如何测试
SpringBoot
的请求?使用spring-boot-starter-test
这个包即可完成测试,SpringBoot
项目为什么需要测试的意义大猪就不跟小伙伴们多聊了哈,重点放在测试代码上,我们直接开撸。
使用说明
导包gradle项目
compile group: 'com.fasterxml.jackson.jaxrs', name:'jackson-jaxrs-xml-provider',version:'2.5.0'
compile group: 'org.springframework.boot', name: 'spring-boot-starter-test', version: '1.5.3.RELEASE'
导包maven项目
org.springframework.boot
spring-boot-starter-test
1.5.3.RELEASE
test
还需要依赖junit包,大家自行导入
关键核心测试例子
@RunWith(SpringRunner.class)
@SpringBootTest(classes = Application.class, webEnvironment = SpringBootTest.WebEnvironment.RANDOM_PORT)
@EnableAutoConfiguration
public class BBTestAA {
@Autowired
private TestRestTemplate testRestTemplate;
//Application.class 为SpringBoot的启动入口类,每个SpringBoot项目大家都会配置
}
GET请求测试
@Test
public void get() throws Exception {
Map multiValueMap = new HashMap<>();
multiValueMap.put("username","lake");//传值,但要在url上配置相应的参数
ActResult result = testRestTemplate.getForObject("/test/get?username={username}",ActResult.class,multiValueMap);
Assert.assertEquals(result.getCode(),0);
}
POST请求测试
@Test
public void post() throws Exception {
MultiValueMap multiValueMap = new LinkedMultiValueMap();
multiValueMap.add("username","lake");
ActResult result = testRestTemplate.postForObject("/test/post",multiValueMap,ActResult.class);
Assert.assertEquals(result.getCode(),0);
}
文件上传测试
@Test
public void upload() throws Exception {
Resource resource = new FileSystemResource("/home/lake/github/wopi/build.gradle");
MultiValueMap multiValueMap = new LinkedMultiValueMap();
multiValueMap.add("username","lake");
multiValueMap.add("files",resource);
ActResult result = testRestTemplate.postForObject("/test/upload",multiValueMap,ActResult.class);
Assert.assertEquals(result.getCode(),0);
}
文件下载测试
@Test
public void download() throws Exception {
HttpHeaders headers = new HttpHeaders();
headers.set("token","xxxxxx");
HttpEntity formEntity = new HttpEntity(headers);
String[] urlVariables = new String[]{"admin"};
ResponseEntity response = testRestTemplate.exchange("/test/download?username={1}",HttpMethod.GET,formEntity,byte[].class,urlVariables);
if (response.getStatusCode() == HttpStatus.OK) {
Files.write(response.getBody(),new File("/home/lake/github/file/test.gradle"));
}
}
请求头信息传输测试
@Test
public void getHeader() throws Exception {
HttpHeaders headers = new HttpHeaders();
headers.set("token","xxxxxx");
HttpEntity formEntity = new HttpEntity(headers);
String[] urlVariables = new String[]{"admin"};
ResponseEntity result = testRestTemplate.exchange("/test/getHeader?username={username}", HttpMethod.GET,formEntity,ActResult.class,urlVariables);
Assert.assertEquals(result.getBody().getCode(),0);
}
PUT
@Test
public void putHeader() throws Exception {
HttpHeaders headers = new HttpHeaders();
headers.set("token","xxxxxx");
MultiValueMap multiValueMap = new LinkedMultiValueMap();
multiValueMap.add("username","lake");
HttpEntity formEntity = new HttpEntity(multiValueMap,headers);
ResponseEntity result = testRestTemplate.exchange("/test/putHeader", HttpMethod.PUT,formEntity,ActResult.class);
Assert.assertEquals(result.getBody().getCode(),0);
}
DELETE
@Test
public void delete() throws Exception {
HttpHeaders headers = new HttpHeaders();
headers.set("token","xxxxx");
MultiValueMap multiValueMap = new LinkedMultiValueMap();
multiValueMap.add("username","lake");
HttpEntity formEntity = new HttpEntity(multiValueMap,headers);
String[] urlVariables = new String[]{"admin"};
ResponseEntity result = testRestTemplate.exchange("/test/delete?username={username}", HttpMethod.DELETE,formEntity,ActResult.class,urlVariables);
Assert.assertEquals(result.getBody().getCode(),0);
}
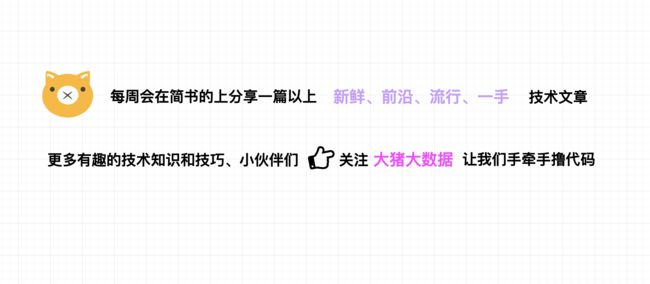