首页下载APP
SpringBoot发送邮件
BestbpF
SpringBoot发送邮件
BestbpF
0.3982018.09.15 11:03:43字数 698阅读 6,870
简述
在日常工作开发中,发送邮件功能有时需要我们去开发使用,这里首先介绍以下与发送接受邮件相关的一些协议:
发送邮件:SMPT、MIME,是一种基于"推"的协议,通过SMPT协议将邮件发送至邮件服务器,MIME协议是对SMPT协议的一种补充,如发送图片附件等
接收邮件:POP、IMAP,是一种基于"拉"的协议,收件人通过POP协议从邮件服务器拉取邮件
嘿嘿,讲的太简单了,具体资料大家可以上网或看书来进一步了解,很多的哦
使用SpringBoot发送邮件
使用SpirngBoot发送邮件,首先需要引入mail依赖
然后在配置文件application.properties中加入相关配置信息
#这里以QQ邮箱为例#QQ邮箱服务器spring.mail.host=smtp.qq.com#你的QQ邮箱账户[email protected]#你的QQ邮箱第三方授权码spring.mail.password=yourPassword#编码类型spring.mail.default-encoding=UTF-8
开启邮箱第三方支持以及获取授权码(以QQ邮箱为例)
打开QQ邮箱,点击设置
选择账户,并下拉找到
开启第一个或前两个,然后点击生成授权码,写到SpringBoot配置文件中
开始撸代码
代码可到springboot2.x发送邮件中下载
代码以自定义成员方法为例,需要准备
privatefinalLogger logger=LoggerFactory.getLogger(MailServiceImpl.class);@Value("${spring.mail.username}")//使用@Value注入application.properties中指定的用户名privateString from;@Autowired//用于发送文件privateJavaMailSender mailSender;
定义发送邮件接口
public interfaceMailService{/**
* 发送普通文本邮件
* @param to 收件人
* @param subject 主题
* @param content 内容
*/voidsendSimpleMail(String to,String subject,String content);/**
* 发送HTML邮件
* @param to 收件人
* @param subject 主题
* @param content 内容(可以包含等标签)
*/voidsendHtmlMail(String to,String subject,String content);/**
* 发送带附件的邮件
* @param to 收件人
* @param subject 主题
* @param content 内容
* @param filePath 附件路径
*/voidsendAttachmentMail(String to,String subject,String content,String filePath);/**
* 发送带图片的邮件
* @param to 收件人
* @param subject 主题
* @param content 文本
* @param rscPath 图片路径
* @param rscId 图片ID,用于在标签中使用,从而显示图片
*/voidsendInlineResourceMail(String to,String subject,String content,String rscPath,String rscId);}
【发送普通邮件】
我们这里的普通邮件是指最为普通的纯文本邮件
publicvoidsendSimpleMail(String to,String subject,String content){SimpleMailMessage message=newSimpleMailMessage();message.setTo(to);//收信人message.setSubject(subject);//主题message.setText(content);//内容message.setFrom(from);//发信人mailSender.send(message);}
【发送HTML文件】
HTML文件就是指在文件内容中可以添加等标签,收件人收到邮件后显示内容也和网页一样,比较丰富多彩
publicvoidsendHtmlMail(String to,String subject,String content){logger.info("发送HTML邮件开始:{},{},{}",to,subject,content);//使用MimeMessage,MIME协议MimeMessage message=mailSender.createMimeMessage();MimeMessageHelper helper;//MimeMessageHelper帮助我们设置更丰富的内容try{helper=newMimeMessageHelper(message,true);helper.setFrom(from);helper.setTo(to);helper.setSubject(subject);helper.setText(content,true);//true代表支持htmlmailSender.send(message);logger.info("发送HTML邮件成功");}catch(MessagingException e){logger.error("发送HTML邮件失败:",e);}}
【发送带附件的邮件】
带附件的邮件在HTML邮件上添加一些参数即可
publicvoidsendAttachmentMail(String to,String subject,String content,String filePath){logger.info("发送带附件邮件开始:{},{},{},{}",to,subject,content,filePath);MimeMessage message=mailSender.createMimeMessage();MimeMessageHelper helper;try{helper=newMimeMessageHelper(message,true);//true代表支持多组件,如附件,图片等helper.setFrom(from);helper.setTo(to);helper.setSubject(subject);helper.setText(content,true);FileSystemResource file=newFileSystemResource(newFile(filePath));String fileName=file.getFilename();helper.addAttachment(fileName,file);//添加附件,可多次调用该方法添加多个附件 mailSender.send(message);logger.info("发送带附件邮件成功");}catch(MessagingException e){logger.error("发送带附件邮件失败",e);}}
【发送带图片的邮件】
publicvoidsendInlineResourceMail(String to,String subject,String content,String rscPath,String rscId){logger.info("发送带图片邮件开始:{},{},{},{},{}",to,subject,content,rscPath,rscId);MimeMessage message=mailSender.createMimeMessage();MimeMessageHelper helper;try{helper=newMimeMessageHelper(message,true);helper.setFrom(from);helper.setTo(to);helper.setSubject(subject);helper.setText(content,true);FileSystemResource res=newFileSystemResource(newFile(rscPath));helper.addInline(rscId,res);//重复使用添加多个图片mailSender.send(message);logger.info("发送带图片邮件成功");}catch(MessagingException e){logger.error("发送带图片邮件失败",e);}}
测试
使用SpirngBoot Junit Test进行简单测试
importorg.junit.Test;importorg.junit.runner.RunWith;importorg.springframework.beans.factory.annotation.Autowired;importorg.springframework.boot.test.context.SpringBootTest;importorg.springframework.test.context.junit4.SpringRunner;importorg.thymeleaf.TemplateEngine;importorg.thymeleaf.context.Context;@RunWith(SpringRunner.class)@SpringBootTestpublicclassServiceTest{@AutowiredprivateMailService mailService;@AutowiredprivateTemplateEngine templateEngine;/**
* 发送简单纯文本邮件
*/@TestpublicvoidsendSimpleMail(){mailService.sendSimpleMail("[email protected]","发送邮件测试","大家好,这是我用springboot进行发送邮件测试");}/**
* 发送HTML邮件
*/@TestpublicvoidsendHtmlMail(){String content="
"+"大家好,这是springboot发送的HTML邮件"+"
";mailService.sendHtmlMail("[email protected]","发送邮件测试",content);}/*** 发送带附件的邮件
*/@TestpublicvoidsendAttachmentMail(){String content="
"+"大家好,这是springboot发送的HTML邮件,有附件哦"+"
";String filePath="your file path";mailService.sendAttachmentMail("[email protected]","发送邮件测试",content,filePath);}/*** 发送带图片的邮件
*/@TestpublicvoidsendInlineResourceMail(){String rscPath="your picture path";String rscId="001";String content="
"+"大家好,这是springboot发送的HTML邮件,有图片哦"+"
"+"如何发送模板邮件?
这里我们提一下很常见的模板邮件,比如说我们注册账户,登录认证所收到的邮件即为模板邮件,特点就是内容不变,只是一些特点信息比如说验证码、认证链接等是动态变化的。
以Thymeleaf模板引擎为例来讲解(具体语法自行了解)
在template文件夹下创建emailTemplate.html
在模板页面中,id是动态变化的,需要我们传参设置,其实就是传参后,将页面解析为HTML字符串,作为我们邮件发送的主体内容
【测试】
/**
* 指定模板发送邮件
*/@TestpublicvoidtestTemplateMail(){//向Thymeleaf模板传值,并解析成字符串Context context=newContext();context.setVariable("id","001");String emailContent=templateEngine.process("emailTemplate",context);mailService.sendHtmlMail("[email protected]","这是一个模板文件",emailContent);}
具体测试结果大家自行测试!
"小礼物走一走,来关注我"
还没有人赞赏,支持一下
BestbpFgithub:https://github.com/BestbpF
如有问题,欢迎指正!
...
总资产61 (约6.06元)共写了3.8W字获得218个赞共65个粉丝
全部评论2
RainBow11212
3楼 08.06 15:17
应该是发送附件中文名称过长就会乱码
RainBow11212
2楼 08.06 15:10
发送亚索文件乱码
被以下专题收入,发现更多相似内容
Java程序员SpringBoot
推荐阅读更多精彩内容
使用SpringBoot发送邮件
前言 在项目中可能会碰到发送邮件的需求,这时,我们就可以使用SpringBoot的mail模块进行快速的开发。 配...
sT丶阅读 217评论 0赞 0
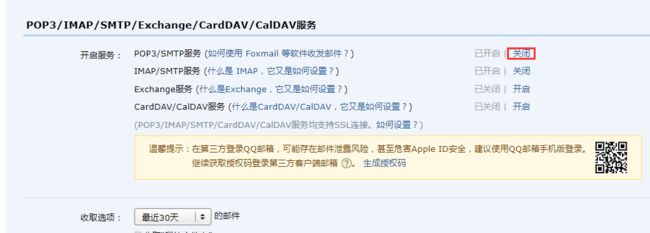
springboot发送邮件,java结合jms发邮件
近期学习java后台开发,学到了springboot发送邮件。感觉这也算是一个很实用的知识点,就在这里总结下,方便...
编程小石头阅读 258评论 0赞 5
Spring Cloud
Spring Cloud为开发人员提供了快速构建分布式系统中一些常见模式的工具(例如配置管理,服务发现,断路器,智...
卡卡罗2017阅读 71,417评论 12赞 116
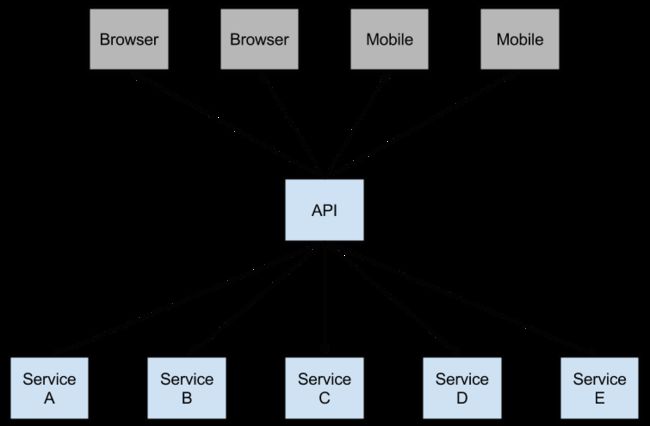
Python3实现自动定时发送邮件功能
Python SMTP发送邮件 SMTP是发送邮件的协议,Python内置对SMTP的支持,可以发送纯文本邮件、H...
SamBrother阅读 5,492评论 4赞 12
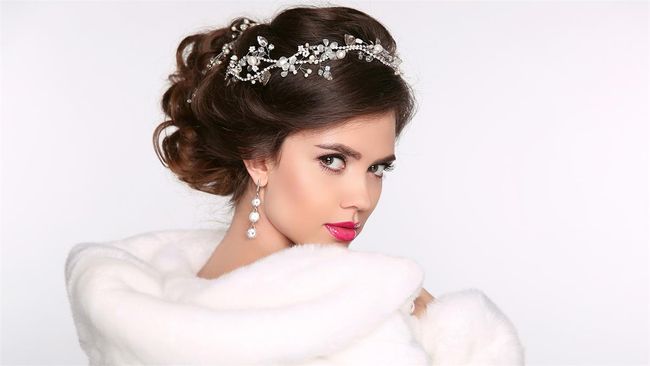
当你老了
当你老了 你要知道 你要适时假装木讷 不要总是敏感哀伤 不要总是失落惆怅 多疑孩子的态度话语 唠叨子孙的衣食住行 ...
陶缨子阅读 147评论 16赞 27
广告
BestbpF
总资产61 (约6.06元)
子类复写父类方法原则
阅读 3
记录一下leetcode中的sql题目(免费部分)
阅读 119
记录一次AOP存在的问题
阅读 37
推荐阅读
胡歌又获点赞:你有教养的样子,真帅
阅读 7,778
张梓琳3岁女儿曝光,看完后我酸酸酸酸酸了
阅读 7,864
巩俐,你凭什么演郎平?
阅读 9,978
为什么被你们吹爆了的《哪吒》,我只看到了满屏的尴尬?
阅读 203,871
58岁大妈装萝莉真相曝光:网上女神为什么这么多?看完这些你就懂了
阅读 35,004
广告