创建.aidl文件
ISipService.aidl内容如下:
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
- package com.csipsimple.api;
- import com.csipsimple.api.SipProfileState;
- import com.csipsimple.api.SipCallSession;
- import com.csipsimple.api.MediaState;
-
- interface ISipService{
-
-
-
-
-
-
- .........
- void makeCallWithOptions(in String callee, int accountId, in Bundle options);
- }
ISipService.aidl中定义了包含makeCallWithOptions
方法的接口ISipService。
自动编译生成java文件
eclipse中的ADT插件会自动在aidl文件中声明的包名目录下生成java文件,如下图所示:
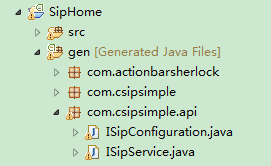
ISipService.java
- package com.csipsimple.api;
- public interface ISipService extends android.os.IInterface
- {
- ……
-
-
- ublic void makeCallWithOptions(java.lang.String callee, int accountId, android.os.Bundle options) throws android.os.RemoteException;
- }
接下来就是
实现
ISipService.aidl中定义的接口,提供接口的实例供客户端调用
IPC实现
项目中拨打电话
void com.csipsimple.api.ISipService.makeCallWithOptions(String msg, String toNumber, long accountId)
结合代码一层层看调用
目录:src\com\csipsimple\ui\dialpad
DialerFragment.java
- private ISipService service;
- private ServiceConnection connection = new ServiceConnection() {
-
- @Override
- public void onServiceConnected(ComponentName arg0, IBinder arg1) {
- service = ISipService.Stub.asInterface(arg1);
- ........
- }
-
- @Override
- public void onServiceDisconnected(ComponentName arg0) {
- service = null;
- }
- };
- <span style="color:#333333;"> @Override
- public void placeCall() {
- placeCallWithOption(null);
- }
-
- private void placeCallWithOption(Bundle b) {
- if (service == null) {
- return;
- }
- String toCall = "";
- Long accountToUse = SipProfile.INVALID_ID;
-
- SipProfile acc = accountChooserButton.getSelectedAccount();
- if (acc != null) {
- accountToUse = acc.id;
- }
-
- if(isDigit) {
- toCall = PhoneNumberUtils.stripSeparators(digits.getText().toString());
- }else {
- toCall = digits.getText().toString();
- }
-
- if (TextUtils.isEmpty(toCall)) {
- return;
- }
-
-
- digits.getText().clear();
-
-
- if (accountToUse >= 0) {
-
- try {
- </span><span style="color:#ff0000;"> service.makeCallWithOptions(toCall, accountToUse.intValue(), b);</span><span style="color:#333333;">
- } catch (RemoteException e) {
- Log.e(THIS_FILE, "Service can't be called to make the call");
- }
- } else if (accountToUse != SipProfile.INVALID_ID) {
-
- CallHandlerPlugin ch = new CallHandlerPlugin(getActivity());
- ch.loadFrom(accountToUse, toCall, new OnLoadListener() {
- @Override
- public void onLoad(CallHandlerPlugin ch) {
- placePluginCall(ch);
- }
- });
- }
- }
- </span>
这里的调用需要先了解Service的机制
service.makeCallWithOptions(toCall, accountToUse.intValue(), b)
方法调用了ISipService的方法,找到它的代码如下:
目录:src\com\csipsimple\service
- 2.服务端
- SipService.java
-
-
-
- public class SipService extends Service {
- ...
-
-
- private final ISipService.Stub binder = new ISipService.Stub() {
- ...
- @Override
- public void makeCallWithOptions(final String callee, final int accountId, final Bundle options)
- throws RemoteException {
- SipService.this.enforceCallingOrSelfPermission(SipManager.PERMISSION_USE_SIP, null);
-
- SipService.this.startService(new Intent(SipService.this, SipService.class));
-
- if(pjService == null) {
- Log.e(THIS_FILE, "Can't place call if service not started");
-
- return;
- }
-
- if(!supportMultipleCalls) {
-
- SipCallSession activeCall = pjService.getActiveCallInProgress();
- if(activeCall != null) {
- if(!CustomDistribution.forceNoMultipleCalls()) {
- notifyUserOfMessage(R.string.not_configured_multiple_calls);
- }
- return;
- }
- }
- getExecutor().execute(new SipRunnable() {
- @Override
- protected void doRun() throws SameThreadException {
- <span style="color:#ff0000;"> pjService.makeCall(callee, accountId, options);
- }</span>
- });
- }
-
/**
* 返回一个实现了接口的类对象,给客户端接收
*/
@Override
public IBinder onBind(Intent intent) {
String serviceName = intent.getAction();
Log.d(THIS_FILE, "Action is " + serviceName );
if (serviceName == null || serviceName.equalsIgnoreCase(SipManager.INTENT_SIP_SERVICE )) {
Log.d(THIS_FILE, "Service returned");
return binder ;
} else if (serviceName. equalsIgnoreCase(SipManager.INTENT_SIP_CONFIGURATION )) {
Log.d(THIS_FILE, "Conf returned");
return binderConfiguration ;
}
Log.d(THIS_FILE, "Default service (SipService) returned");
return binder;
}
...
}
上文说过,需要实现ISipService.aidl中定义的接口,来提供接口的实例供客户端调用。要实现自己的接口,就从ISipService.Stub类继承,然后实现相关的方法。
Stub类继承了Binder,因此它的对象就可以被远程的进程调用了。如果Service中有对象继承了Stub类,那么这个对象中的方法就可以在Activity等地方中使用,也就是说此时makeCallWithOptions
就可以被其他Activity访问调用了。
现在我们通过onBind(Intent intent)方法得到了可供客户端接收的IBinder对象,就可以回头看看刚才DialerFragment.java文件中的调用情况了。
在客户端(此处也就是调用远程服务的Activity)实现ServiceConnection,在ServiceConnection.onServiceConnected()方法中会接收到IBinder对象,调用ISipService.Stub.asInterface((IBinder)service)将返回值转换为ISipService类型。
语句
- <span style="color:#ff0000;">service.makeCallWithOptions(toCall, accountToUse.intValue(), b);</span><span style="font-family: Verdana, Arial, Helvetica, sans-serif;">调用接口中的方法,完成IPC方法。</span>
回到刚才的服务端实现,在继承Service发布服务的代码中,调用了 pjService.makeCall(callee, accountId, options)方法。
先看看这部分代码:
目录:src\com\csipsimple\pjsip
PjSipService.java
- <span style="color:#333333;">public int makeCall(String callee, int accountId, Bundle b) throws SameThreadException {
- if (!created) {
- return -1;
- }
-
- final ToCall toCall = sanitizeSipUri(callee, accountId);
- if (toCall != null) {
- pj_str_t uri = pjsua.pj_str_copy(toCall.getCallee());
-
-
- byte[] userData = new byte[1];
- int[] callId = new int[1];
- pjsua_call_setting cs = new pjsua_call_setting();
- pjsua_msg_data msgData = new pjsua_msg_data();
- int pjsuaAccId = toCall.getPjsipAccountId();
-
-
- pjsua.call_setting_default(cs);
- cs.setAud_cnt(1);
- cs.setVid_cnt(0);
- if(b != null && b.getBoolean(SipCallSession.OPT_CALL_VIDEO, false)) {
- cs.setVid_cnt(1);
- }
- cs.setFlag(0);
-
- pj_pool_t pool = pjsua.pool_create("call_tmp", 512, 512);
-
-
- pjsua.msg_data_init(msgData);
- pjsua.csipsimple_init_acc_msg_data(pool, pjsuaAccId, msgData);
- if(b != null) {
- Bundle extraHeaders = b.getBundle(SipCallSession.OPT_CALL_EXTRA_HEADERS);
- if(extraHeaders != null) {
- for(String key : extraHeaders.keySet()) {
- try {
- String value = extraHeaders.getString(key);
- if(!TextUtils.isEmpty(value)) {
- int res = pjsua.csipsimple_msg_data_add_string_hdr(pool, msgData, pjsua.pj_str_copy(key), pjsua.pj_str_copy(value));
- if(res == pjsuaConstants.PJ_SUCCESS) {
- Log.e(THIS_FILE, "Failed to add Xtra hdr (" + key + " : " + value + ") probably not X- header");
- }
- }
- }catch(Exception e) {
- Log.e(THIS_FILE, "Invalid header value for key : " + key);
- }
- }
- }
- }
-
- </span><span style="color:#ff0000;">int status = pjsua.call_make_call(pjsuaAccId, uri, cs, userData, msgData, callId);</span><span style="color:#333333;">
- if(status == pjsuaConstants.PJ_SUCCESS) {
- dtmfToAutoSend.put(callId[0], toCall.getDtmf());
- Log.d(THIS_FILE, "DTMF - Store for " + callId[0] + " - "+toCall.getDtmf());
- }
- pjsua.pj_pool_release(pool);
- return status;
- } else {
- service.notifyUserOfMessage(service.getString(R.string.invalid_sip_uri) + " : "
- + callee);
- }
- return -1;
- }</span>
由红色部分的语句,我们找到pjsua类。
目录:src\org\pjsip\pjsua
pjsua.java
- package org.pjsip.pjsua;
-
- public class pjsua implements pjsuaConstants {
- public synchronized static int call_make_call(int acc_id, pj_str_t dst_uri, pjsua_call_setting opt, byte[] user_data, pjsua_msg_data msg_data, int[] p_call_id) {
- <span style="color:#ff0000;"> return pjsuaJNI.call_make_call</span>(acc_id, pj_str_t.getCPtr(dst_uri), dst_uri, pjsua_call_setting.getCPtr(opt), opt, user_data, pjsua_msg_data.getCPtr(msg_data), msg_data, p_call_id);
- }
- ..........
- }
继续看调用,找到pjsuaJNI文件。
目录:src\org\pjsip\pjsua
pjsuaJNI.java
/* ----------------------------------------------------------------------------
* This file was automatically generated by SWIG (http://www.swig.org).
* Version 2.0.4
*
* Do not make changes to this file unless you know what you are doing--modify
* the SWIG interface file instead.
* ----------------------------------------------------------------------------- */
package org.pjsip.pjsua;
public class pjsuaJNI {
...
public final static native int call_make_call(int jarg1, long jarg2, pj_str_t jarg2_, long jarg3, pjsua_call_setting jarg3_, byte[] jarg4, long jarg5, pjsua_msg_data jarg5_, int[] jarg6);
...
}
我们看到了native方法call_make_call,它调用的是封装在库libpjsipjni.so中的函数pjsua_call_make_call,进一步可以在jni目录下找到C代码。
目录:jni\pjsip\sources\pjsip\src\pjsua-lib
pjsua_call.c
- PJ_DEF(pj_status_t) pjsua_call_make_call(pjsua_acc_id acc_id,
- const pj_str_t *dest_uri,
- const pjsua_call_setting *opt,
- void *user_data,
- const pjsua_msg_data *msg_data,
- pjsua_call_id *p_call_id)
- {
- pj_pool_t *tmp_pool = NULL;
- pjsip_dialog *dlg = NULL;
- pjsua_acc *acc;
- pjsua_call *call;
- int call_id = -1;
- pj_str_t contact;
- pj_status_t status;
-
-
-
- PJ_ASSERT_RETURN(acc_id>=0 || acc_id<(int)PJ_ARRAY_SIZE(pjsua_var.acc),
- PJ_EINVAL);
-
-
- PJ_ASSERT_RETURN(dest_uri, PJ_EINVAL);
-
- PJ_LOG(4,(THIS_FILE, "Making call with acc #%d to %.*s", acc_id,
- (int)dest_uri->slen, dest_uri->ptr));
-
- pj_log_push_indent();
-
- PJSUA_LOCK();
-
-
-
-
-
-
- if (!pjsua_var.is_mswitch && pjsua_var.snd_port==NULL &&
- pjsua_var.null_snd==NULL && !pjsua_var.no_snd)
- {
- status = pjsua_set_snd_dev(pjsua_var.cap_dev, pjsua_var.play_dev);
- if (status != PJ_SUCCESS)
- goto on_error;
- }
-
- acc = &pjsua_var.acc[acc_id];
- if (!acc->valid) {
- pjsua_perror(THIS_FILE, "Unable to make call because account "
- "is not valid", PJ_EINVALIDOP);
- status = PJ_EINVALIDOP;
- goto on_error;
- }
-
-
- call_id = alloc_call_id();
-
- if (call_id == PJSUA_INVALID_ID) {
- pjsua_perror(THIS_FILE, "Error making call", PJ_ETOOMANY);
- status = PJ_ETOOMANY;
- goto on_error;
- }
-
- call = &pjsua_var.calls[call_id];
-
-
- call->acc_id = acc_id;
- call->call_hold_type = acc->cfg.call_hold_type;
-
-
- status = apply_call_setting(call, opt, NULL);
- if (status != PJ_SUCCESS) {
- pjsua_perror(THIS_FILE, "Failed to apply call setting", status);
- goto on_error;
- }
-
-
- tmp_pool = pjsua_pool_create("tmpcall10", 512, 256);
-
-
-
-
-
-
- if (1) {
- pjsip_uri *uri;
- pj_str_t dup;
-
- pj_strdup_with_null(tmp_pool, &dup, dest_uri);
- uri = pjsip_parse_uri(tmp_pool, dup.ptr, dup.slen, 0);
-
- if (uri == NULL) {
- pjsua_perror(THIS_FILE, "Unable to make call",
- PJSIP_EINVALIDREQURI);
- status = PJSIP_EINVALIDREQURI;
- goto on_error;
- }
- }
-
-
- pj_gettimeofday(&call->start_time);
-
-
- call->res_time.sec = 0;
-
-
-
-
- if (acc->contact.slen) {
- contact = acc->contact;
- } else {
- status = pjsua_acc_create_uac_contact(tmp_pool, &contact,
- acc_id, dest_uri);
- if (status != PJ_SUCCESS) {
- pjsua_perror(THIS_FILE, "Unable to generate Contact header",
- status);
- goto on_error;
- }
- }
-
-
- status = pjsip_dlg_create_uac( pjsip_ua_instance(),
- &acc->cfg.id, &contact,
- dest_uri, dest_uri, &dlg);
- if (status != PJ_SUCCESS) {
- pjsua_perror(THIS_FILE, "Dialog creation failed", status);
- goto on_error;
- }
-
-
-
-
- pjsip_dlg_inc_lock(dlg);
-
- if (acc->cfg.allow_via_rewrite && acc->via_addr.host.slen > 0)
- pjsip_dlg_set_via_sent_by(dlg, &acc->via_addr, acc->via_tp);
-
-
- call->secure_level = get_secure_level(acc_id, dest_uri);
-
-
- call->user_data = user_data;
-
-
-
-
- if (msg_data) {
- call->async_call.call_var.out_call.msg_data = pjsua_msg_data_clone(
- dlg->pool, msg_data);
- }
- call->async_call.dlg = dlg;
-
-
-
-
-
- pjsip_dlg_inc_session(dlg, &pjsua_var.mod);
-
-
- status = pjsua_media_channel_init(call->index, PJSIP_ROLE_UAC,
- call->secure_level, dlg->pool,
- NULL, NULL, PJ_TRUE,
- &on_make_call_med_tp_complete);
- if (status == PJ_SUCCESS) {
- status = on_make_call_med_tp_complete(call->index, NULL);
- if (status != PJ_SUCCESS)
- goto on_error;
- } else if (status != PJ_EPENDING) {
- pjsua_perror(THIS_FILE, "Error initializing media channel", status);
- pjsip_dlg_dec_session(dlg, &pjsua_var.mod);
- goto on_error;
- }
-
-
-
- if (p_call_id)
- *p_call_id = call_id;
-
- pjsip_dlg_dec_lock(dlg);
- pj_pool_release(tmp_pool);
- PJSUA_UNLOCK();
-
- pj_log_pop_indent();
-
- return PJ_SUCCESS;
-
-
- on_error:
- if (dlg) {
-
- pjsip_dlg_dec_lock(dlg);
- }
-
- if (call_id != -1) {
- reset_call(call_id);
- pjsua_media_channel_deinit(call_id);
- }
-
- if (tmp_pool)
- pj_pool_release(tmp_pool);
- PJSUA_UNLOCK();
-
- pj_log_pop_indent();
- return status;
- }
- 通过本文的研究分析,我们了解到CSipSimple通过aidl方法实现进程间通信,从而实现了拨打电话功能。