#define
_CRT_SECURE_NO_WARNINGS
1
#include
<iostream>
#include
<cstdlib>
#include
<stack>
#include
<assert.h>
using
namespace
std;
void
InitMaze(
int
*
maze
,
size_t
n
)
{
assert
(
maze
);
FILE
* fmaze = fopen(
"MazeMap.txt"
,
"r"
);
assert
(fmaze);
for
(
size_t
i = 0; i <
n
; ++i)
{
for
(
size_t
j = 0; j <
n
;)
{
char
ch = fgetc(fmaze);
if
(ch ==
'0'
|| ch ==
'1'
)
{
maze
[i*
n
+ j] = ch -
'0'
;
++j;
}
}
}
fclose(fmaze);
}
void
PrintMaze(
int
*
maze
,
size_t
n
)
{
for
(
size_t
i = 0; i <
n
; ++i)
{
for
(
size_t
j = 0; j <
n
; ++j)
{
cout <<
maze
[i*
n
+ j] <<
" "
;
}
cout << endl;
}
cout << endl;
}
struct
Pos
{
int
_row;
//行
int
_col;
//列
};
//检测访问是否越界
inline
bool
CheckIsAccess(
int
*
maze
,
int
n
,
Pos
pos
)
{
if
(
pos
._row <
n
&&
pos
._col <
n
&&
maze
[
pos
._row*
n
+
pos
._col] == 0)
{
return
true
;
}
return
false
;
}
bool
GetMazePath(
int
*
maze
,
int
n
,
Pos
entry
,
stack
<
Pos
>&
path
)
{
assert
(
maze
);
path
.push(
entry
);
maze
[
entry
._row*
n
+
entry
._col] = 2;
while
(!
path
.empty())
{
Pos
cur =
path
.top();
if
(cur._row ==
n
- 1)
{
return
true
;
}
Pos
next = cur;
next._row--;
//试探上
if
(CheckIsAccess(
maze
,
n
, next))
{
maze
[next._row*
n
+ next._col] = 2;
path
.push(next);
continue
;
}
next = cur;
next._col++;
//试探右
if
(CheckIsAccess(
maze
,
n
, next))
{
maze
[next._row*
n
+ next._col] = 2;
path
.push(next);
continue
;
}
next = cur;
next._row++;
//试探下
if
(CheckIsAccess(
maze
,
n
, next))
{
maze
[next._row*
n
+ next._col] = 2;
path
.push(next);
continue
;
}
next = cur;
next._col--;
//试探左
if
(CheckIsAccess(
maze
,
n
, next))
{
maze
[next._row*
n
+ next._col] = 2;
path
.push(next);
continue
;
}
path
.pop();
}
return
false
;
}
int
main()
{
int
maze[10][10];
InitMaze((
int
*)maze, 10);
Pos
entry = { 2, 0 };
stack
<
Pos
> path;
GetMazePath((
int
*)maze, 10, entry, path);
PrintMaze((
int
*)maze, 10);
system(
"pause"
);
return
0;
}
MazeMap.txt文件中的迷宫
1 1 1 1 1 1 1 1 1 1
1 1 1 1 1 1 1 1 0 1
0 0 0 0 0 0 0 0 0 1
1 1 0 1 1 1 1 1 1 1
1 1 0 1 1 0 0 0 0 1
1 1 0 1 1 0 1 1 0 1
1 1 0 1 1 0 1 1 0 1
1 1 0 0 0 0 1 1 0 1
1 1 0 1 1 1 1 1 0 1
1 1 0 1 1 1 1 1 1 1
|
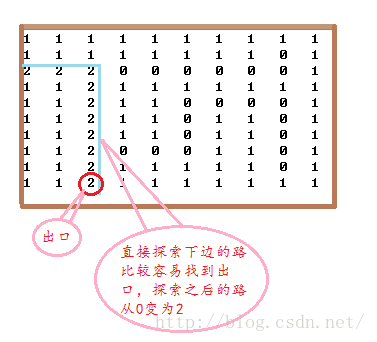
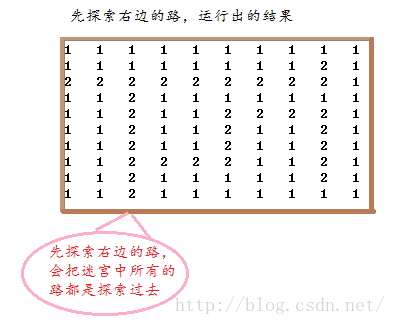