import os
import sys
import numpy as np
import cv2
from matplotlib import pyplot as plt
rawPath = os.path.abspath(__file__)
currentFile = os.path.basename(sys.argv[0])
dataPath = rawPath[:rawPath.find(currentFile)] + r'static\\'
def draw_circle(event, x, y, flags, param):
"""
在图片上双击过的位置绘制一个圆圈
:return:
"""
if event == cv2.EVENT_LBUTTONDBLCLK:
cv2.circle(img, (x, y), 100, (255, 255, 255), -1)
img = np.zeros((500, 500, 3), np.uint8)
cv2.namedWindow('image')
cv2.setMouseCallback('image', draw_circle)
while 1:
cv2.imshow('image', img)
if cv2.waitKey(20) == 27:
break
cv2.destroyAllWindows()
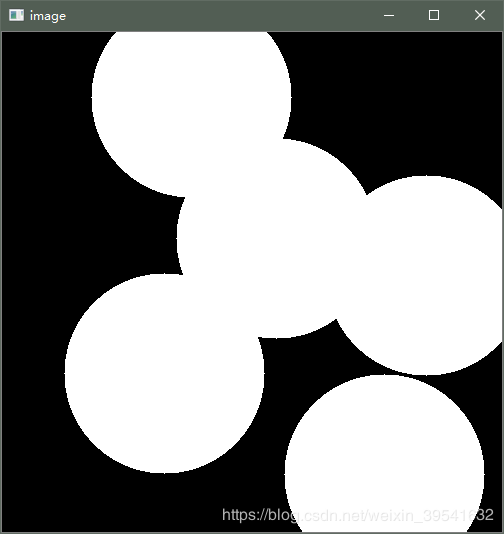
drawing = False
mode = True
ix, iy = -1, -1
def draw_circle(event,x, y, flags, param):
global ix, iy, drawing, mode
if event == cv2.EVENT_LBUTTONDOWN:
drawing = True
ix, iy = x, y
elif event == cv2.EVENT_MOUSEMOVE and flags == cv2.EVENT_FLAG_LBUTTON:
if drawing == True:
if mode == True:
cv2.rectangle(img, (ix, iy), (x, y), (0, 255, 0), -1)
else:
cv2.circle(img, (x, y), 3, (0, 0, 255), -1)
elif event == cv2.EVENT_LBUTTONUP:
drawing = False
if mode == True:
cv2.rectangle(img, (ix, iy), (x, y), (0, 255, 0), -1)
else:
cv2.circle(img, (x, y), 5, (0, 0, 255), -1)
img = np.zeros((512, 512, 3), np.uint8)
cv2.namedWindow('image')
cv2.setMouseCallback('image', draw_circle)
while 1:
cv2.imshow('image', img)
k = cv2.waitKey(1)
if k == ord('m'):
mode = not mode
elif k == 27:
break
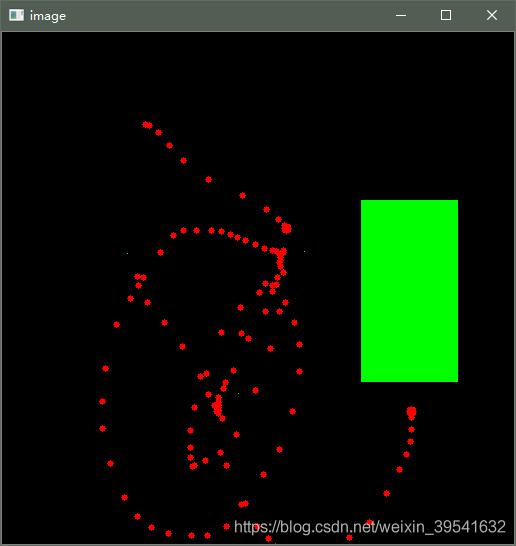