项目介绍
使用到的框架主要包括:JDK + servlet + sqljdbc4
数据库:SQL Server
服务器软件:tomcat
开发工具:eclipse + maven
功能点如下:
1)添加学生(已完成)
2)学生列表(已完成)
3)列表分页显示(未完成)
4)学生详情(已完成)
5)修改学生(已完成)
6)删除学生(已完成)
7)搜索学生(未完成)
8)后台出现异常界面能够给出简单清晰的错误提示(未完成)
9)表单校验的JS功能(未完成)
项目地址
https://github.com/yangzc23/stuweb
思路
1)HttpServlet的作用:对http请求进行处理(service方法)
2)HttpServletRequest表示请求(获取请求里面参数的内容)
3)HttpServletResponse表示响应(发送响应数据给浏览器)
学生注册功能的缺陷
问题描述:输入错误或者输入为空,点击保存,将后台错误暴露给了用户(用户体验差)
问题类型:易用性缺陷
修改建议:给出简单清晰的错误提示
创建学生表
CREATE TABLE STUDENT(
SNO NUMERIC(4) PRIMARY KEY IDENTITY(1001,1), --学号
SNAME VARCHAR(20), --姓名
GENDER CHAR(2), --性别
BIRTH DATE, --生日
PHOTO_URL VARCHAR(50) --照片路径
);
开发环境准备(从SVN服务器上下载基础代码)
依赖配置
com.microsoft.sqlserver
sqljdbc4
4.0
javax.servlet
servlet-api
2.5
provided
commons-fileupload
commons-fileupload
1.3.1
org.apache.commons
commons-io
1.3.2
学生列表界面实现
配置servlet
hello
com.testin.examples.HelloServlet
hello
/welcome
后台代码
package com.testin.examples;
import java.io.IOException;
import java.io.PrintWriter;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.testin.utils.DBUtil;
public class HelloServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
@Override
public void service(HttpServletRequest req, HttpServletResponse resp) {
PrintWriter pw = null;
Connection conn = null;
PreparedStatement stmt = null;
//解决响应正文内容包含中文会出现乱码的问题
resp.setContentType("text/html;charset=utf-8");
StringBuilder str = new StringBuilder();
str.append("学号 姓名 性别 生日 操作 ");
try {
pw = resp.getWriter();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
try {
conn = DBUtil.getConnection();
stmt = conn.prepareStatement("SELECT * FROM STUDENT");
ResultSet rs = stmt.executeQuery();
while(rs.next()) {
//System.out.println(rs.getInt(1)+","+rs.getString(2)+","+rs.getString(3)+","+rs.getInt(4));
str.append(""+rs.getInt(1)+" "+
""+rs.getString(2)+" "+""+rs.getString(3)+" "+
""+rs.getDate(4)+" "+
"详情 "+
"编辑 "+
"删除"+
" ");
}
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
try {
conn.close();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
pw.println("欢迎使用学生管理系统
"+str+"
");
}
}
学生注册界面实现
配置servlet
servlet2
com.testin.examples.NewServlet
servlet2
/new
后台代码
package com.testin.examples;
import java.io.IOException;
import java.io.PrintWriter;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class NewServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
@Override
public void service(HttpServletRequest req, HttpServletResponse resp) throws ServletException,IOException{
//解决响应正文内容包含中文会出现乱码的问题
resp.setContentType("text/html;charset=utf-8");
PrintWriter pw = resp.getWriter();
//
pw.println(""+
""+
""+
"注册学生信息
"+
"");
}
}
头像上传功能实现
配置servlet
servlet8
com.testin.examples.UploadFileServlet
servlet8
/upload/file
后台代码
package com.testin.examples;
import java.io.FileOutputStream;
import java.io.InputStream;
import java.io.PrintWriter;
import java.util.List;
import java.util.UUID;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.apache.commons.fileupload.FileItem;
import org.apache.commons.fileupload.disk.DiskFileItemFactory;
import org.apache.commons.fileupload.servlet.ServletFileUpload;
public class UploadFileServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
@Override
public void service(HttpServletRequest req, HttpServletResponse resp) {
PrintWriter pw = null;
//解决响应正文内容包含中文会出现乱码的问题
resp.setContentType("application/json;charset=utf-8");
try{
pw = resp.getWriter();
//1.得到解析器工厂
DiskFileItemFactory factory = new DiskFileItemFactory();
//2.得到解析器
ServletFileUpload upload = new ServletFileUpload(factory);
//3.判断上传表单的类型
if(!ServletFileUpload.isMultipartContent(req)){
//上传表单为普通表单,则按照传统方式获取数据即可
return;
}
//为上传表单,则调用解析器解析上传数据
List list = upload.parseRequest(req); //FileItem
//遍历list,得到用于封装第一个上传输入项数据fileItem对象
for(FileItem item : list){
if(item.isFormField()){
//得到的是普通输入项
String name = item.getFieldName(); //得到输入项的名称
String value = item.getString();
System.out.println(name + "=" + value);
}else{
//得到上传输入项
String filename = item.getName(); //得到上传文件名 C:\Documents and Settings\ThinkPad\桌面\1.txt
filename = filename.substring(filename.lastIndexOf("\\")+1);
String extName = filename.substring(filename.lastIndexOf("."));//.jpg
String uuid = UUID.randomUUID().toString().replace("-", "");
//新名称
String newName = uuid+extName;//在这里用UUID来生成新的文件夹名字,这样就不会导致重名
InputStream in = item.getInputStream(); //得到上传数据
int len = 0;
byte buffer[]= new byte[1024];
String savepath = this.getServletContext().getRealPath("/upload");
FileOutputStream out = new FileOutputStream(savepath + "\\" + newName); //向upload目录中写入文件
while((len=in.read(buffer))>0){
out.write(buffer, 0, len);
}
in.close();
out.close();
pw.println("{\"result\":\"success\",\"message\":\"上传成功!\",\"url\":\"upload/"+newName+"\"}");
}
}
}catch (Exception e) {
e.printStackTrace();
pw.print("{\"result\":\"fail\",\"message\":\"上传失败!\",\"url\":\"\"}");
}
}
}
JS代码
function f(){
var file = $("#photo").get(0).files[0];
var formData = new FormData();
formData.append("source",file);
$.ajax({
url:"upload/file",
type:"post",
dataType:"json",
cache:false,
data:formData,
contentType:false,
processData: false,
success:function(data){
if(data.result=="success"){
$("#photo2").attr("src",data.url);
$("#filePath").val(data.url);
}
console.log("hello test");
}
});
}
学生信息保存实现
配置servlet
servlet3
com.testin.examples.SaveServlet
servlet3
/save
后台代码
package com.testin.examples;
import java.io.IOException;
import java.io.PrintWriter;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.testin.utils.DBUtil;
public class SaveServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
@Override
public void service(HttpServletRequest req, HttpServletResponse resp) throws ServletException,IOException{
Connection conn = null;
PreparedStatement stmt = null;
//解决响应正文内容包含中文会出现乱码的问题
resp.setContentType("text/html;charset=utf-8");
//解决请求参数内容包含中文会出现乱码的问题
req.setCharacterEncoding("utf-8");
PrintWriter pw = resp.getWriter();
//
String sname = req.getParameter("sname");
//
String gender = req.getParameter("gender").equals("0")?"女":"男";
//
String birth = req.getParameter("birth");
//
String filePath = req.getParameter("filePath");
//
try {
conn = DBUtil.getConnection();
stmt = conn.prepareStatement("INSERT INTO STUDENT(SNAME,GENDER,BIRTH,PHOTO_URL) VALUES(?,?,?,?)");
stmt.setString(1, sname);
stmt.setString(2, gender);
stmt.setString(3, birth);
stmt.setString(4, filePath);
stmt.executeUpdate();
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
try {
conn.close();//关闭数据库的连接
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
pw.println(""
+ ""
+ ""
+ ""
+ "注册成功!页面即将跳转
"
+ "");
}
}
学生信息编辑界面
配置servlet
servlet4
com.testin.examples.EditServlet
servlet4
/edit
后台代码
package com.testin.examples;
import java.io.IOException;
import java.io.PrintWriter;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.testin.utils.DBUtil;
public class EditServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
@Override
public void service(HttpServletRequest req, HttpServletResponse resp) throws ServletException,IOException{
Connection conn = null;
PreparedStatement stmt = null;
//解决响应正文内容包含中文会出现乱码的问题
resp.setContentType("text/html;charset=utf-8");
//
PrintWriter pw = resp.getWriter();
//
int sid = Integer.parseInt(req.getParameter("sid"));
//
try {
conn = DBUtil.getConnection();
stmt = conn.prepareStatement("SELECT * FROM STUDENT WHERE SNO=?");
stmt.setInt(1, sid);
ResultSet rs = stmt.executeQuery();
if(rs.next()) {
pw.println(""+
""+
""+
"修改学生信息
"+
"");
}
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
try {
conn.close();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
}
学生信息更新功能
配置servlet
servlet5
com.testin.examples.UpdateServlet
servlet5
/update
后台代码
package com.testin.examples;
import java.io.IOException;
import java.io.PrintWriter;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.testin.utils.DBUtil;
public class UpdateServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
@Override
public void service(HttpServletRequest req, HttpServletResponse resp) throws ServletException,IOException{
Connection conn = null;
PreparedStatement stmt = null;
//解决响应正文内容包含中文会出现乱码的问题
resp.setContentType("text/html;charset=utf-8");
//解决请求参数内容包含中文会出现乱码的问题
req.setCharacterEncoding("utf-8");
PrintWriter pw = resp.getWriter();
//获取学号
int sno = Integer.parseInt(req.getParameter("sno"));
//
String sname = req.getParameter("sname");
//
String gender = req.getParameter("gender").equals("0")?"女":"男";
//
String birth = req.getParameter("birth");
//
String filePath = req.getParameter("filePath");
//
try {
conn = DBUtil.getConnection();
stmt = conn.prepareStatement("UPDATE STUDENT SET SNAME=?,GENDER=?,BIRTH=?,PHOTO_URL=? WHERE SNO=?");
stmt.setString(1, sname);
stmt.setString(2, gender);
stmt.setString(3, birth);
stmt.setString(4, filePath);
stmt.setInt(5, sno);
stmt.executeUpdate();
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
try {
conn.close();//关闭数据库的连接
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
pw.println(""
+ ""
+ ""
+ ""
+ "修改成功!页面即将跳转
"
+ "");
}
}
学生信息删除功能
配置servlet
servlet6
com.testin.examples.DeleteServlet
servlet6
/delete
后台代码
package com.testin.examples;
import java.io.IOException;
import java.io.PrintWriter;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.testin.utils.DBUtil;
public class DeleteServlet extends HttpServlet {
/**
*
*/
private static final long serialVersionUID = 1L;
@Override
public void service(HttpServletRequest req, HttpServletResponse resp) throws ServletException,IOException{
Connection conn = null;
PreparedStatement stmt = null;
//解决响应正文内容包含中文会出现乱码的问题
resp.setContentType("text/html;charset=utf-8");
//解决请求参数内容包含中文会出现乱码的问题
req.setCharacterEncoding("utf-8");
PrintWriter pw = resp.getWriter();
//获取学号
int sid = Integer.parseInt(req.getParameter("sid"));
try {
conn = DBUtil.getConnection();
stmt = conn.prepareStatement("DELETE FROM STUDENT WHERE SNO = ?");
stmt.setInt(1, sid);
stmt.executeUpdate();
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
try {
conn.close();//关闭数据库的连接
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
pw.println(""
+ ""
+ ""
+ ""
+ "删除成功!页面即将跳转
"
+ "");
}
}
参考资料
[01] servlet入门教程
https://www.runoob.com/servlet/servlet-tutorial.html
[02] JavaEE官方API文档
https://docs.oracle.com/javaee/7/api/toc.htm
[03] JavaScript Timing 事件
http://www.w3school.com.cn/js/js_timing.asp
[04] JSP和Servlet传值中文乱码解决
https://blog.csdn.net/qq_39444779/article/details/78368563
[05] No compiler is provided in this environment. Perhaps you are running on a JRE rather than a JDK?
https://blog.51cto.com/westsky/1584824
[06] maven pom详解
https://blog.csdn.net/zz_lk_xx/article/details/85870848
[07] 使用Maven插件启动tomcat服务
https://blog.csdn.net/ron03129596/article/details/79161139
[08] Eclipse安装svn插件的几种方式
https://www.cnblogs.com/keyi/p/8507508.html
[09] 在Eclipse上使用SVN,安装、提交、拉取代码、解决冲突等操作
https://blog.csdn.net/m0_37758648/article/details/82905282
[10] Eclipse SVN冲突详细解决方案
https://www.cnblogs.com/jpfss/p/9007981.html
[11] jquery+easyui实现页面布局和增删改查操作(SSH2框架支持)
https://blessht.iteye.com/blog/1069749/
[12] 文件的上传(表单上传和ajax文件异步上传)
https://www.cnblogs.com/fengxuehuanlin/p/5311648.html
[13] java实现动态上传多个文件并解决文件重名问题
https://www.cnblogs.com/skycodefamily/p/5344941.html
[14] Java文件上传细讲
https://www.cnblogs.com/Java3y/p/8428591.html
[15] GitGUI出现 Unable to obtain your identity
https://blog.csdn.net/HaleyLiu123/article/details/78823245
[16] 使用AJAX实现数据的增删改查
https://blog.csdn.net/qq_36186690/article/details/81230722
微信扫一扫关注该公众号【测试开发者部落】
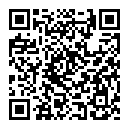
点击链接加入群聊【软件测试学习交流群】
https://jq.qq.com/?_wv=1027&k=5eVEhfN
软件测试学习交流QQ群号: 511619105