ASP.NET MVC 3 Beta中除了推出一种新的视图引擎Razor。还推出了几种新的HtmlHelper。我比较关注的是WebGrid,这篇文章将介绍一下WebGrid的使用。WebGrid提供了分页和排序的功能,在此之前在MVC中分页和排序时需要自己去写的。这篇文章将分别介绍在aspx视图引擎和Razor视图引擎中如何使用它。
我通过ADO.NET Entity Data Model从NORTHWND的Products中表中取数据。在Controller中取数据:
public
class
HomeController : Controller
{
public
ActionResult Index()
{
NORTHWNDEntities entity
=
new
NORTHWNDEntities();
return
View( entity.Products.ToList());
}
}
在aspx视图引擎中使用WebGrid代码如下:
<
div
id
="grid"
>
<%
var grid
=
new
WebGrid(source: Model, defaultSort:
"
ProductName
"
, rowsPerPage:
5
);
%>
<%
=
grid.GetHtml(
tableStyle:
"
grid
"
,
headerStyle:
"
head
"
,
alternatingRowStyle:
"
alt
"
,
columns: grid.Columns(
grid.Column(format: (item)
=>
Html.ActionLink(
"
Edit
"
,
"
Edit
"
,
new
{ id
=
item.ProductID })),
grid.Column(format: (item)
=>
Html.ActionLink(
"
Delete
"
,
"
Delete
"
,
null
,
new
{ onclick
=
string
.Format(
"
deleteRecord('Employee', '{0}')
"
, item.ProductID), @class
=
"
Delete
"
, href
=
"
JavaScript:void(0)
"
})),
grid.Column(
"
ProductName
"
,
"
产品名称
"
),
grid.Column(
"
QuantityPerUnit
"
,
"
每单位数量
"
),
grid.Column(
"
UnitPrice
"
,
"
单价
"
),
grid.Column(
"
UnitsInStock
"
,
"
库存单位
"
),
grid.Column(
"
UnitsOnOrder
"
,
"
订单单位
"
),
grid.Column(
"
ReorderLevel
"
,
"
重新排序级别
"
),
grid.Column(
"
Discontinued
"
,
"
已停产
"
)
)
)
%>
</
div
>
在Razor中使用WebGrid代码如下:
<
div
id
="grid"
>
@{
var grid = new WebGrid(source: Model,
defaultSort: "ProductName",
rowsPerPage: 10);
}
@grid.GetHtml(
tableStyle: "grid",
headerStyle: "head",
alternatingRowStyle: "alt",
columns: grid.Columns(
grid.Column(format: (item) => Html.ActionLink("Edit", "Edit", new { id = item.ProductID })),
grid.Column(format: (item) => Html.ActionLink("Delete", "Delete", null, new { onclick = string.Format("deleteRecord('Product', '{0}')", item.ProductID), @class = "Delete", href = "JavaScript:void(0)" })),
grid.Column("ProductName","产品名称"),
grid.Column("QuantityPerUnit","每单位数量"),
grid.Column("UnitPrice","单价"),
grid.Column("UnitsInStock", "库存单位"),
grid.Column("UnitsOnOrder","订单单位"),
grid.Column("ReorderLevel","重新排序级别"),
grid.Column("Discontinued","已停产")
)
)
</
div
>
WebGrid构造函数如下:
public WebGrid(IEnumerable<dynamic> source, IEnumerable<string> columnNames = null, string defaultSort = null, int rowsPerPage = 10, bool canPage = true, bool canSort = true, string ajaxUpdateContainerId = null, string fieldNamePrefix = null, string pageFieldName = null, string selectionFieldName = null, string sortFieldName = null, string sortDirectionFieldName = null);
常见参数意思是:
1、source 表示数据源
2、columnNames表示显示的列
3、defaultSort 默认按什么排序
4、rowsPerPage 每页多少行数据
5、canPage 是否能排序
上面两段代码的意思是定义了一个既分页又能排序的grid。
运行:
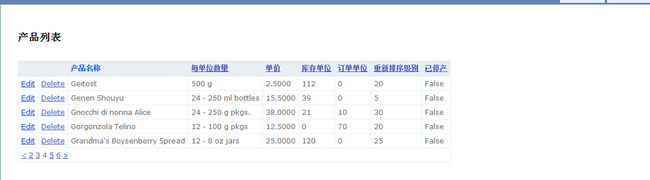
在看看两个view的完整代码:
aspx:
<%
@ Page Title
=
""
Language
=
"
C#
"
MasterPageFile
=
"
~/Views/Shared/Site.Master
"
Inherits
=
"
System.Web.Mvc.ViewPage<List<WebGridAspx.Models.Products>>
"
%>
<
asp:Content ID
=
"
Content1
"
ContentPlaceHolderID
=
"
TitleContent
"
runat
=
"
server
"
>
产品列表
</
asp:Content
>
<
asp:Content ID
=
"
Content2
"
ContentPlaceHolderID
=
"
MainContent
"
runat
=
"
server
"
>
<
script type
=
"
text/javascript
"
>
function deleteRecord(a, b) {
alert(
"
删除:
"
+
b);
}
</
script
>
<
h2
>
产品列表
</
h2
>
<
div id
=
"
grid
"
>
<%
var grid
=
new
WebGrid(source: Model, defaultSort:
"
ProductName
"
, rowsPerPage:
5
);
%>
<%=
grid.GetHtml(
tableStyle:
"
grid
"
,
headerStyle:
"
head
"
,
alternatingRowStyle:
"
alt
"
,
columns: grid.Columns(
grid.Column(format: (item)
=>
Html.ActionLink(
"
Edit
"
,
"
Edit
"
,
new
{ id
=
item.ProductID })),
grid.Column(format: (item)
=>
Html.ActionLink(
"
Delete
"
,
"
Delete
"
,
null
,
new
{ onclick
=
string
.Format(
"
deleteRecord('Employee', '{0}')
"
, item.ProductID), @class
=
"
Delete
"
, href
=
"
JavaScript:void(0)
"
})),
grid.Column(
"
ProductName
"
,
"
产品名称
"
),
grid.Column(
"
QuantityPerUnit
"
,
"
每单位数量
"
),
grid.Column(
"
UnitPrice
"
,
"
单价
"
),
grid.Column(
"
UnitsInStock
"
,
"
库存单位
"
),
grid.Column(
"
UnitsOnOrder
"
,
"
订单单位
"
),
grid.Column(
"
ReorderLevel
"
,
"
重新排序级别
"
),
grid.Column(
"
Discontinued
"
,
"
已停产
"
)
)
)
%>
</
div
>
</
asp:Content
>
Razor:
代码
@model List
<
WebGridRazor.Models.Products
>
@{
View.Title
=
"
产品列表
"
;
}
<
p
>
<
h2
>
产品列表
</
h2
>
<
div id
=
"
grid
"
>
@{
var grid
=
new
WebGrid(source: Model,
defaultSort:
"
ProductName
"
,
rowsPerPage:
3
);
}
@grid.GetHtml(
tableStyle:
"
grid
"
,
headerStyle:
"
head
"
,
alternatingRowStyle:
"
alt
"
,
columns: grid.Columns(
grid.Column(format: (item)
=>
Html.ActionLink(
"
Edit
"
,
"
Edit
"
,
new
{ id
=
item.ProductID })),
grid.Column(format: (item)
=>
Html.ActionLink(
"
Delete
"
,
"
Delete
"
,
null
,
new
{ onclick
=
string
.Format(
"
deleteRecord('Product', '{0}')
"
, item.ProductID), @class
=
"
Delete
"
, href
=
"
JavaScript:void(0)
"
})),
grid.Column(
"
ProductName
"
,
"
产品名称
"
),
grid.Column(
"
QuantityPerUnit
"
,
"
每单位数量
"
),
grid.Column(
"
UnitPrice
"
,
"
单价
"
),
grid.Column(
"
UnitsInStock
"
,
"
库存单位
"
),
grid.Column(
"
UnitsOnOrder
"
,
"
订单单位
"
),
grid.Column(
"
ReorderLevel
"
,
"
重新排序级别
"
),
grid.Column(
"
Discontinued
"
,
"
已停产
"
)
)
)
</
div
>
</
p
>
Razor去掉了那些模板页的代码,使代码看起来更整洁。比较喜欢Razor。
总结:本文很简单,介绍了一下ASP.NET MVC 3 Beta中新功能WebGrid,由于这种方式WebGrid是在内存中分页和排序的,所以不适合大数据量。
代码:http://files.cnblogs.com/zhuqil/MvcApplication11.rar
原文链接: http://www.cnblogs.com/zhuqil/archive/2010/10/17/ASP-NET-MVC-3-Beta-WebGrid.html