument;
_ = MgdAcApplication.DocumentManager.MdiActiveDocument.Editor;
using (Transaction trans = doc.TransactionManager.StartTransaction())
{
DBObject pline = trans.GetObject(id, OpenMode.ForRead);
trans.Commit();
return pline;
}
}
弧度值转角度值
public static double RadianToDegree(this double angle)
{
return angle * (180.0 / Math.PI);
}
角度值转弧度值
public static double DegreeToRadian(this double angle)
{
return angle * (Math.PI / 180.0);
}
将实体对象添加到模型空间
public static ObjectId AddToModelSpace(this Database db, Entity ent)
{
ObjectId entId;
using (Transaction tr = db.TransactionManager.StartTransaction())
{
BlockTable bt = (BlockTable)tr.GetObject(db.BlockTableId, OpenMode.ForRead);
BlockTableRecord btr = (BlockTableRecord)tr.GetObject(bt[BlockTableRecord.ModelSpace], OpenMode.ForWrite);
entId = btr.AppendEntity(ent);
tr.AddNewlyCreatedDBObject(ent, true);
tr.Commit();
}
return entId;
}
添加实体到块表记录
public static ObjectId AddBlockTableRecord(this Database db, string blockName, List<Entity> ents)
{
DocumentLock m_DocumentLock = Autodesk.AutoCAD.ApplicationServices.Application.DocumentManager.MdiActiveDocument.LockDocument();
BlockTable bt = (BlockTable)db.BlockTableId.GetObject(OpenMode.ForRead);
if (!bt.Has(blockName))
{
BlockTableRecord btr = new BlockTableRecord();
btr.Name = blockName;
ents.ForEach(ent => btr.AppendEntity(ent));
bt.UpgradeOpen();
bt.Add(btr);
db.TransactionManager.AddNewlyCreatedDBObject(btr, true);
bt.DowngradeOpen();
}
m_DocumentLock.Dispose();
return bt[blockName];
}
public static ObjectId AddBlockTableRecord(this Database db, string blockName, params Entity[] ents)
{
return AddBlockTableRecord(db, blockName, ents.ToList());
}
将块定义插入到模型空间
public static ObjectId InsertBlockReference(this ObjectId spaceId, string layer, string blockName, Point3d position, double rotateAngle)
{
ObjectId blockRefId;
Database db = spaceId.Database;
BlockTable bt = (BlockTable)db.BlockTableId.GetObject(Autodesk.AutoCAD.DatabaseServices.OpenMode.ForRead);
if (!bt.Has(blockName)) return ObjectId.Null;
DocumentLock m_DocumentLock = Autodesk.AutoCAD.ApplicationServices.Application.DocumentManager.MdiActiveDocument.LockDocument();
BlockTableRecord space = (BlockTableRecord)spaceId.GetObject(Autodesk.AutoCAD.DatabaseServices.OpenMode.ForWrite);
BlockReference br = new BlockReference(position, bt[blockName]);
br.Layer = layer;
br.Rotation = rotateAngle;
blockRefId = space.AppendEntity(br);
db.TransactionManager.AddNewlyCreatedDBObject(br, true);
space.DowngradeOpen();
m_DocumentLock.Dispose();
return blockRefId;
}
删除CAD模型空间内当前实体对象
public static bool DelEntity(ObjectId id)
{
try
{
if (!id.IsNull)
{
using (Database db = HostApplicationServices.WorkingDatabase)
{
using (Transaction trans = db.TransactionManager.StartTransaction())
{
Entity entity = (Entity)trans.GetObject(id, OpenMode.ForWrite, true);
entity.Erase(true);
trans.Commit();
}
}
}
else
{
return false;
}
}
catch
{
return false;
}
return true;
}
判断用户当前是否已选择实体
private static void IsSelected()
{
DocumentCollection acDocMgr = Application.DocumentManager;
Document acDoc = acDocMgr.MdiActiveDocument;
Database acCurDb = acDoc.Database;
using (acDoc.LockDocument())
{
using (Transaction acTrans = acCurDb.TransactionManager.StartTransaction())
{
PromptSelectionResult acSSPrompt = acDoc.Editor.GetSelection();
if (acSSPrompt.Status == PromptStatus.OK)
{
SelectionSet acSSet = acSSPrompt.Value;
foreach (SelectedObject acSSObj in acSSet)
{
if (acSSObj != null)
{
}
}
acTrans.Commit();
}
}
}
}
转载请注明转载地址!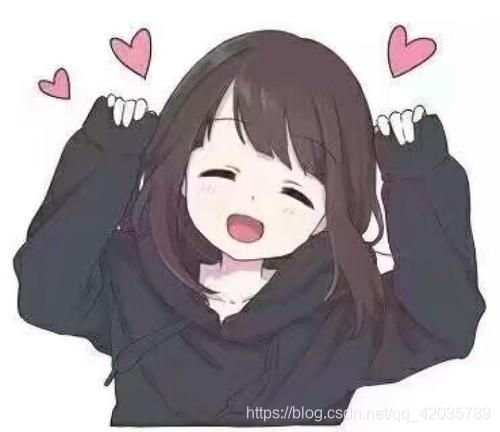