十五天的时间,刷完了所有的简单题,避免遗忘,所以开始简单题的二刷,第一遍刷题的时候过得速度比较快,因为我觉得基础不好的我,不要硬着头皮去想最优的方法,而是应该尽量去学一些算法思想,所以每道题只给自己5-10分钟的时间想,想不出来的就去找相关的答案,所以刷的比较快。二刷的时候按照leetcode官方给出的题目分类展开,同时,将解题思路记录于加深印象。
想要一起刷题的小伙伴,我们一起加油吧!
我的github连接:https://github.com/princewen/leetcode_python
136. Single Number
利用异或,两个相同的数的异或为0。
class Solution(object):
def singleNumber(self, nums):
"""
:type nums: List[int]
:rtype: int
"""
res = 0
for i in nums:
res = res ^ i
return res
190. Reverse Bits
利用十进制转二进制的方法,考虑十进制13
13 / 2 = 6 ----1
6 /2 = 3 ----0
3 /2 = 1 ----1
1 /2 = 0 ----1
二进制是1101,前面补全0,而反转的二进制是1011,后面补全0
所以我们只需要按照除的顺序依次进入数组,后面不组32位用0补齐即可。
class Solution:
# @param n, an integer
# @return an integer
def reverseBits(self, n):
stack = []
while n:
stack.append(n % 2)
n = n / 2
while len(stack) < 32:
stack.append(0)
ret = 0
for num in stack:
ret = ret * 2 + num
return ret
191. Number of 1 Bits
这里用到的二进制知识是:一个数与比自己小1的数按位与运算,会将自己所对应的二进制数的最后一个1变为0。
考虑如下的例子: 13的二进制数为1101,12的二进制数为1100.则 (1101)& (1100) -->(1100);1100代表的数是10,9的二进制数为1001,则:1100 & 1001 -->1000.依次类推.
class Solution(object):
def hammingWeight(self, n):
"""
:type n: int
:rtype: int
"""
count = 0
while n:
n = n & (n-1)
count = count + 1
return count
231. Power of Two
跟191题一样的思路,2的倍数的对应二进制数只有1位是1。
class Solution(object):
def isPowerOfTwo(self, n):
"""
:type n: int
:rtype: bool
"""
return n>0 and not (n & n-1)
268. Missing Number
之前这道题介绍过一种等差数列求和公式的思路,现在我们用前两道题的思路进行求解
class Solution(object):
def missingNumber(self, nums):
"""
:type nums: List[int]
:rtype: int
"""
result = len(nums)
for index,value in enumerate(nums):
result ^= index
result ^= value
return result
342. Power of Four
4的倍数首先要满足2的倍数的条件,然后他减1要是3的倍数。
class Solution(object):
def isPowerOfFour(self, num):
"""
:type num: int
:rtype: bool
"""
return num>0 and (num & num-1)==0 and (num-1)%3==0
371. Sum of Two Integers
思路:强大的位运算,不能用+ and -,考虑位运算,按位加如果两位都是0,则取0,如果两位中有一位是1,则是1,如果两位都是1,则是10,那么我们可以用异或来表示这种关系,但是两个都是1的情况下,还需要在前一位补一个1,所以再用按位与并移1位来表示需要补上的一个1
例如考虑四位二进制位的3(0011)和 5(0101)相加:
第一轮:
a = 0011 ^ 0101 = 0110
b = 0011 & 0101 = 0001 << 1 = 0010
第二轮:
a = 0110 ^ 0010 = 0100
b = 0110 & 0010 = 0010 << 1 = 0100
第三轮:
a = 0100 ^ 0100 = 0000
b = 0100 & 0100 = 0100 << 1 = 1000
最后一轮:
a = 0000 ^ 1000 = 1000
b = 0000 & 1000 = 0000迭代终止,返回a
class Solution(object):
def getSum(self, a, b):
"""
:type a: int
:type b: int
:rtype: int
"""
# 32 bits integer max
MAX = 0x7FFFFFFF
# 32 bits interger min
MIN = 0x80000000
# mask to get last 32 bits
mask = 0xFFFFFFFF
while b != 0:
# ^ get different bits and & gets double 1s, << moves carry
a, b = (a ^ b) & mask, ((a & b) << 1) & mask
# if a is negative, get a's 32 bits complement positive first
# then get 32-bit positive's Python complement negative
"""有疑问,python把有符号数当作无符号数处理"""
return a if a <= MAX else ~(a ^ mask)
389. Find the Difference
可以用hash table,当然更简单的还是利用两个相同的数异或值为0。
class Solution(object):
def findTheDifference(self, s, t):
"""
:type s: str
:type t: str
:rtype: str
"""
res = 0
for i in s+t:
res ^= ord(i)
return chr(res)
461.Hamming Distance
先取异或,然后利用一个数与比自己小1的数按位与运算,会将自己所对应的二进制数的最后一个1变为0这个特点。
class Solution(object):
def hammingDistance(self, x, y):
"""
:type x: int
:type y: int
:rtype: int
"""
t = x ^ y
count = 0
while t != 0:
count = count + 1
t = t & (t-1)
return count
476. Number Complement
利用与二进制位全1的数进行按位异或运算是将二进制位对位取反的性质。
class Solution(object):
def findComplement(self, num):
"""
:type num: int
:rtype: int
"""
i=1
while i<=num:
i = i << 1
return (i-1) ^ num
总结一下,简单题中涉及的二进制的基本知识点有:
1、两个相同的数,按位异或值为0
2、一个数与比他小1的数按位与运算,相当于把该数的二进制位中的最后一位1变为0
3、二进制的加法,可以用异或和按位与运算来解决,参照371题。
如果你喜欢我写的文章,可以帮忙给小编点个赞或者加个关注,我一定会互粉的!
如果大家对leetcode感兴趣,欢迎跟小编进行交流,小编微信为sxw2251,加我要写好备注哟!:
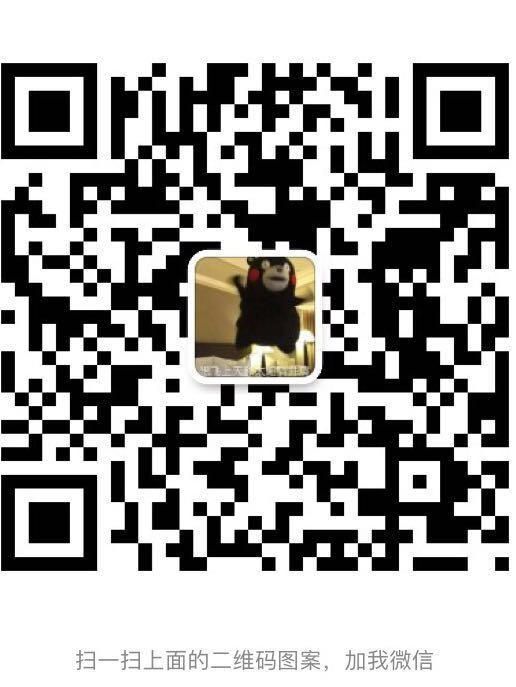