我的目录:sequelize.js
其中../conf/index 是你的配置文件
const Sequelize = require('sequelize');
const config = require('../conf/index');
exports.sequelize = function () {
return new Sequelize(
config.mysql.database,
config.mysql.user,
config.mysql.password, {
'dialect': 'mysql', // 数据库使用mysql
'host': config.mysql.host, // 数据库服务器ip
'port': config.mysql.port, // 数据库运行端口
'timestamp': false, // 这个参数为true是MySQL会自动给每条数据添加createdAt和updateAt字段
'quoteIdentifiers': true,
'freezeTableName': true
}
);
};
配置文件:{
const conf = {
port: 6200, //启动端口 ,这个根据你电脑环境的实际情况来配置
base_url: '0.0.0.0',// 当前api服务器的域名
// host: 'localhost',
mysql: { // mysql数据库信息
host: 'localhost',
port: '3306',
database: 'python', //你可以修改你的数据库名字
user: 'root', //数据库登录账户 你寄己来
password: 'xxx', //数据库登录密码 我就不知道你的是啥啦
charset: 'UTF8mb4'
}
}
module.exports = conf;
}
第二步定义表结构;
我的目录:model/ibook.js
module.exports = function (sequelize, DataTypes) {
return sequelize.define('book', {
id: {
type: DataTypes.INTEGER,
primaryKey: true
},
configId: {
type: DataTypes.STRING(255)
},
count: {
type: DataTypes.INTEGER
},
dataId: {
type: DataTypes.STRING(255)
},
endTime: {
type: DataTypes.DATE
},
name: {
type: DataTypes.STRING(255)
},
order: {
type: DataTypes.STRING(255)
},
startTime: {
type: DataTypes.DATE
},
status: {
type: DataTypes.INTEGER
}
},{
freezeTableName: true,
timestamps: false
})
}
我的目录:model/index.js
第三步:
引入sequelize.js
以及引入表
向外暴露
const db = require("../sequelize/index").sequelize()
const bookModel = db.import("./book.js");
module.exports.bookModel = bookModel;
第四步在路由中使用:
添加数据:
const bookModel = require("../../models/index").bookModel
module.exports = async (ctx, next) => {
const {body: req} = ctx.request;
const {list} = req.result;
try {
for (let i = 0; i < list.length; i++) {
const item = list[i];
await bookModel.create({
configId: item.confId,
count: item.count,
dataId: item.dataId,
endTime: item.endTime || null,
name: item.name,
order: item.order,
startTime: item.startTime,
status: item.status
})
}
ctx.body = 200
} catch (err) {
console.log(err)
ctx.body = 400
}
}
将之前暴露出来的引入.
创建
await bookModel.create({})
查询:
const bookModel = require("../../models/index").bookModel
module.exports = async (ctx, next) => {
const {currentPage: page = 1} = ctx.request.query;
const limit = 10;
try {
const res = await bookModel.findAll({
limit: limit,
offset: (page - 1) * limit
})
ctx.body = {
data: res,
currentPage: page,
limit
}
} catch (err) {
console.log(err)
ctx.body = 400
}
}
查询 await bookModel.findAll
最后目录结构
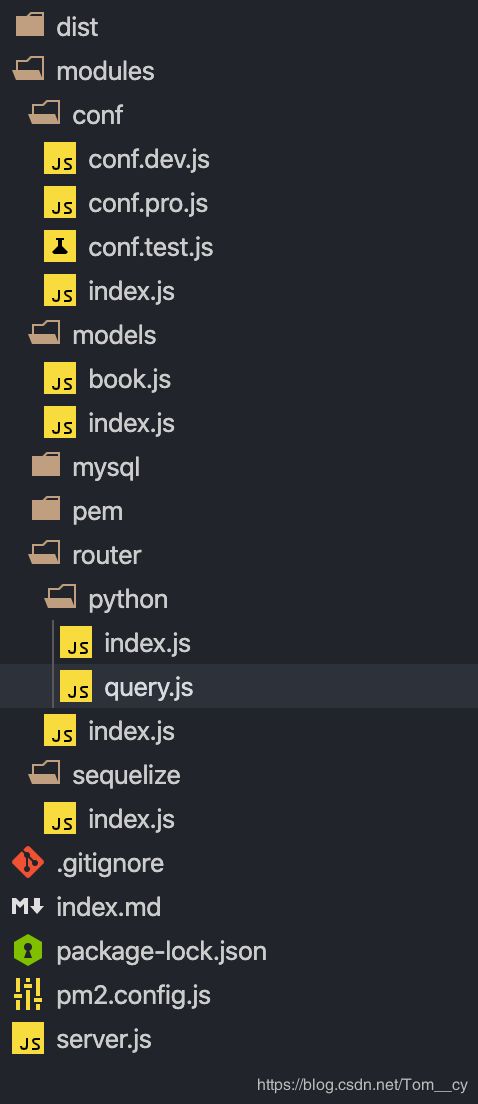