import numpy as np
import tensorflow as tf
import matplotlib.pyplot as plt
date = np.linspace(0, 15, 15)
endPrice = np.array([114.90, 113.82, 116.56, 108.38, 112.43,
120.23, 120.90, 121.34, 121.00, 122.64, 122.06, 119.62, 121.93, 122.15, 120.97])
beginPrice = np.array([116.43, 114.89, 114.26, 119.22, 109.47,
114.72, 119.93, 121.60, 120.48, 120.24, 122.66, 123.00, 121.00, 122.97, 122.87])
for i in range(15):
dateOne = np.zeros([2])
dateOne[0] = i
dateOne[1] = i
priceOne = np.zeros([2])
priceOne[0] = beginPrice[i]
priceOne[1] = endPrice[i]
if endPrice[i] - beginPrice[i] > 0:
plt.plot(dateOne, priceOne, 'r', lw=8)
else:
plt.plot(dateOne, priceOne, 'g', lw=8)
dateNormal = np.zeros([15, 1])
priceNormal = np.zeros([15, 1])
for i in range(15):
dateNormal[i, 0] = i / 14.0
priceNormal[i, 0] = endPrice[i] / 150
with tf.device('/gpu:0'):
x = tf.placeholder(tf.float32, [None, 1])
y = tf.placeholder(tf.float32, [None, 1])
w1 = tf.Variable(tf.random_uniform([1, 10], 0, 1))
b1 = tf.Variable(tf.zeros([1, 10]))
z1 = tf.matmul(x, w1) + b1
a1 = tf.nn.relu(z1)
w2 = tf.Variable(tf.random_uniform([10, 1], 0, 1))
b2 = tf.Variable(tf.zeros([15, 1]))
z2 = tf.matmul(a1, w2) + b2
a2 = tf.nn.relu(z2)
loss = tf.reduce_mean(tf.square(y - a2))
train_step = tf.train.GradientDescentOptimizer(0.1).minimize(loss)
with tf.Session(config=tf.ConfigProto(allow_soft_placement=True, log_device_placement=True)) as sess:
sess.run(tf.global_variables_initializer())
for i in range(10000):
sess.run(train_step, feed_dict={x: dateNormal, y: priceNormal})
pred = sess.run(a2, feed_dict={x: dateNormal})
predPrice = np.zeros([15, 1])
for i in range(15):
predPrice[i, 0] = (pred * 150)[i, 0]
plt.plot(date, predPrice, 'b', lw=1)
plt.show()
好久没有用tensorflow框架了,今天用起来感觉有点手生!!代码以后要长写!
数据用的是新东方的股票走势
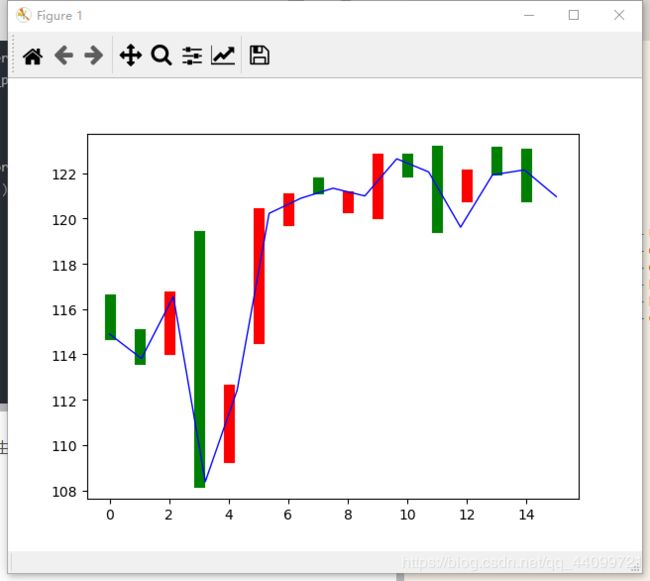