public class BeatBox {
JFrame frame;
JPanel panel;
ArrayList<JCheckBox> checkBoxList;
Sequencer sequencer;
Sequence sequence;
Track track;
int[] instruments = {35, 42, 46, 38, 49, 39, 50, 60, 70, 72, 64, 56, 58, 47, 67, 63};
String[] instrumentName = {"Bass Drum", "Cloud Hi-Hat", "Open Hi-Hat", "Acoustic Snare", "Crash Cymbal", "Hand Clap", "High Tom",
"Hi Bingo", "Marcacas", "Whistle", "Low Conga", "Cowbel", "Vibraslap", "Low-mid Tom", "Hi Agogo", "Open Hi Conga"};
public static void main(String[] args) {
new BeatBox().gui();
}
public void gui(){
frame = new JFrame("Beat-Box");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
BorderLayout layout = new BorderLayout();
JPanel background = new JPanel(layout);
background.setBorder(BorderFactory.createEmptyBorder(10, 10, 10, 10));
checkBoxList = new ArrayList<JCheckBox>();
Box buttonBox = new Box(BoxLayout.Y_AXIS);
JButton start = new JButton("Start");
start.addActionListener(new MyStartListener());
buttonBox.add(start);
JButton stop = new JButton("Stop");
stop.addActionListener(new MyStopListener());
buttonBox.add(stop);
JButton upTempo = new JButton("Tempo up");
upTempo.addActionListener(new MyUpTempoListener());
buttonBox.add(upTempo);
JButton downTempo = new JButton("Tempo down");
downTempo.addActionListener(new MyDownTempoListener());
buttonBox.add(downTempo);
background.add(BorderLayout.EAST, buttonBox);
Box nameBox = new Box(BoxLayout.Y_AXIS);
for(int i = 0; i != 16; ++i){
nameBox.add(new Label(instrumentName[i]));
}
background.add(BorderLayout.WEST, nameBox);
frame.getContentPane().add(background);
GridLayout grid = new GridLayout(16, 16);
grid.setHgap(1);
grid.setVgap(2);
panel = new JPanel(grid);
background.add(BorderLayout.CENTER, panel);
for(int i = 0; i != 256; ++i){
JCheckBox c = new JCheckBox();
c.setSelected(false);
checkBoxList.add(c);
panel.add(c);
}
setUpmidi();
frame.setBounds(50, 50, 300, 300);
frame.pack();
frame.setVisible(true);
}
public void buildTrackAndStart(){
sequence.deleteTrack(track);
track = sequence.createTrack();
int[] trackList = new int[16];
for(int i = 0; i != 16; ++i){
int key = instruments[i];
for(int j = 0; j != 16; ++j){
JCheckBox jc = (JCheckBox) checkBoxList.get(j + 16 * i);
if(jc.isSelected()){
trackList[j] = key;
} else {
trackList[j] = 0;
}
}
makeTracks(trackList);
track.add(makeEvent(176, 1, 127, 0, 16));
}
track.add(makeEvent(192, 9, 1, 0, 15));
try{
sequencer.setSequence(sequence);
sequencer.setLoopCount(Sequencer.LOOP_CONTINUOUSLY);
sequencer.start();
sequencer.setTempoInBPM(120);
}catch(Exception e){ e.printStackTrace();}
}
public MidiEvent makeEvent(int comd, int chan, int one, int two, int tick){
MidiEvent event = null;
try{
ShortMessage message = new ShortMessage();
message.setMessage(comd, chan, one, two);
event = new MidiEvent(message, tick);
} catch (Exception ex){
ex.printStackTrace();
}
return event;
}
public void makeTracks(int[] list){
for(int i = 0; i != 16; ++i){
int key = list[i];
if(key != 0){
track.add(makeEvent(144, 9, key, 100, i));
track.add(makeEvent(128, 9, key, 100, i+1));
}
}
}
public class MyStopListener implements ActionListener{
public void actionPerformed(ActionEvent event){
sequencer.stop();
}
}
public class MyUpTempoListener implements ActionListener{
public void actionPerformed(ActionEvent event){
float tempoFactor = sequencer.getTempoFactor();
sequencer.setTempoFactor((float) (tempoFactor * 1.03));
}
}
public class MyDownTempoListener implements ActionListener{
public void actionPerformed(ActionEvent event){
float tempoFactor = sequencer.getTempoFactor();
sequencer.setTempoFactor((float) (tempoFactor * 0.97));
}
}
public void setUpmidi(){
try{
sequencer = MidiSystem.getSequencer();
sequencer.open();
sequence = new Sequence(Sequence.PPQ, 4);
track = sequence.createTrack();
sequencer.setTempoInBPM(120);
} catch (Exception ex){ ex.printStackTrace(); }
}
public class MyStartListener implements ActionListener {
public void actionPerformed(ActionEvent event){
buildTrackAndStart();
}
}
}
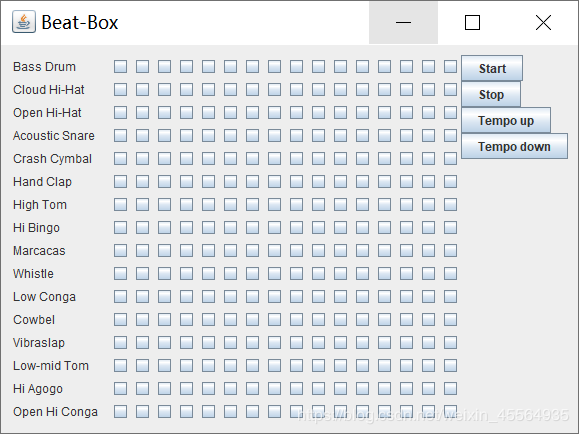