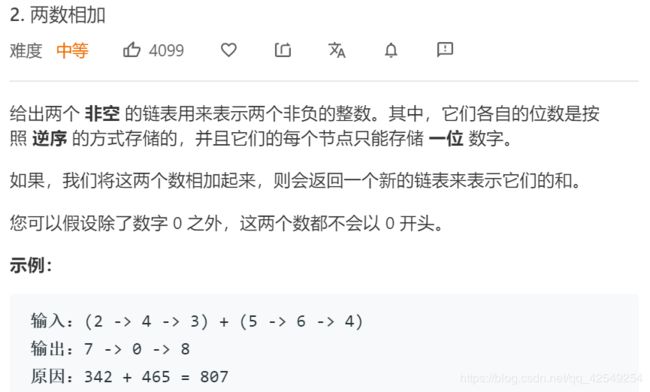
class Solution {
public:
ListNode* addTwoNumbers(ListNode* l1, ListNode* l2) {
ListNode* dummy = new ListNode(-1);
ListNode* pre = dummy;
int carry = 0;
while(l1 || l2)
{
int res = carry;
int a = l1 ? l1->val : 0;
int b = l2 ? l2->val : 0;
res += a + b;
carry = res / 10;
int t = res % 10;
pre->next = new ListNode(t);
pre = pre->next;
if(l1) l1 = l1->next;
if(l2) l2 = l2->next;
}
if(carry) pre->next = new ListNode(1);
return dummy->next;
}
};
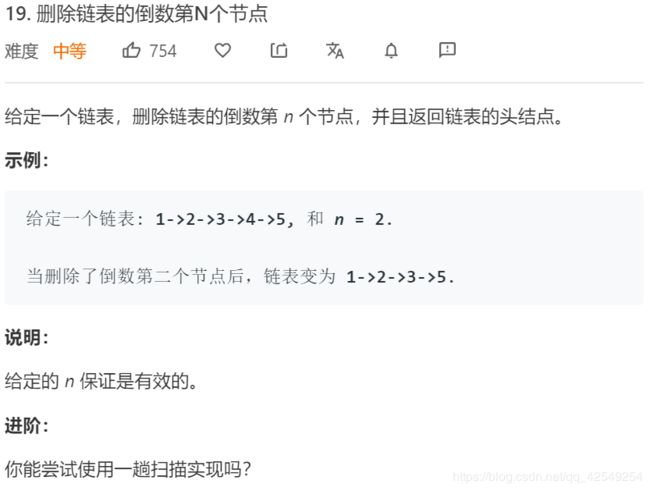
class Solution {
public:
ListNode* removeNthFromEnd(ListNode* head, int n) {
ListNode *dummy = new ListNode(-1);
dummy->next = head;
ListNode *first = dummy, *second = dummy;
for(int i = 0; i < n; i ++) first = first->next;
while(first->next)
{
first = first->next;
second = second->next;
}
second->next = second->next->next;
return dummy->next;
}
};
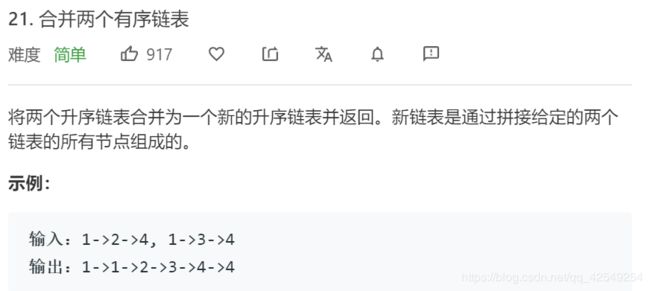
class Solution {
public:
ListNode* mergeTwoLists(ListNode* l1, ListNode* l2) {
ListNode* dummy = new ListNode(-1);
ListNode* pre = dummy;
while(l1 && l2)
{
if(l1->val < l2->val)
{
pre->next = l1;
l1 = l1->next;
}
else
{
pre->next = l2;
l2 = l2->next;
}
pre = pre->next;
}
if(l1) pre->next = l1;
if(l2) pre->next = l2;
return dummy->next;
}
};
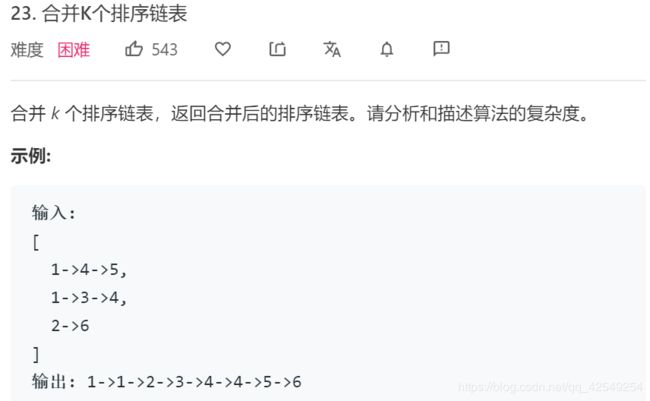
class Solution {
public:
ListNode* mergeKLists(vector<ListNode*>& lists) {
if(lists.size() == 0) return NULL;
if(lists.size() == 1) return lists[0];
if(lists.size() == 2) return mergeTwoLists(lists[0], lists[1]);
int mid = lists.size() / 2;
vector<ListNode*> sub1_lists;
vector<ListNode*> sub2_lists;
for(int i = 0; i < mid; i ++)
sub1_lists.push_back(lists[i]);
for(int i = mid; i < lists.size(); i ++)
sub2_lists.push_back(lists[i]);
ListNode* l1 = mergeKLists(sub1_lists);
ListNode* l2 = mergeKLists(sub2_lists);
return mergeTwoLists(l1, l2);
}
ListNode* mergeTwoLists(ListNode* l1, ListNode* l2)
{
ListNode* dummy = new ListNode(-1);
ListNode* head = dummy;
while(l1 && l2)
{
if(l1->val <= l2->val)
{
head->next = l1;
l1 = l1->next;
}
else
{
head->next = l2;
l2 = l2->next;
}
head = head->next;
}
head->next = (l1 == NULL) ? l2 : l1;
return dummy->next;
}
};
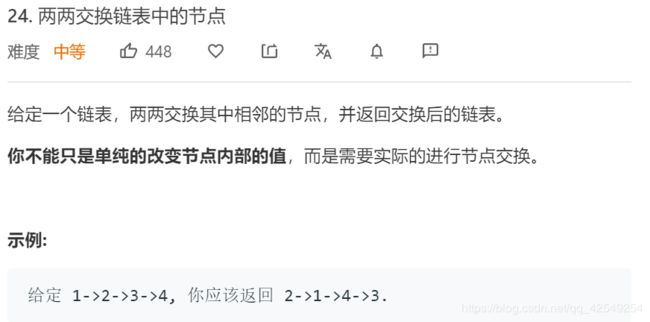
class Solution {
public:
ListNode* swapPairs(ListNode* head) {
if(head == NULL || head->next == NULL) return head;
ListNode* headNext = head->next;
head->next = swapPairs(headNext->next);
headNext->next = head;
return headNext;
}
};
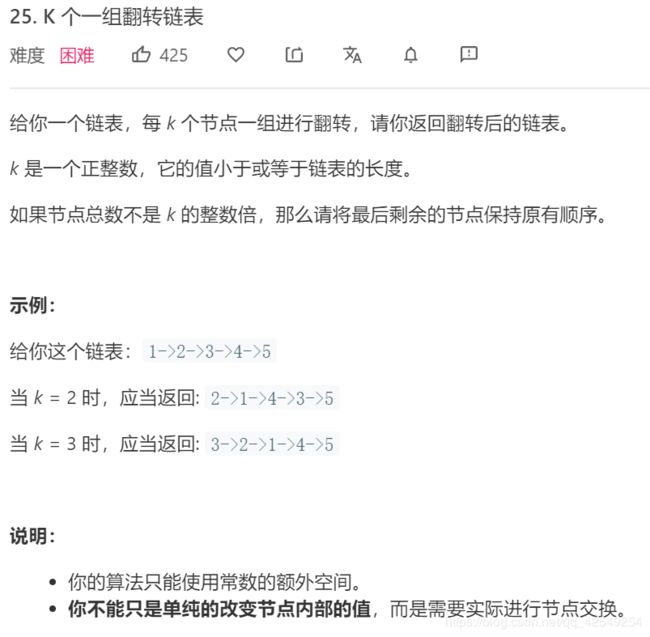
class Solution {
public:
ListNode* reverseKGroup(ListNode* head, int k) {
ListNode* pre = NULL;
ListNode* next = NULL;
ListNode* cur = head;
ListNode* check = head;
int count = 0, len = 0;
while(len < k && check)
{
check = check->next;
len ++;
}
if(len == k)
{
while(count < k && cur)
{
next = cur->next;
cur->next = pre;
pre = cur;
cur = next;
count ++;
}
if(next)
head->next = reverseKGroup(next, k);
return pre;
}
else return head;
}
};
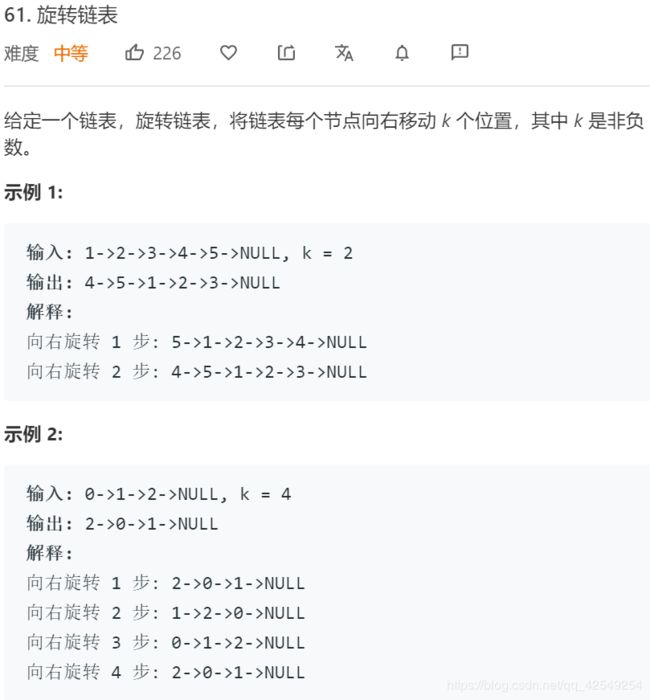
class Solution {
public:
ListNode* rotateRight(ListNode* head, int k) {
if(head == NULL) return head;
ListNode* tail;
ListNode*a = head;
int n = 0;
for(auto p = head; p; p = p->next)
{
n ++;
tail = p;
}
k %= n;
if(!k) return head;
for(int i = 0; i < n - 1 - k; i ++) a = a->next;
ListNode* next = a->next;
tail->next = head;
a->next = NULL;
return next;
}
};
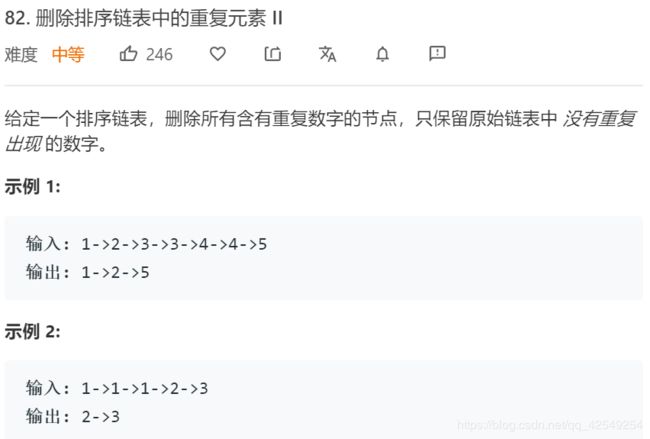
class Solution {
public:
ListNode* deleteDuplicates(ListNode* head) {
ListNode*dummy = new ListNode(-1);
dummy->next = head;
ListNode*p = dummy;
while(p->next)
{
ListNode* q = p->next;
while(q && q->val == p->next->val)
q = q->next;
if(p->next->next == q) p = p->next;
else p->next = q;
}
return dummy->next;
}
};
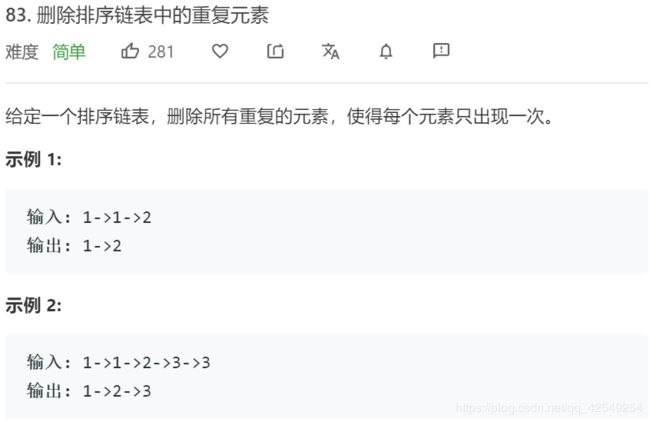
class Solution {
public:
ListNode* deleteDuplicates(ListNode* head) {
if(!head) return 0;
ListNode* p = head, *q = head;
while(p)
{
if(p->val != q->val)
{
q->next = p;
q = p;
}
p = p->next;
}
q->next = NULL;
return head;
}
};
class Solution {
public:
ListNode* deleteDuplicates(ListNode* head) {
ListNode*dummmy = new ListNode(-111111111);
dummmy->next = head;
ListNode*pre = dummmy;
while(pre->next)
{
if(pre->val == pre->next->val) pre->next = pre->next->next;
else pre = pre->next;
}
return dummmy->next;
}
};
class Solution {
public:
ListNode* deleteDuplicates(ListNode* head) {
if(head == NULL) return head;
ListNode*a = head;
while(a->next)
{
if(a->val == a->next->val) a->next = a->next->next;
else a = a->next;
}
return head;
}
};
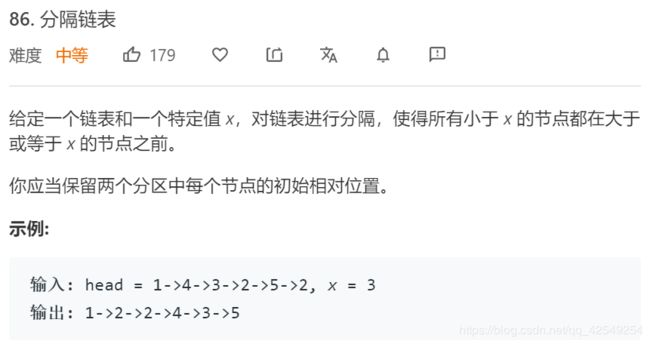
class Solution {
public:
ListNode* partition(ListNode* head, int x) {
ListNode* before = new ListNode(-1);
ListNode* after = new ListNode(-1);
ListNode* pb = before, *pa = after;
for(ListNode* p = head; p; p = p->next)
{
if(p->val < x)
{
pb->next = p;
pb = p;
}
else{
pa->next = p;
pa = p;
}
}
pb->next = after->next;
pa->next = NULL;
return before->next;
}
};
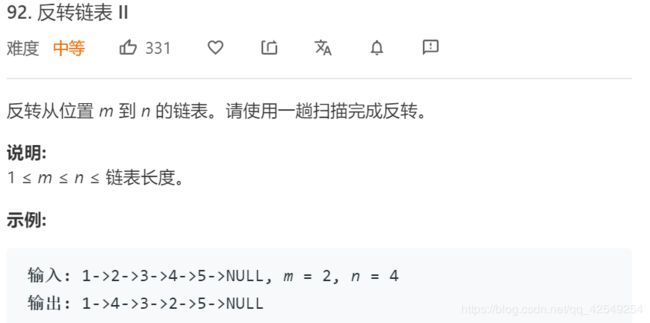
class Solution {
public:
ListNode* reverseBetween(ListNode* head, int m, int n) {
if(m == n) return head;
if(!head) return 0;
ListNode* dummy = new ListNode(-1);
dummy->next = head;
ListNode* a = dummy;
for(int i = 0; i < m - 1; i ++) a = a->next;
ListNode* b = a;
b = b->next;
ListNode* c = b->next;
for(int i = 0; i < n - m; i ++)
{
ListNode* next = c->next;
c->next = b;
b = c;
c = next;
}
ListNode* an = a->next;
a->next = b;
an->next = c;
return dummy->next;
}
};
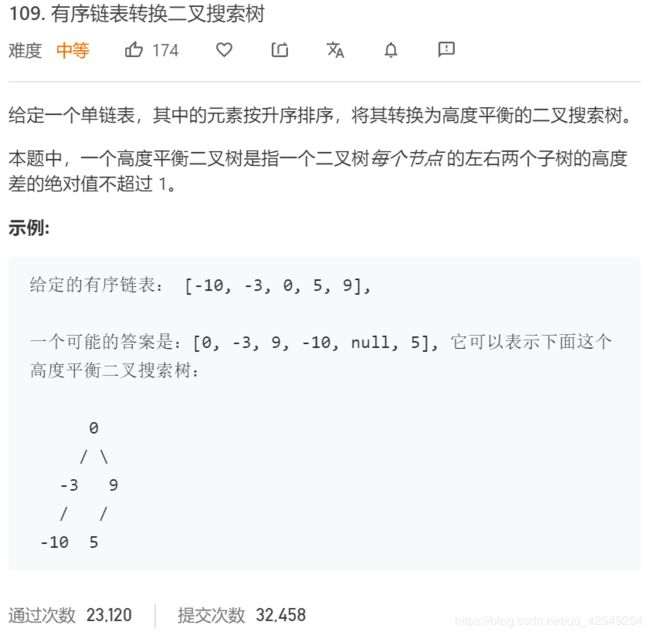
class Solution {
public:
TreeNode* sortedListToBST(ListNode* head) {
if(!head) return 0;
return dfs(head, 0);
}
TreeNode* dfs(ListNode* head, ListNode* tail)
{
if(head == tail) return 0;
ListNode* slow = head;
ListNode* fast = head;
while(fast != tail && fast->next != tail)
{
slow = slow->next;
fast = fast->next->next;
}
TreeNode* root = new TreeNode(slow->val);
root->left = dfs(head, slow);
root->right = dfs(slow->next, tail);
return root;
}
};
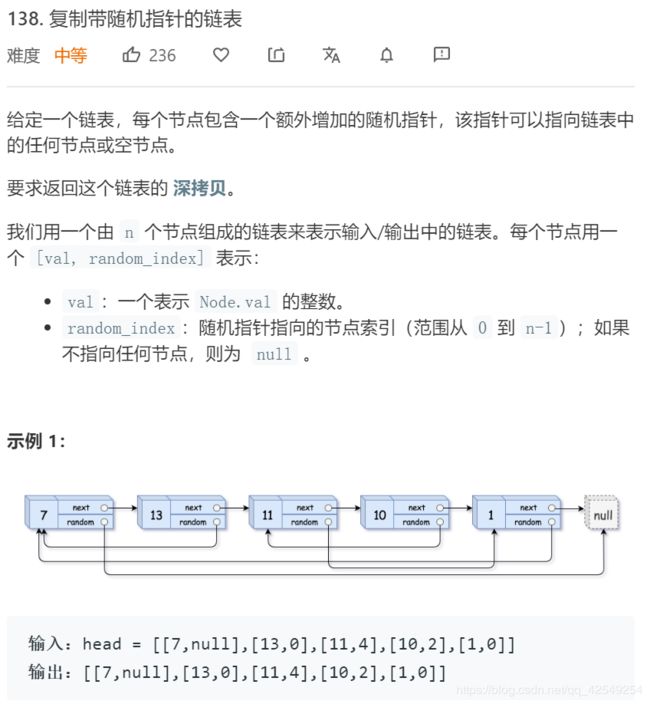
class Solution {
public:
Node* copyRandomList(Node* head) {
if(!head) return head;
Node* p = head, *tempP;
while(p)
{
tempP = new Node(p->val, p->next, p->random);
tempP->next = p->next;
p->next = tempP;
p = p->next->next;
}
p = head;
while(p)
{
if(p->random)
{
p->next->random = p->random->next;
}
else p->next->random = NULL;
p = p->next->next;
}
p = head;
Node* copyHead = p->next;
while(p)
{
tempP = p->next;
p->next = p->next->next;
if(tempP->next)
tempP->next = tempP->next->next;
p = p->next;
}
return copyHead;
}
};
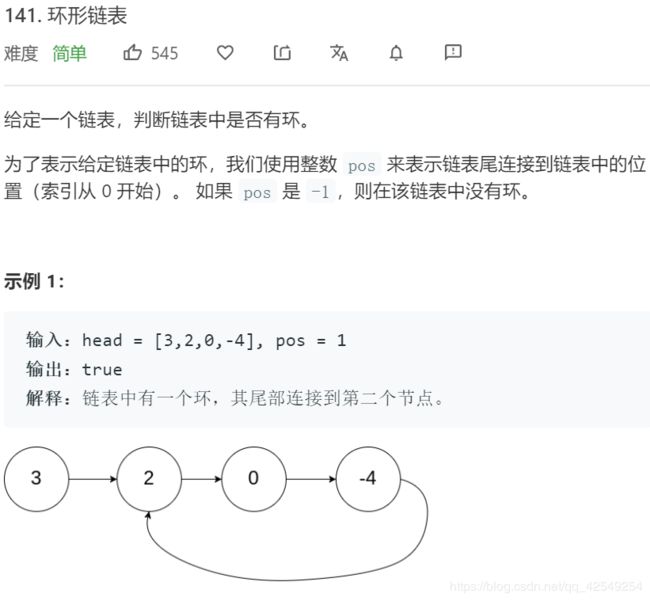
class Solution {
public:
bool hasCycle(ListNode *head) {
if(!head || !head->next) return 0;
ListNode* a = head, *b = head->next;
while(a && b)
{
if(a == b) return true;
a = a->next;
b = b->next;
if(b) b = b->next;
}
return false;
}
};
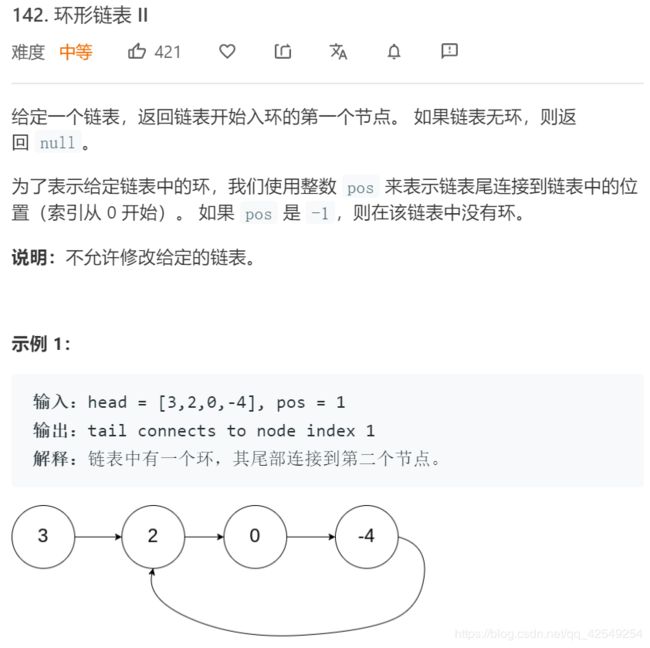
class Solution {
public:
ListNode *detectCycle(ListNode *head) {
if(!head) return head;
ListNode* a = head, *b = head;
while(a && b)
{
a = a->next;
b = b->next;
if(b) b = b->next;
else return 0;
if(a == b)
{
a = head;
while(a != b)
{
a = a->next;
b = b->next;
}
return b;
}
}
return 0;
}
};
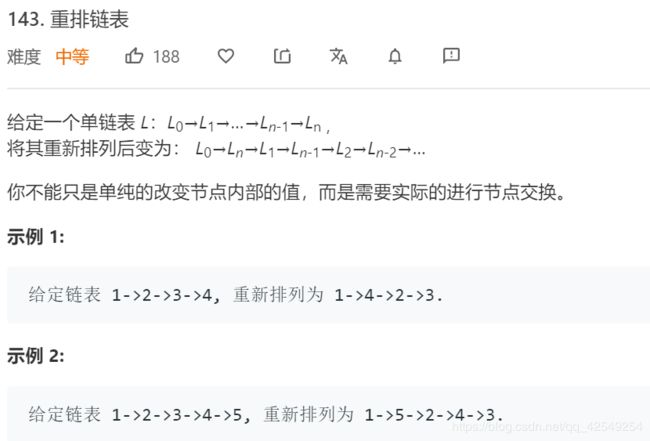
class Solution {
public:
void reorderList(ListNode* head) {
if(!head) return;
int n = 0;
for(auto p = head; p; p = p->next) n ++;
if(n <= 2) return;
ListNode* mid = head;
for(int i = 0; i < (n + 1) / 2 - 1; i ++) mid = mid->next;
ListNode* a = mid->next;
mid->next = NULL;
ListNode* b = a->next;
a->next = NULL;
while(b)
{
ListNode* next = b->next;
b->next = a;
a = b;
b = next;
}
b = head;
while(a)
{
ListNode* next = a->next;
a->next = b->next;
b->next = a;
a = next;
b = b->next->next;
}
}
};

class Solution {
public:
ListNode* insertionSortList(ListNode* head) {
ListNode *dummy = new ListNode(-1);
while(head)
{
ListNode*p = dummy;
while(p->next && p->next->val <= head->val) p = p->next;
ListNode* next = head->next;
head->next = p->next;
p->next = head;
head = next;
}
return dummy->next;
}
};
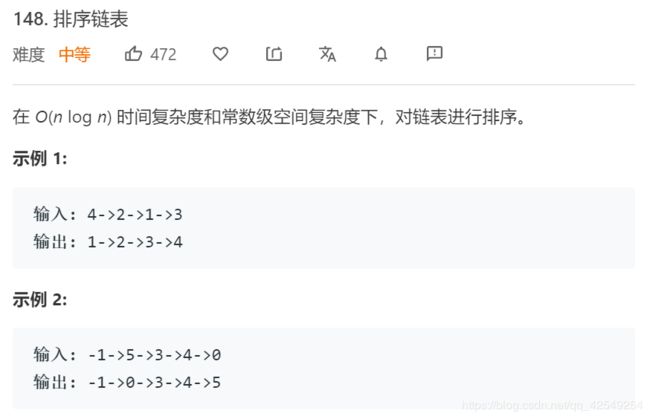
class Solution {
public:
ListNode* sortList(ListNode* head) {
return head == NULL ? NULL : mergeSort(head);
}
ListNode* mergeSort(ListNode* node)
{
if(!node->next) return node;
ListNode* a = node, *b = node;
ListNode* breakN = node;
while(a && a->next)
{
a = a->next->next;
breakN = b;
b = b->next;
}
breakN->next = NULL;
ListNode*l = mergeSort(node);
ListNode*r = mergeSort(b);
return mergeTwoList(l, r);
}
ListNode* mergeTwoList(ListNode*l1, ListNode*l2)
{
ListNode*dummy = new ListNode(-1);
ListNode*cur = dummy;
while(l1 && l2)
{
if(l1->val <= l2->val)
{
cur->next = l1;
l1 = l1->next;
}
else
{
cur->next = l2;
l2 = l2->next;
}
cur = cur->next;
}
cur->next = (l1 == NULL ? l2 : l1);
return dummy->next;
}
};
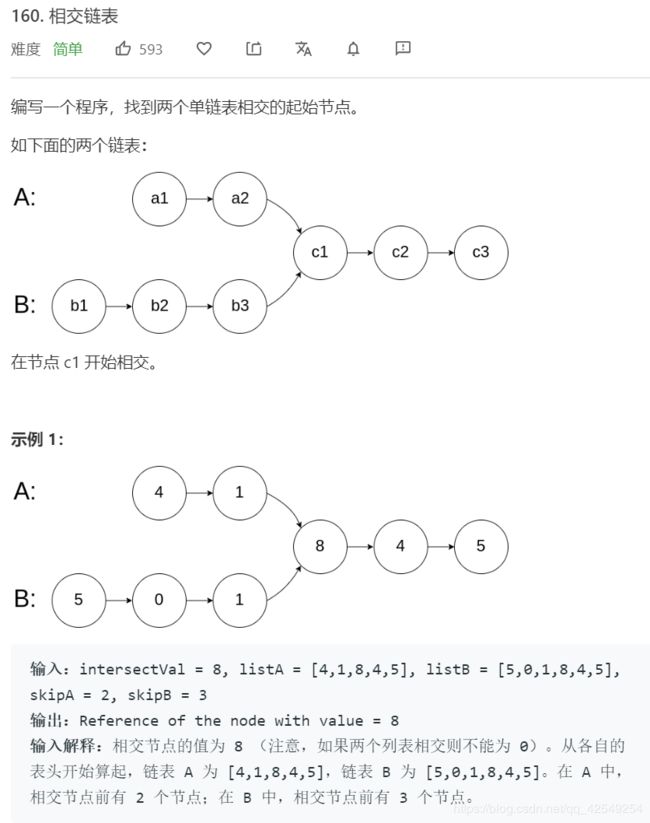
class Solution {
public:
ListNode *getIntersectionNode(ListNode *headA, ListNode *headB) {
ListNode* a = headA, *b = headB;
while(a != b)
{
if(!a) a = headB;
else a = a->next;
if(!b) b = headA;
else b = b->next;
}
return a;
}
};
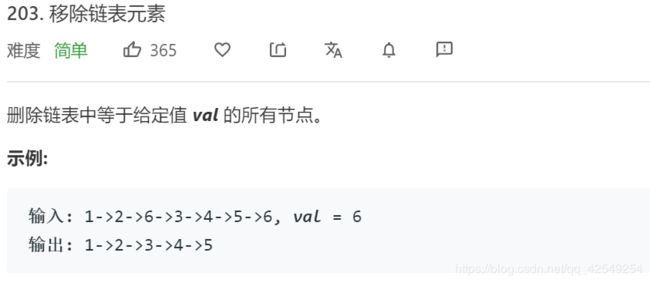
class Solution {
public:
ListNode* removeElements(ListNode* head, int val) {
ListNode *dummy = new ListNode(-1);
dummy->next = head;
for(ListNode *p = dummy; p; )
{
if(p->next && p->next->val == val)
p->next = p->next->next;
else
p = p->next;
}
return dummy->next;
}
};
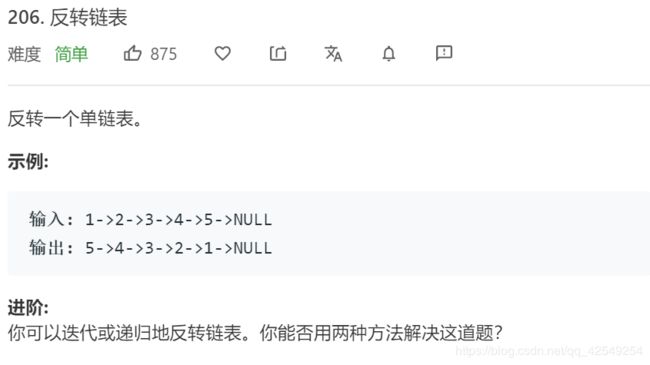
class Solution {
public:
ListNode* reverseList(ListNode* head) {
if(!head) return head;
ListNode *p = NULL;
while(head)
{
ListNode *next = head->next;
head->next = p;
p = head;
head = next;
}
return p;
}
};
class Solution {
public:
ListNode* reverseList(ListNode* head) {
if(!head || !head->next) return head;
ListNode *p = reverseList(head->next);
head->next->next = head;
head->next = NULL;
return p;
}
};
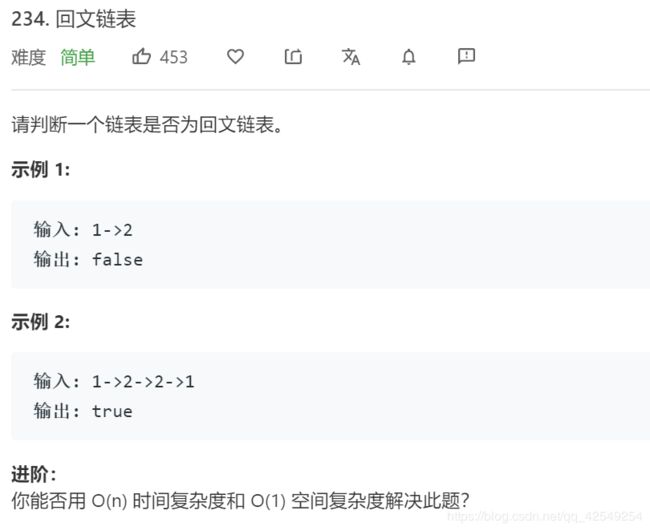
class Solution {
public:
bool isPalindrome(ListNode* head) {
ListNode *fast = head, *slow = head, *pre = NULL;
while(fast)
{
slow = slow->next;
fast = fast->next ? fast->next->next : fast->next;
}
while(slow)
{
ListNode *next = slow->next;
slow->next = pre;
pre = slow;
slow = next;
}
while(head && pre)
{
if(head->val != pre->val)
return false;
head = head->next;
pre = pre->next;
}
return true;
}
};
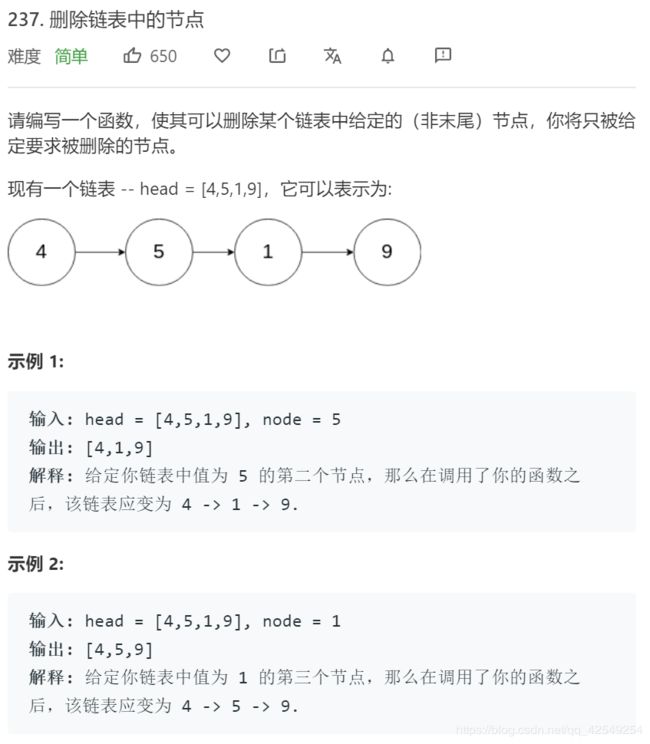
class Solution {
public:
void deleteNode(ListNode* node) {
node->val = node->next->val;
node->next = node->next->next;
}
};
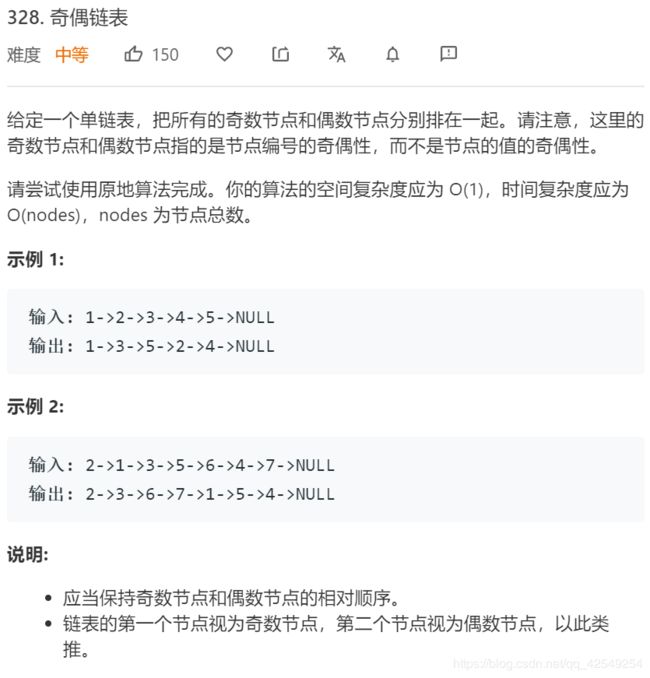
class Solution {
public:
ListNode* oddEvenList(ListNode* head) {
if(head == NULL || head->next == NULL)
return head;
ListNode *o = head;
ListNode *e = head->next;
ListNode *p = e;
while(o->next && e->next)
{
o->next = e->next;
o = o->next;
e->next = o->next;
e = e->next;
}
o->next = p;
return head;
}
};