代码如下:
struct Vertex {
unsigned char r, g, b, a;
float x, y, z;
};
static void render(GLFWwindow * window) {
glClearColor(0, 0, 0, 1);
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
Vertex cubeVertices[] =
{
{ 255, 0, 0, 255, -1.0f, -1.0f, 1.0f },
{ 255, 0, 0, 255, 1.0f, -1.0f, 1.0f },
{ 255, 0, 0, 255, 1.0f, 1.0f, 1.0f },
{ 255, 0, 0, 255, -1.0f, 1.0f, 1.0f },
{ 0, 255, 0, 255, -1.0f, -1.0f, -1.0f },
{ 0, 255, 0, 255, -1.0f, 1.0f, -1.0f },
{ 0, 255, 0, 255, 1.0f, 1.0f, -1.0f },
{ 0, 255, 0, 255, 1.0f, -1.0f, -1.0f },
{ 0, 0, 255, 255, -1.0f, 1.0f, -1.0f },
{ 0, 0, 255, 255, -1.0f, 1.0f, 1.0f },
{ 0, 0, 255, 255, 1.0f, 1.0f, 1.0f },
{ 0, 0, 255, 255, 1.0f, 1.0f, -1.0f },
{ 0, 255, 255, 255, -1.0f, -1.0f, -1.0f },
{ 0, 255, 255, 255, 1.0f, -1.0f, -1.0f },
{ 0, 255, 255, 255, 1.0f, -1.0f, 1.0f },
{ 0, 255, 255, 255, -1.0f, -1.0f, 1.0f },
{ 255, 0, 255, 255, 1.0f, -1.0f, -1.0f },
{ 255, 0, 255, 255, 1.0f, 1.0f, -1.0f },
{ 255, 0, 255, 255, 1.0f, 1.0f, 1.0f },
{ 255, 0, 255, 255, 1.0f, -1.0f, 1.0f },
{ 255, 255, 255, 255, -1.0f, -1.0f, -1.0f },
{ 255, 255, 255, 255, -1.0f, -1.0f, 1.0f },
{ 255, 255, 255, 255, -1.0f, 1.0f, 1.0f },
{ 255, 255, 255, 255, -1.0f, 1.0f, -1.0f }
};
for(int i = 0; i < 24; ++i) {
cubeVertices[i].z -= 5;
}
#if 1
glEnable(GL_DEPTH_TEST);
glInterleavedArrays(GL_C4UB_V3F, 0, cubeVertices);
#else
glEnable(GL_DEPTH_TEST);
glEnableClientState(GL_VERTEX_ARRAY);
glEnableClientState(GL_COLOR_ARRAY);
glVertexPointer(3, GL_FLOAT, sizeof(Vertex), &cubeVertices[0].x);
glColorPointer(4, GL_UNSIGNED_BYTE, sizeof(Vertex), cubeVertices);
#endif
glDrawArrays(GL_QUADS, 0, 24);
glfwSwapBuffers(window);
glfwPollEvents();
}
运行结果:
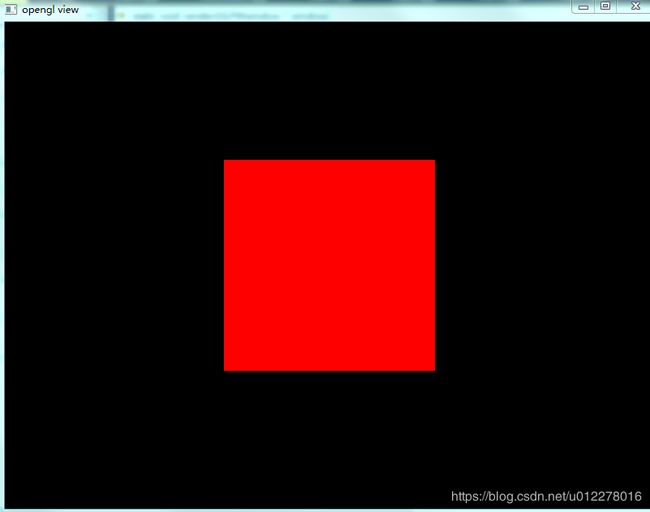