最近要帮老师做个工资管理系统,需要自动生成Word.
就上网找了个Word操作类,再做了点修改,下面公布一下自己的代码:
using
System;
using
System.Collections.Generic;
using
System.Linq;
using
System.Text;
using
System.Data;
using
System.Drawing;
using
Word
=
Microsoft.Office.Interop.Word;
using
Microsoft.Office.Interop.Word;
using
System.Windows.Forms;
/*
***********************************************************************************************************************************
* * 文件名 :
* * 声明 :
* * 创建者 : 黄聪
* * 创建日期 : 2009.10.8
* * 修改者 : 黄聪
* * 最新修改日期 : 2009.10.8
***********************************************************************************************************************************
*/
namespace
Tool
{
/*
*******************************************************************************************************************************
* * 类名 : WordPlayer
* * 声明 :
* * 创建者 : 黄聪
* * 创建日期 : 2009.7.15
* * 修改者 : 黄聪
* * 最新修改日期 : 2009.7.15
*******************************************************************************************************************************
*/
public
class
WordPlayer
{
#region
- 属性 -
private
static
Microsoft.Office.Interop.Word._Application oWord
=
null
;
private
static
Microsoft.Office.Interop.Word._Document odoc
=
null
;
private
static
Microsoft.Office.Interop.Word._Document oDoc
{
get
{
if
(odoc
==
null
)
{
odoc
=
oWord.Documents.Add(
ref
Nothing,
ref
Nothing,
ref
Nothing,
ref
Nothing);
}
return
odoc;
}
set
{
if
(value
!=
null
)
{
odoc
=
value;
}
}
}
private
static
object
Nothing
=
System.Reflection.Missing.Value;
public
enum
Orientation
{
横板,
竖板
}
public
enum
Alignment
{
左对齐,
居中,
右对齐
}
#endregion
#region
- 添加文档 -
#region
- 创建并打开一个空的word文档进行编辑 -
public
static
void
OpenNewWordFileToEdit()
{
oDoc
=
oWord.Documents.Add(
ref
Nothing,
ref
Nothing,
ref
Nothing,
ref
Nothing);
}
#endregion
#endregion
#region
- 创建新Word -
public
static
bool
CreateWord(
bool
isVisible)
{
try
{
oWord
=
new
Microsoft.Office.Interop.Word.Application();
oWord.Visible
=
isVisible;
return
true
;
}
catch
(Exception)
{
return
false
;
}
}
public
static
bool
CreateWord()
{
return
CreateWord(
false
);
}
#endregion
#region
- 打开文档 -
public
static
bool
Open(
string
filePath,
bool
isVisible)
{
try
{
oWord.Visible
=
isVisible;
object
path
=
filePath;
oDoc
=
oWord.Documents.Open(
ref
path,
ref
Nothing,
ref
Nothing,
ref
Nothing,
ref
Nothing,
ref
Nothing,
ref
Nothing,
ref
Nothing,
ref
Nothing,
ref
Nothing,
ref
Nothing,
ref
Nothing,
ref
Nothing,
ref
Nothing,
ref
Nothing,
ref
Nothing);
return
true
;
}
catch
(Exception)
{
return
false
;
}
}
#endregion
#region
- 插入表格 -
public
static
bool
InsertTable(DataTable dt,
bool
haveBorder,
double
[] colWidths)
{
try
{
object
Nothing
=
System.Reflection.Missing.Value;
int
lenght
=
oDoc.Characters.Count
-
1
;
object
start
=
lenght;
object
end
=
lenght;
//
表格起始坐标
Microsoft.Office.Interop.Word.Range tableLocation
=
oDoc.Range(
ref
start,
ref
end);
//
添加Word表格
Microsoft.Office.Interop.Word.Table table
=
oDoc.Tables.Add(tableLocation, dt.Rows.Count, dt.Columns.Count,
ref
Nothing,
ref
Nothing);
if
(colWidths
!=
null
)
{
for
(
int
i
=
0
; i
<
colWidths.Length; i
++
)
{
table.Columns[i
+
1
].Width
=
(
float
)(
28.5F
*
colWidths[i]);
}
}
///
设置TABLE的样式
table.Rows.HeightRule
=
Microsoft.Office.Interop.Word.WdRowHeightRule.wdRowHeightAtLeast;
table.Rows.Height
=
oWord.CentimetersToPoints(
float
.Parse(
"
0.8
"
));
table.Range.Font.Size
=
10.5F
;
table.Range.Font.Name
=
"
宋体
"
;
table.Range.Font.Bold
=
0
;
table.Range.ParagraphFormat.Alignment
=
Microsoft.Office.Interop.Word.WdParagraphAlignment.wdAlignParagraphCenter;
table.Range.Cells.VerticalAlignment
=
Microsoft.Office.Interop.Word.WdCellVerticalAlignment.wdCellAlignVerticalCenter;
if
(haveBorder
==
true
)
{
//
设置外框样式
table.Borders.OutsideLineStyle
=
Microsoft.Office.Interop.Word.WdLineStyle.wdLineStyleSingle;
table.Borders.InsideLineStyle
=
Microsoft.Office.Interop.Word.WdLineStyle.wdLineStyleSingle;
//
样式设置结束
}
for
(
int
row
=
0
; row
<
dt.Rows.Count; row
++
)
{
for
(
int
col
=
0
; col
<
dt.Columns.Count; col
++
)
{
table.Cell(row
+
1
, col
+
1
).Range.Text
=
dt.Rows[row][col].ToString();
}
}
return
true
;
}
catch
(Exception e)
{
MessageBox.Show(e.ToString(),
"
错误提示
"
, MessageBoxButtons.OK, MessageBoxIcon.Error);
return
false
;
}
finally
{
}
}
public
static
bool
InsertTable(DataTable dt,
bool
haveBorder)
{
return
InsertTable(dt, haveBorder,
null
);
}
public
static
bool
InsertTable(DataTable dt)
{
return
InsertTable(dt,
false
,
null
);
}
#endregion
#region
- 插入文本 -
public
static
bool
InsertText(
string
strText, System.Drawing.Font font, Alignment alignment,
bool
isAftre)
{
try
{
Word.Range rng
=
oDoc.Content;
int
lenght
=
oDoc.Characters.Count
-
1
;
object
start
=
lenght;
object
end
=
lenght;
rng
=
oDoc.Range(
ref
start,
ref
end);
if
(isAftre
==
true
)
{
strText
+=
"
\r\n
"
;
}
rng.Text
=
strText;
rng.Font.Name
=
font.Name;
rng.Font.Size
=
font.Size;
if
(font.Style
==
FontStyle.Bold) { rng.Font.Bold
=
1
; }
//
设置单元格中字体为粗体
SetAlignment(rng, alignment);
return
true
;
}
catch
(Exception)
{
return
false
;
}
}
public
static
bool
InsertText(
string
strText)
{
return
InsertText(strText,
new
System.Drawing.Font(
"
宋体
"
,
10.5F
, FontStyle.Bold), Alignment.左对齐,
false
);
}
#endregion
#region
- 设置对齐方式 -
private
static
Microsoft.Office.Interop.Word.WdParagraphAlignment SetAlignment(Range rng, Alignment alignment)
{
rng.ParagraphFormat.Alignment
=
SetAlignment(alignment);
return
SetAlignment(alignment);
}
private
static
Microsoft.Office.Interop.Word.WdParagraphAlignment SetAlignment(Alignment alignment)
{
if
(alignment
==
Alignment.居中)
{
return
Word.WdParagraphAlignment.wdAlignParagraphCenter;
}
else
if
(alignment
==
Alignment.左对齐)
{
return
Word.WdParagraphAlignment.wdAlignParagraphLeft;
}
else
{
return
Word.WdParagraphAlignment.wdAlignParagraphRight; }
}
#endregion
#region
- 页面设置 -
public
static
void
SetPage(Orientation orientation,
double
width,
double
height,
double
topMargin,
double
leftMargin,
double
rightMargin,
double
bottomMargin)
{
oDoc.PageSetup.PageWidth
=
oWord.CentimetersToPoints((
float
)width);
oDoc.PageSetup.PageHeight
=
oWord.CentimetersToPoints((
float
)height);
if
(orientation
==
Orientation.横板)
{
oDoc.PageSetup.Orientation
=
Microsoft.Office.Interop.Word.WdOrientation.wdOrientLandscape;
}
oDoc.PageSetup.TopMargin
=
(
float
)(topMargin
*
25
);
//
上边距
oDoc.PageSetup.LeftMargin
=
(
float
)(leftMargin
*
25
);
//
左边距
oDoc.PageSetup.RightMargin
=
(
float
)(rightMargin
*
25
);
//
右边距
oDoc.PageSetup.BottomMargin
=
(
float
)(bottomMargin
*
25
);
//
下边距
}
public
static
void
SetPage(Orientation orientation,
double
topMargin,
double
leftMargin,
double
rightMargin,
double
bottomMargin)
{
SetPage(orientation,
21
,
29.7
, topMargin, leftMargin, rightMargin, bottomMargin);
}
public
static
void
SetPage(
double
topMargin,
double
leftMargin,
double
rightMargin,
double
bottomMargin)
{
SetPage(Orientation.竖板,
21
,
29.7
, topMargin, leftMargin, rightMargin, bottomMargin);
}
#endregion
#region
- 插入分页符 -
public
static
void
InsertBreak()
{
Word.Paragraph para;
para
=
oDoc.Content.Paragraphs.Add(
ref
Nothing);
object
pBreak
=
(
int
)WdBreakType.wdSectionBreakNextPage;
para.Range.InsertBreak(
ref
pBreak);
}
#endregion
#region
- 关闭当前文档 -
public
static
bool
CloseDocument()
{
try
{
object
doNotSaveChanges
=
Word.WdSaveOptions.wdDoNotSaveChanges;
oDoc.Close(
ref
doNotSaveChanges,
ref
Nothing,
ref
Nothing);
oDoc
=
null
;
return
true
;
}
catch
(Exception)
{
return
false
;
}
}
#endregion
#region
- 关闭程序 -
public
static
bool
Quit()
{
try
{
object
saveOption
=
Word.WdSaveOptions.wdDoNotSaveChanges;
oWord.Quit(
ref
saveOption,
ref
Nothing,
ref
Nothing);
return
true
;
}
catch
(Exception)
{
return
false
;
}
}
#endregion
#region
- 保存文档 -
public
static
bool
Save(
string
savePath)
{
return
Save(savePath,
false
);
}
public
static
bool
Save(
string
savePath,
bool
isClose)
{
try
{
object
fileName
=
savePath;
oDoc.SaveAs(
ref
fileName,
ref
Nothing,
ref
Nothing,
ref
Nothing,
ref
Nothing,
ref
Nothing,
ref
Nothing,
ref
Nothing,
ref
Nothing,
ref
Nothing,
ref
Nothing,
ref
Nothing,
ref
Nothing,
ref
Nothing,
ref
Nothing,
ref
Nothing);
if
(isClose)
{
return
CloseDocument();
}
return
true
;
}
catch
(Exception)
{
return
false
;
}
}
#endregion
#region
- 插入页脚 -
public
static
bool
InsertPageFooter(
string
text, System.Drawing.Font font, WordPlayer.Alignment alignment)
{
try
{
oWord.ActiveWindow.View.SeekView
=
Word.WdSeekView.wdSeekCurrentPageFooter;
//
页脚
oWord.Selection.InsertAfter(text);
GetWordFont(oWord.Selection.Font, font);
SetAlignment(oWord.Selection.Range, alignment);
return
true
;
}
catch
(Exception)
{
return
false
;
}
}
public
static
bool
InsertPageFooterNumber(System.Drawing.Font font, WordPlayer.Alignment alignment)
{
try
{
oWord.ActiveWindow.View.SeekView
=
WdSeekView.wdSeekCurrentPageHeader;
oWord.Selection.WholeStory();
oWord.Selection.ParagraphFormat.Borders[WdBorderType.wdBorderBottom].LineStyle
=
WdLineStyle.wdLineStyleNone;
oWord.ActiveWindow.View.SeekView
=
Word.WdSeekView.wdSeekMainDocument;
oWord.ActiveWindow.View.SeekView
=
Word.WdSeekView.wdSeekCurrentPageFooter;
//
页脚
oWord.Selection.TypeText(
"
第
"
);
object
page
=
WdFieldType.wdFieldPage;
oWord.Selection.Fields.Add(oWord.Selection.Range,
ref
page,
ref
Nothing,
ref
Nothing);
oWord.Selection.TypeText(
"
页/共
"
);
object
pages
=
WdFieldType.wdFieldNumPages;
oWord.Selection.Fields.Add(oWord.Selection.Range,
ref
pages,
ref
Nothing,
ref
Nothing);
oWord.Selection.TypeText(
"
页
"
);
GetWordFont(oWord.Selection.Font, font);
SetAlignment(oWord.Selection.Range, alignment);
oWord.ActiveWindow.View.SeekView
=
Word.WdSeekView.wdSeekMainDocument;
return
true
;
}
catch
(Exception)
{
return
false
;
}
}
#endregion
#region
- 字体格式设定 -
public
static
void
GetWordFont(Microsoft.Office.Interop.Word.Font wordFont, System.Drawing.Font font)
{
wordFont.Name
=
font.Name;
wordFont.Size
=
font.Size;
if
(font.Bold) { wordFont.Bold
=
1
; }
if
(font.Italic) { wordFont.Italic
=
1
; }
if
(font.Underline
==
true
)
{
wordFont.Underline
=
Microsoft.Office.Interop.Word.WdUnderline.wdUnderlineNone;
}
wordFont.UnderlineColor
=
Microsoft.Office.Interop.Word.WdColor.wdColorAutomatic;
if
(font.Strikeout)
{
wordFont.StrikeThrough
=
1
;
//
删除线
}
}
#endregion
#region
- 获取Word中的颜色 -
public
static
WdColor GetWordColor(Color c)
{
UInt32 R
=
0x1
, G
=
0x100
, B
=
0x10000
;
return
(Microsoft.Office.Interop.Word.WdColor)(R
*
c.R
+
G
*
c.G
+
B
*
c.B);
}
#endregion
}
}
新建一个窗体工程,拖入一个按钮,复制下面代码进去,按F5运行
private
void
button2_Click(
object
sender, EventArgs e)
{
if
(WordPlayer.CreateWord()
==
false
)
{
MessageBox.Show(
"
文件创造失败.
"
,
"
错误提示
"
, MessageBoxButtons.OK, MessageBoxIcon.Error);
return
;
}
DataTable storedt
=
new
DataTable();
storedt.Columns.Add(
"
Book_ISBN
"
);
storedt.Columns.Add(
"
Book_Name
"
);
storedt.Columns.Add(
"
Store_Num
"
);
storedt.Columns.Add(
"
CanBorrow_Num
"
);
storedt.Columns.Add(
"
InShop_Num
"
);
storedt.Columns.Add(
"
OutShop_Num
"
);
storedt.Rows.Add(
"
1
"
,
"
1
"
,
"
1
"
,
"
1
"
,
"
1
"
,
"
1
"
);
storedt.Rows.Add(
"
2
"
,
"
2
"
,
"
2
"
,
"
2
"
,
"
2
"
,
"
2
"
);
storedt.Rows.Add(
"
3
"
,
"
3
"
,
"
3
"
,
"
3
"
,
"
3
"
,
"
3
"
);
storedt.Rows.Add(
"
4
"
,
"
4
"
,
"
4
"
,
"
4
"
,
"
4
"
,
"
4
"
);
storedt.Rows.Add(
"
5
"
,
"
5
"
,
"
5
"
,
"
5
"
,
"
5
"
,
"
5
"
);
storedt.Rows.Add(
"
6
"
,
"
6
"
,
"
6
"
,
"
6
"
,
"
6
"
,
"
6
"
);
WordPlayer.SetPage(WordPlayer.Orientation.横板,
18.4
,
26
,
3
,
2.4
,
1.87
,
2.1
);
WordPlayer.InsertText(
"
工 资 变 动 情 况 审 批 表
"
,
new
Font(
"
微软雅黑
"
,
14
, FontStyle.Bold), WordPlayer.Alignment.居中,
true
);
WordPlayer.InsertText(
""
,
new
Font(
"
微软雅黑
"
,
14
, FontStyle.Bold), WordPlayer.Alignment.居中,
true
);
WordPlayer.InsertText(
"
姓名:A 审批单位:广西师范大学
"
,
new
Font(
"
宋体
"
,
12
, FontStyle.Regular), WordPlayer.Alignment.左对齐,
false
);
WordPlayer.InsertTable(storedt,
true
);
WordPlayer.InsertText(
"
制表时间:2007年1月15日
"
,
new
Font(
"
宋体
"
,
12
, FontStyle.Regular), WordPlayer.Alignment.右对齐,
false
);
WordPlayer.Save(Application.StartupPath
+
"
\\test.doc
"
,
true
);
}
最后效果如下:
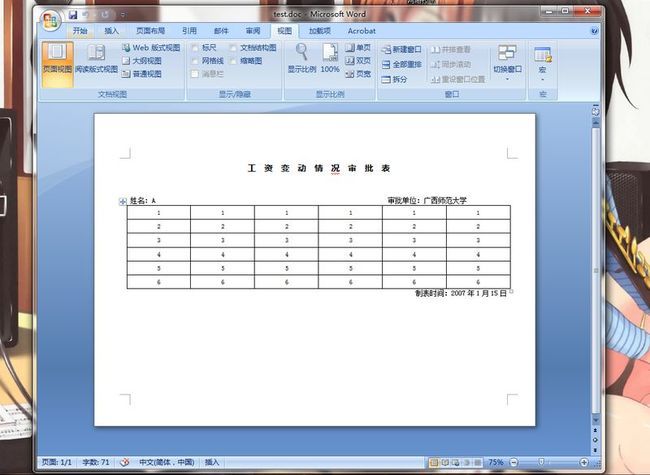